Summary of the Steam Web API#
The Steam Web API is a collection of web-based interfaces provided by Valve Corporation, meant for developers to utilize Steam’s extensive network. The API offers access to a multitude of Steam features. This includes user and friends data, achievements, leaderboards, and more. Some key aspects of the API include:
-
Data formats: The API supports multiple data formats such as XML, JSON, and VDF (Valve Data Format), making it versatile for different platforms and applications.
-
Rate limiting: There's a limit of 100,000 requests per day. This ensures fair usage and prevents abuse.
-
Security: The Steam Web API uses API key authentication. Read on to learn how to get a key.
-
Pricing: The API seems to be completely free for the community to use.
The list of available APIs is quite small however, and most features of Steam are not accessible through this API.
The Steam Partner APIs (Steamworks Web API)#
The reason the Steam Web API is so limited is that its actually part of a much larger set of APIs known as the Steamworks Web API. There's a whole separate suite of APIs that are restricted to Steam partners (you can get a publisher/partner key by following these steps) including microtransactions, player services, and item marketplace.
Enter the Unofficial Steam APIs#
Unfortunately, not everyone can become a Steam partner. Developers have slowly been piecing together the "hidden" or "undocumented" API for Steam to get access to more of the platform's data. One noteworthy resource is the Internal Steam Web API documentation. This provides information on various services used internally from within the Steam app to fetch and mutate data. This documentation isn't officially supported by Valve, and using these undocumented APIs may carry certain risks.
For Comprehensive Steam Data - Consider 3rd Party#
For those looking to access a wide variety of Steam data without having to deal with multiple APIs or complex integrations, third-party services like Steamwebapi offer comprehensive solutions. These services provide data on games, users, inventories, achievements, trades, and more — all from a single API endpoint. Note that these APIs are NOT free, unlike the official and unofficial Steam APIs.
Does Steam offer an OpenAPI/Swagger Specification?#
Unfortunately, Steam does not offer an OpenAPI or Swagger spec for their APIs - and their API documentation is in an not-so-beautiful wiki. Luckily for us, we were able to find a full list of API endpoints which we used to create a Steam OpenAPI repository. You can use those OpenAPI specs directly, or view them in Zudoku for a better reading experience. Public API link, Partner API link.
View API InBenefits and Use Cases of the Steam Web API#
The Steam Web API offers a limited number of APIs covering the following
- Latest news about a game
- Global achievement data for a game
- Access to social data like public profiles and friends lists
- Information about your gaming library, including your owned games, recently played games, achievements, and stats
Some potential usecases include:
- Leveraging game news as a gaming retailer in order to provide more information to prospective buyers
- Comparing your achievements and gaming stats to friends and others globally
What about the Steamworks Partner API?#
- Both the hidden API and the Steamworks Partner API provide much more functionality around the Steam marketplace, item prices, and more that could be leveraged by avid item traders
- Likewise, game developers can benefit by using the Steamworks API for managing microtransactions, matchmaking, game inventories, and other essential services
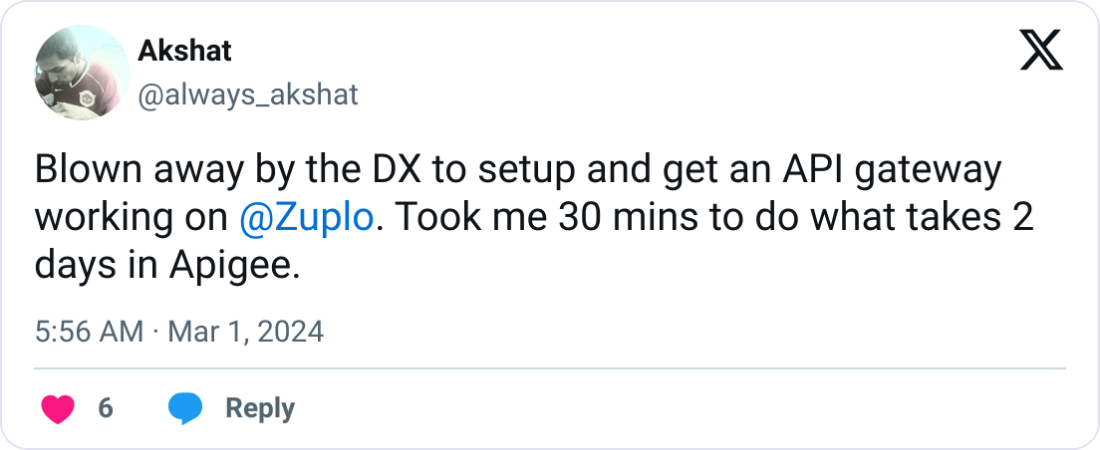
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreHow to Get Access to the Steam Web API#
To get access to the Steam Web API, follow these detailed steps:
- Prerequisites: You must have a Steam account that's not limited. Limited accounts have restricted access to certain features and can't hold funds in their Steam Wallet. To remove these limitations, a $5 (USD) equivalent must have been spent in the Steam store.
- Set Up Steam Mobile Guard: You need to have 2FA set up using the Steam app on your mobile device
- Requesting the API Key: Go to the Steam API Key Registration page. Enter the necessary domain details, agree to the terms and conditions, and register for your key.
- Obtaining the API Key: Once registered, your API key will be displayed.
You should copy and store it securely.
- Configuring and Securely Use the API Key: Add the API key to your server or app configuration. Be cautious with sharing this key.
- Deactivating the API Key: If needed, you can revoke your API key on the same registration page.
Guide on Using the Steam Web API#
To use the Steam Web API, you need to understand how to make HTTP requests.
Here's a guide with code snippets in both JavaScript and curl
.
Setting up#
Ensure you have a Steam API key by creating an account on Steam and generating a key through the Steam API Key page.
The Steam API interface is a bit strange - seemingly modeled after a C++ API.
The URL format is
https://api.steampowered.com/<interface>/<method>/<method_version>/
Making Requests with curl#
Getting a Friend List
curl "http://api.steampowered.com/ISteamUser/GetFriendList/v0001/?key=YOUR_API_KEY&steamid=76561197960435530&relationship=friend"
Getting Global Achievements
curl "http://api.steampowered.com/ISteamCommunity/GetGlobalAchievementPercentagesForApp/v0002/?gameid=440&format=json"
Making Requests with JavaScript#
Using fetch
API
fetch(
`http://api.steampowered.com/ISteamUser/GetFriendList/v0001/?key=YOUR_API_KEY&steamid=76561197960435530&relationship=friend`,
)
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error("Error:", error));
Using axios
const axios = require("axios");
axios
.get(
`http://api.steampowered.com/ISteamUser/GetFriendList/v0001/?key=YOUR_API_KEY&steamid=76561197960435530&relationship=friend`,
)
.then((response) => console.log(response.data))
.catch((error) => console.error("Error:", error));
Alternatives to the Official Steam Web API#
Several alternatives exist for those who find the Official Steam Web API limiting or have specific needs:
-
Third-Party API Wrappers and Libraries: Available for different programming languages like Node.js, Python, etc., to provide a simplified interface and additional functionalities.
-
Third-Party Services: Tools like Steamwebapi enable access to Steam data, surpassing the official API limitations.
These alternatives broaden the scope of possibilities for developers, making it easier to integrate Steam features into various applications.
Steam Web API FAQ#
-
What's the Steam Web API?
It's an HTTP-based API provided by steam, which offers various functionalities for accessing Steam-related data.
-
Types of API Keys
- Public API Key: Used for generic publicly available data.
- Publisher API Key: Required for accessing sensitive data or performing actions. Requires the key to be part of Steam's Closed API Beta.
-
Usage & Authentication
- The API can be accessed from any app capable of making HTTP requests.
- When using Secure HTTP (https), no additional authentication mechanisms are required besides the API key for protected methods.
-
What's the difference between the Steam Web API and Steamworks Partner API?
According to this excellent resource from xPaw the main differences are:
Difference | Steam Web API | Steam Partner API |
---|---|---|
URL | https://api.steampowered.com | https://partner.steam-api.com |
HTTP vs HTTPS | Accessible via HTTP (port 80) and HTTPS (port 443) | HTTPS Only |
Hosting | Hosted behind Akamai's edge cache, so the actual IP addresses you will see for the name will vary based on your location and on ongoing service changes | Not behind Edge Cache |
Authentication | Regular user API key | Must use Publisher API Key |