JSON Patch (RFC 6902
) and
JSON Merge Patch (RFC 7386
) are
two mechanisms for modifying JSON documents. Both are widely used for partial
updates to resources in APIs, but they differ significantly in structure, use
cases, and behavior. Here's a detailed comparison, covering their strengths,
weaknesses, and examples of when to use each.
Overview#
In case you are unfamiliar with either format, here's a brief overview.
- JSON Patch: A fine-grained, operation-based method that specifies a list of operations (add, remove, replace, etc.) to apply to a JSON document.
- JSON Merge Patch: A simpler method that describes modifications by submitting a new version of the JSON object, where only the fields that are changing are included.
Here's a video that'll give you a quick rundown:
Structure#
JSON Patch#
- JSON Patch defines a sequence of operations to perform on the document. Each operation includes a path to the field being modified and the type of modification.
- Operations supported by JSON Patch:
- Add: Insert a new value at a given path.
- Remove: Remove a value from a given path.
- Replace: Replace the value at a given path.
- Move: Move a value from one path to another.
- Copy: Copy a value from one path to another.
- Test: Test if a value exists at a given path (useful for conditional updates).
Example of JSON Patch#
[
{ "op": "replace", "path": "/name", "value": "Adrian" },
{ "op": "add", "path": "/email", "value": "adrian@example.com" },
{ "op": "remove", "path": "/address/street" }
]
In this example:
- The
name
is replaced. - The
email
field is added. - The
street
within theaddress
object is removed.
JSON Merge Patch#
- JSON Merge Patch involves submitting a modified version of the document where
fields that aren't modified are omitted, and fields that are removed are
explicitly set to
null
. - It uses a much simpler format but is less granular than JSON Patch. You cannot explicitly define operations like "move" or "test."
Example of JSON Merge Patch#
{
"name": "Adrian",
"email": "adrian@example.com",
"address": {
"street": null
}
}
In this example:
- The
name
is updated to "Adrian". - The
email
field is added. - The
street
field underaddress
is removed by setting it tonull
.
If you'd like to test out JSON Merge Patch for yourself, check out our new JSON Merge Patch tool and API.
Strengths#
JSON Patch Strengths#
- Fine-Grained Control: JSON Patch is more powerful for complex operations
since it allows fine-grained modifications such as
move
,copy
, andtest
. - Conditional Updates: The
test
operation can be used for conditional updates, making it suitable for scenarios where changes must be validated before being applied. Thetest
command is especially useful in validating that sensitive/protected fields were not modified or deleted. - Precision: Operations such as replacing a specific array element or deeply nested field are handled explicitly.
JSON Merge Patch Strengths#
- Simplicity: JSON Merge Patch is simpler to understand and implement because it mirrors the structure of the original document. It's more intuitive when updating a large section of a document.
- Ease of Use: For basic use cases like modifying or removing fields, JSON Merge Patch is much easier to use. There's no need to specify the exact operation to be performed.
- Efficiency: When making straightforward changes like adding or modifying a few fields, JSON Merge Patch reduces complexity compared to JSON Patch - and also can be represented in a much more compact structure, saving bandwidth.
Weaknesses#
JSON Patch Weaknesses#
- Complexity: The operation-based structure makes JSON Patch harder to work with for developers unfamiliar with the format. It's overkill for simple updates.
- Bulk Updates: For large objects with many fields to update, JSON Patch requires multiple operations, which can be verbose and harder to manage.
- Error-Prone: If the path is incorrect or doesn't exist, operations can fail. The need to be very precise with paths adds room for mistakes, especially if you're less familiar with JSON Pointer.
JSON Merge Patch Weaknesses#
- Lack of Fine Control: JSON Merge Patch lacks the ability to perform complex operations like moving or copying fields.
- Ambiguity in Arrays: JSON Merge Patch does not have explicit support for array modifications, which can be limiting when working with arrays.
- Limited Conditional Support: There’s no mechanism like the
test
operation in JSON Patch, meaning updates are always applied without conditional checks. This required additional code to protect sensitive fields from modification or deletion.
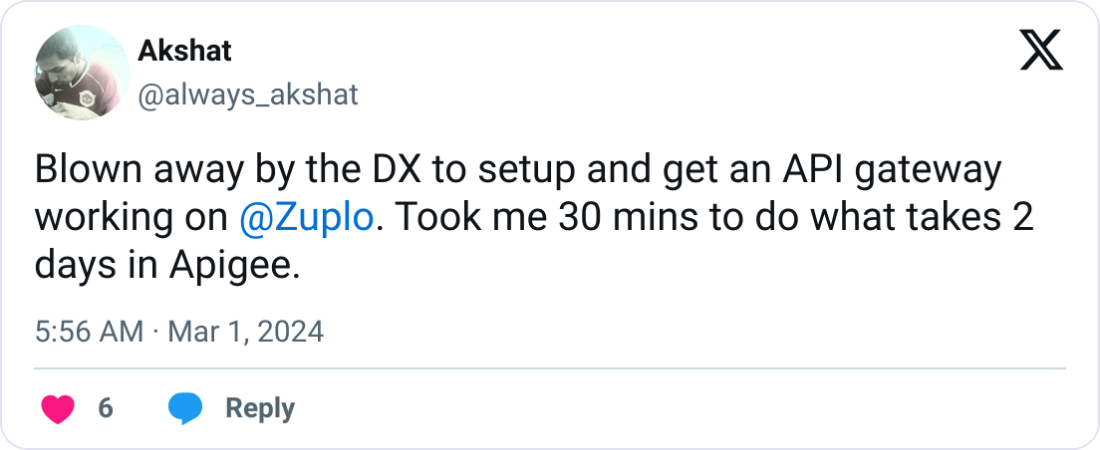
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreUse Cases#
When to Use JSON Patch#
- Complex Modifications: JSON Patch is ideal for scenarios where multiple fields need to be modified with different operations like add, replace, and remove. For example, updating a deeply nested field or handling arrays requires the explicit path-based control JSON Patch offers via JSON Pointer.
- Conditional Updates: When you need to verify a specific condition before
applying an update, JSON Patch’s
test
operation is invaluable. - Partial Changes to an Array: JSON Patch allows you to modify or replace individual elements within an array, something JSON Merge Patch struggles with.
Example
A REST API that allows users to update specific fields of a document or perform conditional updates would benefit from JSON Patch.
[
{ "op": "replace", "path": "/address/zip", "value": "12345" },
{ "op": "test", "path": "/email", "value": "adrian@example.com" }
]
In this case, the update to the zip
is applied only if the email matches.
When to Use JSON Merge Patch#
- Simple Updates: For straightforward cases like adding or updating a few fields in a document, JSON Merge Patch’s simplicity makes it a better fit.
- Updating Large Objects: JSON Merge Patch is better when large portions of the document need to be replaced. You can submit a modified version of the document without explicitly listing each operation.
- APIs with Dynamic Fields: In cases where clients only know parts of the structure and need to update some fields, JSON Merge Patch can easily be used to modify just the known fields.
Example
A REST API that allows users to update their profile would use JSON Merge Patch to update fields without specifying operations:
{
"name": "Adrian",
"phone": "555-1234"
}
In this case, only the name
and phone
fields are changed; the rest of the
profile remains unaffected.
Real World API Examples#
It's typically helpful to see what APIs implementing a pattern actually look like. You can always reach out to their support teams or devs on LinkedIn to inquire about challenges they faced. Here's examples of each.
JSON Patch Example: Every Action API#
The Every Action API has a dedicated page to their implementation of JSON Patch, including a guide.
JSON Merge Patch Example: Worklio#
Similarly, the Worklio API has a dedicated page to their implementation of JSON Merge Patch, with detailed examples.
Conclusion#
- Use JSON Patch when you need precise control over the modifications, especially for complex operations, including conditions and handling array elements.
- Use JSON Merge Patch for simplicity and ease of use when performing basic updates on a JSON document or when the structure is large and only small changes are needed.
Each patching mechanism has its advantages depending on the complexity of the operation and the structure of the document. If you're looking to implement JSON Merge Patch or JSON Patch across your APIs or just want to modernize your APIs more generally, and want advice from an API expert - get in touch.