APIs are the backbone of modern applications, handling traffic between clients and backend services. But poor performance can lead to slow response times, system overloads, and unhappy users. Here's how to fix that.
The 6 Key Strategies to Increase API Performance#
- Caching: Store frequent responses to reduce backend load.
- Payload Optimization: Compress and shrink data for faster transfers.
- Rate Limiting: Control traffic to prevent overloads.
- Regional Endpoints: Serve users closer to their location to lower latency.
- Efficient Authentication: Use serverless functions (ex. Lambda authorizers) to manage security without slowing down requests.
- Performance Monitoring: Track metrics like latency and error rates to spot and fix issues early.
Each strategy tackles specific challenges like high latency, resource bottlenecks, and slow payloads. For example, caching alone can drastically cut response times, while rate limiting ensures stability during traffic spikes.
Quick Overview#
In case you have a specific problem you are trying to solve, here are the sections you should read about:
Challenge | Solution |
---|---|
High Latency | Caching, Regional Endpoints |
Resource Bottlenecks | Rate Limiting, Quotas |
Slow Payloads | Payload Optimization, Compression |
1. Use Effective Caching Strategies#
Adding caching to your API can significantly boost performance by lowering backend workload and speeding up response times. You can consider it a double-win as your customer gets faster responses while your backend is freed up to process other requests. When done right, caching becomes a powerful tool for improving your API's efficiency.
Why Caching Matters#
Caching helps minimize backend strain, reduce delays, and handle more requests, which translates to better scalability, smoother user interactions, and smarter resource use. Tools like Redis and Varnish have shown impressive results in high-demand API setups [1].
Caching is especially important in AI models where compute is expensive.
How to Set Up Caching Policies#
First-and-foremost, it is much easier to roll out caching across your entire API when using an API gateway (its actually one of the most popular features of API gateways). A gateway will help you build a consistent caching implementation (aka a caching policy) that can be reused across endpoints.
To make caching work effectively, focus on these four key areas:
- TTL (Time-to-Live): Decide how long cached data remains valid based on how often it changes. For example, use a short TTL for frequently updated data and a longer one for static content. Some gateways like AWS API Gateway supports TTL values ranging from 0 to 3600 seconds [2], while others like Zuplo are fully customizable.
- Cache Keys: Define unique cache keys using elements like headers, URL paths, or query parameters. This allows for precise control over which responses get cached [2].
- Capacity Planning: Select a cache size that balances performance needs with budget constraints. Monitor hit rates and adjust capacity as usage patterns evolve [2][5].
- Cache Invalidation: Schedule updates to cached data during times of low traffic to keep information accurate.
While caching is great for reducing backend demand, combining it with strategies like optimizing payload sizes can further cut latency and speed up data transfer. Together, these methods form a solid foundation for improving API performance.
2. Reduce Payload Sizes#
Cutting down payload sizes plays a big role in improving API gateway performance. Less data being transmitted means lower latency and better throughput for your API operations.
Transforming Payloads#
Beyond caching, reducing the size of transmitted data speeds up API responses. Again, this is something you should consider doing at the gateway level so you don't have to rewrite serialization/compression across multiple codebases. Many API gateways, offer built-in tools for transforming payloads to make data transfer more efficient.
One standout method is using Protocol Buffers, which create payloads that are 3-10 times smaller than JSON equivalents [1]. The tradeoff here is that you lose the ability to manually inspect and understand your traffic, as well as the need to use a protobuf implementation on your client to deserialize your payload.
Payload Optimization#
A quick win you can get is to remove unnecessary fields in your response body AND headers. Schematizing your responses with OpenAPI and JSON schema helps a lot in keeping track of all your fields. Once you have those definitions, you can use JSON schema validation (aka as Contract Testing in APIs) to ensure your backend is not sending anything unnecessary.
If your API consists of a large list of entities being sent back in the API response, consider supporting pagination to limit the amount of data being sent back without compromising your users' ability to fetch multiple records.
Applying Compression#
Compression is another way to shrink payloads significantly. The algorithm you choose affects both performance and compatibility.
Compression Method | Advantages |
---|---|
GZIP | Works well with text-based data and is widely supported. |
Brotli | Offers 17-25% better compression than GZIP, perfect for modern web apps. |
When choosing a compression method, think about:
- Client compatibility: Can your clients handle the chosen algorithm?
- Processing overhead: Balance the CPU cost against bandwidth savings.
- Data type: Text-based data may benefit more from compression than binary.
Reducing payload sizes helps cut latency, but pairing it with rate limiting ensures your system stays reliable during heavy traffic.
3. Apply Rate Limiting and Quotas#
Rate limiting and quotas help maintain your API's performance by controlling request volumes and ensuring resources are distributed fairly. This keeps your APIs responsive and prevents system overloads.
Setting Thresholds#
Many API rate limiting implementations (whether as a 3rd party module, or as a policy in an API gateway) allow you to configure specific thresholds based on various factors.
Here are some common threshold types and their use cases:
Threshold Type | Example | Purpose |
---|---|---|
Per User | Twitter: 300 tweets/3 hours | Avoids excessive usage by one user |
Per IP Address | Region-based quotas | Protects against DDoS attacks |
Per Application | 10,000 requests/day (free tier) | Supports tiered service plans |
When defining thresholds, consider these factors:
- Traffic patterns: Analyze peak and off-peak usage trends.
- System capacity: Ensure limits align with your infrastructure's capabilities.
- User plans: Adjust thresholds for free and paid users.
Handling Bursts and Throttling#
Token bucket algorithms are a great way to manage traffic bursts. They allow short-term flexibility without compromising system stability.
To manage bursts effectively:
- Use burst tokens: Let users accumulate tokens during low-traffic times for later use.
- Queue excess requests: Instead of dropping them, buffer extra requests to process them later.
- Adaptive throttling: Dynamically adjust limits during high-traffic periods to maintain performance.
For smooth rate limiting:
- Clearly communicate rate limits through response headers and documentation.
- Offer upgrade paths for users who need higher limits.
- Monitor system performance and fine-tune thresholds over time.
- Apply gradual penalties for violations instead of outright blocking users.
Once rate limiting is in place, you can further improve API performance by deploying regional endpoints to minimize latency [3].
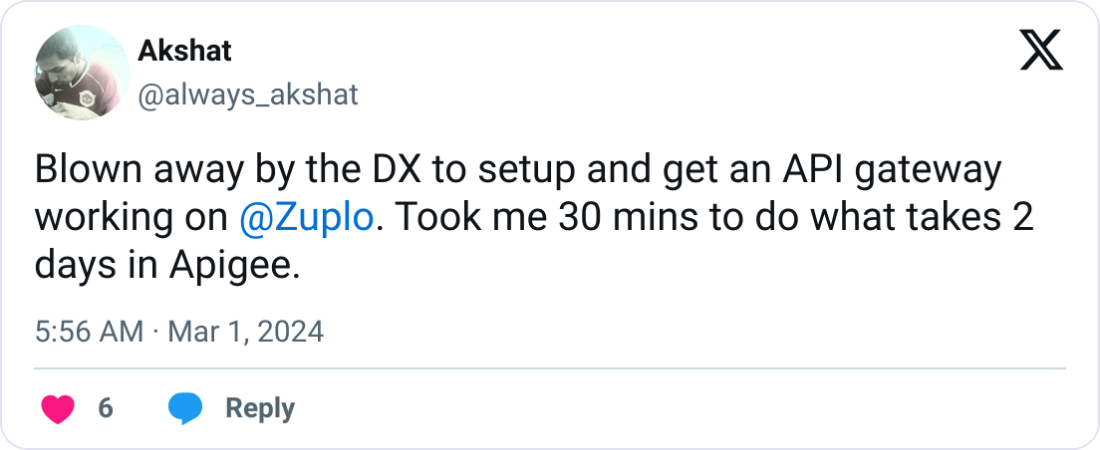
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn More4. Deploy Regional Endpoints#
Setting up regional endpoints helps improve API performance by reducing latency for users located in different parts of the world. Pairing this with caching and rate limiting can make APIs even more efficient.
Why Regional Deployments Matter#
Deploying APIs in regions closer to your users can make a noticeable difference. According to AWS, placing APIs in the same region as your users can cut latency by as much as 70% compared to a single-region setup. Less distance for data to travel means faster response times.
Benefit | Impact | Example |
---|---|---|
Reduced Latency | 40-70% faster response times | US-East users accessing US-East endpoint |
Higher Throughput | 25-35% better request handling | Balancing regional traffic during peak hours |
Improved Reliability | 99.9%+ uptime with redundancy | Automatic failover between regions |
Consider Edge Deployments#
You can take this approach a step further by deploying your API to the Edge. Edge locations are far more numerous than cloud regions (ex. Cloudflare has over 300+ deployment locations) and provide much better latency, with the tradeoff of having to use a custom runtime. A good middle-ground is to have an edge-deployed gateway so you can perform throughput heavy operations (ex. authentication) closer to your user. Caching really shines when combined with edge deployments - edge cache will likely provide the fastest possible response to your user.
Key Steps for Multi-Region Deployment#
Major platforms like Netflix use DNS routing and load balancing to deliver fast, reliable service globally. Here are some ways to set up regional endpoints effectively:
- Configure DNS Routing: Use DNS services like Route 53 to direct users to the nearest endpoint automatically.
- Enable Load Balancing: Deploy global load balancers to manage traffic based on location, endpoint health, and capacity.
- Monitor Performance: Tools like AWS CloudWatch can help track latency, error rates, and traffic flow across regions.
When choosing regions for deployment, focus on areas with the highest user demand to maximize impact.
Once regional endpoints are in place, the next step is to secure and streamline access.
5. Use Serverless Functions for Authentication#
Serverless functions (ex. Lambda, Cloudflare workers) can automatically scale to meet traffic demands - and can be applied to your auth code to avoid bottlenecks. If you're an AWS API gateway user, you might be familiar with Lambda authorizers which implement this concept well. This setup improves gateway performance by spreading the workload more evenly.
Tips for Better Performance#
- Allocate enough memory for complex authentication processes.
- Cache authentication results to avoid repetitive validation.
- Keep response times under 100ms to maintain a smooth user experience.
6. Monitor Performance Metrics#
Keeping an eye on performance metrics isn't just about keeping things running - it's about spotting and fixing potential issues before they affect users. By regularly tracking key indicators, you can ensure your API stays efficient and ready to handle demands.
Key Metrics to Keep an Eye On#
Here are the metrics that matter most for tracking your API's health:
- Latency: Aim for response times under 100ms.
- Error Rates: Keep errors below some threshold defined by your business (ex. 1%).
- Throughput: Monitor to ensure it matches your system's capacity.
- Resource Usage: Stay below 70% to avoid overloading.
These metrics give you a clear picture of your system's performance and help you quickly spot any red flags.
Tools and Practices for Performance Monitoring#
There are several API monitoring tools on the market that can provide advanced error tracking, trend analysis, and deep insights.
Here’s how to make the most of these tools:
- Set Up Monitoring for Every Endpoint: Ensure every API endpoint is being tracked. I hate to beat a dead horse here but PLEASE use a gateway for this - whether you buy one, build your own, self-host an open source gateway - centralization will make your life easier.
- Establish Alerts: Configure alerts for key thresholds like high latency or error spikes.
- Track Resource Usage: Keep an eye on patterns to predict and prevent overloading.
Analyze Trends for Better Optimization#
Understanding performance trends is just as important as monitoring. Dive into the data to uncover patterns:
- Identify peak traffic times and adjust resources accordingly.
- Review error logs to spot recurring issues.
- Monitor how response times change under different loads.
- Study resource usage trends to plan for scaling needs.
Conclusion#
Once performance monitoring is in place, the focus shifts to refining optimization strategies for sustained success. Optimizing an API is a continuous process, crucial for handling the demands of modern applications. The six strategies outlined here tackle major API performance challenges effectively.
For instance, implementing these methods can increase API success rates by up to 50%. Netflix achieved this with tools like EVCache, a distributed caching solution designed to improve performance [1][3].
To maximize the benefits of these strategies, keep these priorities in mind:
- Start with monitoring: Leverage tools such as Moesif or DataDog to establish a performance baseline before making any changes.
- Implement step-by-step: Focus first on strategies that address your most critical performance issues (see the chart at the top).
- Track and refine: Continuously measure key metrics to confirm improvements and make necessary adjustments.
If you’re ready to level up your API's performance, check out Zuplo. It's an edge-deployed API gateway that helps you provide a fast and intuitive developer experience for your customers.
FAQ#
1. Why Is API Performance Important?#
API performance plays a crucial role in the overall user experience and system efficiency. Slow or unresponsive APIs can lead to user dissatisfaction, negative brand perception, and lost revenue opportunities. By prioritizing API performance, you ensure quick data retrieval, reduce timeouts, and improve customer satisfaction. This is especially important for modern web and mobile applications where low latency is expected.
2. What Is API Throughput and Why Does It Matter?#
API throughput measures how many requests an API can handle within a specific time frame, typically expressed as requests per second (RPS) or transactions per second (TPS). High throughput ensures that your API remains responsive under heavy load. Optimizing throughput often involves efficient resource management, load balancing, and caching strategies.
3. How Do I Measure API Performance?#
Measuring API performance involves collecting key API performance metrics, such as response time, latency, error rates, and request rates (throughput). Common monitoring tools include Application Performance Monitoring (APM) solutions like New Relic, Datadog, or Prometheus. By setting up performance alerts, you can quickly identify and resolve issues before they affect end users.
4. Which API Performance Metrics Should I Track?#
- Response Time (Latency): The time it takes to return a response. Lower latency equals better API performance.
- Throughput (RPS or TPS): The volume of requests an API can handle per second.
- Error Rate: The percentage of requests that fail.
- CPU and Memory Usage: Critical for resource allocation and planning.
Tracking these metrics provides a comprehensive view of your API’s health and areas for improvement.
5. How Can REST API Filtering and Pagination Improve Performance?#
REST API filtering and API pagination are strategies to limit the amount of data your endpoint returns. By allowing users (or other services) to specify filters or retrieve smaller data chunks, you reduce the overall payload size. This leads to faster response times and decreases server load. Proper pagination and filtering also help you avoid over-fetching or under-fetching data, contributing to more efficient API throughput.
6. What Tools Can I Use for API Performance Testing?#
There are several API performance testing tools designed to evaluate latency, throughput, and scalability:
- Postman – Offers basic performance and load testing capabilities.
- JMeter – A popular open-source tool for load testing and performance measurement.
- k6 – A modern open-source load testing tool for running tests at scale.
- Locust – Python-based tool for distributed load testing.
These tools help you create and execute tests that simulate real-world traffic, making it easier to identify bottlenecks and improve API performance benchmarks.
7. What Are API Performance Benchmarks?#
API performance benchmarks are standardized measurements or targets that help you compare your API’s performance against industry standards or competitor services. Benchmarks might include average response times under specific loads, acceptable error rates, or throughput levels. By setting and measuring benchmarks, you can continuously evaluate whether your API gateway and underlying infrastructure meet desired performance goals.
8. How Can I Increase API Gateway Performance?#
An API gateway can become a bottleneck if not optimized correctly. To achieve an API gateway performance increase:
- Optimize Routing Rules: Streamline how requests are directed to back-end services.
- Use a Lightweight Gateway: Minimize overhead by choosing or configuring a gateway optimized for speed (ex. Zuplo).
- Enable Caching at the Gateway: Cache responses for commonly requested endpoints.
- Implement Rate Limiting: Control traffic spikes to prevent overloads.
9. How Often Should I Test and Monitor My API’s Performance?#
Regular API performance testing and monitoring should be part of your continuous integration and deployment processes. Ideally, you’ll run automated tests before and after each release, as well as keep an eye on real-time monitoring dashboards. Ongoing monitoring helps you catch issues like slow response times or increased error rates early, allowing you to maintain a consistently high-performing API.