Slow SaaS APIs don't just frustrate users—they actively drive customers to competitors and damage your bottom line. Research shows that even 100ms delays can affect conversion rates, turning potential sales into missed opportunities before you can blink. When every millisecond counts, API performance becomes a competitive advantage you can't afford to ignore.
The business impact of sluggish APIs goes beyond mere inconvenience. Poor performance creates frustrating user experiences, decimates customer retention, and directly hits your revenue. For data-heavy SaaS applications especially, the speed gap between you and competitors could determine who captures those valuable customers—and who loses them.
Ready to transform your API performance from a liability into a strength? Let's dive into proven approaches that'll have your SaaS APIs delivering the blazing speed your customers demand and your business needs.
- Understanding API Performance: The Foundation of User Experience
- Breaking Through API Performance Barriers
- 1. Design for Speed from Day One
- 2. Supercharge Performance with Multi-level Caching
- 3. Fix Your Database: From Bottleneck to Powerhouse
- 4. Balance the Load: Advanced Strategies for Peak Performance
- Transforming APIs for Modern Demands
Understanding API Performance: The Foundation of User Experience#
Think of API performance as the heartbeat of your digital system. When it's strong and steady, everything else runs smoothly. At its core, API performance is about how quickly and efficiently an API processes requests and delivers responses. Whether you're aiming to build an API integration platform or optimize existing services, understanding API performance is crucial.
Three key metrics stand out when measuring API performance:
Latency: The Travel Time That Matters#
Latency refers to the complete travel time for a request and response round trip. Lower latency creates quicker, more responsive interactions, directly impacting how users perceive your application's speed.
Throughput: Handling the Crowd#
Throughput measures how many requests your API can handle within a specific timeframe. Higher throughput means your system manages concurrent requests effectively—essential for busy applications with many simultaneous users.
Response Time: The User Experience Metric#
Response time captures the total duration from the moment a request is sent until the response is received. This holistic measurement gives you the clearest picture of what your users actually experience when interacting with your system.
These aren't just numbers on a dashboard—they're crucial indicators that help you identify bottlenecks and improve both user satisfaction and business outcomes.
Breaking Through API Performance Barriers#
Every system faces performance challenges as demands increase. Many systems weren't designed with today's high-volume, data-intensive operations in mind, which leads to slower response times and reduced throughput. By recognizing and addressing these common bottleneck problems, you can enhance API performance and meet the demands of modern applications.
Database Efficiency Hurdles#
Poorly optimized database queries can create serious performance bottlenecks. When APIs make unnecessary database calls or retrieve excessive data, response times increase dramatically. This is especially problematic when dealing with relational databases that weren't designed for the access patterns modern APIs require.
Network Latency Issues#
Geographic distribution of users and services introduces significant latency challenges. As API architectures become more complex and globally distributed, the time required for data to travel between locations becomes a critical performance factor.
Third-Party Service Dependencies#
APIs that rely on external services inherit their performance limitations. When your API chain includes multiple third-party integrations, each additional service becomes a potential failure point that can degrade overall performance.
Authentication and Security Overhead#
Essential security measures like authentication, authorization, and encryption add processing overhead to each API call. Finding the balance between robust security and optimal performance is a constant challenge. Implementing API security best practices can help you achieve this balance without compromising performance.
In short, API performance issues create a cascade of problems: integration obstacles, operational inefficiencies, and frustrated users. Let’s discuss time-tested strategies for developers to tackle these performance issues from the ground up.
1. Design for Speed from Day One#
Performance needs to be baked into your SaaS API design from the ground up. Too many teams try to optimize their way out of fundamental design flaws, and that's a painful road you don't want to travel.
API Design Principles That Enhance Performance#
Well-designed RESTful SaaS APIs incorporate several performance-enhancing principles. Understanding and effectively applying API design principles can significantly enhance performance.
Resource-oriented design
Structure your API around resources rather than actions. This approach naturally creates better caching opportunities and clearer separation of concerns.
Consistent resource naming
Use intuitive, consistent naming conventions for endpoints. This directly impacts maintainability and helps developers predict how your SaaS API behaves.
Efficient payloads
Implement field filtering to let clients specify exactly what fields they need.
Stripe nails this with requests like GET /v1/customers?fields=id,email,name
to
retrieve only essential data. Less data transferred means faster responses.
Pagination by default
Always implement pagination for collection endpoints. Nothing kills performance faster than trying to return 10,000 records in a single response.
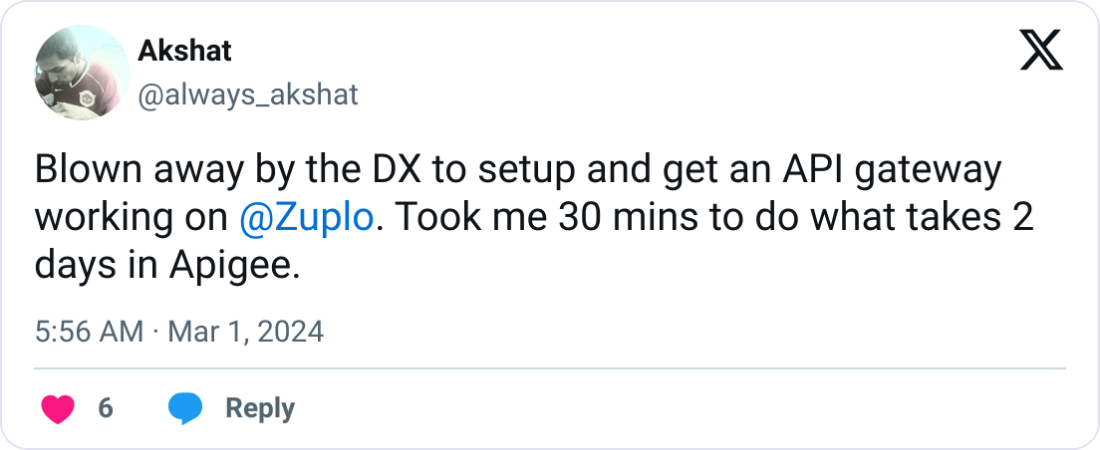
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreVersioning strategy
Choose a versioning approach that won't hurt performance. URL path versioning
(like /v1/resources
) provides clear separation but can complicate caching
strategies across versions.
Additionally, using automated methods to generate APIs from your database can help ensure your API design is both efficient and consistent.
When to Choose Synchronous vs. Asynchronous Approaches#
Deciding between synchronous and asynchronous processing models is a critical performance decision:
Synchronous APIs (request-response model):
- Best for operations that must complete quickly (under 1-2 seconds)
- Simpler to implement and understand
- Ideal for CRUD operations, retrieving data, and simple processing
Asynchronous APIs:
- Essential for long-running operations
- Significantly improve perceived performance by acknowledging requests immediately
- Ideal for report generation, large data exports, complex calculations, or batch operations
For asynchronous approaches, consider webhooks or polling mechanisms. Stripe's SaaS API uses both models effectively: standard CRUD operations are synchronous, while processes like generating account reports are handled asynchronously with webhook notifications when complete.
2. Supercharge Performance with Multi-level Caching#
If you're not using multi-level caching for your SaaS API, you're leaving serious performance gains on the table. Nothing boosts API performance like a well-implemented caching strategy. By learning how to enhance API performance with caching, you can stop hammering your database with duplicate requests. Let's fix that.
Cache Invalidation Strategies for Dynamic SaaS Data#
When working with frequently changing SaaS data, cache invalidation becomes critical:
Time-based expiration#
Set appropriate TTL values based on how frequently your data changes. Critical, frequently-updated resources might have a TTL of seconds or minutes, while more static data could be cached for hours.
Event-based invalidation#
Implement a publish/subscribe system where data mutations trigger cache invalidation events. When a resource is updated, publish an event that notifies caching layers to invalidate or update the related cache entries.
Version-based caching#
Add a version identifier to cached objects that changes whenever the underlying data changes. Instead of invalidating cache entries, your application can check if the cached version matches the current version.
Write-through caching#
Update the cache immediately when writing to the database. This ensures the cache always has the most recent data but adds complexity to your write operations.
Tenant-Aware Caching for Multi-tenant SaaS Applications#
In multi-tenant SaaS applications, you need to ensure that cached data remains isolated between tenants:
Namespace your cache keys#
Prefix all cache keys with a tenant identifier:
cache_key = f"tenant:{tenant_id}:resource:{resource_id}"
Partition your cache#
For large-scale applications, dedicate separate cache instances or partitions to different tenants or tenant groups.
Implement tenant-specific expiration policies#
Different tenants may have different usage patterns, so customize cache TTLs accordingly. High-activity tenants might benefit from longer cache durations.
Use Redis for tenant isolation#
Redis's database selector feature provides a simple way to segregate tenant data:
# Connect to tenant-specific Redis database
redis_client = redis.Redis(host='redis-server', port=6379, db=tenant_id % 16)
For most modern SaaS applications, Redis offers more versatility and rich features that accommodate the complex requirements of multi-tenant environments compared to Memcached.
3. Fix Your Database: From Bottleneck to Powerhouse#
Your database is probably the reason your SaaS API is crawling. Most performance issues stem from lazy database design and queries that would make any DBA weep. Let's transform your database from performance bottleneck to performance powerhouse.
Query Optimization Techniques for SaaS API Access Patterns#
Many performance issues stem from inefficient query patterns that become increasingly problematic as your application scales:
Identify and Fix Missing Indexes#
When your database performs full table scans instead of using indexes, query performance suffers dramatically. Always review your most frequently executed queries and ensure appropriate indexes exist.
-- Before optimization (no index)
SELECT * FROM orders WHERE customer_id = 123;
-- Execution time: 700ms (full table scan)
-- After adding index
CREATE INDEX idx_orders_customer_id ON orders(customer_id);
-- Execution time: 15ms
We've seen adding a single composite index reduce query execution time from 7 seconds to 200 milliseconds in production. The performance gain is particularly noticeable in API endpoints that filter large datasets.
Solve the N+1 Query Problem#
The N+1 query problem occurs when your API makes one query to fetch a list of records, followed by N additional queries to fetch related data for each record.
# Inefficient approach (N+1 problem)
users = db.query("SELECT * FROM users LIMIT 10")
for user in users:
user.orders = db.query("SELECT * FROM orders WHERE user_id = ?", user.id)
# Optimized approach
users = db.query("SELECT u.*, o.* FROM users u
LEFT JOIN orders o ON u.id = o.user_id
WHERE u.id IN (SELECT id FROM users LIMIT 10)")
Use Query Result Caching#
For data that doesn't change frequently, implementing query result caching can dramatically reduce database load. A study by DZone found that query caching reduced database load by 40% during peak hours for a SaaS platform.
Understanding how to convert SQL queries into efficient API requests can help minimize database load and improve API performance.
Optimize Complex Queries#
Break down complex queries with multiple joins and subqueries into smaller, more manageable pieces. Use EXPLAIN to analyze execution plans and identify performance bottlenecks.
Data Sharding and Partitioning for SaaS Scale#
As your SaaS platform grows, a single database instance may no longer be sufficient:
Tenant-Based Sharding#
In multi-tenant SaaS applications, sharding data by tenant ID is an effective strategy. Each tenant's data resides in a separate database partition, allowing for better isolation and performance. A SaaS analytics platform reduced query latency by 80% after implementing tenant-based sharding, as queries no longer needed to scan through unrelated tenant data.
Time-Based Partitioning#
For time-series data, partitioning by time periods (days, months, or years) can significantly improve query performance for both recent and historical data access.
-- Create partitioned table by month
CREATE TABLE events (
id SERIAL,
tenant_id INTEGER,
event_type VARCHAR(50),
created_at TIMESTAMP,
payload JSONB
) PARTITION BY RANGE (created_at);
-- Create monthly partitions
CREATE TABLE events_y2023m01 PARTITION OF events
FOR VALUES FROM ('2023-01-01') TO ('2023-02-01');
CREATE TABLE events_y2023m02 PARTITION OF events
FOR VALUES FROM ('2023-02-01') TO ('2023-03-01');
This strategy is particularly effective for APIs that primarily access recent data, as queries can target specific partitions rather than scanning the entire dataset.
4. Balance the Load: Advanced Strategies for Peak Performance#
Your SaaS API infrastructure needs to handle everything from quiet periods to massive traffic spikes without breaking a sweat. That's where advanced load balancing comes in—not just basic round-robin stuff, but sophisticated strategies that keep your APIs blazing fast even during peak loads.
API Gateway Strategies for SaaS#
A well-implemented API gateway, equipped with essential API gateway features, serves as the front door for all API traffic and can significantly enhance your performance:
Intelligent routing#
Configure your gateway to route requests based on tenant information, allowing for more efficient resource allocation. This ensures premium customers can receive prioritized service while maintaining consistent performance for all users.
Gateway-level caching#
Implement caching at the API gateway to reduce backend load. A properly configured cache can reduce backend load by up to 70%, as seen in an e-learning SaaS platform that implemented tenant-aware routing and caching.
Rate limiting and throttling#
Apply these controls at the gateway level to prevent abuse and ensure fair resource distribution across your multi-tenant environment. If you're encountering issues like 'API Rate Limit Exceeded' errors, understanding how to handle API rate limits can help maintain optimal performance while safeguarding your services.
For implementation, you can leverage cloud-native solutions like AWS API Gateway, Azure API Management, or open-source alternatives like Kong or Tyk. Opting for a hosted API gateway can provide significant benefits over building your own, including reduced maintenance and quicker deployment.
Geographic Distribution for Global Performance#
For SaaS platforms serving a global audience, geographic distribution is essential for delivering consistent performance:
Multi-region deployment#
Deploy your API servers across multiple geographic regions to serve users from the nearest location. This approach significantly reduces latency for your global user base while providing redundancy and disaster recovery options. Utilizing a multi-cloud API gateway can facilitate seamless deployment across various cloud providers and regions, ensuring optimal performance and redundancy.
Content Delivery Networks (CDNs)#
Utilize CDNs to cache and serve API responses from edge locations closer to end-users. This strategy can dramatically reduce response times for users far from your main data centers.
Global load balancing#
Implement DNS-based global load balancing (like AWS Route 53 or Cloudflare) to automatically direct users to the nearest healthy endpoint.
Shopify demonstrates the power of this approach by using a multi-region architecture with sophisticated load balancing to handle high-traffic events like Black Friday, serving over 300 million buyers across 175 countries.
For zero-downtime deployments, implement blue-green deployment strategies:
- Maintain two identical production environments (blue and green)
- Direct all traffic to the active environment (e.g., blue)
- Deploy updates to the inactive environment (green)
- Test the updated environment
- Switch traffic from blue to green once testing is complete
This strategy eliminates downtime during deployments and provides an instant rollback capability if issues arise. Combined with auto-scaling based on traffic patterns, you can ensure your SaaS API maintains optimal performance even during unexpected traffic spikes.
Transforming APIs for Modern Demands#
Bottom line? Optimizing your APIs is about delivering experiences that keep your users happy and your business growing. When you implement these performance strategies, you're not just reducing load times and server costs. You're creating the kind of responsive, reliable system that builds trust and keeps users coming back instead of heading to competitors.
Ready to give your APIs the performance boost they deserve? Zuplo makes it surprisingly simple with our developer-friendly tools and ready-to-deploy optimization policies. You don't need to rebuild everything from scratch—book a meeting with Zuplo today and find out how quickly you can transform those sluggish endpoints into speedy assets.