SOAP APIs are essential for industries like banking and healthcare, ensuring secure and structured data exchange. Testing these APIs is critical to maintain reliability, security, and performance. Here's what you need to know:
- What is SOAP? A protocol using XML for consistent messaging across systems, similar to a postal service for data.
- Why Test SOAP APIs? To validate security, message integrity, compatibility, and fault management.
- Core Components:
- Envelope: Message container.
- Header: Optional, for control data (e.g., authentication).
- Body: Main message content.
- WSDL (Web Services Description Language): Defines SOAP services, operations, and endpoints for seamless integration.
Tools & Steps for Testing#
- Key Tools: SoapUI (free and Pro versions) for functional, security, and load testing or Step CI for a modern, open-source alternative that focused on workflow testing.
- Testing Steps:
- Plan test cases based on business needs.
- Build XML requests and validate responses.
- Use API mocking for safe testing.
- Manage test data for various scenarios.
Basic Requirements#
SOAP Protocol Basics#
SOAP uses an XML-based structure that ensures reliable data exchange in enterprise environments. It includes three main components, each playing a specific role in secure message delivery:
Component | Purpose | Key Elements |
---|---|---|
Envelope | Root container for the message | XML namespace declarations, versioning |
Header (Optional) | Contains control-related information | Authentication, routing, payment data |
Body | Main content of the message | Service requests, responses, fault messages |
Here's an example of a basic SOAP message structure:
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<!-- Header (Optional): Contains metadata -->
<soap:Header>
<auth:Credentials xmlns:auth="http://example.org/auth">
<auth:Username>user123</auth:Username>
<auth:Password>pass456</auth:Password>
</auth:Credentials>
<tx:TransactionID xmlns:tx="http://example.org/transaction">
TX-12345
</tx:TransactionID>
</soap:Header>
<!-- Body: Contains the actual request/response data -->
<soap:Body>
<m:GetStockPrice xmlns:m="http://example.org/stock">
<m:StockName>ACME</m:StockName>
</m:GetStockPrice>
</soap:Body>
</soap:Envelope>
The SOAP envelope acts as the wrapper for all message components and defines the overall XML structure. Headers include optional processing instructions, with two key attributes:
- mustUnderstand: Specifies if a header entry must be processed.
- actor: Identifies the intended recipient of the message.
SOAP is a transmission and packaging protocol, standardized by the World Wide Web Consortium (W3C) [1].
WSDL Basics#
WSDL (Web Services Description Language) acts as a contract between SOAP services and their clients. This XML-based document outlines service operations, message formats, data types, protocols, and endpoint details.
Key sections of a WSDL document include:
Section | Description | Purpose |
---|---|---|
Definitions | Root element | Includes service namespaces |
Types | Defines data structures | Specifies message formats |
Messages | Abstract message definitions | Describes data exchanged |
Port Types | Abstract service operations | Lists available methods |
Bindings | Protocol and data format details | Explains how to access the service |
Services | Endpoint information | Specifies where the service is located |
Here's an example of a basic WSDL for a calculator service:
<?xml version="1.0" encoding="UTF-8"?>
<definitions name="CalculatorService"
targetNamespace="http://example.org/calculator"
xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.org/calculator"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<!-- Data types used by the service -->
<types>
<xsd:schema targetNamespace="http://example.org/calculator">
<xsd:element name="AddRequest">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="a" type="xsd:int"/>
<xsd:element name="b" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
<xsd:element name="AddResponse">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="result" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
</xsd:schema>
</types>
<!-- Messages for the service operations -->
<message name="AddInput">
<part name="parameters" element="tns:AddRequest"/>
</message>
<message name="AddOutput">
<part name="parameters" element="tns:AddResponse"/>
</message>
<!-- Port Type (Interface) -->
<portType name="CalculatorPortType">
<operation name="Add">
<input message="tns:AddInput"/>
<output message="tns:AddOutput"/>
</operation>
</portType>
<!-- Binding to SOAP protocol -->
<binding name="CalculatorSoapBinding" type="tns:CalculatorPortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="Add">
<soap:operation soapAction="http://example.org/calculator/Add"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<!-- Service definition -->
<service name="CalculatorService">
<port name="CalculatorPort" binding="tns:CalculatorSoapBinding">
<soap:address location="http://example.org/calculator"/>
</port>
</service>
</definitions>
These elements provide the foundation for using and testing SOAP services effectively.
Required Tools and Skills#
To test SOAP APIs effectively, you need the following skills and tools:
- Proficiency in XML syntax, including namespaces and schema validation.
- The ability to read and understand WSDL service definitions.
- Experience with API testing concepts.
Testing SOAP APIs can present challenges such as:
- Handling complex XML structures that require rigorous validation.
- Resolving version compatibility issues between tools.
- Dealing with performance overhead caused by XML processing.
- Managing inconsistent error handling across implementations.
Building expertise in these areas is critical for ensuring reliable and secure service operations.
Testing Process Steps#
Planning Test Cases#
Start by identifying the key business functions and error-handling scenarios that your SOAP API needs to support. This helps you pinpoint which situations require detailed testing. Once you've outlined these scenarios, move on to creating specific, actionable test cases.
Building Test Cases#
When building test cases, focus on the XML structure and validation rules. Use the WSDL file to understand the operations available and the required message formats. Here are the main steps:
- Request Preparation
Create XML requests with the correct namespaces, proper encoding, and accurate data types. - Response Validation
Define criteria to verify that responses match the expected schema and adhere to business rules, including appropriate error handling. - Test Data Management
Prepare distinct datasets for valid inputs, edge cases, and invalid scenarios to ensure comprehensive testing.
Testing Tools Guide#
SoapUI Guide#
SoapUI is a cross-platform tool designed for SOAP API testing. It supports functional, security, load, and compliance testing, making it a versatile option for developers and testers alike [3].
To get started with SoapUI for SOAP API testing, follow these steps:
- Project Setup: Import your WSDL file to automatically generate test requests and responses.
- Test Suite Creation: Use the interface to create test cases for various scenarios. It's not exactly modern but it gets the job done.
- Environment Configuration: Set up separate environments (e.g., QA, Dev, Prod) to ensure focused and efficient testing.
These steps lay the groundwork for advanced testing tasks like endpoint simulation and data management.
Here’s a quick comparison of features between the two SoapUI editions:
Feature | SoapUI Open Source | SoapUI Pro |
---|---|---|
WSDL Coverage | No | Yes |
Message Assertion | Yes | Yes |
Test Refactoring | No | Yes |
Scripting Libraries | No | Yes |
Unit Reporting | No | Yes |
As you can see, the Open Source version of SoapUI is quite limited - so let's explore another option.
Step CI Guide#
Step CI is an open-source API Quality Assurance framework designed to run workflow tests (integration and multi-step end-to-end) in your CI pipeline. Here are the key features:
- Language-agnostic: Step CI uses its own yaml-based syntax for describing API workflow tests. It's easy and intuitive to read and write.
- Multi-API support: Supports SOAP, REST, GraphQL, and gRPC - even within the same workflow.
- Self-hosted: You can run Step CI in your own network, locally, or within a CI platform like Github Actions.
The simplest way to use Step CI is via CLI:
npm install -g stepci
Then write a workflow.yml
file for your SOAP test. Let's write a test for the
"number to words" service which converts numbers to an english words equivalent.
version: "1.1"
name: SOAP API
tests:
example:
steps:
- name: POST request
http:
url: https://www.dataaccess.com/webservicesserver/NumberConversion.wso
method: POST
headers:
Content-Type: text/xml
SOAPAction: "#POST"
body: >
<?xml version="1.0" encoding="utf-8"?> <soap:Envelope
xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/">
<soap:Body>
<NumberToWords
xmlns="http://www.dataaccess.com/webservicesserver/">
<ubiNum>500</ubiNum>
</NumberToWords>
</soap:Body>
</soap:Envelope>
check:
status: 200
selectors:
m\:numbertowordsresult: "five hundred "
Finally, run the test:
stepci run workflow.yml
These workflows can include multiple steps so you can test a full CRUD workflow - sending mock data and inspecting response statuses and bodies. For more information on testing SOAP APIs - check out their docs.
Personally, I find Step CI easier to use and more flexible than SoapUI.
API Mocking Guide#
Once your test cases are ready, it’s time to simulate real endpoints without relying on live systems. API mocking helps you:
- Create virtual services that mimic actual SOAP endpoints.
- Safely test edge cases and error scenarios.
- Work with APIs that are still in development.
- Validate service behavior without affecting production systems.
To set up effective mocks, you can either used the paid version of SOAP UI or use a dedicated mocking tool like Mockbin. Additionally, you can add mocking to your API gateway directly so no URL switching is needed down the line.
Test Data Management#
With your test cases and environments in place, managing test data efficiently is crucial for consistent results. Here are some strategies to streamline your test data management:
- Data Organization
Separate datasets for different scenarios, ensuring they align with your WSDL specifications. Use structured formats for easy access and updates. - Environment Management
If you're using SoapUI, it has an environment-switching feature to maintain unique configurations for each testing stage. This includes managing endpoints, credentials, and test data specific to each environment. If you're using StepCI - simply set an environment variable like${{env.host}}
to switch environments. - Automation Integration
Integrate either library with your CI/CD pipelines using its command-line support. Here's an example integrating Step CI into your Github Action:
on: [push]
jobs:
api_test:
runs-on: ubuntu-latest
name: API Tests
steps:
- uses: actions/checkout@v3
- name: Step CI Action
uses: stepci/stepci@main
with:
workflow: "tests/workflow.yml"
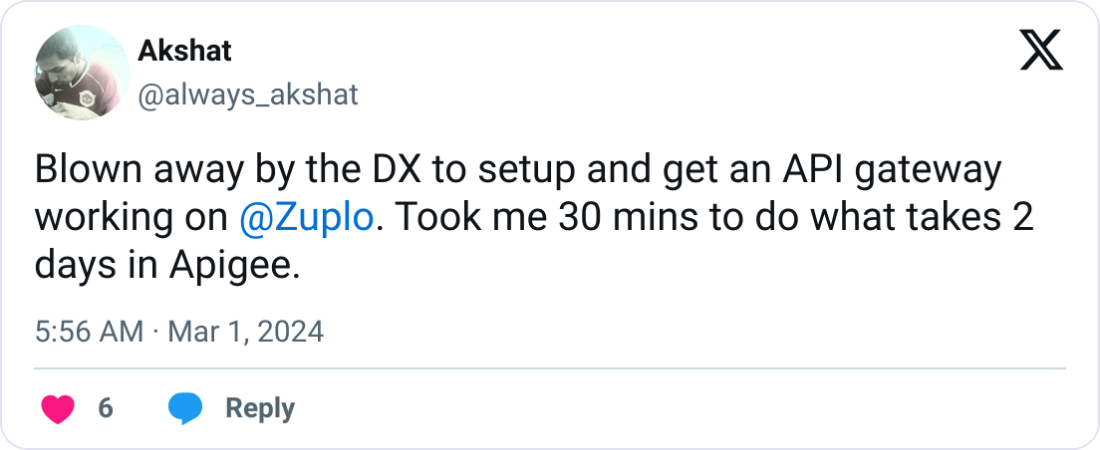
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreTips and Problem Solving#
Testing Tips#
Thorough SOAP API testing is key to ensuring reliability. Here are some important practices to follow:
WSDL Validation and Management Keep your WSDL documentation up to date and regularly validate it. This helps catch structural issues early. SoapUI and StepCI offer built-in validation features to help identify inconsistencies.
Environment Configuration Set up environment variables to differentiate between development, staging, and production testing. Here's a quick breakdown:
Environment | Purpose | Key Considerations |
---|---|---|
Development | Quick testing/debugging | Use mock services to handle dependencies |
Staging | Integration testing | Match production settings as closely as possible |
Production | Final validation | Focus on non-destructive testing actions |
These practices help streamline the testing process and address potential challenges effectively.
Common Problems and Fixes#
SOAP testing often comes with its own set of challenges. Here's how to tackle some of the most common ones:
Data Integrity Issues When testing text casing, ensure inputs like 'AbCdE' return the expected output, such as 'aBcDe'. You can use JavaScript in Postman's test tab to verify these results.
Security Testing Gaps Focus on validating XML signatures, testing SOAP header authentication, and checking for vulnerabilities like XML injection. These steps help ensure your API is secure.
By addressing these issues, you can improve both the reliability and security of your SOAP API testing.
CI/CD Integration Steps#
Incorporating SOAP API tests into your CI/CD pipeline can improve efficiency and maintain quality. Here's how to do it:
- Configure automated test execution using either SoapUI's or Step CI's command-line interface to seamlessly integrate with your CI/CD pipeline.
- Use environment-specific variables to handle different testing stages, like development or production.
- Set up detailed test reporting to monitor results and identify patterns over time.
These steps help maintain continuous quality checks while aligning with earlier testing efforts.
Advanced Automation#
With SoapUI, you can take your testing to the next level with Groovy scripting. It can help you:
- Pre-process API requests
- Post-process responses
- Run conditional tests based on specific criteria
- Customize test reports for better insights
With Step CI, many features you would use scripting for like setup and teardown are built into the workflow syntax. You can use Step CI as a node library if you want more control over the execution or results handling.
Conclusion#
Summary Points#
SOAP API testing plays a crucial role in ensuring APIs function reliably and perform as expected. By using structured testing methods and the right tools, businesses can enhance the quality and dependability of their APIs.
Here’s a quick look at what SOAP API testing can achieve:
Testing Area | Purpose | Result |
---|---|---|
Reliability | Identifies potential issues | Minimizes system disruptions |
Performance | Assesses load capacity | Keeps services stable |
Security | Detects vulnerabilities | Safeguards data integrity |
Integration | Supports system connections | Simplifies communication |
These outcomes contribute to a smoother and more efficient testing process. If you're looking to improve your API development process - check out Zuplo! It's a multi-protocol API gateway that allows you to build, secure, and document REST, SOAP, and GraphQL APIs for free.
FAQs#
What are the main challenges of testing SOAP APIs, and how can they be resolved?#
Testing SOAP APIs can present several challenges, including handling complex XML requests and responses, ensuring compatibility with strict SOAP standards, and managing security protocols like WS-Security. These challenges require careful planning and the right tools to address effectively.
To overcome these issues, use testing tools like SoapUI, Step CI, or event Postman to simplify the creation and validation of SOAP requests and responses. Validate that the service adheres to its WSDL (Web Services Description Language) specifications to ensure compatibility. Pay close attention to security measures, such as encrypting sensitive data and properly configuring authentication mechanisms, to protect the API and its users. Regular testing under various conditions can help identify and resolve performance bottlenecks early on.
How does adding SOAP API testing to a CI/CD pipeline improve the development workflow?#
Integrating SOAP API testing into a CI/CD pipeline significantly enhances the development process by automating the validation of your APIs at every stage. This ensures that any issues are identified early, reducing the risk of bugs reaching production.
Automated testing in the pipeline increases test coverage, improves team collaboration, and speeds up the time-to-market. It also allows developers and QA teams to work together seamlessly, ensuring reliable and high-performing SOAP-based services while saving time and resources.
What role does WSDL play in SOAP API testing, and how can it be used effectively?#
WSDL (Web Services Description Language) is an XML-based format that defines how SOAP web services communicate. It specifies the operations available, the inputs and outputs required, and how messages are structured, making it an essential tool for testing SOAP APIs.
By using WSDL, you can ensure interoperability between systems, even if they are developed in different programming languages. It also enables automation by allowing testing tools to generate client-side stubs and other components, which simplifies development and reduces errors. Leveraging WSDL ensures your SOAP APIs are well-documented, reliable, and easier to test effectively.