With 74% of developers choosing APIs over code, understanding the differences between building simple Web APIs and REST APIs is crucial. These digital connectors dominate the landscape, but developers often confuse them or use the terms interchangeably, leading to rookie mistakes that can tank your performance, scalability, and maintenance.
This guide cuts through the confusion with practical, no-nonsense comparisons between Web APIs and REST APIs. Forget the theoretical fluff. We're looking at real-world applications where each type absolutely crushes it.
And here's the good news: Zuplo's code-first platform supports both API types, so you can implement what actually works instead of compromising. Let’s break it down.
- Why Your Business Can't Survive Without APIs
- Supercharge Your Development with Simple Web APIs
- Unlocking the Magic of REST APIs
- REST vs Web APIs: The Ultimate Showdown
- Unleashing the Power of Simple Web APIs: When Less is More
- 5 Scenarios Where REST APIs Reign Supreme
- Unleash Your API’s Power with Zuplo's Code-First Platform
- Future-Proof Your Project: Picking the Perfect API Approach
Why Your Business Can't Survive Without APIs#
Think of an API as your application's personal butler. It takes your request to the kitchen and brings back exactly what you ordered, no more and no less. Your app makes a request, and the API handles all the messy details. Boom, you get what you need.
Every digital service you use daily runs on APIs—from scrolling through TikTok to paying for that overpriced coffee. They're the secret sauce for:
- Seamless System Integration: APIs connect different systems that were never designed to talk to each other. Magic? Nope, just good engineering.
- Cross-Platform Workflows: That task that starts on your phone and finishes on your laptop? Thank an API for that smooth handoff.
- Device-Agnostic Service Delivery: APIs don't care if you're on a pricey MacBook or a budget Android. They deliver the same data either way.
- New Revenue Streams: Companies monetize their data through APIs, creating entirely new business models. Ka-ching!
The two heavyweight champions in the API world are Web APIs and REST APIs. Web APIs give you broad flexibility with HTTP protocols, while REST APIs follow specific architectural rules that make them web communication powerhouses.
This distinction isn't just academic. The differences shape everything about how you build. REST APIs use standardized constraints that some developers find limiting, while Web APIs give you more freedom to implement whatever crazy solution your project needs.
Both became wildly popular because they're straightforward and scalable, especially in web environments. But your choice between them affects literally everything downstream, from development speed to long-term maintainability.
Supercharge Your Development with Simple Web APIs#
Web APIs are the rebel teenagers of the API world. They follow some basic rules around HTTP protocols but otherwise do their own thing. Unlike their straitlaced REST cousins with their strict architectural principles, Web APIs let you structure things your way. That's pure gold when you need to ship fast or tackle unique requirements.
Think of Web APIs as setting up a direct phone line between applications—they establish basic communication rules while letting you choose exactly what language you want to speak.
Freedom to Build Your Way#
Web APIs support multiple approaches beyond REST, including SOAP and XML-RPC. This flexibility lets you:
- Use HTTP/HTTPS as your transport mechanism
- Work with whatever data format makes sense (XML, JSON, SOAP)
- Build custom solutions for specific business problems
- Skip architectural constraints when they just slow you down
- Focus on solving problems rather than following doctrine
ASP.NET Web API is a perfect example—it creates HTTP services that any client can consume, proving that sometimes simpler approaches just work better.
Perfect for Practical Solutions#
Web APIs absolutely crush it when you need:
- Internal tools that don't need complex architecture
- Integration with ancient legacy systems that refuse to die
- Development sprints with aggressive timelines
- Simple services without REST's architectural overhead
- Support for custom protocols or specialized data formats
We've seen countless companies deploy Web APIs on their intranets to connect internal systems without exposing them to the world.
Pros and Cons of Simple Web APIs#
Reasons to Choose a Web API | Challenges of Web APIs |
---|---|
Choose your protocols and patterns based on needs, not dogma | Scaling becomes harder without standardized practices |
Skip unnecessary constraints that don't add value | Inconsistent implementations mean slower team onboarding |
Build exactly what your problem requires | System integration gets more complex |
Deploy faster and iterate quicker | Maintenance costs tend to climb over time |
Support those weird edge-case requirements | Documentation needs grow as conventions vary |
Web APIs shine brightest when your priority is crafting custom solutions or getting to market quickly rather than architectural purity.
Unlocking the Magic of REST APIs#
Ever wonder what makes REST APIs so special? They follow specific architectural principles that set them apart. These principles, first outlined in Roy Fielding's doctoral dissertation, create a framework that developers worldwide instantly recognize.
Architectural Principles of a REST API#
- Client-Server Separation: Creates a clean division between clients and servers, allowing independent evolution and faster development across teams.
- Statelessness: Every request contains all necessary information with no session data stored between calls, making scaling across multiple servers seamless.
- Strategic Caching: Responses clearly indicate if they can be cached, preventing stale data while reducing server load.
- Uniform Interface: Standardizes client-server interactions through consistent URIs and messaging patterns, acting like a universal remote for all API interactions.
- Layered System Architecture: Components only communicate with adjacent layers, enabling invisible addition of authentication, load balancing, or caching features.
- Code on Demand (Optional): Servers can send executable code to extend client functionality when needed.
app.get('/api/products/:id', (req, res) => {
const product = getProductById(req.params.id);
if (!product) {
return res.status(404).json({ error: 'Product not found' });
}
res.status(200).json(product);
});
From GitHub to Twitter, major platforms build on these REST principles, typically serving data as JSON. The emphasis on statelessness and caching makes these APIs incredibly scalable across distributed systems, with built-in support for infrastructure like proxies and CDNs. This standardized approach often leads to lower long-term maintenance costs compared to the varied implementations of Web APIs.
Now that we understand how REST APIs work, let's see how they stack up against their simpler cousins.
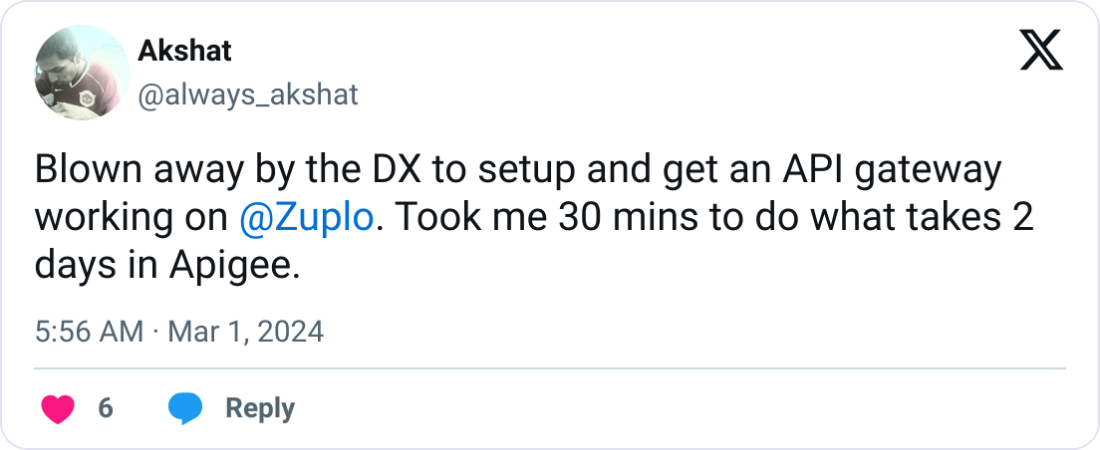
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreREST vs Web APIs: The Ultimate Showdown#
Here's the thing about APIs—not all are created equal. While REST APIs follow strict architectural rules, Web APIs give you the freedom to build however you please. It's like comparing a classical symphony to freestyle jazz—both make beautiful music, just differently.
Remember: all REST APIs are Web APIs, but not all Web APIs qualify as RESTful. REST follows specific constraints defined by Roy Fielding, while Web APIs include any application programming interface accessible over HTTP/HTTPS.
Let's break down the key differences:
Web APIs | REST APIs | |
---|---|---|
Protocols | Primarily use HTTP/HTTPS but can embrace other protocols when needed | Exclusively use HTTP/HTTPS with standardized methods (GET, POST, PUT, DELETE) mapped directly to CRUD operations |
Data Formats | Use whatever format works for your needs—XML, JSON, SOAP, or custom formats | Primarily use JSON today, optimizing for lightweight parsing and cross-platform compatibility |
Scalability | Offer flexibility for specialized needs or legacy integration, but typically require extra work to scale effectively | Built for straightforward horizontal scaling through stateless design—servers don't need to remember clients between requests |
Caching | May implement caching but lack REST's systematic approach to making responses reusable | Include built-in caching controls so CDNs and browsers can optimize response storage |
State Management | Allow server-side state management between requests, trading simplicity for scaling challenges | Require statelessness—each request must contain everything needed for processing |
Architecture | Offer flexible implementation patterns that might vary between endpoints | Follow uniform interface constraints with standardized resource identifiers |
Team Alignment | .NET developers can harness all the ASP.NET Web API features built into their framework | JavaScript teams often gravitate toward REST's JSON-focus, which aligns well with modern web development stacks |
Choose between these approaches based on your specific needs for scalability, caching requirements, and standardization—not just what's trending. REST APIs deliver consistency and scalability but sacrifice some flexibility, while Web APIs offer greater implementation freedom with fewer guarantees.
REST APIs accelerate your development through standardized tools and clear architectural guidelines, allowing your team to focus on building features instead of reinventing communication protocols. Web APIs offer speed when you need to ship fast or tackle unique requirements, especially for specific or internal use cases, by skipping unnecessary constraints.
Unleashing the Power of Simple Web APIs: When Less is More#
Simple Web APIs truly shine in situations where REST's strict principles would be overkill. Wondering if they're the right tool for your project? Let's explore when simplicity beats complexity and how to make the smart choice for your specific needs.
Perfect for Getting Sh*t Done#
Web APIs dominate in small to medium-sized applications where REST's full constraints would just slow you down. They work beautifully for:
- Internal tools with a limited user base
- Applications that need to ship yesterday
- Projects where simplicity trumps standardization
We've seen teams deploy internal apps in days using Web APIs where REST implementations would have taken weeks. Sometimes simple really is better.
Ship Faster, Refactor Later#
Skip the architectural overhead and ship your API faster with Web APIs. Your team can focus on building actual features instead of following architectural patterns. Teams consistently report faster development cycles when freed from REST constraints, especially when they're new to API development.
When CRUD Just Doesn't Cut It#
Web APIs handle operations that don't fit neatly into REST's resource model. They absolutely crush it with:
- Complex calculations and transformations
- Multi-step workflows with state
- Process-oriented services
- Operations that need to combine multiple resources
Sometimes business processes don't map cleanly to resources, and that's where Web APIs really shine.
Connecting Your Enterprise Spaghetti#
Web APIs adapt to your existing infrastructure instead of forcing standardization. This makes them perfect for:
- Legacy system integration (yes, that ancient COBOL system)
- Environments with mixed protocols
- Projects with varied data format requirements
- Specialized communication needs
Enterprise integration is messy. Web APIs help you cope with that reality.
Moving Big Binary Data Efficiently#
Web APIs handle binary data transfers like a boss, making them ideal for:
- Media streaming services
- File handling applications
- Raw data processing
- Direct binary format support without base64 bloat
We've deployed Web APIs that stream video content with custom protocols that would be impossible with strict REST principles.
Web APIs Win When You Need#
- A focused solution for a specific user base
- Quick internal tool deployment with minimal fuss
- Development speed over architectural purity
- Freedom from resource-based modeling constraints
- Support for multiple data formats in one API
- Complex enterprise integrations that just work
- Efficient binary data handling without the overhead
Our advice? Be pragmatic about REST compliance—implement REST principles where they add value, and keep things simple everywhere else. The key is building an API that serves your actual needs without unnecessary complexity.
But what about situations where REST truly shines? Let's switch gears and look at the flip side of this architectural coin.
5 Scenarios Where REST APIs Reign Supreme#
REST APIs deliver specific advantages when their architectural patterns align with your project needs. Here are five scenarios where REST APIs absolutely dominate:
1. Public-Facing Services#
REST APIs offer instant recognition and predictability for third-party developers through standardized patterns and a resource-oriented structure. Just look at GitHub's API. This clarity, combined with standardized tools and clear architectural guidelines, significantly accelerates development for internal teams, allowing them to focus on features. Teams have reported slashing development time by 40% after adopting solid REST patterns.
2. High-Scale Systems#
The stateless nature of REST APIs makes them perfect for high-scale environments. Since each request contains all needed information, servers don't track client sessions. This means you can:
- Scale horizontally by adding servers like Lego blocks
- Balance loads without sticky sessions
- Handle requests on any server for better fault tolerance
There's a reason Netflix runs on REST architecture to serve millions of concurrent users—they can scale API servers dynamically without complex session management headaches. REST's stateless design and caching capabilities make horizontal scaling straightforward.
3. Performance Through Caching#
REST's built-in caching delivers massive performance gains:
- Mark responses as cacheable or non-cacheable
- Leverage standard HTTP caching mechanisms
- Let CDNs cache responses automatically
This makes REST perfect for read-heavy apps with relatively stable data, like content delivery networks and news sites.
4. Microservices Architecture#
REST APIs form the backbone of microservices with their stateless nature, uniform interface, and clear service boundaries. A standardized REST approach pays off handsomely as you grow—one software company slashed API maintenance costs by 30% after transitioning to REST APIs, thanks to shared tooling and knowledge across teams.
5. Mobile Applications#
Mobile apps need efficient data transfer in bandwidth-constrained environments. REST APIs crush it here with lightweight JSON payloads that parse quickly on mobile devices, reducing battery drain and data usage.
When to Choose REST for Your API#
Consider REST for your project if you have:
- Resource-Based Data: Your domain maps naturally to resources with CRUD operations
- Growth Plans: Your API needs to scale horizontally for unpredictable traffic spikes
- Caching Needs: Your app benefits from HTTP's built-in caching mechanisms
- Long-Term Vision: You're building an API that needs to last for years
- Diverse Clients: Multiple platforms (web, mobile, IoT) will consume your API
These requirements make REST's architectural discipline worth the investment, delivering scalability, interoperability, and maintainability benefits that simpler web APIs just can't match.
Unleash Your API’s Power with Zuplo's Code-First Platform#
Wondering how to get your APIs deployed quickly without configuration headaches? Zuplo's code-first platform transforms API management for both RESTful and Web APIs by replacing complex configuration with straightforward code deployment. Write APIs in your preferred language and let Zuplo handle the infrastructure heavy lifting.
For REST APIs, Zuplo preserves those essential architectural principles like statelessness and uniform interfaces. Your API runs across 300+ global data centers, multiplying REST's inherent scalability benefits through edge execution.
Web APIs get all the same advantages plus deeper customization options. You can build custom auth flows, manage sessions, or create specialized caching—all through direct code rather than clicking through endless configuration screens, leveraging advanced API management strategies.
Getting your API live takes just four steps:
- Write your API logic in your language of choice
- Push your code to Zuplo
- Configure routing and edge deployment settings
- Watch your endpoints perform globally
The code-first approach truly excels for complex business logic and custom authentication that traditional configuration tools simply can't handle. By leveraging a hosted API gateway, you can benefit from managed infrastructure and focus on code.
Managing both REST and Web APIs on one platform standardizes your monitoring, auth, and deployment while preserving each API type's unique benefits.
Future-Proof Your Project: Picking the Perfect API Approach#
REST and Web APIs excel in different scenarios. Success comes from matching the right tool to your actual needs. REST APIs deliver standardization, scalability, and universal accessibility through stateless design and built-in caching, making them ideal for high-traffic services and mobile apps. Web APIs offer flexibility and simpler development for enterprise environments and specialized cases where REST's constraints might slow you down.
Choose based on your team's skills, project requirements, and business goals.
Start building better APIs today with Zuplo's code-first platform and implement the approach that best fits your specific project needs, without the configuration overhead.