QuickBooks, one of the leading accounting software solutions, provides businesses with the tools they need to manage their finances effectively. The QuickBooks API opens up this powerful platform to developers, allowing seamless integration of QuickBooks functionalities into custom applications. This connection transforms complex financial data management into streamlined workflows, reduces manual data entry, and enhances operational efficiency across the business.
By connecting QuickBooks with other systems through its API, companies break down technical barriers and ensure financial data flows exactly where it's needed. Organizations increasingly want to integrate accounting capabilities into everything from e-commerce platforms to CRM systems, and QuickBooks' developer ecosystem has evolved to meet these demands.
Understanding QuickBooks API#
The QuickBooks API is a robust interface that allows developers to programmatically access the accounting features of QuickBooks Online. It provides access to essential financial functionalities, including managing customers, vendors, invoices, payments, expenses, and reports through standardized endpoints.
This API follows REST principles with JSON-formatted responses, making it accessible across various programming languages. Here's what a basic API response might look like when retrieving customer information:
{
"Customer": {
"Id": "1",
"DisplayName": "ABC Company",
"PrimaryEmailAddr": {
"Address": "contact@abccompany.com"
},
"PrimaryPhone": {
"FreeFormNumber": "(555) 555-5555"
},
"Balance": 1250.0
},
"time": "2023-05-18T10:30:45.000Z"
}
Instead of building accounting systems from scratch, you can tap into QuickBooks' established infrastructure, inheriting reliable financial management capabilities while concentrating on your core business processes.
Key Benefits of QuickBooks API Integration#
QuickBooks API integration offers significant time savings by accessing established accounting functions rather than developing financial management solutions from scratch. Companies can automate financial processes, including invoicing, payment processing, customer data synchronization, and business workflows with real-time financial information.
Perhaps most importantly, when applications connect directly to QuickBooks services, companies avoid the cost and complexity of maintaining separate accounting systems while ensuring accurate financial data.
Getting Started with QuickBooks API#
To begin, you'll need access credentials through a QuickBooks developer account. Start at the QuickBooks Developer Portal and create a free account. After verification, create a new app to obtain your API keys.
During app creation, you'll select sandbox or production environments based on your development stage. The platform generates your unique Client ID and Client Secret. These credentials that must be kept secure and used with all API requests.
Accessing the API Explorer and Documentation#
The QuickBooks API documentation provides comprehensive guidance for all available endpoints. Resources are organized by type—customers, invoices, payments—with sample requests, expected responses, and limitations.
QuickBooks also provides detailed guidance on authentication methods, error handling, and performance optimization best practices.
Developing with QuickBooks API#
QuickBooks API uses OAuth authentication methods for secure access. Your application obtains an access token using your Client ID and Client Secret, allowing authorized API access without exposing sensitive user credentials.
For making API calls, include the access token in the Authorization header of your HTTPS requests. Here's an example of what a request to retrieve customer information might look like:
// Example GET request to retrieve customer information
const axios = require("axios");
async function getCustomer(customerId, accessToken, realmId) {
try {
const response = await axios.get(
`https://quickbooks.api.intuit.com/v3/company/${realmId}/customer/${customerId}`,
{
headers: {
Authorization: `Bearer ${accessToken}`,
Accept: "application/json",
},
},
);
return response.data;
} catch (error) {
console.error("Error fetching customer:", error);
}
}
Creating a new invoice through the API requires sending a POST request with the invoice details in JSON format:
// Example POST request to create a new invoice
async function createInvoice(invoiceData, accessToken, realmId) {
try {
const response = await axios.post(
`https://quickbooks.api.intuit.com/v3/company/${realmId}/invoice`,
invoiceData,
{
headers: {
Authorization: `Bearer ${accessToken}`,
"Content-Type": "application/json",
},
},
);
return response.data;
} catch (error) {
console.error("Error creating invoice:", error);
}
}
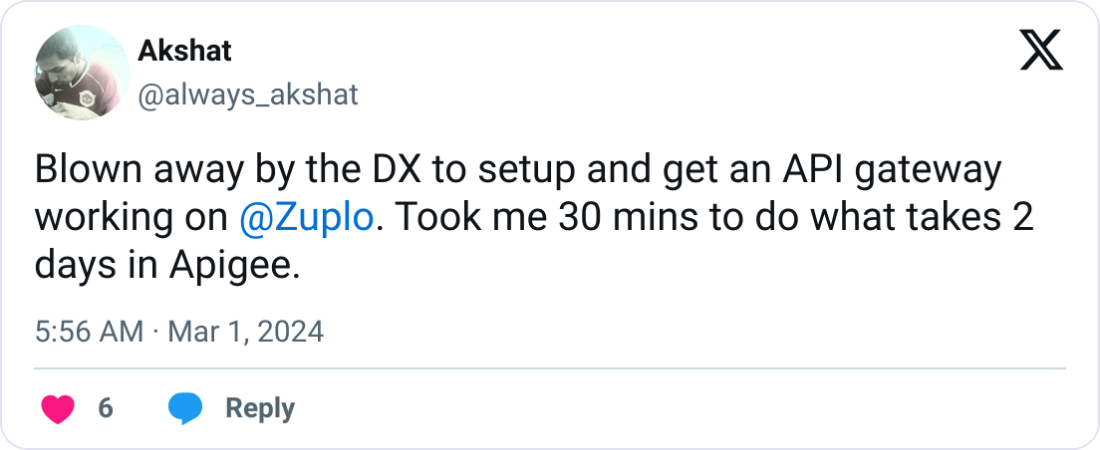
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreTesting Your Application#
Before going live, thoroughly test your integration using QuickBooks' sandbox environment. The sandbox provides access to a test company with sample data, perfect for testing without impacting real financial data.
Test both successful transactions and error scenarios: invalid inputs, network interruptions, and expired tokens. The testing process also helps establish performance expectations, though real-world performance may vary based on network conditions and data volume.
Common Development Tools and SDKs#
While direct API calls work fine, QuickBooks offers official SDKs for popular languages to speed up development. Here's an example of using the Node.js SDK to create a customer:
// Example using the Node.js SDK to create a customer
const QuickBooks = require("node-quickbooks");
const qbo = new QuickBooks(
clientId,
clientSecret,
accessToken,
refreshToken,
realmId,
false, // sandbox = false (production)
true, // debug = true
);
const customerData = {
DisplayName: "New Customer LLC",
PrimaryEmailAddr: {
Address: "customer@example.com",
},
};
qbo.createCustomer(customerData, function (err, customer) {
if (err) {
console.log(err);
return;
}
console.log("Customer created: ", customer.Id);
});
These SDKs handle many integration complexities, including OAuth 2.0 authentication, request formatting, and error parsing.
Webhooks and Real-Time Data#
QuickBooks supports webhooks for event-driven integrations, notifying your application in real-time when certain events occur in QuickBooks. Here's an example webhook handler that processes payment notifications:
// Example webhook handler for payment notifications
app.post("/webhooks/quickbooks", (req, res) => {
// Verify webhook signature
const signature = req.headers["intuit-signature"];
if (!verifyWebhookSignature(signature, req.body, webhookSecret)) {
return res.status(401).send("Invalid signature");
}
const event = req.body;
// Handle payment event
if (event.eventType === "PAYMENT") {
console.log("Payment received:", event.payload);
// Process payment notification
// e.g., update order status, notify customer, etc.
}
res.status(200).send("Webhook received");
});
To implement webhooks, register your endpoint URLs in your QuickBooks app settings and subscribe to the events you want to monitor.
Batch Operations and Data Queries#
For applications working with large datasets, the QuickBooks API supports batch processing to efficiently handle multiple operations in a single request. Here's an example of a batch request:
{
"BatchItemRequest": [
{
"bId": "1",
"operation": "create",
"Invoice": {
"Line": [
{
"Amount": 100.0,
"DetailType": "SalesItemLineDetail",
"SalesItemLineDetail": {
"ItemRef": {
"value": "1",
"name": "Services"
}
}
}
],
"CustomerRef": {
"value": "1"
}
}
},
{
"bId": "2",
"operation": "update",
"Customer": {
"Id": "2",
"DisplayName": "Updated Customer Name"
}
}
]
}
These optimization strategies reduce overall processing time and costs, especially for applications handling substantial volumes of financial data.
Common Challenges and Troubleshooting#
Rate limiting presents a common obstacle, as QuickBooks enforces usage limits. You’ll need to implement retry strategies with exponential backoff and cache frequently accessed data to reduce API calls. This example shows a simple retry strategy:
// Example retry strategy for API requests
async function makeRequestWithRetry(requestFn, maxRetries = 3) {
let retries = 0;
while (retries < maxRetries) {
try {
return await requestFn();
} catch (error) {
if (error.response && error.response.status === 429) {
// Rate limit exceeded
retries++;
const waitTime = Math.pow(2, retries) * 1000; // Exponential backoff
console.log(`Rate limit exceeded. Retrying in ${waitTime}ms...`);
await new Promise((resolve) => setTimeout(resolve, waitTime));
} else {
throw error; // Re-throw other errors
}
}
}
throw new Error("Maximum retries exceeded");
}
Data consistency is critical in accounting applications. Implement checks to prevent duplicate entries, validate data before sending, and handle error responses appropriately.
Troubleshooting Tips and Resources#
Common error scenarios include:
- 401 Unauthorized: Authentication failure (invalid token)
- 400 Bad Request: Malformed request (invalid JSON or missing required fields)
- 429 Too Many Requests: Rate limit exceeded
- 500 Internal Server Error: Server errors (temporary issues)
For persistent problems, the QuickBooks Developer Community offers support from both Intuit staff and experienced developers.
Exploring Alternatives to the QuickBooks API#
While QuickBooks dominates the accounting software market, several alternatives offer API capabilities worth considering:
- Xero API provides similar accounting functionalities with a comprehensive REST API. Many developers find Xero's documentation and developer experience excellent, making it a strong contender especially for businesses already using Xero's accounting platform.
- FreshBooks API offers a user-friendly interface for service-based businesses. Its API is particularly strong for time-tracking, project management, and client billing scenarios.
- Sage Intacct API targets mid-market and enterprise businesses with more complex accounting needs. If your application requires multi-entity consolidation or advanced financial reporting, this might be a better fit.
- Wave API provides free accounting services for small businesses, though its API capabilities are more limited than commercial alternatives.
When selecting between QuickBooks and alternatives, consider not just the technical aspects of the API but also which accounting system your target users are likely to use, as this significantly impacts adoption.
QuickBooks API Pricing#
QuickBooks API access comes with different subscription tiers based on your QuickBooks Online plan. Access to the API is generally included in your QuickBooks Online subscription, but usage limits and available features may vary.
For higher transaction volumes or advanced features, businesses might need to upgrade to a higher-tier QuickBooks Online plan. Enterprise customers with custom requirements can access additional capabilities through partnership arrangements, including dedicated support channels and custom agreements tailored to specific business needs.
All tiers provide access to the same API structure, with differences primarily in usage limits and available features rather than API functionality.
QuickBooks API Makes Complex Financial Tasks Accessible#
By connecting QuickBooks' accounting capabilities with other systems, companies gain efficiencies and insights impossible with traditional manual processes. Whether you're building e-commerce platforms or creating business management solutions, the QuickBooks API provides a foundation for innovation without sacrificing accuracy.
For enterprise-grade API management that enhances your QuickBooks integration, consider Zuplo's API gateway. Book a demo today to discover how it can add security, performance, and monitoring capabilities to your financial integrations.