If you work with APIs or any form of data exchange, you've likely encountered JSON (JavaScript Object Notation). JSON is a popular format for transmitting structured data because it is lightweight and easily understandable. However, as your projects scale, the complexity of your JSON data can also increase. This is where JSON Schema becomes invaluable.
Think of JSON Schema as a blueprint for your JSON data. It defines the structure, the types of values allowed, and even specific formats or constraints. By verifying your JSON against its schema, you gain confidence that can save you from countless future headaches. Bugs are caught early, integrations go more smoothly, and your documentation becomes self-validating.
This guide will provide an overview of JSON Schema, covering everything from the basics to advanced techniques, giving you the tools to validate your JSON and design robust schemas that will enhance your data-driven projects. Whether you're an experienced API developer or new to structured data, understanding JSON Schema will lead to cleaner code, improved data reliability, and happier users.
What is JSON Schema and its Importance in Validating JSON Data#
At its core, JSON Schema is a language for describing the structure and constraints of JSON data. Think of it as a contract or a set of rules your JSON must adhere to. It uses a JSON-based format itself, which means it's as easy to read and write as the data it's designed to validate. Here are a few key reasons you should use JSON Schemas:
Data Integrity: Schemas ensure your JSON data is well-formed and meets your expectations. This helps prevent errors that could arise from malformed or unexpected data.
Early Bug Detection: By validating your JSON against a schema early in the development process (or even as part of automated testing), you can catch issues before they become major problems.
Clear Documentation: A well-defined schema is excellent documentation. It clearly explains the expected structure and content of your JSON data, making it easier for others (or yourself in the future) to understand and work with.
Automation: JSON Schema validation can be integrated into your tools and workflows, providing automatic checks on incoming or outgoing data.
JSON Schema validation is an essential component of effective data management. It's a proactive way to maintain data quality, improve collaboration, and build more robust applications.
Creating and Understanding JSON Schemas#
A JSON schema is a JSON object, making it easy to work with. At its core, it uses keywords to define the structure and validation rules for your JSON data. The type keyword is fundamental, specifying the expected data type, which can be a string, number, integer, boolean, object (for nested structures), array, or null. When dealing with JSON objects, the properties keyword allows you to define a nested schema for each property, specifying its structure and constraints. For arrays, the items keyword dictates the schema that each item within the array must conform to. The required keyword is an array listing the property names that are mandatory within your JSON object. Let's take a look at an example of a valid schema definition next.
{
"$schema": "https://json-schema.org/draft/2020-12/schema",
"type": "object",
"properties": {
"name": { "type": "string" },
"age": { "type": "integer", "minimum": 0 },
"email": { "type": "string", "format": "email" }
},
"required": ["name", "email"]
}
In this example, we define a schema for an object that has properties name (a string), age(an integer greater than or equal to 0), and email (a string that must follow the email format). The name and email properties are required.
Understanding JSON Schema Keywords and Advanced Features#
JSON Schema defines a rich set of keywords to express various constraints and validation rules for your data. You can use minimum and maximum to set limits on numbers, minLength and maxLength to control string lengths, and pattern to enforce specific formats with regular expressions. The enum keyword lets you restrict a value to a set of allowed choices. And for common formats like email addresses, dates, or URLs, the format keyword is your go-to. Keywords like anyOf, allOf, and oneOf let you create more complex validation logic.
The capabilities of JSON Schema extend far beyond the basic keywords. As you dive deeper into its functionality, you'll encounter features like references (using the $ref keyword) that enable you to reuse sections of your schema, promoting modularity and reducing duplication. You'll also have the flexibility to define custom validation keywords, tailoring the schema language to your specific requirements. Conditional logic (using if, then, else) allows you to apply different validation rules based on the context of your data. Using these advanced features, you can develop schemas that accurately model even the most intricate data structures.
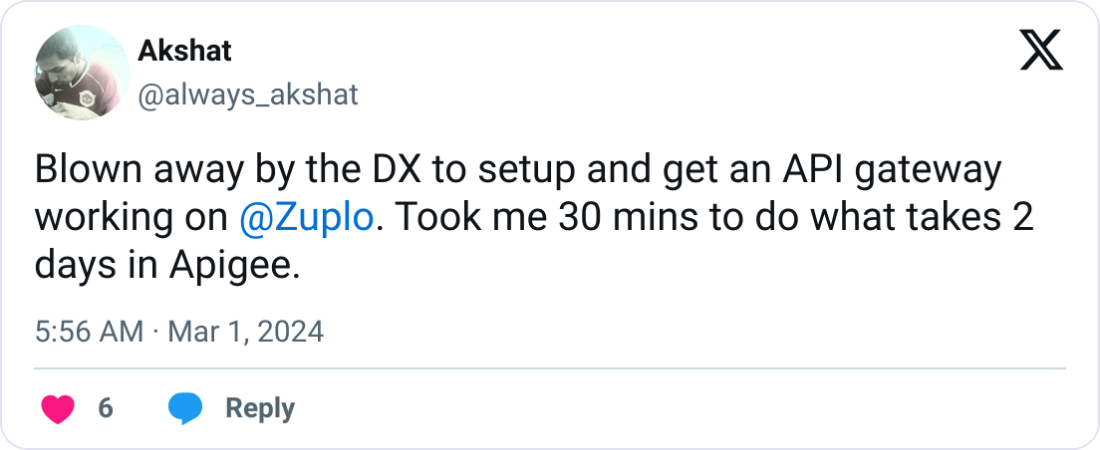
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreWorking with JSON Data and Schemas#
Now that we have a schema, let's create JSON data and see if it passes the validation test.
{
"name": "Jane Smith",
"age": 30,
"email": "jane.smith@example.com"
}
This JSON object seems to match the structure defined in our schema, it has the required properties name and email, and the age is a positive integer. To validate the JSON data against the schema, you'll need a JSON Schema validator. Many libraries and tools are available in various programming languages, we'll explore some popular options shortly.
Using JSON Schemas to Validate JSON Data in Different Scenarios#
JSON Schema validation isn't just for one-off checks. One common use case is API request/response validation. By ensuring incoming requests adhere to the correct structure before processing and validating outgoing responses against the defined schema, you enhance the reliability and predictability of your API interactions. In data transformation and ETL (Extract, Transform, Load) pipelines, JSON Schema validation plays a crucial role in verifying the structure of data as it moves between systems. This early error detection prevents downstream issues, ensuring the integrity of your data throughout the transformation process.
JSON Schema is invaluable for validating configuration files, which are often a JSON document. By enforcing the correct structure and values within configuration files, you enhance the robustness of your applications and prevent misconfigurations that could lead to unexpected behavior. When dealing with user input in web applications, validating the provided JSON data against a schema before storing or processing it is essential. This practice helps maintain data quality and allows you to provide immediate feedback to users if their input doesn't align with expectations.
JSON Schema validation is a versatile tool that can be applied in numerous ways to enhance the reliability and robustness of your data-driven applications.
Advanced JSON Schema Topics#
As your JSON schemas become more complex, leveraging advanced features is crucial for maintaining clarity and effectiveness. References ($ref) allow you to reuse schema definitions, eliminating redundancy and simplifying complex data structures. Conditional logic (if, then, else) introduces flexibility by applying validation rules based on specific property values. Additionally, schema composition (allOf, anyOf, oneOf) enables you to create sophisticated validation rules by combining multiple schemas, ensuring your data adheres to various criteria simultaneously.
In this example, the address property is defined once in $defs and reused throughout the schema.
{
"$schema": "(https://json-schema.org/draft/2020-12/schema)",
"type": "object",
"properties": {
"address": { "$ref": "#/$defs/address" }
},
"$defs": {
"address": {
"type": "object",
"properties": {
"street": { "type": "string" },
"city": { "type": "string" }
// ... other address properties
},
"required": ["street", "city"]
}
}
}
Here, the guardian property is required only if the age is less than 18.
{
"type": "object",
"properties": {
"age": { "type": "integer" },
"guardian": { "type": "string" }
},
"if": { "properties": { "age": { "maximum": 17 } } },
"then": { "required": ["guardian"] }
}
This schema allows a string with a maximum length of 10 or a positive number.
{
"anyOf": [
{ "type": "string", "maxLength": 10 },
{ "type": "number", "minimum": 0 }
]
}
Implementing JSON Schema Validation#
To verify whether your JSON data adheres to your schema, you'll need a JSON Schema validator. These tools analyze both your schema and data to determine if the data conforms to the rules defined in the schema. Various libraries and tools are available for implementing JSON Schema validation, catering to different programming languages, and offering diverse features. Here are some popular libraries and tools:
-
JavaScript:
- Ajv: A fast and popular validator with extensive features and customization options.
- Zod: A TypeScript-first schema validation library that integrates well with modern JavaScript frameworks.
-
Python:
- jsonschema: A well-maintained and feature-rich library for Python.
- fastjsonschema: A high-performance validator optimized for speed.
-
Java:
- JSON Schema Validator: A comprehensive validator that supports various JSON Schema drafts.
- Everit: Another solid option with good support for custom formats and keywords.
The following steps highlight the basic validation flow when JSON Schema is used to validate JSON documents.
- Load Your Schema: Read your JSON schema document from a file or string.
- Instantiate the Validator: Create an instance of your chosen validator library, passing in the schema.
- Validate Your Data: Call the validator's validation function, passing in the JSON data you want to check.
- Handle the Results: The validator will typically return a boolean indicating whether the data is valid. If it's not, you can usually access detailed error messages explaining the validation failures.
In practice, here's an example using the JavaScript library Ajv.
const Ajv = require("ajv");
const ajv = new Ajv();
const schema = {
// ... (your JSON schema)
};
const validate = ajv.compile(schema);
const data = {
// ... (your JSON data)
};
const valid = validate(data);
if (!valid) {
console.log(validate.errors);
}
Tips for Efficient Validation of Multiple JSON Documents#
When optimizing JSON Schema validation for multiple documents, consider several approaches. Caching the compiled validation function is recommended for repeated validation against the same schema, significantly reducing processing time and avoiding unnecessary recompilation. For scenarios with multiple documents, utilizing libraries that support batch validation can enhance efficiency. Incremental validation techniques are advantageous for large JSON documents, allowing validation of data segments as they're processed instead of waiting for the entire document to load. By choosing the right validator and employing these optimization techniques, you can make JSON Schema validation a seamless part of your development process, ensuring the quality and reliability of your JSON data.
Best Practices for Using JSON Schemas to Validate JSON Data#
While JSON Schema provides a powerful framework for data validation, employing best practices is important to maximizing its effectiveness and maintaining the health of your schemas. These practices help ensure your schemas are robust, adaptable, and easy to understand, leading to more reliable data validation and smoother development processes.
-
Be Specific: Define your schema as strictly as possible. The more specific you are, the more errors you'll catch and the more reliable your validation will be.
-
Use the Right Version: Specify the JSON Schema draft you're using in the $schema keyword. This ensures consistent behavior across validators.
-
Leverage Built-in Formats: JSON Schema provides many standard formats (e.g., email, date, uri). Use them to simplify your schemas and improve validation accuracy.
-
Keep it DRY(Don't Repeat Yourself): Use references ($ref) to reuse common schema definitions and avoid redundancy.
-
Start Simple, Iterate: Begin with a basic schema and gradually add complexity as needed. Avoid over-engineering your schemas upfront.
-
Test Thoroughly: Write comprehensive tests for your schemas to ensure they correctly validate valid and invalid data.
-
Use Descriptive Error Messages: When validation fails, provide informative error messages that pinpoint exactly what's wrong and how to fix it.
-
Automate: Integrate JSON Schema validation into your automated tests and build processes to catch errors early.
-
Document Your Schemas: Add clear comments and descriptions to your schemas to make them self-explanatory and easier for others to understand.
-
Consider Versioning: If your schema is likely to change over time, consider using a versioning strategy (e.g., semantic versioning) to manage compatibility.
Using JSON Schema to Validate API Requests in Zuplo#
One great part about JSON Schemas is their versatility in the world of API development. With Zuplo, you can use your JSON Schema to validate incoming requests to your API, ensuring that the request body matches the expected format.
To do this, in Zuplo you can add your JSON Schema to your OpenAPI spec to ensure that the data your API will use is described. With your OpenAPI doc complete with JSON schema, you can then use the Request Validation Inbound policy that will check the incoming request against the schema and block it from hitting the upstream API if the format does not match.
Even better, if you are exposing your APIs through the Zuplo Developer Portal, Zuplo can use the JSON Schema and any examples in it to generate API documentation and examples in the portal. This can help make developers' lives easier by giving them accessible examples to ease their usage of your APIs.
Conclusion#
JSON Schema brings order, predictability, and reliability to your JSON data projects. By mastering this tool, you can validate your data to ensure its integrity and correctness, preventing errors before they become problems. You can also use JSON Schema to create precise, self-describing specifications for your data formats, making collaboration and understanding easier among your team. When you integrate validation into your workflows, you can catch issues early in development, streamlining the entire process. Using JSON Schema allows you to establish a common language for data exchange, simplifying integration between systems and reducing the chance of misinterpretations.
Whether you're building APIs, working with configuration files, or managing complex data pipelines, JSON Schema is an indispensable tool. It allows you to create more robust applications, deliver better user experiences, and maintain the highest data quality standards.
Want to leverage JSON Schema within your APIs? Sign up for Zuplo today to use JSON schema to enforce request validation and generate examples and docs in minutes to make your APIs easier to use and manage.