Have you ever noticed how some apps feel lightning-fast while others make you want to throw your phone across the room? That split-second delay—or lack thereof—often comes down to API latency. Every millisecond counts when data travels from client to server and back, and if that journey takes too long, user trust evaporates faster than morning dew.
The good news? You can take control of latency by implementing smart strategies to give your application the snappy response times it deserves. In this guide, building on API fundamentals, we'll explore everything you need to know about API latency—what causes it, how to measure it accurately, and, most importantly, proven techniques to optimize it. Let's dive in.
Understanding API Latency: More Than Just “Slowness”#
When we talk about API latency, we're specifically focusing on the travel time of requests and responses—the journey data takes across the network. This differs from API response time, which includes both travel time and server processing. Making this distinction helps developers pinpoint exactly where improvements are needed.
Think of latency like a road trip. The speed limit (bandwidth) matters, but so does the distance traveled, traffic congestion, and number of stops along the way. When you understand each part of the journey, you can make smarter decisions about which routes to optimize.
Several key elements shape latency:
Network Latency: This is the physical distance and conditions your data must navigate. Bandwidth limitations and network congestion can create traffic jams for your data, while inefficient routing might send packets on unnecessary detours. Implementing solutions like CDNs and hosted API gateways can address these issues by caching content and optimizing network traffic.
Server Processing Time: Once your request arrives at its destination, the server needs to do its job—running computations, querying databases, and assembling a response. How quickly this happens depends on the efficiency of your hardware, architecture, and code.
Queuing Time: During traffic spikes, your server might become overwhelmed with incoming requests, creating a virtual waiting line. Effective load balancing and auto-scaling help manage these queues and keep things moving.
Client Processing Time: After the response arrives, the client still needs to parse and render the data. Well-structured responses and efficient client-side code ensure this final step doesn't introduce additional delays.
By separating latency from total response time, you gain clarity about whether slowdowns stem from network conditions, server processing bottlenecks, or both. This helps you target your optimization efforts where they'll have the greatest impact.
Common Causes of Elevated API Latency#
Let's look at what typically causes latency issues and slows down your APIs.
Network Issues#
The physical distance between your users and servers creates an unavoidable baseline latency. When your users are halfway around the world from your servers, that round trip naturally takes longer. Inefficient routing paths can make matters worse, sending your data through multiple unnecessary hops before reaching their destination, adding precious milliseconds each time.
CDNs help address this by caching content at locations physically closer to your users. By strategically placing your static assets around the globe, you can dramatically cut down on that physical distance constraint, especially for assets that don't change frequently.
Another strategy is to optimize your network protocols. For example, HTTP/2 and HTTP/3 offer significant improvements over older protocols by allowing multiple simultaneous requests over a single connection and reducing handshake times. This is particularly valuable for mobile users or those on less reliable connections.
Server Overload#
Your servers have finite resources, and when demand exceeds capacity, performance suffers. This commonly happens during traffic surges like product launches, flash sales, or when your latest feature goes viral on social media. Your server can only process so many requests before things start to slow down. Signs of server overload include increased response times, failed requests, and—in worst cases—complete system crashes.
To prevent this, implement load balancing to distribute traffic across multiple servers, auto-scaling to add resources during peak times, and optimize your code to make the most efficient use of available resources. Techniques like implementing rate limiting also help protect servers from overload. Modern cloud platforms make this easier than ever with services that dynamically adjust your capacity based on current demand. Additionally, focusing on improving API performance through code optimization can help manage server resources efficiently.
Database connection pooling is another technique that helps manage server resources efficiently. By maintaining a pool of open connections, you avoid the overhead of establishing new database connections for each request, significantly reducing latency during high-traffic periods.
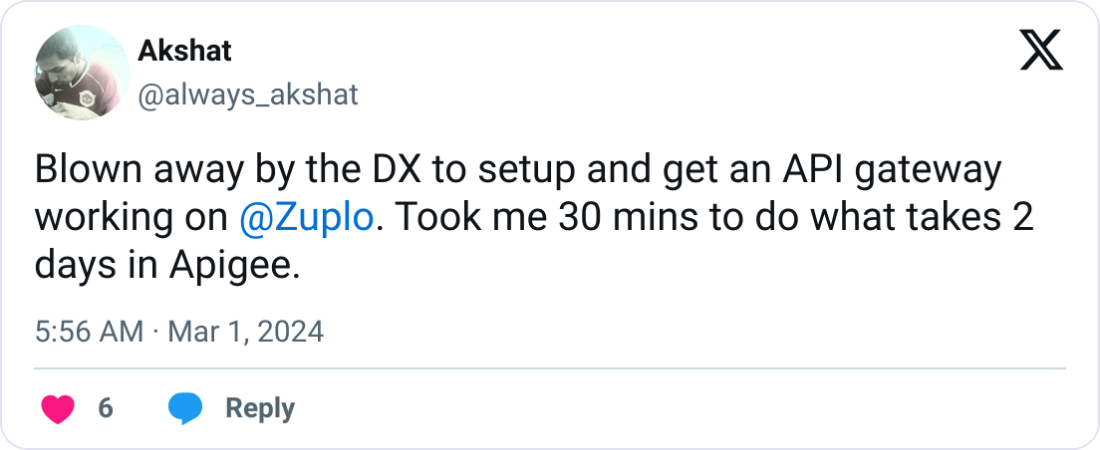
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreInefficient Code#
Sometimes, the problem isn't external factors but inefficiencies in your own code. Every unnecessary operation adds up, especially at scale. Common culprits include:
- Unindexed database queries that force systems to scan entire tables when they could be using optimized lookup paths
- Nested loops or recursive functions that multiply processing time, especially when dealing with large datasets
- Redundant data calls that repeat work unnecessarily or fetch the same information multiple times
- Memory leaks that gradually consume available resources until the system slows to a crawl
- Synchronous operations that block the execution thread when they could be running asynchronously
Strategic code optimization—streamlining algorithms, indexing databases, and eliminating unnecessary operations—can dramatically improve performance without changing your hardware or infrastructure. At the same time, remember that premature optimization can be counterproductive. Start by building features that work correctly and then measure to identify actual bottlenecks, rather than making assumptions about where problems might be.
Measuring API Latency: You Can't Improve What You Don't Measure#
Before implementing optimizations, you need an accurate picture of your current performance. Good measurement provides baseline metrics and helps identify specific bottlenecks. Effective API performance testing helps achieve this objective.
To get meaningful data that reflects real-world conditions:
- Simulate authentic user conditions by testing with different network speeds and from various geographic locations. Consider using services that allow testing from multiple regions to understand how your global users experience your API.
- Vary your test scenarios to include both typical usage patterns and edge cases that might reveal unexpected performance issues. Test with different payload sizes and request frequencies to understand how your system responds under various loads.
- Monitor latency over time to identify trends and patterns before they become critical problems. Look for cyclical patterns (daily, weekly, monthly) and correlate spikes with known events or changes to your infrastructure.
- Track error rates alongside latency to understand the relationship between performance and reliability. Sometimes, APIs appear fast only because they're failing quickly rather than completing successfully.
- Define acceptable thresholds and set up alerting when performance falls below these standards. Consider using percentiles rather than averages, as they better represent the user experience.
- Implement distributed tracing across your services to see exactly where time is being spent as requests flow through your system. This helps pinpoint specific components causing delays.
Remember that one-time tests only provide a snapshot—continuous monitoring gives you the full picture of how your APIs perform across different conditions and over time. The goal isn't just fast performance in ideal conditions, but consistent reliability under real-world usage.
Technical Strategies for Optimizing API Latency#
Now for the part you've been waiting for—how to make your APIs faster! Here are proven strategies that can significantly reduce latency:
Edge Computing: Bring Processing Closer to Users#
Edge computing is ideal for speeding up APIs. By deploying APIs on the edge, instead of sending everything to faraway servers, data is processed closer to your users. Less travel distance means faster responses—we're talking about cutting wait times from hundreds of milliseconds to just single digits.
The benefits are clear across various industries. E-commerce platforms deliver instant AI-driven personalized recommendations, financial services process transactions with minimal delay and reduced fraud risk, and IoT applications analyze sensor data locally before sending only what matters to the cloud. Your users might not know why your app suddenly feels more responsive, but they'll definitely appreciate the difference!
To delve deeper into edge computing strategies, explore Edge Computing best practices.
Code Efficiency: Streamline Your Requests#
Smart coding practices can work wonders for API latency without requiring massive infrastructure changes. It's all about making your requests work smarter, not harder.
Consider bundling multiple requests together when possible. Tools like Webpack and Rollup are great for asset bundling, while GraphQL lets users grab exactly what they need in one efficient query. Client-side caching using IndexedDB or localStorage can eliminate network requests altogether for information that doesn't change often.
Don't underestimate the power of HTTP persistent connections either! Keeping connections open saves you from repeatedly going through that time-consuming TCP handshake dance. And always keep an eye on your payload size—compression, efficient data formats, and trimming unnecessary fields can significantly lighten the load.
Caching Strategies: Don't Repeat Yourself#
If there's a latency-reduction superhero, it's definitely caching. Nothing beats avoiding a request completely! Understanding and implementing effective caching strategies is key. Browser-based client-side caching stores resources locally, making repeat visits lightning fast. Server-side caching keeps frequently accessed data in memory, helping you avoid expensive database queries. For relatively static endpoints, full response caching with tools like Redis can deliver complete API responses in record time.
And don't forget about CDNs. Services like Cloudflare, Akamai, or Fastly specialize in delivering cached content from locations all around the world, bringing your data closer to users no matter where they are.
The trick with caching is finding that sweet spot between performance and freshness. Cache too aggressively and users might see outdated information. Too conservatively, and you miss out on the speed benefits. Finding that balance takes some experimentation, but the performance gains are well worth the effort.
Monitoring and Continued Optimization#
Optimizing API latency isn't a one-time project but an ongoing process. Establish continuous monitoring to maintain peak performance as conditions change.
Define Your Metrics#
Start by identifying which metrics matter most for your specific application. Response time percentiles capture the experience of your slowest requests, while throughput measurements help you understand your system's capacity under load. Error rates reveal reliability issues that often correlate with performance problems, and uptime tracking ensures you're monitoring overall system availability. These metrics together provide a comprehensive view of your API performance.
Implement Proactive Monitoring
Don't wait for user complaints to identify problems. Set up real-time dashboards that visualize latency across different endpoints and configure alerts for when performance crosses predefined thresholds. Adopting proactive monitoring strategies can help establish these early warning systems to address issues before they impact users or escalate into outages.
By correlating traffic patterns with latency spikes, you can identify potential bottlenecks and understand how your system performs under different conditions, letting you anticipate problems before they occur. Utilizing the right API monitoring tools is essential for this process.
Link Performance to Business Outcomes#
The most effective optimization efforts connect technical metrics to business KPIs. Map latency improvements to conversion rates, user engagement, or retention to demonstrate tangible value beyond technical excellence. This connection helps justify investment in performance optimization and ensures you're focusing on improvements that matter to your business.
Categorize metrics by their operational impact and prioritize optimizations that directly support business objectives. When engineering efforts align with organizational goals, they deliver measurable value beyond purely technical improvements, creating a virtuous cycle where performance optimization contributes directly to business success.
Future Trends in API Latency Optimization#
The landscape of API optimization continues to evolve. Here are emerging trends worth watching:
AI-Driven Optimization#
Machine learning algorithms can now predict traffic patterns before they occur, automatically identify and mitigate performance bottlenecks, and optimize resource allocation in real-time. These capabilities allow systems to proactively adjust rather than merely reacting to problems after they emerge.
GraphQL and Tailored Responses#
GraphQL continues to gain adoption by allowing clients to request exactly the data they need in a single query. This reduces overfetching and underfetching, optimizing both bandwidth usage and processing time. When choosing the right API design, it's important to consider how different architectures affect latency and performance. Additionally, tools that allow for generating APIs from databases can streamline development and improve efficiency.
WebSocket APIs for Real-Time Applications#
For applications requiring continuous data exchange, WebSockets provide persistent connections that eliminate the overhead of repeated HTTP requests. This approach significantly reduces latency for chat applications, collaborative tools, and live dashboards.
Predictive Prefetching#
By analyzing user behavior patterns, applications can predict and preload resources before they're explicitly requested. This technique effectively hides latency by having data ready before users ask for it.
Your API Latency Action Plan#
Speed isn't just a nice bonus—it's the heartbeat of great user experience that drives real business results. When your APIs snap to attention quickly, users stick around and you edge out the competition. We've covered a lot of ground here—from edge computing to smart caching, code tweaks to monitoring tricks—giving you a complete toolkit to tackle latency from all sides.
So what's your next move? Start by getting a clear picture of where you stand today. Find those performance bottlenecks that are slowing you down, target them with the right fixes, and keep an eye on things with good monitoring. Ready to kick your API performance into high gear? Give Zuplo's developer-friendly platform a try.