NASA gathers an impressive 15 Terabytes of data daily, and thanks to a White House mandate, this wealth of information is available to the public through the robust NASA API ecosystem. Whether you're looking to track near-Earth asteroids, access stunning space imagery, or monitor Earth's changing climate, the NASA API provides developers with a universe of possibilities.
The NASA API serves as a critical solution to the challenge of distributing, using, and reusing NASA's vast data collections. By making this information accessible through standardized APIs, NASA has significantly lowered the barrier to entry for developers, researchers, and space enthusiasts alike.
From the popular Astronomy Picture of the Day (APOD) to specialized services like the Near Earth Object Web Service (NeoWs), NASA offers diverse endpoints that can power applications across educational, research, media, and commercial domains. With a simple API key from api.nasa.gov, you can begin exploring space data and creating inspiring applications. Let’s dig in to this topic a bit more. 🛰️
What is the NASA API?#
The NASA API is a digital interface that allows developers, researchers, students, and space enthusiasts to programmatically access NASA's extensive datasets. For anyone understanding APIs, it opens up a wealth of possibilities. This API significantly lowers the barriers for external users to interact with public information that would otherwise be difficult to obtain or utilize.
Overview of Available NASA APIs#
NASA offers several specialized APIs within their ecosystem:
- Astronomy Picture of the Day (APOD): Delivers high-resolution images of astronomical objects with scientific explanations.
- Near Earth Object Web Service (NeoWs): Provides information about near-Earth asteroids, including orbits and potential hazards.
- Earth Polychromatic Imaging Camera (EPIC): Offers full-disc imagery of Earth taken from space.
- Earth Observatory Natural Event Tracker (EONET): Delivers metadata on natural events, including storm imagery.
- NASA Image and Video Library: Grants access to NASA's extensive collection of space-related media.
These varied APIs open up a universe of possibilities for developers!
Getting Started with the NASA API#
Step-by-Step Guide to Obtaining an API Key#
- Visit the NASA API Portal: Navigate to api.NASA.gov.
- Register for an API Key: Complete the simple registration form.
- Receive Your API Key: Your unique API key will be sent to your email.
While NASA allows exploration with a DEMO_KEY (limited to 30 requests per hour and 50 requests per day per IP), obtaining a personal API key provides much higher rate limits.
Authentication Process#
Understanding how to secure your API is crucial when working with NASA data. The NASA API generally offers two authentication methods:
- API Key Authentication: Append your API key as a parameter in your API requests:
https://api.nasa.gov/planetary/apod?api_key=YOUR_API_KEY
- OAuth2 Authentication: For Earthdata Login, requiring application registration and token acquisition.
To understand the differences and best use cases, it's useful to compare API key vs JWT authentication methods.
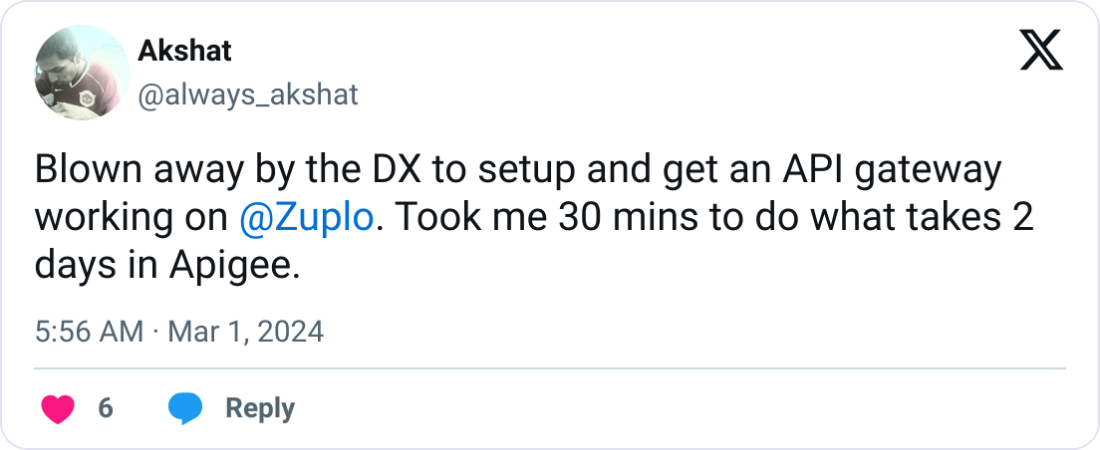
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreSetting Up the Development Environment#
- Choose Your Programming Language: The NASA API works with any language that can make HTTP requests.
- Set Up an API Sandbox: Create a testing environment for your API integrations.
- Prepare Your HTTP Client: Select the appropriate HTTP client library for your language.
- Implement Error Handling: Build robust error handling into your code.
- Consider Rate Limiting: Design your application to respect NASA's rate limits.
Working with the NASA API: Practical Examples#
Code Examples for Key NASA APIs#
Astronomy Picture of the Day (APOD)#
HTML structure:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>NASA APOD Viewer</title>
</head>
<body>
<h1>NASA Picture of the Day</h1>
<img id="image" src="" alt="NASA Picture of the Day" />
<p id="description"></p>
<script src="app.js"></script>
</body>
</html>
JavaScript implementation:
function displayData(data) {
document.getElementById("image").src = data.url;
document.getElementById("description").innerText = data.explanation;
}
fetch("https://api.nasa.gov/planetary/apod?api_key=DEMO_KEY")
.then((response) => response.json())
.then((data) => displayData(data));
Mars Rover Photos API#
const apiKey = "YOUR_API_KEY";
const apiUrl = `https://api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos?sol=1000&camera=fhaz&api_key=${apiKey}`;
fetch(apiUrl)
.then((response) => response.json())
.then((data) => console.log(data.photos))
.catch((error) => console.error("Error:", error));
API Response Processing#
The NASA API returns data in JSON format, which is easy to work with in JavaScript. Here's a sample APOD response:
{
"date": "1995-06-16",
"explanation": "Today's Picture: If the Earth could somehow be transformed to the ultra-high density of a neutron star...",
"hdurl": "https://apod.nasa.gov/apod/image/e_lens.gif",
"media_type": "image",
"service_version": "v1",
"title": "Neutron Star Earth",
"url": "https://apod.nasa.gov/apod/image/e_lens.gif"
}
To create engaging visualizations with this data, you can:
- Display images in galleries or slideshows
- Create interactive maps for Earth or Mars data
- Build data visualizations for asteroid tracking
- Generate timelines of space events or missions
For more detailed guidance, visit How to Access NASA Open APIs.
Advanced Usage Patterns and Optimization#
Rate Limiting, Caching, and Asynchronous Requests#
NASA enforces these rate limits:
- Default Rate Limit: 1,000 requests per hour for registered API keys
- DEMO_KEY Limits: 30 requests per hour and 50 requests per day per IP
Response headers show your current usage:
X-RateLimit-Limit: 1000
X-RateLimit-Remaining: 998
Understanding API rate limiting is crucial to ensure compliance and optimize your application's performance. Implement caching to reduce API calls:
- Store frequently accessed data in localStorage or server-side caches
- Use service workers to intercept and cache network requests
Implementing monitoring tools can help you ensure API performance and identify bottlenecks.
Use asynchronous requests to parallelize API calls:
async function fetchMultipleEndpoints() {
try {
const [apodData, marsData, neoData] = await Promise.all([
fetch("https://api.nasa.gov/planetary/apod?api_key=YOUR_API_KEY"),
fetch(
"https://api.nasa.gov/mars-photos/api/v1/rovers/curiosity/photos?sol=1000&api_key=YOUR_API_KEY",
),
fetch("https://api.nasa.gov/neo/rest/v1/feed?api_key=YOUR_API_KEY"),
]);
// Process results after all requests complete
const apodJson = await apodData.json();
const marsJson = await marsData.json();
const neoJson = await neoData.json();
return { apod: apodJson, mars: marsJson, neo: neoJson };
} catch (error) {
console.error("Error fetching data:", error);
}
}
Implementing Caching to Improve Performance & Minimize Calls#
Here's a quick tutorial on how to implement caching with Zuplo to minimize API calls and improve your performance:
Optimization Techniques#
Throttling Requests:
async function throttledRequests(urls, delay = 1000) {
const results = [];
for (const url of urls) {
const response = await fetch(url);
const data = await response.json();
results.push(data);
await new Promise((resolve) => setTimeout(resolve, delay));
}
return results;
}
Lazy Loading Images:
const observer = new IntersectionObserver((entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
const lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
observer.unobserve(lazyImage);
}
});
});
document.querySelectorAll(".lazy-image").forEach((img) => {
observer.observe(img);
});
For more advanced API rate limiting strategies, consider implementing dynamic limits based on user behavior. If you're developing your own APIs, knowing how to rate limit APIs is essential for maintaining performance and security.
Common Use Cases and Project Ideas#
Potential Applications#
- Space Weather App: Monitor solar activity and its potential impact on Earth. In 2012, Delta Air Lines redirected flights due to a solar radiation storm.
- Asteroid Tracker: Visualize near-Earth objects and their trajectories using the Near Earth Object Web Service.
- Mars Weather Station: Display current Martian weather conditions from NASA's rovers.
- Earth Change Visualizer: Create time-lapse visualizations showing environmental changes over time.
- Space Trivia Game: Develop educational games using NASA data.
By integrating multiple NASA datasets into an API integration platform, you can develop advanced applications that provide comprehensive insights.
Real-Life Applications#
The practical applications extend beyond theory. In 2022, a geomagnetic storm caused by solar activity rendered at least 40 SpaceX's Starlink satellites inoperable. Applications using NASA's Solar Flare and Geomagnetic Storm APIs could help satellite operators predict such events.
Another use case involves monitoring space radiation for aviation safety. The Flight Safety Foundation has documented potential health risks to flight crews from increased radiation during solar events.
NASA API Troubleshooting and Best Practices#
Common Issues and Solutions#
- Rate Limiting Exceeded: Implement caching for frequently accessed data.
- Overwhelming Data Volume: Filter requests to include only necessary data.
- Data Latency Issues: Check documentation for update frequencies.
- Complex Data Interpretation: Consult NASA's documentation or collaborate with domain experts.
Error Handling Best Practices#
try {
fetch(apiUrl)
.then((response) => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.json();
})
.then((data) => console.log(data))
.catch((error) => console.error("Fetch error:", error));
} catch (error) {
console.error("General Error:", error);
}
Additional best practices:
- Always validate data received from the API
- Use asynchronous programming techniques
- Implement appropriate timeout handling
- Log errors comprehensively
Keeping Apps Updated#
- Schedule periodic reviews of NASA API documentation
- Check version information in API responses
- Maintain a testing environment for compatibility verification
- Subscribe to NASA's developer channels for announcements
- Implement feature flags to manage functionality affected by API changes
Future-Proofing Your Application#
To ensure compatibility as the NASA API evolves:
- Regularly check NASA's API documentation for updates
- Build modular code that can easily incorporate new data sources
- Implement version checking in your API calls
- Create abstraction layers separating business logic from API implementation
- Use feature detection to handle missing endpoints gracefully
Unlock the Universe of Data with the NASA API#
The NASA API opens the door to an incredible world of space data—15 terabytes collected daily, from awe-inspiring astronomy images to essential near-Earth object tracking. This wealth of information is now available for developers, researchers, and space enthusiasts to leverage in creating applications that educate, inspire, and push the boundaries of innovation.
By simply signing up for a free API key from api.nasa.gov, you can start building your own space-themed projects, whether it's tracking celestial bodies, monitoring Earth’s environmental changes, or crafting immersive visualizations. The possibilities are endless.
Harnessing vast datasets doesn’t have to be daunting—it’s an opportunity to explore and create. Start your cosmic coding journey today, and if you’re looking to streamline your API development and management process, explore Zuplo’s powerful tools designed to optimize your workflows, making it easier to bring your ideas to life. 🧑🚀 🚀