Profiling API endpoints helps you measure and improve performance by analyzing key metrics like response times, request volumes, and error rates. This ensures faster, more reliable APIs and better resource usage. Here's a quick summary of how to get started:
-
Key Metrics to Monitor:
- Response Times: Time to First Byte (TTFB), total response time, and processing time.
- Request Volume: Requests per second, peak usage times, and concurrent requests.
- Error Tracking: Error rate, error types (4xx/5xx), and recurring error patterns.
-
Tools and Techniques:
- Use monitoring software for real-time analytics.
- Implement request tracing to identify bottlenecks.
- Conduct load, stress, and endurance tests to evaluate system capacity.
-
4-Step Process:
- Define performance goals.
- Set up measurement tools like API gateways and monitoring systems.
- Analyze baseline and peak performance data.
- Optimize and test changes with load testing.
-
Continuous Monitoring:
- Set alerts for response time thresholds, error spikes, and unusual traffic patterns.
- Track resource usage and integrate performance checks into your CI/CD pipeline.
Profiling ensures your APIs run efficiently, meet user expectations, and adhere to SLAs.
Core Performance Metrics#
Tracking the right metrics is key to understanding and improving your API's performance. Here are some critical measurements to keep an eye on.
Response Times#
Response time has a direct effect on user experience and application efficiency. It measures how quickly your API processes and responds to requests. Key metrics to monitor include:
- Time to First Byte (TTFB): The time between sending a request and receiving the first byte of the response.
- Total Response Time: The full round-trip time from when the request is sent to when the response is complete.
- Processing Time: The duration spent executing the endpoint's business logic.
With API monitoring tools (covered later), developers can quickly identify slow endpoints and make improvements to boost response times. Next, let’s look at how tracking request volume can provide insights into usage patterns.
Request Volume#
Monitoring request volume helps you understand usage trends and plan for capacity. Here’s what to measure:
Metric | Description | Why It Matters |
---|---|---|
Requests per Second | Number of API calls received per second | Helps determine scaling needs |
Peak Usage Times | Times with the highest request volume | Aids in predicting resource demands |
Concurrent Requests | Number of simultaneous API calls | Highlights potential bottlenecks |
Error Tracking#
Keeping an eye on errors ensures your API remains reliable and doesn’t frustrate users. Key error metrics include:
- Error Rate: The percentage of failed requests compared to total requests.
- Error Types: Categories of errors, such as 4xx (client-side) and 5xx (server-side).
- Error Patterns: Repeated issues that could signal deeper problems.
Profiling Tools and Methods#
Using the right tools and methods is key to analyzing API performance effectively. Below, we'll break down some essential techniques to help teams collect actionable performance data.
Request Tracing#
While monitoring gives an overview, request tracing dives into the specifics of API behavior. This technique is great for identifying:
- Bottlenecks across services
- Latency between components
- Resource usage trends
- Error propagation paths
Key elements for effective request tracing include:
Component | Purpose | Key Benefits |
---|---|---|
Trace IDs | Assigns a unique ID to each request | Tracks the full request lifecycle |
Span Collection | Captures timing data for services | Highlights slow-performing components |
Context Propagation | Maintains request context across systems | Links distributed operations seamlessly |
Once you map out request flows, load testing can reveal how the system handles high traffic. A good standard to adopt for tracing is Open Telemetry.
Load Testing#
Load testing evaluates API performance under various traffic levels. A strong load testing plan includes:
1. Baseline Testing: Establish normal performance benchmarks before ramping up traffic.
2. Stress Testing: Gradually increase the load to find the system's maximum capacity and identify breaking points.
3. Endurance Testing: Run extended tests to uncover memory leaks, excessive resource use, and potential performance degradation over time.
Popular API load testing tools include k6 from Grafana and Artillery. Both are open source and both also offer a managed cloud offering to make management easier.
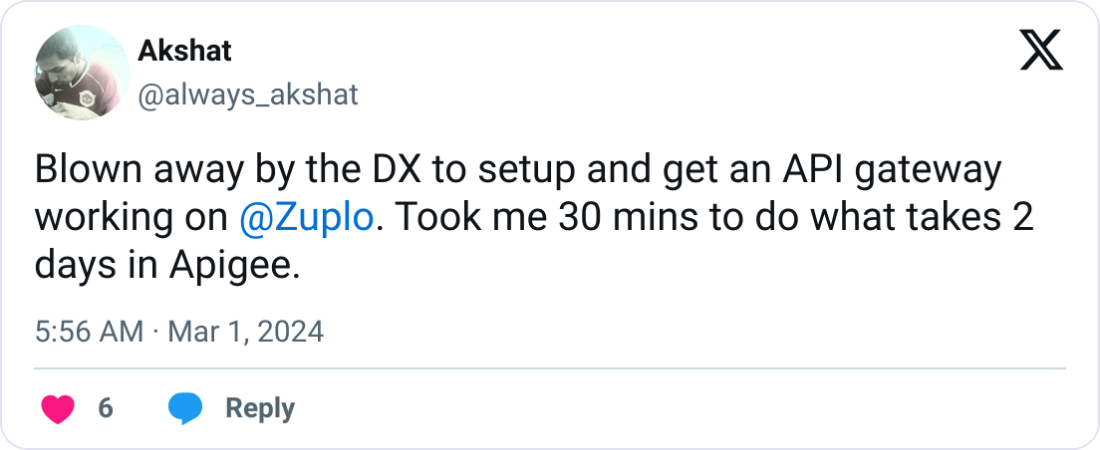
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn More4-Step Performance Analysis#
Follow this four-step process to pinpoint and fix API performance issues.
1. Set Performance Goals#
Start by defining clear, measurable performance targets. Focus on these key metrics:
Metric Type | Example Target | Measurement Frequency |
---|---|---|
Response Time | < 200ms at p95 | Continuous |
Error Rate | < 0.1% of requests | Hourly |
Throughput | 1,000 requests/second | Daily |
Availability | 99.9% uptime | Monthly |
Make sure your targets align with both your system's technical capabilities and your business needs. Tools like rate limiting and request quotas can help maintain steady performance over time.
2. Set Up Measurement Tools#
Use your API gateway and monitoring tools to track critical metrics effectively:
- API Gateways
API gateways (ex. Zuplo, Apigee) often include basic usage analytics within their web portals. This includes reports on metrics like the ones mentioned before, but also gateway-specific metrics. Gateway specific metrics typically focus on functionality implemented in your gateway, including auth (ex. number of calls per API key/JWT token/userId) and rate limiting (ex. Which users got rate limited). - Monitoring Infrastructure
Set up API monitoring tools to capture request/response cycles, resource usage, error patterns, and unusual performance behaviors. Check out our monitoring tool recommendation list - which includes both API-specific monitoring tools (ex. Moesif) as well as generic monitoring tools that you might already be using elsewhere in your stack (ex. DataDog).
Once everything is configured, begin gathering data for analysis.
3. Measure and Compare#
With your monitoring tools in place, start collecting and analyzing performance data. Pay attention to:
- Baseline Performance: Understand normal operating conditions.
- Peak Usage Patterns: Spot high-traffic periods.
- Error Correlations: Examine how traffic spikes relate to error rates.
- Response Time Variations: Track performance across various endpoints.
Your developer portal's analytics can help you monitor these metrics and uncover trends that guide your next steps.
4. Implement and Test Changes#
Fix issues by optimizing code, scaling resources, or adjusting configurations. Use load testing to confirm improvements.
Ongoing Performance Monitoring#
Alert Systems#
Set up alerts to catch potential issues before they impact users. Focus on:
- Response Time Thresholds: Get notified when latency exceeds acceptable limits.
- Error Rate Spikes: Monitor for sudden increases in API errors.
- Resource Utilization: Keep an eye on CPU, memory, and bandwidth usage.
- Traffic Anomalies: Detect unusual patterns in request volumes.
Level | Trigger Conditions | Response Time |
---|---|---|
Critical | Error rate > 5% or p95 latency > 1000ms | Immediate notification |
Warning | Error rate > 1% or p95 latency > 500ms | Within 15 minutes |
Info | Traffic spike > 200% baseline | Daily digest |
Pair these alerts with in-depth metric tracking for better insights.
Performance Metrics Breakdown#
Response Time Analysis
- Monitor response times for each endpoint and HTTP method.
- Evaluate performance during high-traffic periods.
Resource Usage Patterns
- Correlate traffic trends with resource consumption.
- Spot memory leaks and CPU bottlenecks.
- Keep tabs on cache hit rates and database efficiency.
Leverage these metrics to enhance automated CI/CD performance testing.
CI/CD Performance Checks#
Incorporate performance monitoring into your CI/CD pipeline to avoid regressions.
Automated Performance Tests
Run load, stress, and endurance tests with every deployment to ensure:
- p95 latency stays under 200ms at double the normal load.
- The system can handle five times the load weekly without errors.
- Stability is maintained over a 24-hour period during endurance tests.
Gateway Configuration Validation
- Confirm rate limiting settings are correct.
- Ensure API specifications align with expectations.
- Test the performance of custom middleware.
Use OpenAPI specs to keep API documentation and gateway configurations in sync.
Test Type | Frequency | Success Criteria |
---|---|---|
Load Test | Every deployment | p95 < 200ms under 2x normal load |
Stress Test | Weekly | Handle 5x normal load without errors |
Endurance Test | Monthly | Stable performance over 24 hours |
Conclusion#
API profiling combines monitoring tools, clear metrics, and automated testing to boost reliability and performance.
Key practices for effective API profiling include:
- Setting clear performance baselines and thresholds
- Using automated monitoring and alert systems
- Adding performance checks to CI/CD pipelines
- Regularly analyzing metrics and making improvements
Tools like Zuplo make this process easy by integrating these elements into one platform. In addition to native monitoring and analytics built into Zuplo's API gateway, you can easily integrate with logging providers to get a complete picture of your API's performance. Sign up for a free Zuplo account today and get a better picture of your API performance today!