Slow APIs are silent conversion killers. When your API lags, users bounce, revenue drops, and your infrastructure costs soar. Whether you're building microservices, mobile apps, or web platforms, every millisecond matters in today's performance-obsessed landscape.
Even elegantly designed APIs face challenges that can frustrate users and limit growth. A/B testing offers a methodical, data-driven approach to API optimization that eliminates guesswork. When Statsig optimized their async request handling, they slashed response times by 4.9% while reducing CPU usage by 1.9%. That's real business impact — something you can’t afford to skimp on. Let's look at how you can implement effective A/B testing strategies to transform your API performance and delight your users.
- Mastering the Metrics That Matter: Your Performance Dashboard
- Building Your A/B Testing Engine: Infrastructure Essentials
- Crafting Tests That Win: Strategic Experiment Design
- Performance Boosters: Optimization Strategies Worth Testing
- Beyond The Numbers: Interpreting Results That Matter
- Beyond One-Off Tests: Building a Performance Culture
- Beyond Basics: Advanced API Testing Techniques
- From Tests to Transformation: Your API Performance Roadmap
- Turbocharging API Performance
Mastering the Metrics That Matter: Your Performance Dashboard#
Before optimizing anything, you need to know what to measure. Utilizing API monitoring tools can help focus on these high-impact indicators:
Response Time and Latency#
The cornerstone of API performance is response speed. Look beyond averages to percentiles that reveal the full user experience:
- p50 (median): What typical users experience
- p95: What 95% of your users experience
- p99: The slowest acceptable responses
A p95 of 300ms means 95% of requests finish in under 300ms—helping you identify performance issues affecting your most vulnerable users.
Throughput#
Measured in requests per second (RPS), throughput reveals your API's capacity under various conditions. This metric helps you understand:
- Maximum capacity limits
- Performance at different traffic levels
- Scaling capabilities
The holy grail is maintaining consistent response times even as throughput increases.
Error Rates and Types#
Tracking errors by category illuminates reliability issues:
- 4xx errors: Client problems like invalid parameters
- 5xx errors: Server issues in your infrastructure
- Timeout errors: Requests that exceed time limits
Categorization helps pinpoint whether problems originate from clients, servers, or infrastructure components.
Resource Utilization Metrics#
These metrics expose how efficiently your API uses infrastructure:
- CPU usage: High consumption may indicate inefficient code
- Memory consumption: Reveals potential leaks or poor data handling
- Network I/O: Shows data transfer bottlenecks
- Disk I/O: Critical for storage-intensive APIs
Cache Hit Ratio#
For APIs using caching, this metric shows the percentage of requests served from cache:
- 80%+ typically indicates effective caching
- Low ratios highlight optimization opportunities
Establishing Meaningful Baselines#
Effective measurement requires solid baseline data:
- Gather metrics during both peak and normal usage
- Segment by endpoint, user type, and location
- Account for traffic patterns (daily, seasonal)
- Document baselines with clear definitions
Different APIs prioritize different metrics—data-heavy APIs may focus on throughput and caching, while computational APIs emphasize CPU use and p99 latency. By targeting the metrics that matter most for your specific use case, you'll focus optimization efforts where they'll have maximum impact.
Building Your A/B Testing Engine: Infrastructure Essentials#
Creating reliable API tests requires purpose-built infrastructure. When A/B testing backend configurations, the foundation is directing users to different API variants. Here's how to construct a framework that delivers consistent, meaningful results.
Traffic Splitting Mechanisms#
The foundation of any A/B test is directing users to different API variants. You have two primary approaches:
Gateway-Level Splitting
This handles traffic distribution before requests reach your application:
// Example using an API Gateway with AWS Lambda
const AWS = require("aws-sdk");
const apiGateway = new AWS.APIGateway();
// Create a canary deployment with traffic split
const params = {
restApiId: "your-api-id",
stageName: "prod",
patchOperations: [
{
op: "replace",
path: "/canarySettings/percentTraffic",
value: "20",
},
],
};
apiGateway
.updateStage(params)
.promise()
.then((data) => console.log("Canary deployment updated"))
.catch((err) => console.error("Error updating canary deployment", err));
Benefits include no code changes and easy scaling. Implementing smart routing for APIs helps in managing traffic at the gateway level.
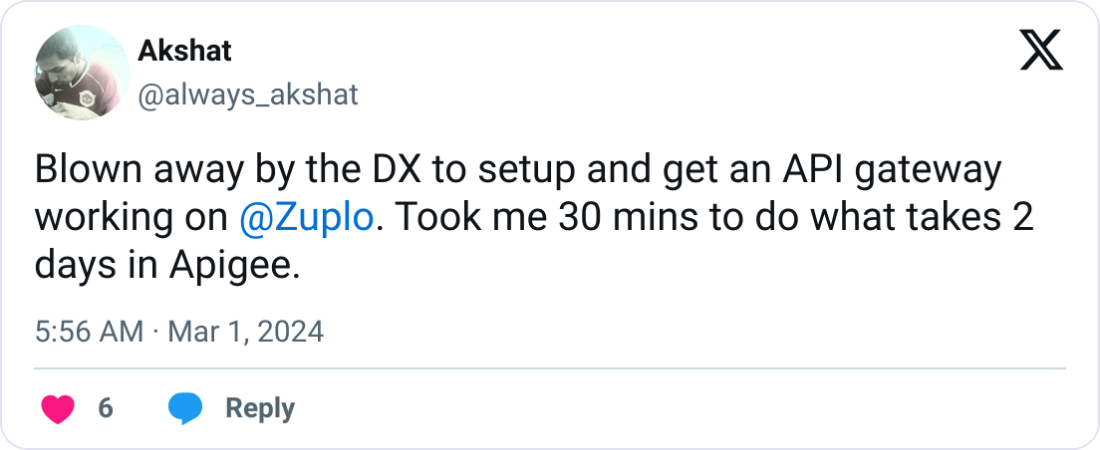
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreApplication-Level Splitting
For more granular control, split traffic within your application:
# Example using a simple request-based approach
import random
def route_request(request):
# Assign user to variant based on identifier or random assignment
user_id = request.headers.get('user-id', '')
test_group = hash(user_id) % 100
if test_group < 50: # 50% of traffic
return call_api_version_a(request)
else:
return call_api_version_b(request)
Feature Flags for Controlled Experiments#
Feature flags give you fine-grained control over API behavior:
// Example using a feature flag service
public Response processApiRequest(Request request) {
String userId = request.getUserId();
// Check if user should see the new API behavior
if (featureFlagService.isEnabled("new-api-algorithm", userId)) {
return newApiImplementation(request);
} else {
return standardApiImplementation(request);
}
}
Many teams use dedicated services like LaunchDarkly or Split.io, though you can build your own solution.
Metric Collection Systems#
Accurate performance measurement relies on comprehensive telemetry:
// Example middleware for Express.js API to track response times
app.use((req, res, next) => {
const start = Date.now();
// Add listener for when response finishes
res.on("finish", () => {
const duration = Date.now() - start;
// Record metrics with variant information
metrics.recordLatency({
endpoint: req.path,
method: req.method,
statusCode: res.statusCode,
durationMs: duration,
variant: req.headers["x-test-variant"] || "control",
});
});
next();
});
Essential metrics include response time distributions, error rates (including how to handle rate limit errors), and resource utilization.
Statistical Analysis Tools#
Ensure test validity with proper statistical methods:
# Example code for analyzing API test results
import scipy.stats as stats
def analyze_test_results(control_data, test_data, confidence_level=0.95):
# Run t-test to compare means
t_stat, p_value = stats.ttest_ind(control_data, test_data)
# Calculate confidence interval
margin_error = stats.sem(test_data) * stats.t.ppf((1 + confidence_level) / 2, len(test_data) - 1)
mean_difference = np.mean(test_data) - np.mean(control_data)
return {
'is_significant': p_value < (1 - confidence_level),
'p_value': p_value,
'mean_difference': mean_difference,
'confidence_interval': (mean_difference - margin_error, mean_difference + margin_error)
}
For statistically valid results:
- Run A/A tests to verify your infrastructure
- Calculate required sample sizes before testing
- Consider Bayesian methods for dynamic traffic allocation
This infrastructure creates a solid foundation for reliable, insightful A/B testing on your API endpoints.
Crafting Tests That Win: Strategic Experiment Design#
Random testing yields random results. Strategic API testing starts with laser-focused hypotheses tied to business outcomes. Instead of vague goals like "make the API faster," create specific, measurable hypotheses: "Implementing gzip compression will reduce response size by 65% and cut latency by 20% without increasing CPU load."
Determining proper sample size is non-negotiable. Using statistical power calculators ensures you can detect meaningful differences without false positives. High-traffic APIs need fewer testing hours, while low-traffic endpoints require longer periods. Skip this step at your peril—underpowered tests produce misleading results that lead to costly mistakes.
Control external variables by isolating tests through:
- Time period segmentation to account for usage patterns
- User cohort consistency to prevent demographic skew
- Geography-based testing to neutralize network effects
Minimize risk during testing with these safeguards:
- Start with small traffic percentages (5-10%)
- Implement circuit breakers that auto-revert if error rates spike
- Conduct A/A tests first to validate your measurement framework
This methodical approach has helped companies cut API response times by up to 30% while maintaining system stability throughout testing—delivering significant performance improvements that translate directly to improved user satisfaction and business metrics.
Performance Boosters: Optimization Strategies Worth Testing#
Systematic testing of these optimization strategies can help you increase API performance and deliver remarkable performance gains across your API architecture.
Response Payload Optimization#
How you structure API responses dramatically affects transmission speed:
- Compression Algorithms: Using gzip or Brotli can reduce bandwidth and significantly lower latency.
- Field Filtering: Allow clients to request only needed fields with
parameters like
?fields=id,name,email
to avoid over-fetching. - Protocol Selection: Test different API architectures:
- REST: Simple and widely supported, but sometimes returns excess data
- GraphQL: Enables precise field selection to minimize payloads
- gRPC: Uses binary Protocol Buffers for highly efficient data transfer
Before Optimization:
{
"user": {
"id": 12345,
"name": "Jane Smith",
"email": "jane@example.com",
"address": {
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "94043",
"country": "USA"
},
"phone": "555-123-4567",
"favorites": [1, 7, 23, 42],
"lastLogin": "2023-04-15T08:30:45Z",
"accountCreated": "2022-01-10T15:20:30Z",
"preferences": {
"theme": "dark",
"notifications": true,
"language": "en-US"
}
}
}
Size: ~362 bytes
After Optimization (with field filtering):
{
"user": {
"id": 12345,
"name": "Jane Smith",
"email": "jane@example.com"
}
}
Size: ~77 bytes (78% reduction)
Caching Strategies#
Effective caching dramatically reduces database load and accelerates responses:
- Client-Side Caching: Use HTTP headers like
ETag
andCache-Control
to enable local storage and validation. - Server-Side Caching: Implement memory caching with Redis or Memcached to avoid redundant database queries.
- Cache Invalidation Techniques: Test approaches like time-based expiration, event-driven invalidation, and versioned caching.
- Distributed Caching: For high-traffic APIs, implement multi-level caching with CDN edge distribution.
Database Query Optimization#
Databases often become API performance bottlenecks:
- Query Rewriting: Restructure complex queries and leverage database-specific optimizations.
- Index Optimization: Add strategic indexes on frequently queried columns. Proper indexing can improve query performance by orders of magnitude.
- Connection Pooling: Maintain ready database connections to eliminate connection setup overhead.
- Data Sharding: For large datasets, test horizontal partitioning to distribute query load.
Load Balancing and Scaling Patterns#
As traffic grows, test different scaling approaches:
- Horizontal vs. Vertical Scaling: Compare adding servers against upgrading existing ones for cost-efficiency.
- Load Shedding and Rate Limiting: Implement graceful degradation during traffic spikes by prioritizing critical requests and consider API rate limiting best practices to prevent resource exhaustion.
- Traffic Mirroring: Use VPC traffic mirroring to test optimizations against real production traffic without affecting users.
Testing these strategies systematically helps identify which approaches deliver the biggest performance improvements for your specific API usage patterns. Focus on one optimization at a time to clearly link performance gains to specific changes.
Beyond The Numbers: Interpreting Results That Matter#
Data without interpretation is just noise. Here's how to translate test results into meaningful insights and actions.
Statistical Methods That Reveal Truth#
Proper analysis starts with solid statistical approaches:
- P-values and confidence intervals: A p-value under 0.05 suggests your results aren't random chance. But remember, this doesn't mean there's a 95% chance your results are correct—it means there's a 5% risk that random chance produced what you're seeing, as explained by conversion experts.
- Statistical power: Aim for at least 80% power to reliably detect real differences when they exist, which requires adequate sample sizes.
- Frequentist vs. Bayesian methods: Traditional approaches evaluate significance only after tests finish. Bayesian methods continuously update the probability of an effect, potentially providing faster insights.
Avoiding Interpretation Pitfalls#
Watch for these common mistakes:
- Insufficient sample size: Low-powered tests often exaggerate effect sizes, making improvements look better than they are.
- Multiple testing problem: Examining too many metrics simultaneously increases false positive risks. Consider correction methods when evaluating multiple outcomes.
- Premature peeking: Checking results before reaching predetermined sample sizes leads to false positives or exaggerated effects.
- Ignoring confidence intervals: A test showing a 5% improvement with a ±4% confidence interval may not be reliable at scale.
- Sample Ratio Mismatch (SRM): This occurs when traffic isn't split as intended. Run A/A tests first to catch sampling issues before real experiments.
Connecting Technical Metrics to Business Impact#
Technical improvements only matter if they drive business value:
- Link performance metrics to user engagement and conversion metrics
- Build funnels that connect API performance to business outcomes
- Segment results by user demographics, devices, and traffic sources
In one real case, an e-commerce platform optimized search queries with composite indexes, making them 70% faster and increasing search-to-purchase conversion by 15% during peak hours.
Making Data-Backed Implementation Decisions#
When deciding whether to implement changes:
- Balance statistical vs. practical significance: A statistically significant 2% latency improvement might not justify complex changes requiring substantial engineering effort.
- Weigh implementation costs against benefits: Consider engineering time, maintenance overhead, and potential risks against expected business impact.
- Roll out gradually: Start with a small percentage of traffic and increase exposure while monitoring both technical and business metrics.
A comprehensive analysis approach helps you make confident, data-driven decisions that improve both technical performance and business results while avoiding interpretation pitfalls.
Beyond One-Off Tests: Building a Performance Culture#
One-time optimizations fade. True performance excellence requires embedding A/B testing into your development DNA. Integrate performance testing directly into CI/CD pipelines with automated performance gates that must pass before deployment, just like unit tests but focused on latency and throughput.
Create visible feedback loops through dashboards highlighting performance metrics from both production and test environments. Nurture a performance-minded engineering culture by:
- Establishing clear performance SLAs for all API endpoints
- Conducting regular performance reviews alongside feature planning
- Celebrating wins when optimizations deliver measurable improvements
Leverage tools like Apache JMeter for load testing, Postman for functional validation, Grafana and Prometheus for metrics visualization, or AWS VPC Traffic Mirroring to safely replicate production traffic.
Your CI/CD workflow should include dedicated performance environments where A/B tests run automatically after functional tests pass but before production deployment, with clear results visualization and automated regression alerts.
Beyond Basics: Advanced API Testing Techniques#
Whether you're conducting end-to-end API testing or implementing advanced techniques, these methods can help optimize complex API systems.
Multi-variant Testing for Complex Systems#
While A/B testing compares two versions, multi-variant testing evaluates several API configurations simultaneously. This works well for complex systems with interdependent components.
You might test combinations of:
- Different caching strategies
- Various database query optimizations
- Multiple compression techniques
Ensure you have sufficient traffic for statistical significance across all test groups—each variant needs adequate data for reliable results.
Canary Releases for Safer Deployment#
Canary releases gradually roll out changes to a small user subset before wider deployment, minimizing risk while providing real-world performance data.
Implementation steps:
- Start small (1-5% of traffic)
- Monitor key metrics closely
- Gradually increase exposure if performance is positive
- Maintain quick rollback capabilities
Microsoft's experiments with reverse proxy configurations demonstrated the importance of robust telemetry and rapid rollbacks when testing networking changes.
Machine Learning for Optimization Discovery#
ML can identify patterns and optimization opportunities that traditional testing might miss:
- Anomaly detection: ML algorithms spot performance irregularities in real-time
- Traffic prediction: Models forecast API load patterns for preemptive scaling
- Parameter tuning: ML tests thousands of configuration combinations
A European performance marketing client used real-time API optimizations with ML-based calculations, increasing margins by 35% through continuous optimization.
Predictive Performance Modeling#
Instead of waiting for actual issues, predictive modeling simulates API behavior under various conditions:
- Build mathematical models of your API system
- Forecast performance under different load scenarios
- Test hypothetical infrastructure changes before implementation
- Identify potential bottlenecks proactively
Choosing the Right Testing Approach#
Not all APIs need the same testing approach. Consider these factors:
- Traffic volume: High-traffic APIs can use short tests with small traffic percentages; low-traffic APIs need longer testing periods.
- Risk tolerance: For critical systems, use lower-risk approaches like VPC Traffic Mirroring, which copies production traffic to test environments without affecting users.
- Performance goals: Define clear metrics like maximum latency or throughput targets to guide your testing.
- Available resources: Some approaches require significant infrastructure or expertise. Match your method to your capabilities.
These advanced techniques take you beyond basic performance testing to create truly optimized API systems that scale effectively and deliver exceptional user experiences.
From Tests to Transformation: Your API Performance Roadmap#
API performance optimization isn't optional—it's a business-critical discipline that demands systematic testing and continuous refinement. The most successful strategies target high-impact areas like payload optimization, caching, and query enhancement with methodical testing.
To implement these strategies effectively:
- Establish clear baselines: Know your current response times, error rates, and latency distribution before making changes.
- Focus on high-impact areas: Target optimizations that directly affect user experience and business metrics.
- Validate with data: Use A/B testing to confirm each change before full deployment.
Ready to launch your first API A/B test? Use this checklist:
- Define specific success metrics (response time, error rate, resource usage)
- Set up proper test and control groups with randomized assignment
- Calculate appropriate sample size for statistical validity
- Implement comprehensive monitoring for all performance metrics
- Complete tests fully before drawing conclusions
- Analyze results with confidence intervals, not just averages
Turbocharging API Performance#
API performance optimization isn't just a technical checkbox—it's a business-critical discipline that directly impacts user satisfaction and your bottom line. The most successful strategies target high-impact areas like payload optimization, caching, and query enhancement through methodical testing and continuous refinement.
Ready to unlock your API's full potential? Sign up for a free Zuplo account today and start implementing these powerful optimization techniques with our developer-friendly platform. Whether you're managing legacy systems or building cutting-edge services, Zuplo provides the tools you need to measure, test, and transform your API performance into a true competitive advantage.