Ever relied on an API that suddenly went MIA? It happens to the best of us. In today's interconnected world, API timeouts are like surprise thunderstorms—they will happen, and they'll test your application's resilience when they do.
When timeout errors crash the party, they can trigger a domino effect that makes your entire system collapse faster than a house of cards. Without proper timeout simulation during testing, your production environment becomes your testing ground—with real users as unwitting guinea pigs. By simulating API timeouts for stress testing with mock APIs, you'll identify weaknesses before they become user-facing disasters and build applications that gracefully navigate the inevitable turbulence of distributed systems.
So, how do you prepare your application for these timeout scenarios? Let's dive into the world of API timeout simulation and discover how to build applications that bend but don't break when faced with the harsh realities of network communication.
- When Good APIs Go Bad: Understanding Timeout Behavior Patterns
- Building Your Timeout Testing Playground: Mock API Setup
- Timeout Testing Tactics: Four Killer Strategies That Reveal Weak Points
- Beyond Simulation: Building Resilient Systems That Withstand Real-World Timeouts
- From Simulation to Survival: Building APIs That Withstand the Storm
When Good APIs Go Bad: Understanding Timeout Behavior Patterns#
APIs don't just fail in boring, predictable ways—they get creative with their meltdowns. Understanding these failure patterns is essential for building truly resilient applications.
Types of API Failures#
- Hard Timeouts: These occur when a service stops waiting for a response after a predetermined time. They prevent resource blocking but can trigger cascading failures throughout your system.
- Slow Responses: The silent killers of performance. These responses don't trigger timeout thresholds but creep along with high latency, quietly wasting resources as services hold connections open while waiting.
- Intermittent Failures: The most frustrating kind—working sometimes and failing unpredictably at others. These erratic failures create debugging nightmares that'll have your team questioning their career choices.
Cascading Failures in Microservice Architectures#
In microservice architectures, timeout failures are particularly dangerous because they can cascade:
- An initial timeout in a downstream service causes upstream services to fail.
- These failures propagate through the system as more services become unresponsive.
- Eventually, this can lead to system-wide outages.
DoorDash has observed that a single service experiencing high latency can trigger a "death spiral" where more traffic routes to remaining healthy nodes, overwhelming them and causing complete system collapse.
The business impact isn't pretty—these failures lead to lost revenue, damaged reputation, and increased operational costs as teams scramble to restore service. To prevent such outcomes, employing strategies like smart routing for microservices can be crucial.
Timeout Patterns That Will Haunt Your Systems#
Let's cut through the confusion around different timeout types:
Connection vs. Read Timeouts:
- Connection Timeouts: The maximum time allowed to establish a connection to the server.
- Read Timeouts: The maximum time allowed to wait for data after a connection is established.
Socket vs. Request Timeouts:
- Socket Timeouts: Low-level network timeouts at the TCP layer.
- Request Timeouts: Higher-level timeouts for the entire HTTP request/response cycle.
According to KrakenD, many developers make the rookie mistake of using a single timeout value rather than configuring these different types appropriately.
Retry Mechanisms and Backoff Strategies#
When timeouts occur, retry mechanisms can help recover, but they must be implemented carefully.
- Simple Retries: Attempting the same request again immediately (about as dangerous as texting your ex at 2 AM).
- Exponential Backoff: Gradually increasing the delay between retry attempts.
- Jitter: Adding randomness to retry intervals to prevent synchronized retries.
API Park notes that poorly implemented retry logic can worsen outages by creating "retry storms" that further overwhelm already struggling services. Proper managing request limits is essential to prevent such issues. Additionally, implementing effective API rate limiting can help control traffic and reduce the likelihood of outages.
Key Metrics to Monitor#
During timeout scenarios, you'll want to keep your eyes on these metrics:
- Latency percentiles (p50, p95, p99) - to spot degradation before it causes timeouts.
- Error rates by service and endpoint.
- Timeout occurrence frequency.
- Resource utilization (CPU, memory, connections).
- Circuit breaker status - tracking when failure thresholds are reached.
Catchpoint recommends combining real-time monitoring with synthetic API checks to detect emerging timeout patterns before they impact users. Using effective API monitoring tools can help track these metrics and alert you to issues promptly.
Now that we understand how timeouts can wreak havoc on our systems, let's explore how to create controlled environments for testing these scenarios.
Building Your Timeout Testing Playground: Mock API Setup#
Testing with real production APIs is like playing with fire in a fireworks factory—unpredictable, expensive, and potentially disastrous. Mock APIs provide a controlled sandbox where you can deliberately create delays, simulate failures, and test your application's resilience without the production drama. With tools that support rapid API mocking, you can set up these environments quickly and efficiently.
Why Mock APIs Are Ideal for Timeout Testing#
Mock APIs aren't just handy—they're essential. They let you:
- Create specific timeout scenarios that rarely happen in production.
- Test extreme cases without waiting for them to occur naturally.
- Build repeatable test conditions for consistent results.
- Skip the costs of hammering third-party APIs.
- Test without needing external services to be available.
Understanding API Simulation Approaches#
Before you start coding, know your options:
- Stubbing: The simplest approach—just return predetermined responses for specific requests. Stubs are stateless and give you basic functionality for testing.
- Mocking: More advanced than stubbing, mocks can verify expected calls and have programmed behaviors about how they should be used.
- Service Virtualization: The full package—simulating the complete behavior of a service including states, protocols, and performance characteristics.
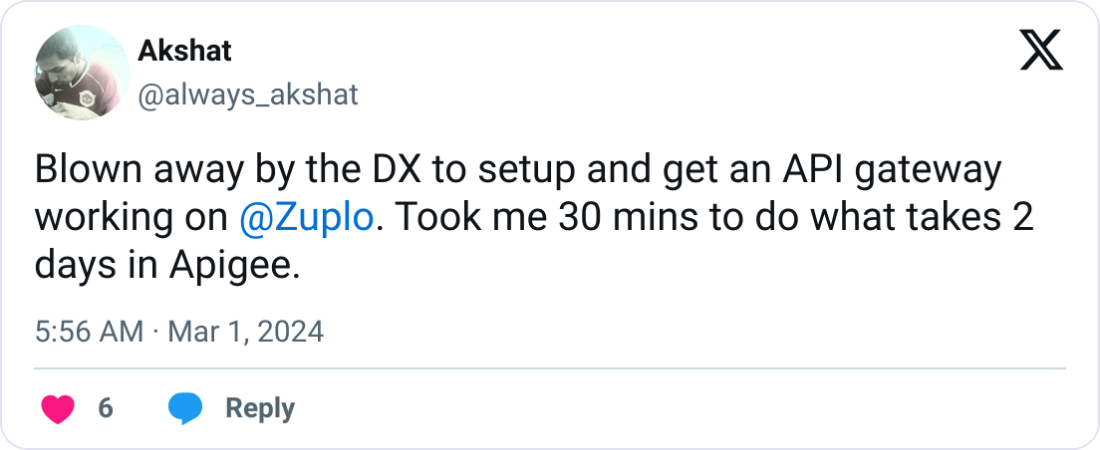
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreSetting Up a Basic Mock API Environment#
Here's how to set up a mock API in popular programming languages to simulate API timeouts for stress testing.
Node.js Example#
Using Express.js, you can create a simple mock API with configurable delays:
const express = require("express");
const app = express();
const port = 3000;
app.use(express.json());
// Route with configurable delay
app.get("/api/data", (req, res) => {
// Get delay from query parameter or use default
const delay = parseInt(req.query.delay) || 0;
setTimeout(() => {
res.json({ message: "This is mock data", timestamp: new Date() });
}, delay);
});
// Timeout simulation endpoint
app.get("/api/timeout", (req, res) => {
// This route will never respond, simulating a complete timeout
});
app.listen(port, () => {
console.log(`Mock API server running at http://localhost:${port}`);
});
Python Example#
Using Flask, you can implement a similar mock API:
from flask import Flask, jsonify, request
import time
app = Flask(__name__)
@app.route('/api/data')
def get_data():
# Get delay from query parameter or use default
delay = request.args.get('delay', default=0, type=int)
# Simulate processing time
time.sleep(delay / 1000) # Convert to seconds
return jsonify({"message": "This is mock data", "timestamp": time.time()})
@app.route('/api/timeout')
def timeout():
# Get delay from query parameter
delay = request.args.get('delay', default=300000, type=int) # Default 5 minutes
# Simulate very long processing time
time.sleep(delay / 1000)
return jsonify({"message": "You shouldn't see this response"})
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5000)
Configuring Variable Response Times#
For realistic timeout testing, you'll want to simulate different response scenarios:
- Random Delays: Add unpredictability to mimic network instability.
- Progressive Delays: Gradually increase response times to test timeout thresholds.
- Conditional Delays: Apply delays based on request parameters or headers.
Here's a Node.js example showing these patterns:
// Random delay between min and max values
app.get("/api/random-delay", (req, res) => {
const min = parseInt(req.query.min) || 0;
const max = parseInt(req.query.max) || 5000;
const delay = Math.floor(Math.random() * (max - min)) + min;
console.log(`Responding with random delay: ${delay}ms`);
setTimeout(() => {
res.json({
message: "Response after random delay",
delay: delay,
});
}, delay);
});
// Progressive delay that increases with each request
let progressiveDelay = 100; // Starting delay
app.get("/api/progressive-delay", (req, res) => {
console.log(`Responding with progressive delay: ${progressiveDelay}ms`);
setTimeout(() => {
res.json({
message: "Response with progressive delay",
delay: progressiveDelay,
});
// Increase the delay for next request
progressiveDelay += 100;
}, progressiveDelay);
});
With your mock API environment set up, it's time to explore specific strategies for simulating timeout scenarios that will put your application to the test.
Timeout Testing Tactics: Four Killer Strategies That Reveal Weak Points#
Want to build systems that don't crumble under pressure? You need to know how your apps handle API timeouts before your users find out the hard way. Here are four powerful strategies to simulate API timeouts for stress testing in your controlled environment.
Deterministic Timeout Simulation#
Let's start with the basics—creating fixed, predictable delays. This lets you test specific timeout thresholds and see if your app handles them like a champ or falls apart like a cheap suit.
In Express.js, you can create an endpoint with a fixed delay:
const express = require("express");
const app = express();
// Endpoint with a fixed 5-second delay
app.get("/api/fixed-timeout", (req, res) => {
const DELAY_MS = 5000;
setTimeout(() => {
res.json({ message: "Response after fixed delay", delay: DELAY_MS });
}, DELAY_MS);
});
// Configurable timeout endpoint
app.get("/api/timeout/:duration", (req, res) => {
const duration = parseInt(req.params.duration) || 3000;
setTimeout(() => {
res.json({ message: "Response after configured delay", delay: duration });
}, duration);
});
app.listen(3000, () =>
console.log("Timeout simulation server running on port 3000"),
);
This approach tests how your application behaves when waiting for responses that take exactly 5 seconds, or any duration you specify—perfect for testing timeout thresholds in client apps or middleware.
Probabilistic Timeout Patterns#
Real-world API timeouts don't play by the rules. They're unpredictable beasts! For realistic stress testing, implement probabilistic timeout patterns that generate random response times following certain distributions.
A Gaussian (normal) distribution works like a charm for simulating real-world latency variations:
const express = require("express");
const app = express();
// Helper function to generate Gaussian-distributed random numbers
function gaussianRandom(mean, standardDeviation) {
// Box-Muller transform for normal distribution
let u = 0,
v = 0;
while (u === 0) u = Math.random();
while (v === 0) v = Math.random();
const z = Math.sqrt(-2.0 * Math.log(u)) * Math.cos(2.0 * Math.PI * v);
return z * standardDeviation + mean;
}
// Endpoint with Gaussian-distributed response times
app.get("/api/gaussian-timeout", (req, res) => {
// Mean: 500ms, Standard deviation: 200ms
const mean = 500;
const stdDev = 200;
const delay = Math.max(50, Math.round(gaussianRandom(mean, stdDev)));
setTimeout(() => {
res.json({
message: "Response with Gaussian-distributed delay",
delay: delay,
distribution: "gaussian",
mean,
stdDev,
});
}, delay);
});
app.listen(3000, () =>
console.log("Probabilistic timeout server running on port 3000"),
);
This simulation creates more realistic testing scenarios with occasional outliers, helping you spot how your system handles varying response times. Just adjust the mean and standard deviation to model different service behaviors.
Progressive Degradation Simulation#
Timeout issues don't just hit you like a truck—they sneak up as systems gradually degrade under load. Simulate this with time-dependent latency functions that increase delays over time:
const express = require("express");
const app = express();
// Variables to track progressive degradation
let serverStartTime = Date.now();
let requestCount = 0;
// Endpoint with progressive degradation
app.get("/api/degrading-performance", (req, res) => {
requestCount++;
// Calculate delay based on elapsed time and request count
const timeElapsedMinutes = (Date.now() - serverStartTime) / (1000 * 60);
const baseDelay = 100; // Base delay in ms
const timeMultiplier = Math.pow(1.1, timeElapsedMinutes); // 10% increase per minute
const loadMultiplier = 1 + requestCount / 100; // Load factor
const delay = Math.round(baseDelay * timeMultiplier * loadMultiplier);
setTimeout(() => {
res.json({
message: "Response from degrading service",
delay: delay,
requestCount: requestCount,
timeElapsedMinutes: timeElapsedMinutes.toFixed(2),
});
}, delay);
});
// Reset degradation simulation
app.post("/api/reset-degradation", (req, res) => {
serverStartTime = Date.now();
requestCount = 0;
res.json({ message: "Degradation simulation reset" });
});
app.listen(3000, () =>
console.log("Progressive degradation simulation running on port 3000"),
);
This simulation tests how your application handles gradually worsening conditions rather than sudden failures—perfect for testing circuit breakers and retry strategies.
Conditional Timeout Scenarios#
Timeouts often depend on specific request characteristics or resource conditions. These conditional scenarios test more targeted failure modes:
const express = require("express");
const app = express();
// Parse JSON request bodies
app.use(express.json());
// Request-dependent timeout simulation
app.post("/api/conditional-timeout", (req, res) => {
// Timeout based on payload size
const payloadSize = JSON.stringify(req.body).length;
const delay = Math.min(10000, payloadSize); // 1ms per byte, max 10 seconds
setTimeout(() => {
res.json({
message: "Response delay based on payload size",
payloadSize: payloadSize,
delay: delay,
});
}, delay);
});
// Resource-dependent timeout simulation
let resourceUtilization = 0.2; // 20% utilization to start
app.get("/api/resource-timeout", (req, res) => {
// Simulate increasing load
if (Math.random() < 0.1) {
resourceUtilization = Math.min(0.95, resourceUtilization + 0.05);
}
// Calculate delay based on resource utilization (exponential relationship)
const baseDelay = 100;
const delay = baseDelay * Math.pow(10, resourceUtilization * 2); // Exponential growth with utilization
setTimeout(() => {
res.json({
message: "Response delay based on resource utilization",
resourceUtilization: resourceUtilization.toFixed(2),
delay: Math.round(delay),
});
}, delay);
});
// Reset resource utilization
app.post("/api/reset-resources", (req, res) => {
resourceUtilization = 0.2;
res.json({ message: "Resource utilization reset" });
});
app.listen(3000, () =>
console.log("Conditional timeout simulation running on port 3000"),
);
These conditional scenarios let you test how your application handles specific failure modes related to payload size, resource constraints, or other factors—ideal for stress testing and finding edge cases in your error handling.
Beyond Simulation: Building Resilient Systems That Withstand Real-World Timeouts#
Now that you've mastered simulating API timeouts for stress testing, it's time to implement resilience patterns that will help your application survive when real timeouts occur. The insights gained from your timeout simulations should directly inform these implementations.
Circuit Breakers#
Circuit breakers "trip" when error thresholds are exceeded, temporarily blocking requests to problematic services and giving them time to recover. Operating in three states—closed (allowing requests), open (blocking requests), and half-open (testing recovery)—this pattern prevents resource exhaustion when downstream services fail. Your timeout simulations help fine-tune trip thresholds, recovery timeouts, and fallback mechanisms for optimal resilience.
Timeouts at Every Level#
Implement timeouts across multiple layers of your application stack—from socket-level network connections to high-level business operations. This creates a defense-in-depth strategy that prevents component failures from hanging your entire system. Configure inner timeouts (lower in the stack) to be shorter than outer timeouts (higher in the stack). Your simulation tests help calibrate these timeouts for optimal performance.
Smart Retry Strategies#
Not all failures are permanent, but naive retry implementations can worsen outages by creating "retry storms" that overwhelm already struggling services. Implement intelligent retry strategies with exponential backoff to gradually increase wait times between attempts, and add jitter (randomization) to prevent synchronized retry patterns across multiple clients.
Remember to set reasonable retry budgets for different failure types—immediate retries might make sense for connection errors, while server errors could require longer backoff periods. Your timeout simulations will reveal how different retry patterns affect system stability and recovery time, giving you real-world data to optimize these parameters.
Response Caching#
Sometimes, slightly outdated data beats no data at all. The "stale-while-revalidate" pattern serves cached responses immediately while asynchronously fetching fresh data in the background, maintaining responsiveness even when backends are slow or unavailable. Consider different freshness requirements for different data types—static reference data might remain valid for days, while transactional data could expire in seconds.
Your timeout simulations reveal which components are most vulnerable to failures and which data can reasonably be served from cache during outages, helping you determine optimal cache durations and invalidation strategies that balance data freshness against system availability.
From Simulation to Survival: Building APIs That Withstand the Storm#
API timeouts are inevitable in distributed systems. They're not a matter of if, but when. By simulating API timeouts for stress testing with mock APIs, you're preparing your application to handle these failures gracefully, ensuring a smooth experience for your users even when the underlying services are struggling.
The resilience patterns we've discussed—circuit breakers, multi-level timeouts, intelligent retry strategies, and response caching—provide a robust foundation for building systems that bend rather than break under pressure. Implementing these patterns based on insights gained from your timeout simulations will vastly improve your application's stability and user experience. Ready to transform how your APIs handle timeouts? Sign up for a free Zuplo account today and leverage our powerful tools for API management and resilience testing.