Cross-Site Request Forgery (CSRF) attacks are the silent predators of the API world—they trick authenticated users into performing actions they never intended, all without raising a single alarm. When attackers exploit these vulnerabilities, they can execute unauthorized transactions, steal sensitive data, or even gain administrative access to your entire system.
Think about it: without proper protection, a simple malicious link could trigger a $5,000 bank transfer when clicked by an authenticated user. And the scariest part? Real-world companies fall victim to attacks like these every day.
This means that understanding how to prevent cross-site request forgery in API calls isn't just a nice-to-have—it's essential for your digital survival. Let's get into the strategies that will keep your APIs safe from these invisible threats.
- The Unbreakable Shield: Core Principles of CSRF Prevention
- Tokens to the Rescue: Powerful Anti-CSRF Strategies for Modern APIs
- Cookies and Headers: Your Frontline Defense Against CSRF
- Beyond the Basics: Advanced CSRF Protection for Complex API Ecosystems
- Framework Defense: Implementing CSRF Protection in Your Favorite Tools
- Implementation Roadmap: Your Path to CSRF-Proof APIs
- Fortify Your APIs: Building an Unbreakable Defense System
The Unbreakable Shield: Core Principles of CSRF Prevention#
Protecting your APIs from CSRF attacks demands a strategic approach based on fundamental principles that work in harmony. By mastering these foundations, you'll build an impenetrable defense system that keeps attackers at bay.
Request Origin Verification#
The first principle is request origin verification—your API must distinguish between legitimate requests from your frontend and malicious ones from attackers' sites. This is where custom headers and Same-Origin Policy implementation become crucial tools in your security arsenal.
While following API authentication best practices forms the foundation of your security strategy, remember that CSRF attacks specifically target users who are already authenticated. This means your protection must go beyond just verifying identities—it needs to validate the legitimacy of each request.
State-Changing Operations Protection#
Pay special attention to state-changing operations like updating user data or transferring funds. These actions are prime targets for attackers and require additional verification mechanisms such as unique tokens and Role-Based Access Control that validate each request's authenticity.
User Intent Verification#
User intent verification answers a critical question: did your user actually mean to perform that action, or were they tricked? Implementing re-authentication for sensitive operations ensures that critical actions happen only when genuinely intended by your users.
Defense-in-Depth Strategy#
Never rely on a single security measure. A defense-in-depth strategy, incorporating API security best practices, implements multiple controls at different stages of the request process, creating redundant layers of protection. When one layer fails, your other defenses keep you protected—this isn't paranoia, it's prudent security planning.
Industry Standards Implementation#
Finally, don't reinvent the wheel. The OWASP CSRF Prevention Cheat Sheet provides battle-tested recommendations on anti-CSRF tokens, session management, and cookie attributes that have proven effective across countless applications.
Tokens to the Rescue: Powerful Anti-CSRF Strategies for Modern APIs#
When it comes to blocking CSRF attacks, token-based strategies are your heavy artillery. These approaches use unique, unpredictable tokens that make it virtually impossible for attackers to forge valid requests. Let's explore the two most effective methods that security professionals rely on.
The Synchronizer Token Pattern#
The Synchronizer Token Pattern remains the gold standard for CSRF protection despite its simplicity. Here's how it works: when a user logs in, your server generates a cryptographically strong random token that gets stored in the user's session. Every subsequent state-changing request must include this token or face immediate rejection.
Implementing this in Node.js is straightforward:
const crypto = require('crypto');
// Generate CSRF token
function generateCSRFToken() {
return crypto.randomBytes(32).toString('hex');
}
// Middleware to set CSRF token
app.use((req, res, next) => {
if (!req.session.csrfToken) {
req.session.csrfToken = generateCSRFToken();
}
res.locals.csrfToken = req.session.csrfToken;
next();
});
// Validate CSRF token
function validateCSRFToken(req, res, next) {
if (req.method === 'POST') {
if (req.body._csrf !== req.session.csrfToken) {
return res.status(403).send('Invalid CSRF token');
}
}
next();
}
*// Validate CSRF token*
function validateCSRFToken(req, res, next) {
if (req.method \=== 'POST') {
if (req.body.\_csrf \!== req.session.csrfToken) {
return res.status(403).send('Invalid CSRF token');
}
}
next();
}
While highly effective, this pattern does require server-side storage, which might be a consideration for highly scalable stateless architectures.
The Double-Submit Cookie Method#
For those building stateless APIs or microservices, the Double-Submit Cookie method offers a compelling alternative. This approach sets a cookie with a random CSRF token when a user authenticates. For every state-changing request, the client must submit this same token in a request header or parameter. The server simply compares the tokens—if they match, the request proceeds; if not, it's rejected.
Here's a practical implementation:
const crypto = require("crypto");
// Set CSRF cookie
app.use((req, res, next) => {
if (!req.cookies.csrfToken) {
const csrfToken = crypto.randomBytes(32).toString("hex");
res.cookie("csrfToken", csrfToken, { httpOnly: true, sameSite: "strict" });
}
next();
});
// Validate CSRF token
function validateCSRFToken(req, res, next) {
const cookieToken = req.cookies.csrfToken;
const headerToken = req.headers["x-csrf-token"];
if (!cookieToken || !headerToken || cookieToken !== headerToken) {
return res.status(403).send("Invalid CSRF token");
}
next();
}
The beauty of this method is that it eliminates the need for server-side storage, making it ideal for RESTful APIs and microservices architectures. However, it does depend heavily on cookie security, so additional precautions are necessary.
Your choice between these methods will likely depend on your architecture. Building stateless APIs? The Double-Submit Cookie method is probably your best bet. Working with applications that maintain server-side state? The Synchronizer Token Pattern offers simplicity and rock-solid security.
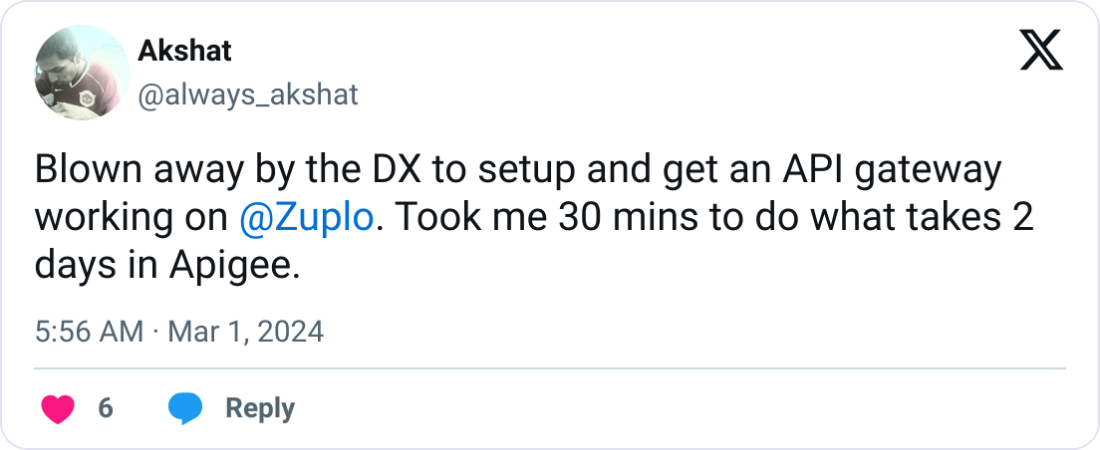
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreCookies and Headers: Your Frontline Defense Against CSRF#
While tokens form the core of your protection strategy, properly configured headers and cookies act as your first line of defense, stopping many CSRF attempts before they even reach your application logic. These browser-based security features provide powerful protection with minimal implementation effort.
SameSite Cookie Attributes#
SameSite cookies function like bouncers for your API requests—they control which cross-origin requests can include your cookies. With three different settings, you can precisely control cookie behavior:
- Strict: Cookies only go out when the request comes directly from your site.
- Lax: A balanced option where cookies are included when users navigate to your site from elsewhere.
- None: Cookies are sent with all requests but must use HTTPS.
Setting up SameSite cookies requires just a simple configuration:
res.cookie("sessionId", "abc123", {
httpOnly: true,
secure: true,
sameSite: "strict",
});
While powerful, SameSite cookies have limitations—they don't protect against attacks from subdomains, and older browsers might ignore this setting altogether. That's why a multi-layered approach is essential.
Custom HTTP Headers Implementation#
Custom HTTP headers create a "secret handshake" between your frontend and API. Thanks to browser Same-Origin Policy restrictions, malicious sites cannot set custom headers on cross-origin requests, making them excellent verification tools.
The X-Requested-With
header has long been a standard approach. Many JavaScript
frameworks automatically set this to XMLHttpRequest
for AJAX calls:
app.use((req, res, next) => {
if (
req.method === "POST" &&
req.headers["x-requested-with"] !== "XMLHttpRequest"
) {
return res.status(403).json({ error: "CSRF validation failed" });
}
next();
});
Token-Based Header Protection#
The X-CSRF-Token
approach takes this concept further by requiring a unique
token for each session:
const csrf = require("csurf");
const csrfProtection = csrf({ cookie: true });
app.use(csrfProtection);
app.get("/form", (req, res) => {
res.render("form", { csrfToken: req.csrfToken() });
});
app.post("/process", (req, res) => {
res.send("Data is being processed");
});
In practice, combining SameSite cookies with custom headers creates an exceptionally strong defense. This multi-layered approach follows the principle of defense in depth—forcing attackers to overcome multiple barriers before they can successfully exploit your API.
Beyond the Basics: Advanced CSRF Protection for Complex API Ecosystems#
As your API ecosystem grows in complexity, so too must your security strategies. Single-page applications, third-party integrations, and microservice architectures all introduce unique challenges that require sophisticated protection approaches.
Single-Page Application Challenges#
Client-side CSRF vulnerabilities present special challenges, particularly in single-page applications that rely heavily on AJAX for state changes. Unlike traditional server-rendered apps, SPAs operate differently and require tailored protection strategies.
Custom HTTP headers remain one of your strongest defenses. Implement them in your frontend AJAX calls like this:
// Add this to your frontend AJAX calls
fetch("/api/update-profile", {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-Requested-With": "XMLHttpRequest",
},
body: JSON.stringify(data),
});
Token handling requires special care in SPAs. Never store anti-CSRF tokens in localStorage or sessionStorage where they're vulnerable to XSS attacks. Instead, use HttpOnly cookies when possible, or implement secure token rotation strategies.
Origin and Referrer Verification#
Origin and Referrer headers provide additional verification layers. While not foolproof on their own, they add valuable security when combined with other protections:
// Server-side validation
if (req.headers.origin !== "https://yourapp.com") {
return res.status(403).send("Invalid origin");
}
Content Security Policy (CSP) prevents malicious script execution that might forge requests:
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted-cdn.com;
Third-Party Integration Security#
Third-party code integration demands extra vigilance. Not all libraries prioritize security the way you do. Always use Subresource Integrity (SRI) when loading external scripts:
<script
src="https://cdn.example.com/script.js"
integrity="sha384-oqVuAfXRKap7fdgcCY5uykM6+R9GqQ8K/uxy9rx7HNQlGYl1kPzQho1wx4JwY8wC"
crossorigin="anonymous"
></script>
For maximum security, consider self-hosting critical libraries. While it creates additional maintenance work, it gives you complete control over your code.
CORS and Input Validation#
A comprehensive defense strategy must also include proper CORS configuration. Be specific with your allowed origins:
// Don't do this in production!
res.header("Access-Control-Allow-Origin", "*");
// Instead, be explicit
res.header("Access-Control-Allow-Origin", "https://yourapp.com");
Input validation, such as query parameter validation, on both client and server sides prevents injection attacks that might bypass CSRF protections. Control referrer information with appropriate policies:
Referrer-Policy: strict-origin-when-cross-origin
Monitoring and Security Culture#
Implement monitoring and logging to detect suspicious patterns like traffic spikes from specific origins, failed CSRF validations, or missing custom headers.
Remember that security awareness should permeate your development culture. Regular security audits and ongoing team education about CSRF risks are just as important as your technical measures.
Framework Defense: Implementing CSRF Protection in Your Favorite Tools#
Most modern frameworks include built-in CSRF protection, but using these features correctly is what separates secure applications from vulnerable ones. Let's explore how to implement effective protection across popular development frameworks.
Express.js Implementation#
Express doesn't include CSRF protection by default, but the csurf
middleware
makes implementation straightforward:
const express = require("express");
const csrf = require("csurf");
const cookieParser = require("cookie-parser");
const app = express();
// Parse cookies
app.use(cookieParser());
// Enable CSRF protection
app.use(csrf({ cookie: true }));
// Add CSRF token to all responses
app.use((req, res, next) => {
res.locals.csrfToken = req.csrfToken();
next();
});
For your forms or AJAX requests, include the token like this:
<form action="/submit" method="POST">
<input type="hidden" name="_csrf" value="{{csrfToken}}" />
<!-- Other form fields -->
</form>
While Express offers flexibility, that same quality means you must carefully configure security settings, especially for API-only applications. For practical implementations, you can refer to Zuplo examples that demonstrate how to integrate security features into your API development.
Django Protection Features#
Django takes a proactive security stance with CSRF protection enabled by default. Ensure the middleware is active in your settings:
MIDDLEWARE = [
# ...
'django.middleware.csrf.CsrfViewMiddleware',
# ...
]
In your templates, include the token in forms:
<form method="POST">
{% csrf_token %}
<!-- Form fields -->
</form>
For AJAX requests, grab the token from cookies:
const csrftoken = getCookie("csrftoken");
fetch(url, {
method: "POST",
headers: {
"X-CSRFToken": csrftoken,
},
// ...
});
Django's protection works excellently for traditional web apps, but requires adjustments for API-only backends or SPAs.
Ruby on Rails Security#
Ruby on Rails provides built-in CSRF protection through protect_from_forgery
:
class ApplicationController < ActionController::Base
protect_from_forgery with: :exception
end
Rails automatically handles token insertion in forms:
<%= form_for @user do |f| %>
<%= f.text_field :name %>
<%= f.submit %>
<% end %>
For AJAX requests, Rails includes the CSRF token in meta tags:
$.ajax({
url: "/users",
type: "POST",
beforeSend: function (xhr) {
xhr.setRequestHeader(
"X-CSRF-Token",
$('meta[name="csrf-token"]').attr("content"),
);
},
// ...
});
ASP.NET Core Protection#
ASP.NET Core provides robust CSRF protection via AntiForgeryToken
. Configure
it in your startup:
services.AddAntiforgery(options => options.HeaderName = "X-CSRF-TOKEN");
Protect your controller actions:
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Create(User user)
{
// Action logic
}
Include the token in forms:
<form asp-action="Create" method="post">
@Html.AntiForgeryToken()
<!-- Form fields -->
</form>
For AJAX requests:
$.ajax({
url: "/api/users",
type: "POST",
beforeSend: function (xhr) {
xhr.setRequestHeader(
"X-CSRF-TOKEN",
$('input:hidden[name="__RequestVerificationToken"]').val(),
);
},
// ...
});
Verification Testing#
To verify that your protection actually works, create a form or API endpoint that performs an important action, then try submitting without the CSRF token. You should receive an error message, not a successful response. Then add the correct token and confirm it works properly.
Implementation Roadmap: Your Path to CSRF-Proof APIs#
Moving from theory to practice requires a clear plan. Follow this step-by-step roadmap to systematically strengthen your API defenses against CSRF attacks.
Assess Your Current Vulnerabilities#
- Conduct a thorough security audit of existing endpoints, focusing on state-changing operations
- Identify authentication mechanisms that might be susceptible to CSRF attacks
- Review your application architecture to determine appropriate protection strategies
- Document all form submissions, AJAX calls, and other client-server interactions
Choose Your Protection Strategy#
- Select token-based approaches that align with your application architecture
- Determine appropriate cookie security settings based on your user experience requirements
- Decide on custom header implementations that complement your frontend technology
- Plan for defense-in-depth by implementing multiple protective layers
Implement Core Protections#
- Add CSRF token generation and validation to your authentication flow
- Configure cookies with appropriate SameSite attributes and other security flags
- Modify frontend code to include tokens or custom headers with all state-changing requests
- Update your API endpoints to validate incoming requests properly
Test Your Defenses#
- Create specific test cases that attempt to bypass your CSRF protections
- Perform cross-browser testing to ensure compatibility with your security mechanisms
- Use penetration testing tools to simulate actual attack scenarios
- Verify that legitimate requests work correctly while malicious ones are blocked
Monitor and Maintain#
- Implement logging for all CSRF validation failures to catch potential attack attempts
- Establish alerts for suspicious patterns that might indicate CSRF attacks
- Schedule regular security reviews to address emerging vulnerabilities
- Keep your protection mechanisms updated as browsers and standards evolve
Educate Your Team#
- Train developers on CSRF risks and proper implementation of protective measures
- Create clear documentation for your CSRF protection strategy
- Establish security standards for new features and endpoints
- Include CSRF testing in your code review process
Fortify Your APIs: Building an Unbreakable Defense System#
CSRF attacks continue to threaten API ecosystems, and the stakes have never been higher. The most important lesson? No single defense can provide complete protection. A multi-layered approach creates redundant security barriers that protect your systems even when one defense fails. Evaluating your current CSRF defenses, identifying gaps in your protection strategy, and implementing the techniques we've covered creates a truly secure API ecosystem that earns your users' trust.
Don't wait until after a breach to take API security seriously. Your users trust you with their data and transactions—prove that their trust is well-placed with uncompromising security practices that go beyond basic authentication and build the foundation for long-term security success.
Ready to elevate your API security? Try Zuplo's comprehensive API management platform and experience what truly robust, enterprise-grade protection feels like without the complexity. Sign up for your free account today.