Ever noticed how the best RESTful APIs feel intuitive, like they're reading your mind? That's no accident. Behind every smooth API experience is a thoughtful design that balances simplicity, performance, and developer experience.
Most developers waste hours wrestling with complex configurations when building APIs. Here's the truth: a code-first approach can dramatically speed up your development without sacrificing performance. It is actually possible to build RESTful APIs that developers want to use, with CRUD operations as your foundation. Let’s break down how CRUD operations power the core of every well-designed RESTful API—and how to get them right.
- The CRUD Superheroes: Your API's Essential Building Blocks
- REST Like a Boss: Core Principles for Scalable APIs
- From Zero to Hero: Building Your First CRUD API
- Code That Rocks: Implementing CRUD Operations
- Show and Tell: Documentation That Doesn't Suck
- Level Up: Taking Your API to Production
- Build Better APIs, Start Today
The CRUD Superheroes: Your API's Essential Building Blocks#
CRUD operations are the backbone of data-driven applications, providing a standardized way to interact with any persistence layer and understand API fundamentals.
Definition of CRUD Operations#
Think of CRUD as the data management superheroes your application needs:
- Create: Where the magic begins. Adding new records to your database happens here, whether it's a user signing up or a new product hitting your inventory.
- Read: The workhorse of most applications. This retrieves information from your database and powers everything from browsing products to scrolling through social media.
- Update: Change is inevitable. This operation modifies existing data when users edit profiles or update settings.
- Delete: Sometimes things need to disappear. This operation removes records from your database—like when users delete comments or remove items from carts.
These operations translate directly to real business functionality in virtually every data-driven application. In RESTful APIs, CRUD operations map beautifully to specific HTTP methods:
Create → POST: Creates a new resource, returning a 201 (Created) status code.
Read → GET: Retrieves resources, returning a 200 (OK) with the requested data.
Update → PUT/PATCH: PUT replaces an entire resource while PATCH updates specific fields.
Delete → DELETE: Removes a resource, typically returning 204 (No Content).
For example, a product management API might use:
POST /products (Create a new product)
GET /products/123 (Read product #123)
PUT /products/123 (Update all fields of product #123)
PATCH /products/123 (Update specific fields)
DELETE /products/123 (Remove product #123)
When operations fail, your API should return appropriate error codes, such as 400 for invalid inputs or 404 when resources don't exist.
REST Like a Boss: Core Principles for Scalable APIs#
Understanding what makes an API truly RESTful helps you create interfaces that remain manageable even as applications grow.
What is a RESTful API?#
REST is an architectural style introduced by Roy Fielding in 2000 that defines a set of constraints for web services.
- Statelessness: Each request contains everything needed to complete it. No server-side session data means horizontal scaling becomes a breeze.
- Client-Server Architecture: The client and server evolve independently, connected only through the uniform interface.
- Layered System: Clients can't tell whether they're connected directly to the server or through intermediaries like load balancers.
- Uniform Interface: Resources are identified in requests, manipulated through representations, and include self-descriptive messages.
These principles create APIs that scale horizontally, enable caching, and allow independent evolution of client and server components—critical advantages for distributed systems.
Important Design Considerations#
Building RESTful APIs that developers love requires attention to several key design aspects.
Naming Conventions#
Resource names should be plural nouns (e.g., /products
not /product
), and
endpoints should describe resources, not actions. This creates intuitive,
discoverable APIs.
Versioning#
Managing API versions and deprecations carefully can prevent breaking client applications faster than you can say "regression testing." Utilizing proper API versioning strategies and API deprecation management is crucial. Here are effective strategies:
- URL path versioning:
/api/v1/products
- Custom headers:
X-API-Version: 1
- Accept header with versioning:
Accept: application/vnd.company.v1+json
Error Handling#
Error responses should provide clear information about what went wrong and how to fix it. A consistent structure might look like:
{
"error": "ValidationError",
"message": "Invalid product data",
"details": ["Price must be positive", "Name is required"]
}
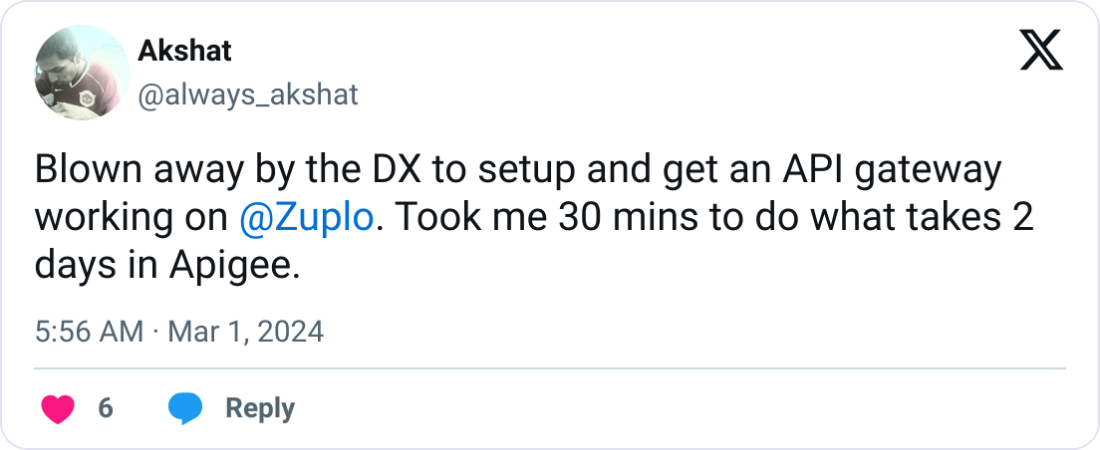
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreAuthentication and Authorization#
Secure your APIs with various API authentication methods:
- API keys for simple internal services
- JWT (JSON Web Tokens) for stateless authentication
- OAuth 2.0 for third-party integrations
From Zero to Hero: Building Your First CRUD API#
The difference between a good API and a great one often comes down to implementation details. Let's explore how to bring CRUD operations to life and start creating a CRUD API with clean, maintainable code.
Getting Started with API Development#
Building a CRUD API starts with picking the right tools for your stack:
- Node.js: Express.js gives you a lightweight framework with excellent middleware support.
- Python: Flask keeps it simple (checkout our guide to building Flask APIs), while Django REST Framework delivers batteries-included functionality. FastAPI is also quickly becoming the most popular framework (see our FastAPI tutorial)
- Java: Spring Boot (see API tutorial) eliminates configuration hell with smart defaults.
- Go: Gin and Chi frameworks deliver blazing-fast APIs with minimal overhead. Huma seems to be the new community framework (you bet we have a Huma tutorial too)
A code-first approach simplifies this whole process by focusing on business logic rather than endless configuration files. For practical steps on creating a CRUD API, consider using Express.js (please follow their installation instructions to set up your project before proceeding):
app.post("/products", (req, res) => {
// Validate request body
const newProduct = productService.create(req.body);
res.status(201).json(newProduct);
});
This approach eliminates complex YAML or JSON configuration files that separate implementation from interface definition.
Crafting APIs with Best Practices#
CRUD Naming Conventions and URL Design#
- Use nouns, not verbs:
/products
not/getProducts
(because HTTP methods already express the verb). - Express hierarchies with nested resources:
/users/123/orders
. - Keep URLs simple and readable:
/products?category=electronics
.
Poor design: /api/getProductById/123/doUpdate
Better design: /api/products/123
Versioning Strategies#
Each approach has trade-offs:
- URL path versioning (
/v1/products
) is explicit but clutters URLs. - Header-based versioning keeps URLs clean but is less visible.
- Content negotiation (
Accept: application/vnd.api.v2+json
) is RESTful but complex.
URL versioning works best for most teams because it's simple to understand.
Error Handling and Exceptions#
Standardize error responses across all endpoints:
{
"status": 400,
"code": "INVALID_INPUT",
"message": "Validation failed",
"details": [{ "field": "email", "issue": "Invalid format" }]
}
Code That Rocks: Implementing CRUD Operations#
Let's look at real-world code examples that bring CRUD operations to life in a
RESTful context. In the examples below, we use Product
as a stand-in for your
data model - this can vary based on the data store you use. Here's some examples
of using
Firebase/Firestore,
MySQL, and
Supabase.
Create Operation (POST):
// Express.js example
app.post("/products", async (req, res) => {
try {
// Input validation
const { error } = validateProduct(req.body);
if (error) return res.status(400).json({ error: error.details });
const product = await Product.create(req.body);
return res.status(201).json(product);
} catch (err) {
return res.status(500).json({ error: "Failed to create product" });
}
});
Read Operation (GET):
// Single resource
app.get("/products/:id", async (req, res) => {
try {
const product = await Product.findById(req.params.id);
if (!product) return res.status(404).json({ error: "Product not found" });
return res.status(200).json(product);
} catch (err) {
return res.status(500).json({ error: "Server error" });
}
});
// Collection
app.get("/products", async (req, res) => {
// Support filtering, pagination
const { category, limit = 20, page = 1 } = req.query;
const skip = (page - 1) * limit;
try {
const query = category ? { category } : {};
const products = await Product.find(query).limit(limit).skip(skip);
return res.status(200).json(products);
} catch (err) {
return res.status(500).json({ error: "Server error" });
}
});
Always validate input data before processing, handle errors gracefully, and return appropriate status codes with meaningful responses. For developers transitioning from SQL to RESTful APIs, understanding how to convert SQL queries to API requests is essential.
Update Operations (PUT/PATCH):
// Full replacement (PUT)
app.put("/products/:id", async (req, res) => {
try {
const { error } = validateProduct(req.body);
if (error) return res.status(400).json({ error: error.details });
const product = await Product.findByIdAndUpdate(req.params.id, req.body, {
new: true,
runValidators: true,
});
if (!product) return res.status(404).json({ error: "Product not found" });
return res.status(200).json(product);
} catch (err) {
return res.status(500).json({ error: "Update failed" });
}
});
// Partial update (PATCH)
app.patch("/products/:id", async (req, res) => {
try {
// Only validate fields that are being updated
const product = await Product.findByIdAndUpdate(
req.params.id,
{ $set: req.body },
{ new: true, runValidators: true },
);
if (!product) return res.status(404).json({ error: "Product not found" });
return res.status(200).json(product);
} catch (err) {
return res.status(500).json({ error: "Update failed" });
}
});
Delete Operation (DELETE):
app.delete("/products/:id", async (req, res) => {
try {
const product = await Product.findByIdAndDelete(req.params.id);
if (!product) return res.status(404).json({ error: "Product not found" });
return res.status(204).send();
} catch (err) {
return res.status(500).json({ error: "Deletion failed" });
}
});
Consider these important aspects when implementing updates and deletes:
Idempotency#
PUT and DELETE should be idempotent—call them once or a hundred times, and you'll get the same result. This makes retry logic dramatically simpler when network glitches occur.
Soft Deletes#
For many applications, implementing "soft deletes" (flagging records as deleted rather than actually removing them) preserves data integrity and audit trails.
Rate Limiting#
Implementing and managing API rate limits helps prevent abuse, especially for destructive operations like DELETE. Nothing ruins your day faster than an API that lets someone delete your entire database in a few seconds.
Show and Tell: Documentation That Doesn't Suck#
An API without documentation is like a house with no doors: technically impressive but completely useless to everyone. Good docs transform a good API into a great one.
Importance of API Documentation#
Comprehensive documentation serves as both a contract and guide for API consumers. According to Stack Overflow's 2023 Developer Survey, inadequate documentation remains among developers' top frustrations.
OpenAPI (formerly Swagger) has emerged as the de facto standard for API documentation. It allows you to:
- Describe Your API's Capabilities: Document endpoints, parameters, request bodies, and responses in a standardized format.
- Generate Interactive Documentation: Turn dry specs into interactive playgrounds where developers can test your API directly in the browser.
- Create Client Libraries Automatically: Generate SDK code in multiple languages, saving hundreds of development hours.
- Enable Contract Testing: Verify that your API implementation matches its specification, catching drift before it breaks clients.
Tools like Zudoku transform OpenAPI definitions into interactive documentation.
Documentation should include:
- Authentication requirements
- Request/response formats with examples
- Error codes and their meanings
- Rate limiting policies
- Versioning information
For code-first approaches, libraries like swagger-jsdoc let you generate OpenAPI specs from code comments:
/**
* @swagger
* /products:
* get:
* summary: Returns all products
* responses:
* 200:
* description: List of products
*/
app.get("/products", (req, res) => {
// Implementation
});
Testing and Ensuring Quality#
Robust testing ensures your API behaves as expected and maintains reliability as it evolves. For comprehensive guidelines on API testing best practices, consider the following testing types:
Unit Testing#
Test individual functions and controllers in isolation:
test("createProduct returns 400 for invalid input", async () => {
const req = { body: { price: -10 } };
const res = mockResponse();
await productController.createProduct(req, res);
expect(res.status).toHaveBeenCalledWith(400);
});
Integration Testing#
Verify interactions between components:
test("POST /products creates a product in database", async () => {
const response = await request(app)
.post("/products")
.send({ name: "Test Product", price: 19.99 });
expect(response.status).toBe(201);
const savedProduct = await Product.findById(response.body.id);
expect(savedProduct).not.toBeNull();
});
Postman and Insomnia provide user-friendly interfaces for manual and automated API testing, while testing libraries like Jest, Mocha, and pytest support programmatic tests.
Implementing continuous integration to run tests on every code change prevents regressions and keeps quality high. GitHub Actions makes this straightforward to set up.
Level Up: Taking Your API to Production#
As your API grows, API lifecycle management becomes important for maintaining performance and reliability in real-world scenarios.
Scaling and Performance Optimization#
High-traffic APIs require thoughtful optimization and adherence to API security best practices. For strategies on improving API performance, consider:
- Caching Strategies - Implement HTTP caching with appropriate Cache-Control headers, use Redis for frequently accessed data, and develop smart cache invalidation strategies.
- Load Distribution - Deploy your APIs to multiple regions using edge computing platforms to reduce latency for global users.
- Database Optimization - Index fields used in common queries, consider read replicas for query-heavy workloads, and implement pagination to limit response sizes.
Monitoring and Observability#
Track key metrics like:
- Request volume and latency
- Error rates by endpoint
- Resource utilization
Real-World Examples and Case Studies#
Learning from real-world implementations can provide valuable insights:
- Payment Processing APIs: Stripe's API is the gold standard of RESTful design with clear resource naming and consistent error handling.
- E-Commerce Product Management: Platforms like Shopify show how to design APIs that handle complex inventory and catalog management.
- Content Management Systems: WordPress REST API demonstrates how to evolve a traditional application into a headless CMS.
When examining these examples, pay attention to:
- How they handle authentication and authorization
- Their approach to versioning and backward compatibility
- Implementation of business rules and validations
- Strategies for bulk operations and performance optimization
Build Better APIs, Start Today#
Great APIs aren't born perfect; they evolve through real-world usage and feedback. Start with clean design and solid implementation, then refine based on actual use cases and performance data.
Ready to build APIs that developers love? Skip the configuration headaches and focus on delivering business value faster. Sign up for Zuplo today and start building, securing, and managing your APIs with less hassle and more confidence. Your developers (and your future self) will thank you.