API security has become a critical concern for businesses of all sizes. With organizations increasingly relying on APIs to power their applications and services, robust security measures are essential. The OWASP API Security Cheat Sheet offers invaluable guidance to help secure APIs, protect sensitive data, maintain user trust, and meet industry standards and regulatory requirements.
API-based attacks are on the rise, with the OWASP API Security Top 10 identifying broken object-level authorization and broken authentication as the top two API security risks. This growing threat landscape requires organizations to align with established security best practices to mitigate risks while maintaining development efficiency. This approach allows teams to focus on innovation without compromising security.
The API security landscape constantly evolves. In this article, we'll explore essential API security concepts, examine OWASP's recommendations, and discuss best practices to create secure, high-performing APIs.
- Understanding API Security Basics with The OWASP API Security Cheat Sheet
- Overview of The OWASP API Security Cheat Sheet
- Core Security Measures Recommended by The OWASP API Security Cheat Sheet
- Advanced Security Controls
- Implementation Strategies Using The OWASP API Security Cheat Sheet
- Gap Opportunities and Enhancements in API Security
- Strengthening Your API Security with OWASP Best Practices
Understanding API Security Basics with The OWASP API Security Cheat Sheet#
API security is the practice of protecting APIs from unauthorized access, malicious attacks, and data breaches. As APIs have become fundamental components of modern software architecture, using APIs responsibly and securing them has become critically important for maintaining user trust and protecting sensitive data. The OWASP REST Security Cheat Sheet provides essential guidelines to address these concerns effectively.
Definition of API Security#
API security encompasses measures designed to safeguard the integrity, confidentiality, and availability of APIs. It involves implementing authentication, authorization, encryption, and monitoring mechanisms to ensure that only authorized users and applications can access API endpoints.
Common API Security Threats#
Several security threats plague APIs, making them attractive targets for attackers:
- Broken Object Level Authorization (BOLA): APIs fail to properly verify that a user is authorized to access specific resources. An attacker might access another user's data by simply modifying object identifiers in API requests.
- Broken Authentication: Poor implementation of authentication mechanisms can allow attackers to compromise tokens or exploit flaws to impersonate legitimate users.
- Excessive Data Exposure: APIs may inadvertently expose sensitive data by returning more information than necessary in responses.
- Injection Attacks: Malicious input can manipulate API behavior, potentially leading to unauthorized data access or system compromise.
- Improper Asset Management: Outdated or poorly documented APIs can create security vulnerabilities if left unmanaged.
Importance of Proactive Security Measures#
Implementing proactive security measures is crucial for protecting APIs from these threats and is a key component of effective API lifecycle management. According to the OWASP API Security Project, a reactive approach to API security just doesn't cut it anymore.
Proactive security measures include:
- Implementing robust authentication and authorization checks consistently across all API endpoints.
- Enforcing strict input validation and sanitization to prevent injection attacks.
- Using encryption for data in transit and at rest to protect sensitive information, reinforcing data protection and security.
- Regularly auditing and updating API documentation and access controls.
- Monitoring API usage for suspicious activity and implementing rate limiting to prevent abuse.
API security best practices don't have to be complex. Modern API management platforms allow developers to implement security measures directly in their code, rather than through complex configurations. This code-first approach integrates security seamlessly into development workflows, ensuring security is built into APIs from the ground up.
Overview of The OWASP API Security Cheat Sheet#
The OWASP REST Security Cheat Sheet is a cornerstone resource for API security, giving developers, tech leads, and security professionals a practical guide to securing their APIs. This tool is part of the larger OWASP Cheat Sheet Series, which provides practical guidance on various application security topics.
Purpose and Authority#
The OWASP REST Security Cheat Sheet offers clear, actionable advice for addressing the unique security challenges of modern APIs. It turns complex security requirements into practical steps, making it an essential reference for teams at all stages of API development.
OWASP's authority in application security is unmatched, with over two decades of community-driven expertise behind its recommendations. This collective knowledge ensures the cheat sheet addresses real-world challenges, not just theoretical concepts.
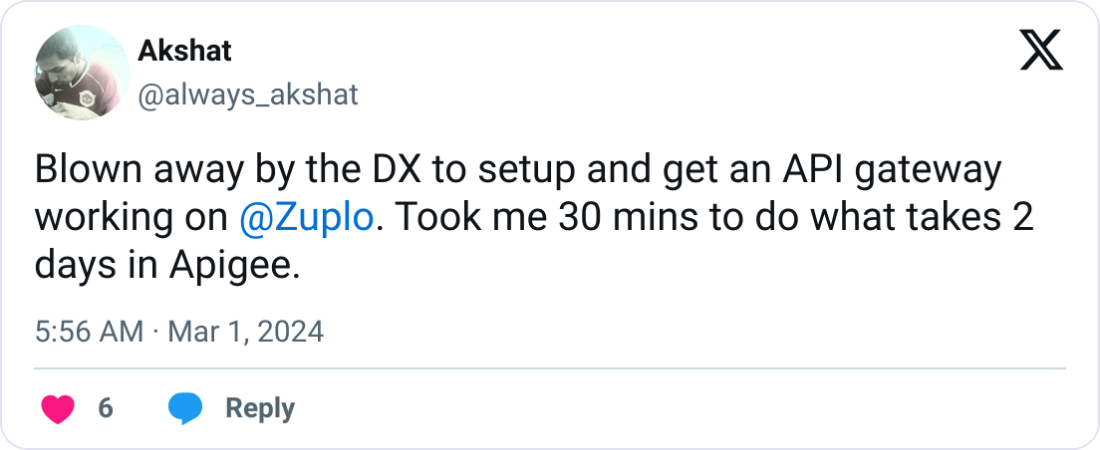
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreUnderstanding Cheat Sheets#
OWASP cheat sheets are practical tools for implementing complex security protocols. They connect high-level security principles with concrete implementation details. For API security, this means specific guidance on issues like:
- Transport Layer Security: The REST Security Cheat Sheet emphasizes that "Secure REST services must only provide HTTPS endpoints" to protect authentication credentials in transit.
- Authentication Mechanisms: Detailed advice on secure password handling, token-based authentication, and multi-factor authentication is provided, aligning with best practices outlined in the OWASP Authentication Cheat Sheet.
- Authorization: The cheat sheet stresses the importance of implementing proper object-level and function-level authorization to prevent common vulnerabilities like Broken Object Level Authorization (BOLA).
- Input Validation: Guidance on validating and sanitizing all input to prevent injection attacks and other security risks is a key focus.
- Error Handling: Recommendations for secure error handling that doesn't expose sensitive information to potential attackers.
- Rate Limiting: Strategies for implementing rate limiting to protect against abuse and denial-of-service attacks.
The structured approach of The OWASP API Security Top 10 provides a methodical way to address critical API security risks. This framework helps development teams prioritize their security efforts, focusing on high-impact areas first.
Core Security Measures Recommended by The OWASP API Security Cheat Sheet#
The OWASP REST Security Cheat Sheet provides comprehensive guidance on implementing robust security measures for your APIs. Let's explore the fundamental security controls recommended by OWASP and how you can effectively implement them.
Authentication and Authorization#
Proper authentication and authorization are critical for protecting your API from unauthorized access and are central to API management best practices:
- Use secure authentication protocols like OAuth 2.0 or OpenID Connect.
- Implement multi-factor authentication for sensitive operations.
- Enforce proper object-level and function-level authorization checks.
For example, when implementing object-level authorization:
@app.route('/api/records/<record_id>', methods=['GET'])
@jwt_required
def get_record(record_id):
current_user = get_jwt_identity()
record = Record.query.get(record_id)
if not record:
return jsonify({"error": "Record not found"}), 404
if record.owner_id != current_user and not user_has_admin_role(current_user):
logging.warning(f"User {current_user} attempted unauthorized access to record {record_id}")
return jsonify({"error": "Not authorized to access this record"}), 403
return jsonify(record.to_dict()), 200
This example demonstrates proper authorization checks, ensuring that only the record owner or an admin can access the data.
Data Validation and Encoding#
To prevent injection attacks and other data-related vulnerabilities, it is crucial to implement security features such as:
- Validate all input parameters for length, format, and type.
- Use strong typing and restrict string inputs with regular expressions.
- Implement server-side validation in addition to any client-side checks.
- Sanitize and encode all output to prevent XSS attacks.
Data Encryption#
Protecting sensitive data both in transit and at rest is crucial:
- Use HTTPS for all API communication to encrypt data in transit.
- Implement proper encryption for sensitive data stored in databases.
- Use strong, up-to-date encryption algorithms and key management practices.
To enforce HTTPS usage, you can implement strict transport security headers:
from flask import Flask
app = Flask(__name__)
@app.after_request
def add_security_headers(response):
response.headers['Strict-Transport-Security'] = 'max-age=31536000; includeSubDomains'
return response
This code adds the Strict-Transport-Security header to all responses, instructing browsers to always use HTTPS for your API.
Advanced Security Controls#
When it comes to API security, basic measures are just the starting point. Advanced security controls protect against sophisticated threats.
Rate Limiting and Throttling#
Rate limiting and throttling prevent abuse and protect your API from denial-of-service (DoS) attacks. These controls keep your API available and responsive, even under heavy load or during attacks.
To implement effective rate limiting:
- Set appropriate limits based on your API's capacity and expected usage.
- Use a sliding window algorithm for more precise control.
- Return a 429 (Too Many Requests) HTTP status code when limits are exceeded.
- Provide clear documentation on rate limits for your API consumers.
You can check out our full guide to understanding and building API rate limiting to learn more about best practices for rate limit design and implementation.
Monitoring and Logging#
Comprehensive monitoring and logging, utilizing effective API monitoring tools, help detect and respond to security incidents. These practices give you insights into your API's usage patterns and potential security threats.
Key aspects of effective monitoring and logging include:
- Tracking authentication failures, issues with authorization mechanisms, and input validation errors.
- Monitoring for unusual access patterns or traffic spikes.
- Logging all API requests and responses for auditing.
- Implementing real-time alerting for critical security events.
Security Testing and Audits#
Regular security testing and audits identify vulnerabilities before they become major problems. Incorporating these practices into your development workflow helps catch potential issues early.
Consider implementing:
- Automated security scanning as part of your CI/CD pipeline.
- Regular penetration testing by security experts.
- Code reviews focused on security best practices.
- Periodic security audits to assess overall API security.
Implementation Strategies Using The OWASP API Security Cheat Sheet#
Implementing The OWASP REST Security Cheat Sheet recommendations requires integrating security throughout the API development lifecycle, including in your API deployment strategies and leveraging effective API management solutions.
Design Phase Security Considerations#
When designing your API, making informed infrastructure choices and incorporating security requirements based on The OWASP REST Security Cheat Sheet recommendations is essential. Start with threat modeling exercises like STRIDE to identify where specific controls are most needed.
Consider adopting security stories in your Agile sprints:
"As a developer, I will implement input validation for all API parameters using regular expressions to prevent injection attacks."
This approach treats security as a functional requirement rather than an afterthought.
Integrating Security in Development Cycles#
During development, enforce coding standards that align with OWASP recommendations:
- Mandate input validation and sanitization using recommended libraries.
- Implement secure authentication and authorization mechanisms as outlined in the OWASP Authentication Cheat Sheet.
- Integrate security unit tests that check for parameter boundary conditions and proper output encoding.
Embed security checks into your CI/CD pipeline:
- Run API security scanners like OWASP ZAP as part of your build and testing process.
- Configure your pipeline to fail builds if high-severity vulnerabilities or OWASP Top 10 issues are detected.
Gap Opportunities and Enhancements in API Security#
As API security evolves, new methodologies, tools, and strategies are emerging to address changing threats. Organizations need to stay ahead of these developments to maintain strong API security and build secure API environments.
Emerging Trends in API Security#
Zero Trust Architecture is gaining momentum in API security. This approach goes beyond traditional perimeter-based security models by requiring verification for every request, regardless of origin.
Shift-Left Security is becoming standard in API development. Rather than treating security as an afterthought, this approach integrates security considerations early in the development process.
Leveraging Machine Learning and AI#
AI and Machine Learning are transforming API security practices, enhancing threat detection and response:
- Anomaly Detection: AI systems analyze API traffic patterns to identify unusual behavior that may indicate security threats.
- Automated Threat Response: Machine learning algorithms can automatically respond to certain security incidents, reducing the burden on security teams.
- Predictive Analysis: AI helps predict potential API vulnerabilities based on historical data and emerging threat patterns. For example, tools like RateMyOpenAPI uses a mix of AI and heuristics to identify security issues in your API specification and suggests fixes.
- Intelligent Rate Limiting: Machine learning dynamically adjusts rate limits based on user behavior and API usage patterns.
To stay current with these emerging trends and technologies, organizations should regularly review and update their API security strategies. The OWASP API Security Project provides valuable guidance on implementing these advanced security measures.
Strengthening Your API Security with OWASP Best Practices#
The OWASP REST Security Cheat Sheet offers vital guidance for securing APIs in today’s threat-heavy environment. Core practices like strong authentication, input validation, encryption, rate limiting, and monitoring help reduce exposure to common attacks.
Maintaining robust API security also requires ongoing effort: updating controls for new threats, integrating security into CI/CD pipelines, training development teams, and applying a defense-in-depth strategy in line with OWASP recommendations.
By adopting these best practices, organizations can build APIs that are secure, resilient, and capable of delivering outstanding digital experiences—while also protecting critical data and reducing the long-term costs of breaches.
Still, putting these practices into action can be challenging without the right tools. That’s where Zuplo comes in. Our API gateway helps you enforce OWASP-aligned protections—like authentication, rate limiting, and monitoring—right out of the box. Let Zuplo handle the security heavy lifting so your team can focus on building great products - try it for free today!