Input/output validation is your API's silent bodyguard—invisible when working properly, but catastrophic when absent. This critical process checks all data entering and leaving your system against expected formats, types, and business rules, forming the foundation of robust API security and performance.
Proper validation is essential for creating robust APIs that withstand real-world usage. Beyond security, validation ensures seamless communication between systems, prevents data corruption, and builds developer trust in your API ecosystem. In modern development workflows, validation integrates directly with your business logic, creating more maintainable systems that users actually want to work with.
Let's explore how proper validation can transform your API from a security liability into a rock-solid asset that delivers value for years to come.
- Your API's First Line of Defense: Why Validation Matters
- Building Rock-Solid Validation: Core Principles That Work
- Practical Validation: Real-World Implementation Approaches
- Guarding the Exit: Why Output Validation Matters
- Scaling Up: Advanced Validation for Complex Systems
- Protecting Your API Inside and Out: The Validation Journey
Your API's First Line of Defense: Why Validation Matters#
Input/output validation serves both as a critical security measure and a powerful performance optimizer in API development.
From a security standpoint, proper validation shields your API against common attack vectors:
- SQL injection: Preventing malicious SQL code from manipulating your database
- Cross-site scripting (XSS): Blocking attackers from injecting harmful client-side scripts
- Buffer overflows: Stopping excess data from overwhelming memory boundaries
Implementing effective API security measures is crucial to protect your system from such threats, and the real-world consequences of not doing so can be severe. In 2017, Equifax suffered a catastrophic breach exposing 147 million people's personal data due to improper input validation. Similarly, in 2019, Coinbase identified and fixed a vulnerability in their trading API that could have allowed attackers to steal funds by manipulating decimal values.
Performance benefits are equally compelling:
- Invalid requests get rejected before consuming valuable resources
- Backend services avoid reformatting improperly structured data
- Downstream systems receive only properly formatted data
- Server resources remain available for legitimate requests
Building Rock-Solid Validation: Core Principles That Work#
Schema Validation Fundamentals#
Schema validation ensures incoming data structures match expected patterns before processing. Using JSON Schema or XML Schema, you define exactly what your API expects:
{
"type": "object",
"properties": {
"username": { "type": "string", "minLength": 3, "maxLength": 20 },
"email": { "type": "string", "format": "email" },
"age": { "type": "integer", "minimum": 18 }
},
"required": ["username", "email"]
}
Implementing schema validation early in your request handling process helps reject malformed data before it reaches your business logic, thus protecting your API from bad inputs. In addition to schema validation, implementing proper API authentication methods ensures that only authorized users can access certain data.
Type Checking and Data Coercion#
Type checking verifies data matches expected formats, preventing issues like calculations on string data or inserting text into numeric database fields. When dealing with type mismatches, you can either reject the request with an appropriate error or attempt to coerce the data to the correct type:
try:
age = int(user_input)
if age < 0 or age > 120:
raise ValueError("Age out of valid range")
except ValueError as e:
print(f"Invalid age input: {e}")
Be cautious with automatic type coercion—explicit validation with clear error messages is generally safer.
Size and Range Validation#
Size and range validation prevent denial-of-service attacks and ensure data falls within acceptable boundaries by validating:
- String length (minimum and maximum)
- Array size (number of items)
- Numeric ranges (minimum and maximum values)
- File size for uploads
Setting appropriate limits protects your servers from resource exhaustion while preventing logical errors in your application.
Content Validation Strategies#
Content validation ensures actual data values are acceptable through pattern matching, format validation, and harmful content checks. Regular expressions are powerful tools:
^\[a-zA-Z0-9.\_%+-\]+@\[a-zA-Z0-9.-\]+\\.\[a-zA-Z\]{2,}$
When implementing content validation, choose between:
- Whitelisting (allowlisting): Define exactly what's permitted and reject everything else—the OWASP-recommended approach
- Blacklisting (denylisting): Block known bad inputs—generally less effective as attackers can often find workarounds
Contextual Validation#
Contextual validation applies business logic rules beyond syntax checking by considering relationships between different data points, such as:
- Ensuring start dates precede end dates
- Verifying sufficient funds for purchases
- Checking that database references point to existing records
- Validating business-specific constraints
While more complex than basic syntactic validation, contextual validation is essential for maintaining data integrity and preventing logical errors.
Practical Validation: Real-World Implementation Approaches#
Client-side vs. Server-side Validation#
These aren't competing approaches—they're complementary tools with different purposes:
Client-side validation:
- Provides instant feedback without server roundtrips
- Reduces server load by catching obvious errors immediately
- Creates responsive user experiences
- Cannot be relied upon for security, as attackers can bypass it
Server-side validation:
- Cannot be bypassed, making it your security foundation
- Protects against malicious requests regardless of source
- Validates data from any client, official or otherwise
Implementation Examples in Common Languages#
JavaScript/Node.js with Joi:
const Joi = require("joi");
// Define validation schema
const userSchema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
age: Joi.number().integer().min(18).max(120),
password: Joi.string().pattern(new RegExp("^[a-zA-Z0-9]{8,30}$")).required(),
});
// Validate data
function validateUser(userData) {
const { error, value } = userSchema.validate(userData);
if (error) {
throw new Error(`Validation error: ${error.details[0].message}`);
}
return value; // Validated data
}
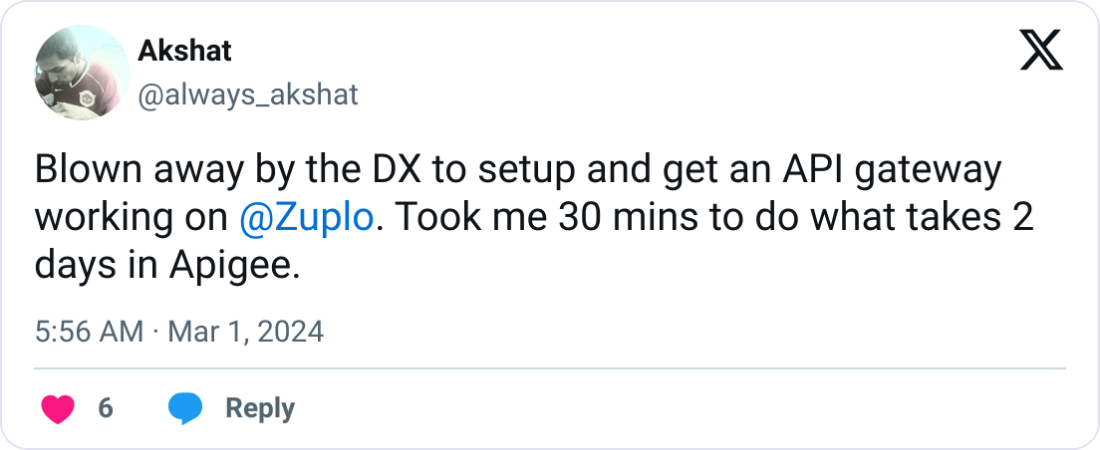
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreJava with Bean Validation API:
import javax.validation.constraints.*;
public class User {
@NotNull
@Size(min = 3, max = 30)
@Pattern(regexp = "^[a-zA-Z0-9]+$")
private String username;
@NotNull
@Email
private String email;
@Min(18)
@Max(120)
private int age;
@NotNull
@Size(min = 8, max = 30)
private String password;
// Getters and setters
}
// Using the validation
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import javax.validation.Validation;
import java.util.Set;
import javax.validation.ConstraintViolation;
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set<ConstraintViolation<User>> violations = validator.validate(user);
if (!violations.isEmpty()) {
// Handle validation errors
}
Python with Pydantic:
from pydantic import BaseModel, Field, EmailStr, validator
from typing import Optional
class User(BaseModel):
username: str = Field(..., min_length=3, max_length=30)
email: EmailStr
age: Optional[int] = Field(None, ge=18, le=120)
password: str
@validator('username')
def username_alphanumeric(cls, v):
if not v.isalnum():
raise ValueError('Must be alphanumeric')
return v
@validator('password')
def password_strength(cls, v):
if len(v) < 8:
raise ValueError('Password must be at least 8 characters')
return v
Handling Validation Failures Gracefully#
Your error handling determines whether developers love or hate your API:
- Use standardized error responses:
{
"status": "error",
"code": "VALIDATION_ERROR",
"message": "One or more fields failed validation",
"details": [{ "field": "email", "message": "Must be a valid email address" }]
}
- Never expose implementation details that could help attackers
- Log detailed errors internally but return cleaned-up messages to users
- Include field-specific errors so legitimate users can fix mistakes
- Use appropriate HTTP status codes (typically 400 Bad Request for validation errors)
Validation Middleware Patterns#
Implementing validation as middleware prevents code duplication across endpoints:
Express.js middleware example:
const validateUser = (req, res, next) => {
const { error } = userSchema.validate(req.body);
if (error) {
return res.status(400).json({
status: "error",
message: "Validation failed",
details: error.details.map((detail) => ({
field: detail.path[0],
message: detail.message,
})),
});
}
// If validation passes, continue to the route handler
next();
};
// Usage in routes
app.post("/users", validateUser, createUserHandler);
API Gateway validation pattern:
// Simplified API Gateway validation middleware
function validationMiddleware(schema) {
return async (req, res, next) => {
try {
// Apply schema validation based on route
const validatedData = await schema.validate(req.body, {
abortEarly: false,
});
// Replace request body with validated data
req.body = validatedData;
next();
} catch (error) {
res.status(400).json({
status: "error",
message: "Validation failed",
details: error.errors,
});
}
};
}
This pattern is invaluable for microservices architectures where consistent validation across services is critical. For more detailed guidance and API request validation best practices, explore our dedicated articles on this topic - including our guide to incoming body validation with JSON Schema. You can also watch this video to learn how to validate an API using your OpenAPI specification:
Guarding the Exit: Why Output Validation Matters#
Most developers focus on validating what enters their APIs while neglecting what leaves them—like installing a security system at the front door while leaving the back door wide open. Output validation completes your security equation.
Preventing Data Leakage#
Output validation prevents accidental exposure of sensitive information:
- API keys, user credentials, or internal metadata
- Debugging information revealing implementation details
- PII (Personally Identifiable Information) that could trigger regulatory issues
Implement data minimization by only returning necessary information:
// Before sending response, sanitize sensitive data
function sanitizeUserResponse(user) {
const { password, internalNotes, securityQuestions, ...safeUserData } = user;
return safeUserData;
}
Ensuring Response Consistency#
Consistent response formats serve as both a security feature and developer convenience by providing:
- Proper HTTP status codes accurately reflecting results
- Standardized error formats that don't reveal internal secrets
- Predictable data structures clients can reliably parse
Leveraging the Problem Details standard can help ensure consistency in error responses and improve developer experience.
For RESTful APIs, standardize on a consistent error response format:
{
"status": 400,
"message": "Invalid input provided",
"details": [{ "field": "email", "issue": "Format is invalid" }],
"requestId": "a1b2c3d4"
}
When dealing with partial updates to resources, utilizing techniques like JSON Merge Patch can help ensure response consistency and proper handling of data modifications.
Content-Type Enforcement#
Proper output validation enforces correct Content-Type headers to:
- Prevent MIME type confusion attacks
- Ensure browsers interpret data correctly
- Maintain proper character encoding
Always verify that response headers match the actual content being sent.
Implementation Examples for Response Validation#
Using JSON Schema for Response Validation:
const Ajv = require("ajv");
const ajv = new Ajv();
const responseSchema = {
type: "object",
properties: {
users: {
type: "array",
items: {
type: "object",
properties: {
id: { type: "string" },
name: { type: "string" },
email: { type: "string" },
},
required: ["id", "name", "email"],
additionalProperties: false,
},
},
count: { type: "integer" },
},
required: ["users", "count"],
additionalProperties: false,
};
app.get("/api/users", (req, res) => {
// Process request and prepare response
const responseData = {
users: userData,
count: userData.length,
};
// Validate response against schema
const valid = ajv.validate(responseSchema, responseData);
if (!valid) {
// Log the error internally but don't expose validation details to client
console.error("Response validation failed:", ajv.errors);
return res.status(500).json({ error: "Internal server error" });
}
// Response is valid, send it
res.json(responseData);
});
Output Encoding to Prevent XSS:
const escapeHtml = require("escape-html");
app.get("/api/comments", (req, res) => {
const comments = fetchComments();
// Encode HTML special characters in content that might contain user input
const safeComments = comments.map((comment) => ({
...comment,
content: escapeHtml(comment.content),
}));
res.json({ comments: safeComments });
});
This dual-validation approach significantly reduces security vulnerabilities and data leakage in your API ecosystem, protecting both systems and users.
Scaling Up: Advanced Validation for Complex Systems#
Basic validation works for simple APIs, but as your system grows, you need more sophisticated approaches to handle complex scenarios, microservices, and high-traffic environments.
Handling Nested Objects and Complex Data Structures#
For deeply nested objects, field-by-field validation is insufficient. JSON Schema enables validation rules that handle complex object graphs:
{
"type": "object",
"properties": {
"username": { "type": "string", "minLength": 3, "maxLength": 20 },
"email": { "type": "string", "format": "email" },
"age": { "type": "integer", "minimum": 18 },
"address": {
"type": "object",
"properties": {
"street": { "type": "string" },
"city": { "type": "string" },
"zipCode": { "type": "string", "pattern": "^[0-9]{5}$" }
},
"required": ["street", "city"]
}
},
"required": ["username", "email"]
}
This schema validates deep into nested objects, ensuring everything from top-level fields to deeply nested properties meets requirements. Validate early in your request lifecycle to prevent invalid data from infiltrating your system.
Validation in Microservices Architectures#
Microservices require a multi-layered validation approach:
- Gateway-level validation: Reject obviously malformed requests before they reach services
- Service-specific validation: Each microservice validates inputs based on domain-specific rules
- Contract testing: Use tools like Pact or Spring Cloud Contract to maintain validation consistency
Shared validation libraries help maintain consistency while allowing service-specific rules. Research shows implementing comprehensive validation across microservices can reduce API-related incidents by up to 30%.
Cross-Field and Conditional Validation#
Real-world business logic often requires validating relationships between fields or applying conditional validation:
interface User {
username: string;
email: string;
age: number;
role: "admin" | "user";
permissions?: string[];
}
function validateUser(user: User): ValidationResult {
const errors = [];
// Basic field validation
if (user.age < 18) errors.push("User must be at least 18 years old");
// Conditional validation based on role
if (
user.role === "admin" &&
(!user.permissions || user.permissions.length === 0)
) {
errors.push("Admin users must have at least one permission");
}
return { valid: errors.length === 0, errors };
}
For complex scenarios, consider using rule engines or expression languages to define validation based on business conditions.
Performance Optimization for High-Throughput APIs#
Robust validation actually improves performance—APIs with proper input validation show 24% lower average response times compared to those without, according to performance studies.
Optimization strategies include:
-
Compiled schemas: Precompile validation schemas instead of parsing them on every request
-
Progressive validation: Check critical fields first to reject invalid requests quickly
-
Rate limiting: Prevent validation from becoming a DoS attack vector:
const rateLimit = require("express-rate-limit");
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
});
app.use("/api/", apiLimiter);
Implementing API rate limiting is essential for maintaining performance and preventing abuse. Implementing rate limiting helps to manage request limits and prevent validation from becoming a DoS attack vector.
- Caching validation results: Store validation outcomes for frequently validated objects
A large-scale API gateway deployment found that implementing strict input validation reduced average response time by 18% and boosted throughput by 22%.
By implementing these advanced validation techniques, you'll build APIs that are more secure, reliable, and faster. Remember that validation isn't just about rejecting bad inputs—it's about creating a rock-solid foundation for your entire API ecosystem.
Protecting Your API Inside and Out: The Validation Journey#
Proper validation represents one of the highest-impact investments you can make in your API ecosystem. By implementing the techniques outlined in this guide, you'll create APIs that are not only more secure but also more reliable, performant, and developer-friendly. Remember that validation is an ongoing process—as threats evolve and your API grows, your validation strategies should mature alongside them.
Ready to transform your API validation approach? Zuplo provides the tools and infrastructure to implement comprehensive validation across your entire API ecosystem. Sign up for a free Zuplo account and see how proper validation can become your API's competitive advantage.