Your applications are only as good as their ability to handle errors gracefully. When things go sideways (and they will), how does your code respond? Mock APIs become your secret weapon in building robust applications that can withstand the chaos of the digital world.
These powerful tools let you simulate everything from basic HTTP errors to complete network meltdowns without risking your production systems. You can put your app through the wringer in a completely controlled environment, catching disasters before your users become unwitting testers.
This guide will help you master this approach so you can build applications that handle chaos with grace.
- Breaking Things on Purpose: The Art of Error Simulation
- Disaster Scenarios: Common API Failures to Prepare For
- Why Robust Error Handling Makes or Breaks Your App
- Blueprint for Error-Proof Apps: Implementation Guide
- The Best Tools for Breaking Things: Mocking Frameworks
- Leveling Up: Advanced Techniques for Error Resilience
- How Zuplo Takes Error Handling to the Next Level
- Error-Proof Your APIs Starting Today
Breaking Things on Purpose: The Art of Error Simulation#
Mock APIs are simulated interfaces that mimic the behavior of real APIs without connecting to actual backend systems. They provide predetermined responses to specific API requests, allowing developers to test their applications in a controlled environment. You can easily set up a mock API (even easier via OpenAPI) to facilitate this testing.
Think of mock APIs as stunt doubles for your real APIs. They look and act the part without any of the risks. They give you a sandbox where you can safely crash-test your application's error handling without taking down production systems.
Why Mock APIs Outshine Real APIs for Error Testing#
Real APIs make terrible testing partners when it comes to errors (try asking your production payment API to randomly return 500 errors!)
Mock APIs let you trigger specific error conditions repeatedly—something production APIs simply can't do. They operate without touching your actual data—no risk of corrupting databases during tests. They respond consistently every time—unlike real APIs with variable performance.
When Mock APIs Save Your Bacon#
Mock APIs really shine in a few key situations. They're super handy early on when your backend team is still hammering out the real APIs. Plus, they're a lifesaver in CI/CD pipelines where you need tests that run the same way every single time.
Trying to test those weird, edge-case errors that are a nightmare to recreate in your live environment? Mock APIs have got you covered. And if you're bringing new folks onto the team, mock APIs give them a safe space to play around with APIs without needing full access to everything right away.
Disaster Scenarios: Common API Failures to Prepare For#
When developing applications that interact with APIs, it's crucial to test how your code handles various error scenarios. Let's explore the most common API error scenarios you should consider simulating:
Server Errors (5xx Series)#
- 500 Internal Server Error - The classic "something broke but we're not telling you what" response
- 503 Service Unavailable - The digital equivalent of "sorry, we're closed for renovations"
- 504 Gateway Timeout - The server fell asleep on the job and didn't respond in time
Client Errors (4xx Series)#
- 400 Bad Request - Your app sent something the server couldn't understand
- 401 Unauthorized - The digital bouncer just checked your ID and said "nope"
- 403 Forbidden - The "you're not on the guest list" of API responses
- 404 Not Found - The digital equivalent of showing up to a party at the wrong address
- 431 Request Header Fields Too Large - When the server refuses to process the request because the headers are too large
Network Issues#
- Timeouts - Sometimes servers take forever to respond
- Connection Refused - The server straight-up rejected your connection attempt
- Partial Responses - Getting half a response is often worse than no response at all
Rate Limiting and Throttling#
- 429 Too Many Requests - The digital equivalent of "you're talking too fast, slow down!" It's important to understand how to manage request limits to prevent this error. To avoid hitting rate limits, it's essential to implement rate-limiting strategies in your application. Understanding API rate-limiting strategies can help both prevent and properly handle the 429 Too Many Requests errors.
Malformed Data Responses#
- Invalid JSON or XML - The response is syntactically broken and can't be parsed
- Schema Changes - The API changed what fields it returns without warning
Service Degradation#
- Slow Response Times - APIs that respond but take forever can frustrate users more than complete failures
Why Robust Error Handling Makes or Breaks Your App#
Using mock APIs to simulate error scenarios isn't just good practice—it's the difference between an application that crumbles under pressure and one that handles curveballs with grace. Here's why this approach is worth every minute you invest.
Improved Application Reliability#
Your application is only as reliable as its worst error handling. By methodically testing how your app responds to every flavor of failure and applying API rate limiting best practices, you can identify and fix problems before they impact users.
Enhanced Stability Through Controlled Testing#
Mock APIs give you complete control over when and how errors occur. This means you can reproduce issues exactly, debug them thoroughly, and verify your fixes actually work.
Expanded Testing Coverage#
Some error conditions are practically impossible to trigger with real APIs. Mock APIs let you create these nightmare scenarios safely, from network timeouts to bizarre edge cases like partial authorization success.
Streamlined Development Process#
Frontend developers aren't waiting for backend teams to finish endpoints. Quality assurance can perform end-to-end API testing for error scenarios without special environment setup. Everyone can work in parallel instead of being blocked by dependencies.
Cost-Effective Testing#
Every call to a third-party API costs something, whether it's direct billing, rate limit consumption, or infrastructure wear and tear. Mock APIs let you run thousands of tests without burning through API quotas or triggering usage alerts.
Improved Error Handling and User Experience#
Instead of generic "Something went wrong" messages, you can craft specific, helpful, structured error responses for each error scenario. Payment declined? Show relevant options to fix the issue. API timeout? Offer retry options with clear expectations.
Facilitated Continuous Integration and Automated Testing#
Mock APIs provide consistent, reproducible test environments that work the same way every time, making them perfect for CI/CD pipelines.
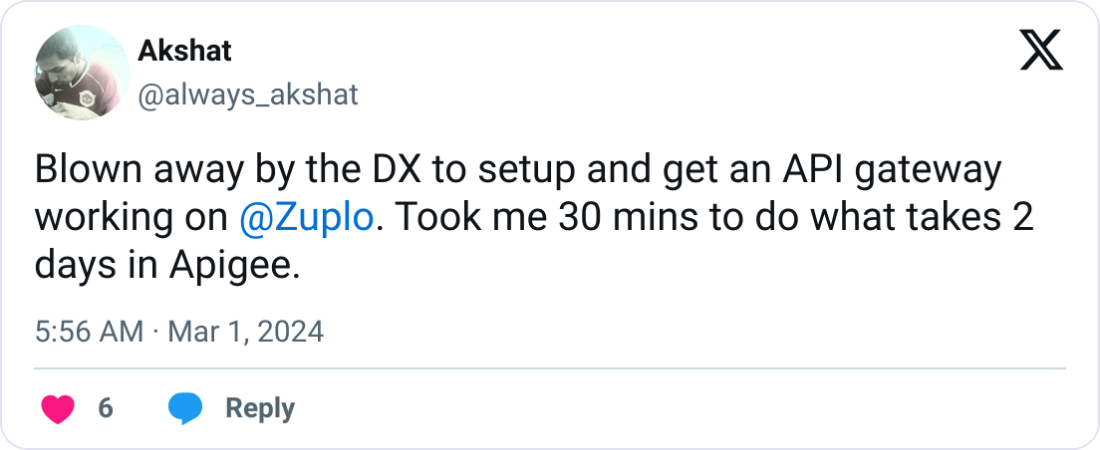
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreBlueprint for Error-Proof Apps: Implementation Guide#
Want to build apps that handle curveballs like a pro? Let's walk through setting up error simulations that will stress-test your application's resilience.
1. Identifying Critical API Touchpoints#
Start by identifying all API interactions in your system. Then prioritize them based on:
- Which ones support core user flows? (Think payment processing or authentication)
- Which endpoints see the heaviest traffic?
- Where would failures cause the biggest headaches?
2. Cataloging Possible Error Scenarios#
For each critical API touchpoint, brainstorm what could go wrong:
- What happens when the server is technically available but extremely slow?
- What if authorization succeeds but the subsequent data request fails?
- What if the API returns valid HTTP status but malformed response bodies?
Document these scenarios in a testing matrix to create a blueprint for comprehensive error testing.
3. Designing Appropriate Error Responses#
Understanding different API rate limit strategies can help you design appropriate error responses for these cases. Craft realistic error responses that match what you'd see in the wild. Here's what a rate limit error might look like:
{
"status": 429,
"headers": {
"Content-Type": "application/json",
"Retry-After": "60"
},
"body": {
"error": "Too Many Requests",
"message": "API rate limit exceeded. Please try again in 60 seconds.",
"code": "RATE_LIMIT_EXCEEDED"
}
}
4. Configuring Mock APIs#
Choose a tool that supports dynamic responses and error simulation, then:
- Configure endpoints to match your production API structure
- Set up rules to trigger specific errors based on conditions
- Add realistic latency to simulate network issues
Here's how you can use Zuplo's API gateway to easily set up a mock from your OpenAPI specification:
The advantage of using your API gateway for mocking is two-fold:
- You don't need to swap out your mock URLs during integration time.
- The performance of your mock will be closer to your prod performance.
If you'd prefer a dedicated mocking tool that's free and Open Source, Mockbin is a great option! Here's a little tutorial:
5. Writing Tests for Error Handling#
With your mock API configured, verify your application handles these errors gracefully. You can create unit tests by mocking to ensure your error-handling code works as expected:
- Create test cases for each error scenario in your matrix
- Execute the tests against your mock API
- Verify your application detects the error conditions
- Confirm appropriate user feedback is displayed
- Check that recovery mechanisms work as expected
Here's a simple test example using Jest and Axios:
test('handles rate limiting gracefully', async () => {
// Make 6 requests to trigger rate limiting
for (let i = 0; i < 6; i++) {
await api.getUsers();
}
// Attempt another request, expect rate limit error
await expect(api.getUsers()).rejects.toThrow('API rate limit exceeded');
// Verify the application shows appropriate user feedback
expect(ui.getErrorMessage()).toBe('Too many requests. Please try again later.');
});
6. Integrating with CI/CD Pipelines#
Make these tests part of your continuous integration pipeline:
- Add your mock API configuration to version control
- Configure your CI system to start the mock API during test runs
- Include your error handling tests in the test suite
- Set up appropriate failure thresholds and alerts
This integration ensures your error handling remains solid as your codebase evolves.
The Best Tools for Breaking Things: Mocking Frameworks#
Finding the right tools for API error simulation is like picking the perfect set of kitchen knives—the right ones make everything easier. Let's cut through the noise and look at what actually works, including options for rapid API mocking.
Popular Tools for API Error Simulation#
- Mockbin: The Swiss Army knife of API mocking with OpenAPI-powered mocking. Free and Open source with no signup required.
- Postman: A classic that refuses to go out of style with intuitive Mock Servers
- Mockoon: Offers godlike control over your mock APIs with desktop-based configuration
- WireMock: The battle-tested veteran with fine-grained control for complex error patterns
- Mockfly: Generate errors with AI-powered data that looks surprisingly realistic
Criteria for Choosing the Right Tool#
- Ease of use: Do you need to get up and running in minutes?
- Customizability: Need precise control over every header and response body?
- Collaboration features: Is your team distributed?
- Protocol and format support: Working with something beyond basic HTTP?
- Automation and CI/CD integration: Planning to run error tests automatically?
- Data realism: Want errors with believable test data?
- Scalability: Building something big?
- Security and isolation: Working with sensitive data?
- Cost: Working with budget constraints?
Leveling Up: Advanced Techniques for Error Resilience#
Basic try/catch blocks won't save you when things really go sideways. To build truly resilient applications, you need to level up your error-handling game.
Implementing the Circuit Breaker Pattern#
The Circuit Breaker pattern is like having a smart electrical panel for your API calls:
- Monitor the success/failure rate of API calls
- When failures exceed a threshold, open the circuit
- While open, fail fast without even attempting the call
- After a cooldown period, try a single test call
- If successful, close the circuit and resume normal operations
Libraries like Hystrix, Resilience4j, and Polly make this pattern easy to implement.
Using Retry Mechanisms with Exponential Backoff#
Not all errors are created equal. Sometimes, just trying again is the right move—but hammering an overloaded server with immediate retries is a recipe for disaster:
async function retryWithExponentialBackoff(operation, maxRetries = 5) {
let retries = 0;
while (retries < maxRetries) {
try {
return await operation();
} catch (error) {
if (retries === maxRetries - 1) throw error;
const delay = Math.pow(2, retries) * 100;
await new Promise(resolve => setTimeout(resolve, delay));
retries++;
}
}
}
Fallback Strategies for Graceful Degradation#
The best applications don't just fail—they degrade gracefully:
- Serving cached data when fresh data is unavailable
- Showing generic recommendations when personalization services fail
- Switching to simplified views that require fewer API dependencies
- Offering offline functionality that syncs when connectivity returns
Chaos Engineering Principles#
Netflix pioneered this approach with their infamous Chaos Monkey. The key is starting small:
- Define what "normal" looks like with clear metrics
- Create a hypothesis about what will happen during a failure
- Run controlled experiments that introduce realistic failures
- Analyze how your system responds and what breaks
- Fix the weaknesses you discover before they affect real users
Leveraging Event-Driven Architecture for Asynchronous Error Handling#
An event-driven approach lets you decouple error detection from handling:
- Process error events asynchronously
- Implement smart retry strategies for important operations
- Aggregate similar errors to detect patterns
- Trigger alerts only when certain thresholds are crossed
Advanced Logging and Monitoring for Error Detection#
You can't fix what you can't see:
- Structured logging with consistent formats and severity levels
- Correlation IDs that track requests across distributed systems
- Real-time alerting for unusual error patterns
- Dashboards that visualize error rates and patterns
- Error aggregation to group similar issues
How Zuplo Takes Error Handling to the Next Level#
Zuplo provides specialized features designed to make API error handling more straightforward and more effective. Here's how Zuplo can transform your error handling strategy:
Built-in Error Handling Policies#
Zuplo's policy-based approach lets you define reusable error handlers that can be applied consistently across your API endpoints:
- Create custom error responses with appropriate status codes and messages
- Define different error-handling strategies for different types of errors
- Apply policies at the route level or globally across your API
- Include correlation IDs automatically for better debugging
Error Response Normalization#
One of the biggest challenges in API error handling is maintaining consistent error formats. Zuplo solves this by:
- Normalizing error responses across your entire API
- Creating a standard error format that your consumers can rely on
- Transforming errors from upstream services into your standard format
- Hiding sensitive implementation details from error responses
Advanced Request Validation#
Zuplo's request validation helps catch errors before they even reach your backend:
- Validate request bodies against JSON schemas
- Automatically reject malformed requests with descriptive error messages
- Apply rate limiting to prevent abuse
- Custom validation logic for complex business rules
Comprehensive Error Analytics#
Understanding error patterns is critical for improving your API:
- Track error rates across all endpoints
- Identify the most common error types
- Monitor error trends over time
- Get alerts when error rates spike
Mock Response Generation#
Zuplo makes creating mock responses for testing incredibly simple:
- Define mock responses directly in your API configuration
- Create dynamic mocks that simulate different scenarios
- Schedule intermittent errors to test resilience
- Switch between mock and real backends with a configuration change
Error-Proof Your APIs Starting Today#
Error handling is like flossing. Everyone knows they should do it properly, but many skip it until something starts hurting. Simulating API error handling scenarios with mock APIs gives you the perfect opportunity to fix problems before they cause real pain for your users and midnight emergency calls for your team.
Ready to build APIs that fail gracefully and communicate clearly? Sign up for Zuplo today and transform how you handle API errors.