Authentication vulnerabilities in your APIs are like invisible cracks in a dam—they seem minor until they suddenly fail catastrophically. When authentication breaks down, attackers simply walk through your front door using stolen credentials or by exploiting impersonation flaws.
API security breaches don't just leak data—they compromise your entire digital ecosystem. There's a reason OWASP ranks API authentication vulnerabilities as the #2 risk in their OWASP API Security Top 10—the consequences are devastating, with each vulnerability creating ripple effects across connected services.
The good news? With proper implementation, these devastating yet preventable security failures can be avoided. Let's dive into why authentication vulnerabilities persist, how edge computing complicates security, and what concrete steps you can take to protect your APIs from becoming the next cautionary tale.
- When Good Authentication Goes Bad: Common API Vulnerabilities
- Living on the Edge: Authentication Challenges in Distributed Computing
- Building Your Defense: Authentication Best Practices
- Defensive Architecture: Building a Robust Authentication Program
- Authentication in the Microservices Era: When Your App Becomes a Neighborhood
- Securing the Gates of Your API Kingdom
When Good Authentication Goes Bad: Common API Vulnerabilities#
Authentication security forms the first line of defense for your API endpoints. When these mechanisms falter, attackers can easily compromise tokens or exploit implementation flaws to assume others' identities. Here's what leaves APIs most vulnerable:
Weak Authentication Mechanisms#
Most basic authentication schemes offer protection as effective as a paper lock on a bank vault:
- Basic Authentication Over Unencrypted Channels: Transmitting credentials over HTTP instead of HTTPS, especially when using Basic Authentication implementation, is practically inviting attackers to intercept them. This fundamental mistake remains surprisingly common, especially in internal APIs where developers mistakenly assume network isolation provides adequate protection. A packet sniffer can easily capture these credentials in plaintext, giving attackers immediate access to your systems.
// Vulnerable implementation
const express = require("express");
const app = express();
app.get("/api/user", (req, res) => {
const authHeader = req.headers.authorization;
// Basic auth with no TLS requirement
if (validateBasicAuth(authHeader)) {
return res.json({ data: "sensitive information" });
}
res.status(401).send("Unauthorized");
});
// Secure implementation
const express = require("express");
const app = express();
app.use((req, res, next) => {
if (!req.secure) {
return res.status(403).send("HTTPS required");
}
next();
});
- Absence of Rate Limiting: Without proper rate limiting, your authentication endpoints become sitting ducks for brute force attacks. The importance of rate limiting cannot be overstated—attackers can hammer your login API thousands of times per minute until they crack valid credentials. NoxPlayer discovered this the hard way when attackers hammered their update APIs to serve malware to users' devices. The absence of rate limiting also enables credential stuffing attacks, where attackers try massive lists of leaked username/password combinations against your API.
- Single-Factor Authentication for Sensitive Operations: Password-only authentication creates a single point of failure that's vulnerable to phishing, social engineering, and credential theft. Marriott learned this when attackers obtained login credentials from two employees and triggered a massive data breach that shocked the security community.
Token Troubles: Where Most APIs Fail#
Your token security is only as strong as its implementation—and most fall dangerously short:
- JWT Algorithm Vulnerabilities: Failing to validate algorithms or accepting the "none" algorithm creates critical security gaps. Apache Pulsar's bug allowed account takeovers because it skipped signature verification when the algorithm was set to "none", allowing complete account takeovers.
// Vulnerable implementation
function verifyToken(token) {
const decoded = jwt.decode(token);
if (decoded) {
return true; // Only decodes without verifying signature
}
return false;
}
// Secure implementation
function verifyToken(token) {
try {
const decoded = jwt.verify(token, secretKey, {
algorithms: ["HS256"], // Explicitly specify allowed algorithms
});
return true;
} catch (err) {
return false;
}
}
- Excessive Token Lifetimes: Some developers set token expiration to months or years for convenience, not realizing they're creating a massive security liability. Long-lived tokens continue granting access long after a breach occurs, giving attackers ample time to extract sensitive data or establish persistent backdoors in your systems.
- Improper Token Storage: Browser-based applications often store JWT tokens in vulnerable locations like localStorage, which is accessible to any JavaScript running on the page. This practice makes tokens easy targets for cross-site scripting (XSS) attacks.
- Missing Token Validation: Without comprehensive validation, tokens become easily forgeable credentials that bypass your security controls entirely. SOLARMAN learned this lesson painfully when their API skipped JWT signature verification, allowing attackers to forge tokens and take over accounts.
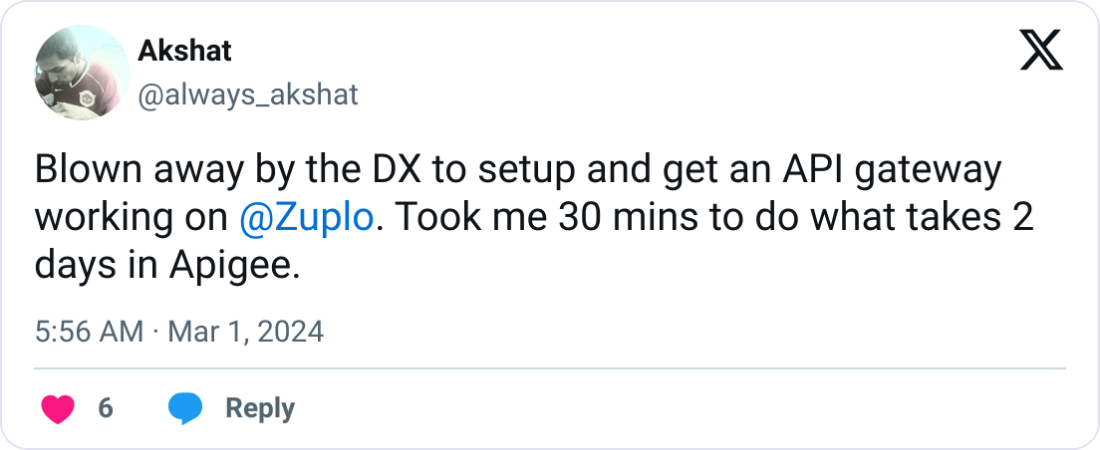
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreImplementation Flaws: The Devil in the Details#
Even well-designed authentication systems can be undermined by sloppy implementation:
- Insecure Password Reset Flows: Honda's e-commerce platform allowed password resets without proper verification—essentially letting anyone who knew your email change your password. Common flaws include emailing temporary passwords in plaintext, using easily guessable reset tokens, or failing to verify the reset request came from the legitimate user.
- Exposed Authentication Endpoints: Parler's breach exposed user data because their API simply didn't require authentication. Anyone could access and download sensitive information without even trying to hack it.
- Missing Authentication Checks: Even when authentication systems exist, developers sometimes forget to apply them consistently across all endpoints. Clubhouse leaked 1.3 million user records and LinkedIn had 700 million profiles scraped due to insufficient controls on their public APIs.
- Improper Session Management: Failing to invalidate sessions after logout or using predictable session IDs creates persistent vulnerabilities. Many applications only clear client-side session data during logout without invalidating the session on the server, allowing session hijacking attacks.
# Vulnerable implementation
@app.route('/logout')
def logout():
session.clear() # Just clears client-side session
return redirect(url_for('login'))
# Secure implementation
@app.route('/logout')
def logout():
session_id = request.cookies.get('session_id')
# Invalidate on server side
invalidate_session_in_database(session_id)
# Clear client side
session.clear()
response = redirect(url_for('login'))
response.delete_cookie('session_id')
return response
- Hard-coded Credentials: Embedding credentials in code is like writing your password on a sticky note attached to your monitor. This practice is particularly dangerous because hard-coded credentials often survive in source code repositories long after developers forget they exist. They frequently appear in configuration files, test scripts, or emergency "backdoor" access mechanisms that become permanent security liabilities.
Living on the Edge: Authentication Challenges in Distributed Computing#
Edge computing transforms the authentication landscape, creating unique security challenges traditional API deployments never faced when deploying APIs on edge networks. When your infrastructure is scattered across global edge nodes like digital confetti, securing APIs against broken authentication vulnerabilities becomes dramatically more complex.
Distributed Trust: The New Security Frontier#
Forget centralized security models—edge computing demolishes them entirely. We're dealing with outposts scattered worldwide, each requiring robust protection:
- Physical security vulnerabilities: Edge locations often exist in shared spaces with minimal physical safeguards, exposing hardware to potential tampering.
- Resource limitations: Many edge devices operate with minimal computing resources, making sophisticated authentication challenging.
- Scale and heterogeneity: Securing thousands of different devices running varied software versions across diverse networks creates exponential complexity.
Zero trust architecture becomes essential in these environments. "Never trust, always verify" isn't just a security mantra—it's a survival strategy for securing APIs against broken authentication vulnerabilities where traditional security perimeters have dissolved. Every device, user, and connection must prove itself continuously.
Speed vs. Security: Authentication in Low-Latency Environments#
How do you implement bulletproof authentication without adding latency that compromises performance? Balancing strong security with the need for optimizing API performance requires strategic approaches:
- Lightweight authentication protocols: JWTs shine here—they're compact, self-contained, and can be validated locally without central server queries.
- Local token validation: Push validation directly to edge nodes to eliminate round-trips to central servers that introduce latency.
- Strategic authentication caching: Carefully cache authentication results with robust protections around that cached data.
- Pre-authenticated secure channels: For time-critical operations, establish secure channels with pre-authentication.
Implementing techniques like BFF authentication can help secure APIs without compromising performance. The stakes are high—in autonomous vehicles, edge nodes need authentication decisions faster than humans can blink. A central validation server adding even 100ms latency could mean the difference between safety and disaster.
Global Harmony: Maintaining Security Consistency Across Edge Nodes#
Synchronizing security across a global network of edge nodes requires sophisticated approaches:
- Distributed trust architecture: Blockchain and distributed ledger technologies provide decentralized trust records that edge nodes can independently verify.
- Dynamic, context-aware access control: Static permissions fail in edge environments—your access control must adapt based on location, network conditions, and threat intelligence.
- Automated certificate management: Manual certificate handling across thousands of edge nodes is impossible—automation is essential for consistent security.
- Offline authentication capabilities: Edge nodes will inevitably lose connection to central services. Secure offline authentication must maintain security without central verification.
Implementing systems like federated gateways can help manage this complexity. Securing APIs against broken authentication vulnerabilities at the edge requires continuous monitoring, regular updates, and intelligent automation to manage complexity and evolving threats.
Building Your Defense: Authentication Best Practices#
Implementing robust authentication requires both technical controls and strategic planning. Here are some best practices for securing APIs to build an effective defense against authentication vulnerabilities:
Strong Authentication Foundations#
Start with these fundamental practices to strengthen your authentication security:
- Multi-factor authentication (MFA): Implement MFA for all sensitive operations and administrative access. This creates multiple layers attackers must breach to succeed.
- Proper password management: Enforce strong password policies and secure storage practices. Use bcrypt or Argon2 for password hashing—never store plaintext credentials.
- OAuth 2.0 and OpenID Connect: Leverage these established protocols rather than building custom authentication. They provide standardized, tested approaches to authorization and authentication.
- API keys with minimal permissions: Generate API keys with principle of least privilege—grant only the specific permissions each integration needs, nothing more.
- Certificate-based authentication: Implement mutual TLS (mTLS) for service-to-service communication to ensure both client and server verify each other's identity.
Token Security Done Right#
Proper token handling is critical for API security:
- Short-lived tokens with refresh capability: Keep access tokens short-lived (15-60 minutes) and implement secure refresh mechanisms. This limits the damage from token theft.
- Proper token storage: Store tokens securely—avoid local storage in browsers, use HTTP-only cookies with secure and SameSite flags for web applications.
- Token revocation mechanisms: Implement token blacklisting or immediate revocation capabilities for compromised accounts.
- Comprehensive token validation: Always validate token signature, expiration, issuer, and audience. Never accept tokens with the "none" algorithm.
- Token binding: Bind tokens to specific contexts (IP ranges, devices, or sessions) to prevent token replay across environments.
Implementation Security: Beyond the Basics#
Security details matter—these practices prevent common implementation flaws:
- Rate limiting and brute force protection: Implement API rate limiting, progressive delays, account lockouts, and CAPTCHA challenges to prevent credential stuffing and brute force attacks.
- Secure logout mechanisms: Properly invalidate sessions and tokens on both client and server sides during logout.
- Secure credential recovery: Implement secure password reset flows that require verification through a secondary channel before allowing changes.
- Session management best practices: Generate cryptographically secure session identifiers, implement appropriate timeouts, and refresh tokens securely.
- Authentication logging and monitoring: Track all authentication activities with detailed logging to detect suspicious patterns and potential breaches.
When updating your authentication systems, consider approaches that allow a zero-downtime migration, ensuring continuous availability during the transition.
Defensive Architecture: Building a Robust Authentication Program#
Creating comprehensive protection requires a structured approach to authentication security, guided by rigorous security and compliance policies.
Governance Framework: Setting the Rules#
Establish clear authentication policies and practices:
- Authentication policy hierarchy: Define acceptable protocols, token management practices, and authentication requirements based on risk levels.
- Security responsibility matrix: Clarify who's accountable for authentication security across your organization—developers, operations, security teams.
- Regular policy revision: Continually update your policies as threats evolve and new best practices emerge.
Measuring Success: Key Security Metrics#
Track meaningful metrics to gauge your authentication program's effectiveness:
- Authentication failure rates: Monitor for potential attacks through unusual failure patterns.
- Token lifecycle health: Ensure tokens aren't living beyond their intended lifespans.
- Authentication anomaly detection time: Measure how quickly you identify suspicious authentication patterns.
- MFA adoption rate: Track the percentage of your APIs implementing multi-factor authentication.
Maturity Roadmap: Evolving Your Authentication Security#
Follow this progression to advance your authentication capabilities:
- Foundation: Implement proper authentication with strong password policies and secure credential storage.
- Intermediate: Deploy OAuth 2.0/OpenID Connect, implement JWTs with proper validation, and add rate limiting.
- Advanced: Implement context-aware authentication, add adaptive MFA, and integrate security testing into CI/CD pipelines.
- Leading: Adopt Zero Trust architecture, implement advanced token validation at every layer, and deploy real-time monitoring with behavioral analytics.
Implementing these API security best practices will help you build a robust defense against authentication vulnerabilities. Take immediate steps to advance your authentication capabilities, regardless of your current maturity level. Effective security comes through continuous evolution—improving your defenses as both threats and authentication technologies advance.
Authentication in the Microservices Era: When Your App Becomes a Neighborhood#
Remember when your application was like a cozy house with a single front door? Those days are gone. Today's microservices landscape looks more like a bustling neighborhood with dozens of buildings, each with its own entrance and security system. This fundamental shift has completely transformed how we approach API authentication, including new challenges that we need to overcome.
The Microservices Authentication Puzzle#
Let's face it—when your app breaks into multiple independent services, your authentication strategy gets messy fast. Most teams discover this the hard way when they realize:
- Your Services Need to Trust Each Other: Imagine if every room in your house required a different key, and you needed to authenticate every time you moved from the kitchen to the living room. That's essentially what happens with service-to-service communication in microservices. Each internal request needs proper authentication, or you risk one compromised service becoming a skeleton key to your entire system.
- Session Management Gets Awkward: Traditional session cookies worked great when everything lived on one server. Now? Not so much. Sharing session info across services creates bottlenecks and dependencies that defeat the purpose of going with microservices in the first place.
- Credentials Start Multiplying Like Rabbits: Before you know it, you've got credentials scattered everywhere—database access here, third-party API keys there, internal service tokens everywhere else.
Making Authentication Work When Everything's Distributed#
Don't worry, it's not all doom and gloom. There are smart ways to tackle these challenges:
- Let Your Infrastructure Handle the Heavy Lifting: Service meshes like Istio and Linkerd are like hiring security guards for your neighborhood instead of making each homeowner figure out their own security system. They handle authentication, authorization, and encryption between services so your developers can focus on building features, not reinventing security.
- Pass the Identity Baton: Instead of making users re-authenticate at every service, implement identity propagation. JWTs work great here—they're like digital ID badges that carry just enough information about who someone is and what they're allowed to do as they move between services.
- Stop Hiding Keys Under the Doormat: Centralized secrets management platforms give you one secure place to store all those credentials, with automatic rotation and tight access controls. No more hardcoded secrets in config files or—even worse—committed to repositories. Tools like HashiCorp Vault are essentially your neighborhood's secure key locker.
- Trust No One, Not Even Yourself: Apply zero-trust principles even to internal communication. Every service interaction should require authentication, even between services that seem trustworthy. Think of it as friendly suspicion—even your most trusted neighbors should ring the doorbell rather than walking right in.
Securing the Gates of Your API Kingdom#
Authentication security is an ongoing commitment to protecting your API ecosystem, whether you're working with monolithic applications or complex microservices architectures. Start by addressing the fundamentals: implement strong authentication protocols, proper token handling, and rigorous validation. Then evolve toward a zero-trust model where every request is verified, regardless of source.
Most importantly, remember that authentication security isn't a destination but a journey requiring continuous improvement. By implementing the practices outlined in this guide, you'll significantly reduce your vulnerability to the most common and devastating API attacks.
Ready to transform your API security with powerful authentication protection? Zuplo's developer-friendly platform makes implementing these best practices straightforward with easy-to-deploy policies and comprehensive monitoring. Sign up for a free Zuplo account today and take the first step toward bulletproof API authentication.