SOAP (Simple Object Access Protocol) is a protocol for exchanging XML-based messages between systems. It's widely used in enterprise environments for secure, reliable communication. Here's a quick summary of its key aspects:
- Message Structure: SOAP messages follow a strict XML format with an Envelope, optional Header, Body, and Fault elements for error handling.
- WSDL: The Web Services Description Language (WSDL) defines the operations, messages, and bindings for SOAP APIs, simplifying development.
- Transport Protocols: SOAP works over HTTP, HTTPS, SMTP, and more, ensuring flexibility in communication.
- Security: Features like WS-Security make SOAP ideal for handling sensitive data and complex transactions.
- Use Cases: Commonly used in industries requiring high security, integration with legacy systems, and strict standards.
SOAP vs. REST: Quick Comparison#
Feature | SOAP | REST |
---|---|---|
Protocol Type | Protocol-based | Architectural style |
Message Format | XML only | XML, JSON, plain text |
Service Definition | WSDL | Swagger/OpenAPI |
Transport Protocol | HTTP, SMTP, TCP | Primarily HTTP |
Error Handling | Built-in fault elements | No built-in mechanism |
Security | WS-Security | Transport layer (e.g., HTTPS) |
State | Can be stateful | Stateless |
Coupling | High client-server | Minimal coupling |
SOAP is best for enterprise tasks requiring security, transactions, and legacy system integration, while REST is simpler and more lightweight.
How to Get Started#
- Define your WSDL to outline operations and messages.
- Build your SOAP API using tools or libraries like
soap
in Node.js. - Test your API with tools like SoapUI or Postman.
- Manage and optimize your SOAP API using platforms/gateways like Zuplo for security, performance, and monitoring.
SOAP remains a reliable choice for enterprise systems, especially when security and standardization are critical. Use the steps above to implement and manage your SOAP APIs effectively.
SOAP Structure and Components#
SOAP messages are built using structured XML elements, which include an Envelope, an optional Header, a Body, and occasionally a Fault element [3].
Message Structure#
A SOAP message is essentially an XML document. It has an Envelope, which
declares the default namespace (http://www.w3.org/2001/12/soap-envelope
). The
Header is optional and typically contains metadata like authentication
details, routing information, or processing instructions
[4].
The Body holds the main application data or, in cases of errors, includes a
Fault element
[3][4].
Here's an example of a basic SOAP message structure:
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<!-- Header (Optional): Contains metadata -->
<soap:Header>
<auth:Credentials xmlns:auth="http://example.org/auth">
<auth:Username>user123</auth:Username>
<auth:Password>pass456</auth:Password>
</auth:Credentials>
<tx:TransactionID xmlns:tx="http://example.org/transaction">
TX-12345
</tx:TransactionID>
</soap:Header>
<!-- Body: Contains the actual request/response data -->
<soap:Body>
<m:GetStockPrice xmlns:m="http://example.org/stock">
<m:StockName>ACME</m:StockName>
</m:GetStockPrice>
</soap:Body>
</soap:Envelope>
Here's how a SOAP error response (with Fault element) would look:
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2001/12/soap-envelope">
<soap:Body>
<soap:Fault>
<soap:Code>
<soap:Value>soap:Client</soap:Value>
</soap:Code>
<soap:Reason>
<soap:Text xml:lang="en">Invalid stock symbol</soap:Text>
</soap:Reason>
<soap:Detail>
<error:StockError xmlns:error="http://example.org/errors">
<error:Message>Stock symbol 'ACME' not found</error:Message>
<error:ErrorCode>E404</error:ErrorCode>
</error:StockError>
</soap:Detail>
</soap:Fault>
</soap:Body>
</soap:Envelope>
Each element serves a specific purpose in the SOAP communication process:
- Envelope: The root element that identifies the XML document as a SOAP message
- Header: Contains metadata like authentication, transaction information, or other processing instructions
- Body: Houses the primary request or response payload
- Fault: Appears in the Body when errors occur, providing standardized error reporting
WSDL Explained#
The Web Services Description Language (WSDL) is an XML-based schema that outlines the operations, messages, data types, bindings, and services for SOAP. It simplifies the development process by allowing automatic generation of client stubs and server skeletons [5].
Here's an example of a basic WSDL for a calculator service:
<?xml version="1.0" encoding="UTF-8"?>
<definitions name="CalculatorService"
targetNamespace="http://example.org/calculator"
xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.org/calculator"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<!-- Data types used by the service -->
<types>
<xsd:schema targetNamespace="http://example.org/calculator">
<xsd:element name="AddRequest">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="a" type="xsd:int"/>
<xsd:element name="b" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
<xsd:element name="AddResponse">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="result" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
</xsd:schema>
</types>
<!-- Messages for the service operations -->
<message name="AddInput">
<part name="parameters" element="tns:AddRequest"/>
</message>
<message name="AddOutput">
<part name="parameters" element="tns:AddResponse"/>
</message>
<!-- Port Type (Interface) -->
<portType name="CalculatorPortType">
<operation name="Add">
<input message="tns:AddInput"/>
<output message="tns:AddOutput"/>
</operation>
</portType>
<!-- Binding to SOAP protocol -->
<binding name="CalculatorSoapBinding" type="tns:CalculatorPortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="Add">
<soap:operation soapAction="http://example.org/calculator/Add"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<!-- Service definition -->
<service name="CalculatorService">
<port name="CalculatorPort" binding="tns:CalculatorSoapBinding">
<soap:address location="http://example.org/calculator"/>
</port>
</service>
</definitions>
This WSDL document defines five key components:
- Types: Defines data types using XML Schema
- Messages: Defines the data elements for each operation
- Port Type: Defines operations (like functions) and associated messages
- Binding: Specifies protocol details for operations
- Service: Specifies the service endpoint location
Client applications can import this WSDL to automatically generate code that knows how to communicate with the service, handling XML formatting and HTTP requests behind the scenes.
Protocols and Standards#
SOAP is a protocol established by the W3C for exchanging typed XML messages. It
often uses HTTP or HTTPS as its transport layer, with a Content-Type
of
text/xml; charset=utf-8
[1][4].
WSDL bindings can follow either document or RPC styles, which influence how the
Body is structured
[4].
WSDL Binding Styles: Document vs. RPC#
WSDL binding defines how SOAP messages are structured and how operations relate to the transport protocol. The two main binding styles are Document style and RPC (Remote Procedure Call) style, each with distinct characteristics and use cases.
Document Style Binding#
Document style binding treats the SOAP Body as an XML document without imposing a specific structure. This style is more flexible and is the preferred approach for most modern SOAP services.
<binding name="StockQuoteBinding" type="tns:StockQuotePortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetStockPrice">
<soap:operation soapAction="http://example.com/GetStockPrice"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
With document style:
- The SOAP body contains a complete XML document
- Better for complex data structures and validation
- More extensible and interoperable
- Typically paired with "literal" encoding for schema validation
RPC Style Binding#
RPC style binding structures the SOAP Body to resemble a function call with parameters, focusing on the operation name and parameters as distinct elements. This style was common in earlier SOAP implementations.
<binding name="StockQuoteBinding" type="tns:StockQuotePortType">
<soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetStockPrice">
<soap:operation soapAction="http://example.com/GetStockPrice"/>
<input>
<soap:body use="encoded" namespace="http://example.com/stockquote"
encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</input>
<output>
<soap:body use="encoded" namespace="http://example.com/stockquote"
encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/>
</output>
</operation>
</binding>
With RPC style:
- The operation name becomes a wrapper element in the SOAP body
- Parameters appear as child elements of the operation wrapper
- Often paired with "encoded" use for SOAP encoding rules
- More straightforward mapping to programming language method calls
- Less flexible for complex XML structures
Most modern SOAP services use document/literal style for better interoperability and XML Schema validation capabilities, while RPC style remains in some legacy systems where procedure call semantics are important.
Security with WS-Security#
WS-Security (Web Services Security) is an extension to SOAP that provides end-to-end message-level security, addressing three key aspects:
- Authentication: Verifies the identity of the message sender using security tokens
- Integrity: Ensures messages haven't been tampered with using XML Signature
- Confidentiality: Protects sensitive information using XML Encryption
A basic WS-Security SOAP header looks like this:
<soap:Header>
<wsse:Security xmlns:wsse="http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd">
<!-- Username token authentication -->
<wsse:UsernameToken>
<wsse:Username>johndoe</wsse:Username>
<wsse:Password Type="...#PasswordDigest">base64EncodedDigest</wsse:Password>
<wsse:Nonce>base64EncodedNonce</wsse:Nonce>
<wsu:Created>2023-04-24T11:42:00Z</wsu:Created>
</wsse:UsernameToken>
<!-- Digital signature -->
<ds:Signature xmlns:ds="http://www.w3.org/2000/09/xmldsig#">
<!-- Signature content -->
</ds:Signature>
</wsse:Security>
</soap:Header>
WS-Security is more comprehensive than REST's transport-level security (HTTPS), making it suitable for enterprise environments with strict security requirements.
With these components in mind, the next step is to compare SOAP with REST to help you decide which approach fits your needs.
SOAP and REST Differences#
Knowing the differences between SOAP and REST helps developers pick the right approach for their project needs.
Message Formats#
SOAP strictly uses XML, which can increase payload size. REST, on the other hand, works with XML, JSON, and plain text, with JSON being the lightweight and commonly used choice [6].
Use Cases#
SOAP is better suited for:
- Enterprise-level tasks: It supports WS-Security, guaranteed delivery, and ACID transactions [7].
- Integrating with older SOAP systems [8].
Technical Comparison#
Feature | SOAP | REST |
---|---|---|
Protocol Type | Protocol-based | Architectural style |
Message Format | XML only | Multiple formats (e.g., JSON, XML) |
Service Definition | WSDL | Swagger/OpenAPI |
Transport Protocol | HTTP, SMTP, TCP | Primarily HTTP |
Error Handling | Built-in fault elements | No built-in mechanism |
Security | WS-Security | Transport layer (e.g., HTTPS) |
State | Can be stateful | Stateless |
Coupling | High client-server | Minimal coupling |
This table underscores SOAP's strength in enterprise settings, offering features like advanced security and error handling, while REST stands out for its simplicity and adaptability. The decision between the two largely depends on your project's requirements for security, transaction management, and system integration. Up next, we'll dive into building and testing SOAP APIs.
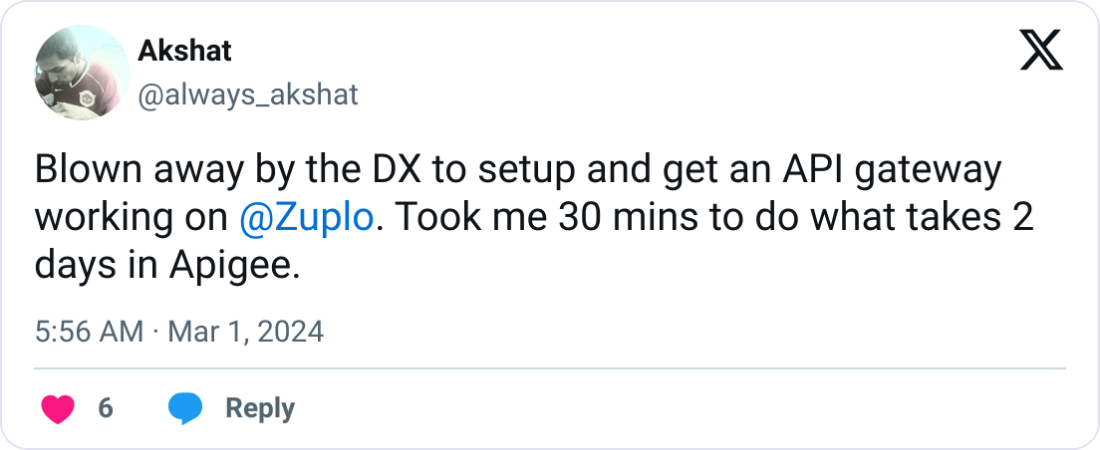
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreBuilding and Testing SOAP APIs#
Now that we’ve covered SOAP’s structure and how it compares to REST, let’s dive into implementing and testing a SOAP API.
Steps to Implement a SOAP API#
- Define the WSDL: Specify operations, messages, data types, and bindings. (Check out the 'WSDL Explained' section for more details on schema.)
- Handle XML: Parse and serialize XML while exposing HTTP endpoints.
- Service Operations: Create service operations with well-defined inputs and structured responses.
Tools for Testing#
We have a full guide to SOAP API testing but the tl;dr is tools like SoapUI, Step CI, or Postman are great for testing SOAP APIs. They let you import WSDL files, view available operations, and generate requests for different scenarios.
Automating Integration#
You can use libraries in various programming languages to handle WSDL parsing
and XML processing. For example, in Node.js, the soap
package simplifies SOAP
API integration:
const soap = require("soap");
const url = "http://example.com/calculator?wsdl";
soap.createClient(url, function (err, client) {
client.Add({ num1: 5, num2: 3 }, function (err, result) {
console.log("Result:", result);
});
});
FAQs#
What are the key benefits of using SOAP APIs for enterprise applications compared to REST APIs?#
SOAP APIs offer several key advantages for enterprise applications, especially when it comes to security, reliability, and standardization. SOAP includes robust security features like WS-Security, which supports authentication, encryption, and message integrity, making it ideal for handling sensitive data.
Another benefit is SOAP's reliable messaging capabilities, with built-in error handling and retry mechanisms that ensure messages are delivered even in unstable network conditions. Additionally, SOAP uses WSDL (Web Services Description Language) to define strict service contracts, which enhances interoperability across different platforms and programming languages.
These features make SOAP a strong choice for industries like finance, healthcare, and government, where secure and reliable communication is critical.
What steps can I take to secure my SOAP API when working with sensitive data?#
To protect your SOAP API when handling sensitive data, start by implementing WS Security, which provides standards for authentication, encryption, and message integrity. This includes using digital signatures, XML encryption to safeguard data, and X.509 certificates for secure identification. You can also include security credentials, such as usernames and passwords, in the SOAP header for added protection.
Additionally, adopt best practices like regular security testing, input validation to prevent malicious data, and monitoring API requests for unusual activity. Implementing identity and access management (IAM) ensures only authorized users can access your API, further enhancing its security.
What are the best tools for testing and managing a SOAP API during development?#
To effectively test and manage your SOAP API, consider using SoapUI, a widely-used open-source tool designed specifically for API testing. For more advanced needs, such as load testing and API virtualization, you can explore ReadyAPI, which offers additional features tailored for professional development workflows.
Both tools are highly regarded for their ability to simplify SOAP API testing and integration, making them excellent choices for developers aiming to streamline their processes. Also consider StepCI as a free and open-source solution that handles multiple protocol,s including SOAP.