Setting up service discovery for APIs is the secret sauce that makes your APIs talk to each other without awkward introductions. Think of it as the ultimate matchmaker for your services, connecting them automatically so they can scale and stay reliable even when your system gets as complicated as a season finale of your favorite drama series.
Why should you care? Because hardcoding service locations is like tattooing your friend's address on your arm—it works great until they move! With service discovery, your services find each other dynamically, which is absolutely critical when instances come and go due to scaling, updates, or when things inevitably break.
The numbers don't lie either: according to a recent survey by O'Reilly, 77% of organizations have adopted microservices, with 92% experiencing success with this architectural style. In this guide, we'll dive into everything from fundamentals to implementation strategies, and show you how API gateways can supercharge your discovery capabilities. Let's get started!
Service Discovery Basics: Your Microservices' Social Network#
Service discovery is like having a constantly updated phonebook for your microservices—but one that actually works, unlike those paper doorstops we used to get. It lets your services find and communicate with each other automatically without needing fixed addresses. This dynamic connection is absolutely essential when juggling dozens or hundreds of microservices in cloud environments where the only constant is change.
The core components aren't complicated, but they're powerful as hell:
Service Registry#
Think of this as the brain of your discovery system—a central database that knows what services exist and where to find them. It's constantly updating as services come and go.
Service Registration#
This is how services announce, "I'm here and ready to work!" They register themselves with details about their location, health status, and what they offer.
Health Checking#
No zombie services allowed! Regular health checks ensure every service in the registry is actually alive and capable of handling requests.
Service Lookup#
When one service needs another, this is how it finds it—by asking the registry "Where can I find the payment service right now?"
Service discovery directly solves problems that would otherwise make your developers pull their hair out:
- Moving targets? No problem. Cloud services love to change IP addresses like teenagers change social media profiles.
- Scaling up or down? Handled automatically. As services multiply to handle load or shrink to save costs, service discovery keeps the directory updated.
- Managing multiple versions? You bet. When running different versions simultaneously, discovery helps route traffic appropriately.
For the business folks, this translates directly to better reliability and fault tolerance. When services inevitably hiccup (and they will), service discovery reroutes traffic away from problems, helping maintain operations even when individual components fail.
Client vs. Server: Choosing Your Discovery Superhero#
When setting up service discovery for your APIs, you've got two main paths to choose from: client-side discovery and server-side discovery. Each approach has its own superpowers and kryptonite that'll impact how your microservices communicate.
Client-Side Discovery#
With client-side discovery, the client does all the heavy lifting—and we mean ALL of it. Here's the play-by-play:
- Your client app asks the service registry: "Hey, where can I find the user service?"
- The registry responds with all available instances.
- The client picks one (using its own load-balancing smarts).
- The client makes the request directly to that service instance.
Advantages:
- The client talks straight to the service with no middlemen, creating fewer network hops and potentially faster responses.
- You get granular control over load balancing. Want to prioritize services in the same data center? Implement your own algorithm!
- When things go sideways, clients can detect failures immediately and try alternative instances.
Downsides:
- Your client code gets bloated with discovery logic—what happened to separation of concerns?
- Clients become tightly coupled to your service registry implementation.
- Different clients might implement discovery differently, creating inconsistent behavior.
Server-Side Discovery#
With server-side discovery, you're bringing in a traffic cop—a router or load balancer that handles all the discovery heavy lifting:
- Your client makes a request to a fixed endpoint (the router).
- The router checks the service registry for available instances.
- The router picks one and forwards the request.
- The response travels back through the router to the client.
Advantages:
- Client code stays blissfully simple—it doesn't need to know anything about service discovery.
- You maintain centralized control over routing and load balancing policies across all services.
- Behavior stays consistent across different client languages and frameworks.
Trade-Offs:
- That extra network hop through the router might impact latency.
- Your router could become a bottleneck if not properly scaled.
- Your infrastructure setup gets more complex with another critical component to maintain.
When choosing between these approaches, consider your microservices ecosystem complexity, client technology diversity, team experience, and latency sensitivity. Don't feel locked into one approach—many mature architectures use both in different parts of the system.
Tech Titans: The Big Three Discovery Tools You Should Know#
Let's cut through the noise and look at three powerhouse technologies that dominate API service discovery: Consul, Eureka, and Kubernetes. Each brings something special to the table—here's what you need to know.
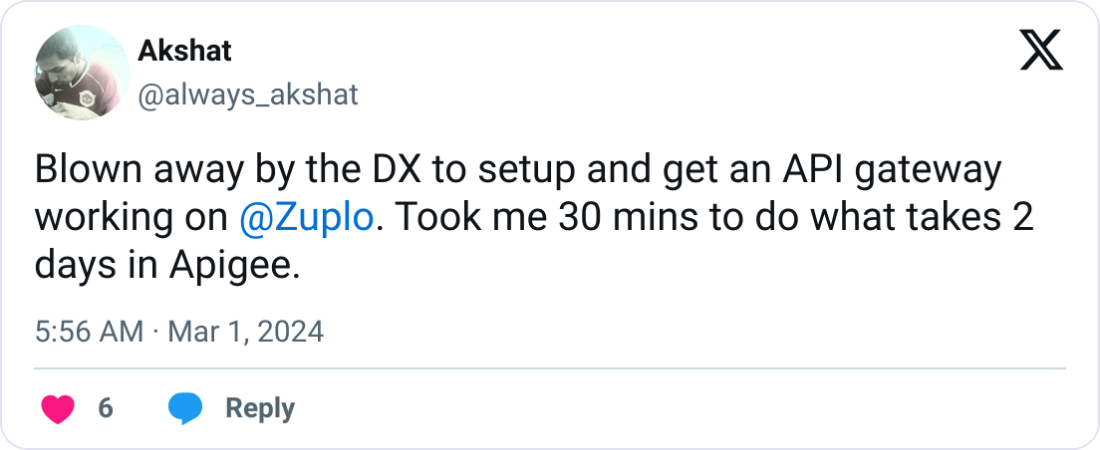
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreConsul#
Consul, HashiCorp's distributed system tool, isn't just a one-trick pony for service discovery—it's a Swiss Army knife that also handles configuration management and network segmentation.
What makes Consul shine:
- Rock-solid distributed service registry that scales like a boss.
- Comprehensive health checking that catches problems before users do.
- Built-in key-value store for configuration.
- Multi-datacenter support for global applications.
Here's how Consul tackles service discovery:
- Services register themselves with the local Consul agent.
- Consul servers maintain a consistent catalog of all available services.
- Clients query Consul when they need to find healthy instances.
Want to see Consul in action? Here's what registering a service looks like:
{
"service": {
"name": "web",
"port": 80,
"check": {
"http": "http://localhost/health",
"interval": "10s"
}
}
}
Consul shines brightest in large, complex environments where its feature richness and scalability really pay off.
Eureka#
Eureka takes a simpler approach to service discovery, focusing on REST-based service registration and discovery specifically tailored for AWS environments. It's particularly well-suited for Java microservices and the Spring ecosystem.
What makes Eureka worth your attention:
- Client-side load balancing that reduces latency.
- Fault tolerance built into its DNA (it was designed to survive AWS zone failures).
- Seamless integration with other Netflix tools like Ribbon and Hystrix.
Here's how dead simple registering a service with Eureka can be in Spring Boot:
@SpringBootApplication
@EnableEurekaClient
public class MyServiceApplication {
public static void main(String[] args) {
SpringApplication.run(MyServiceApplication.class, args);
}
}
Eureka particularly shines in Spring Boot applications where its straightforward approach and tight Java integration make it a natural fit.
Kubernetes#
Kubernetes isn't just for container orchestration—it packs a powerful built-in service discovery system that's changing how developers think about service connectivity. It uses DNS under the hood, along with environment variables, to help services find each other.
What makes Kubernetes service discovery so damn good:
- Zero-effort service registration—just deploy your stuff and it works.
- Integrated load balancing that distributes traffic intelligently.
- Health checking that prevents traffic from hitting dead pods.
- DNS-based discovery that feels natural to developers.
Kubernetes uses Services to create stable endpoints for groups of Pods. Here's a basic Service definition:
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: MyApp
ports:
- protocol: TCP
port: 80
targetPort: 9376
This creates a DNS entry for my-service
that automatically points to your
matching pods. Magic!
When comparing these technologies, consider scalability needs, fault tolerance approaches, and integration with your existing stack. Your choice often comes down to your existing tech stack, team skills, and specific requirements. There's no universal "best"—just the right tool for your particular situation.
API Gateways: The Ultimate Discovery Upgrade#
API gateways absolutely transform service discovery in microservices architectures. They're not just traffic cops—they're the air traffic controllers of your API ecosystem, intelligently managing all inbound requests. By sitting between clients and your backend services, API gateways simplify setting up service discovery while adding a ton of value.
Traffic Management on Steroids#
When it comes to traffic handling, API gateways don't just play the game—they dominate it with capabilities like:
- Dynamic routing that adapts to service health and availability in real-time.
- Intelligent load balancing that distributes requests to maximize performance.
- Request transformation that keeps clients and services compatible even as your APIs evolve.
Embracing federated gateways can accelerate developer productivity by enabling teams to manage their own gateways independently while still adhering to organizational policies.
Security and Access Control#
API gateways put security on steroids by centralizing crucial protections:
- Unified authentication and authorization that works across all your services.
- Comprehensive API key management that tracks who's using what.
- Rate limiting that prevents bad actors from bringing your system to its knees.
These security features ensure only authorized clients can discover and use your services, dramatically reducing your attack surface. Implementing robust RBAC analytics helps in monitoring and fine-tuning access controls across your microservices.
Programmable Service Discovery Logic#
Modern API gateways like Zuplo take service discovery to the next level by making it programmable:
- Write complex routing rules based on request properties, headers, or payloads.
- Integrate with any service registry through custom code.
- Update routing logic on the fly without downtime.
Zuplo's TypeScript-based approach means developers can implement custom service discovery logic that perfectly matches their architecture's needs. For organizations looking to monetize AI models or delve into ecommerce API monetization, programmable gateways provide the flexibility to adapt to rapidly changing markets.
Edge Execution for Improved Performance#
Want to blow your users' minds with API performance? API gateways that support edge execution, like Zuplo with its network of 300+ global data centers, make this possible:
- Process requests closer to your users, slashing latency.
- Make service discovery decisions at the edge, eliminating network delays.
- Efficiently manage global traffic patterns without complex infrastructure.
For businesses aiming to promote and market an API or explore strategic API monetization, leveraging the capabilities of a hosted API gateway offers significant advantages in scalability and ease of use.
Discovery Done Right: Best Practices You Can Count On#
Nailing service discovery isn't just nice-to-have—it's critical for managing APIs in microservices architectures. We've learned (sometimes the hard way) that following these best practices creates reliable, scalable service interactions while keeping your API infrastructure running like a well-oiled machine.
Health Checks That Actually Work#
Your service registry is only as good as its data is accurate. Keep it trustworthy with aggressive health monitoring:
- Run health checks frequently—every 10-30 seconds is the sweet spot.
- Set reasonable timeouts that account for normal performance variations without triggering false alarms.
- Implement circuit breakers that temporarily remove unhealthy services and gradually test them before bringing them back fully.
Consul shines here with its configurable health check intervals, timeouts, and failure thresholds. Want your service registry to be reliable as hell? Don't skimp on health checks.
Configuration That Doesn't Lead to Chaos#
Your service discovery infrastructure needs consistent configuration to avoid chaos:
- Store configurations centrally using purpose-built tools like etcd or Consul's Key-Value store.
- Keep configurations in version control with Git or similar tools so you can track changes and roll back when needed.
- Automate configuration updates through CI/CD pipelines to eliminate human error.
Security That Doesn't Suck#
Let's be blunt: an insecure service discovery system is a massive liability. Lock it down tight:
- Encrypt all communication between services and the registry using TLS—no exceptions.
- Implement role-based access control (RBAC) so only authorized services can register or query the registry.
- Automate certificate management with tools like cert-manager in Kubernetes environments to eliminate expired cert disasters.
For performance optimization that keeps your discovery system snappy:
- Cache frequently accessed service information in memory to reduce constant registry lookups.
- Consider a distributed registry architecture for large-scale deployments to improve fault tolerance.
- Use efficient data structures for service lookups—consistent hashing works wonders for load balancing.
Automated Recovery Strategies#
When services go down—as they inevitably will—having automatic recovery mechanisms separates the pros from the amateurs. Don't leave your system hanging when failures happen:
- Implement graceful degradation patterns so your API ecosystem continues functioning even when some services are unavailable.
- Set up retry policies with exponential backoff to handle temporary failures without overwhelming recovering services.
- Create self-healing processes that automatically restart failed services instead of waiting for manual intervention.
- Testing recovery scenarios regularly ensures your automation actually works when real disasters strike. Mock service failures during off-peak hours to verify your discovery system correctly reroutes traffic and implements fallbacks as designed.
Documentation That Doesn't Gather Digital Dust#
Service discovery setups love to become mysterious black boxes nobody understands six months later. Keep your system approachable with living documentation:
- Maintain visual service maps showing dependencies and communication paths that update automatically as your architecture evolves.
- Document discovery patterns and decisions in a centralized knowledge base accessible to all team members.
- Create onboarding materials explaining your service discovery implementation so new team members can get productive quickly.
- Consider tools like Zudoku for automatically generating and maintaining API documentation, combined with discovery metadata that shows which instances are currently available. This transparency prevents the "it works but nobody knows why" syndrome that plagues many microservices implementations.
Power Up Your API Infrastructure#
Service discovery isn't just plumbing—it's the backbone that makes your microservices architecture actually work in the real world. By automating how services find and connect with each other, you can build systems that scale dynamically while maintaining rock-solid reliability. The benefits speak for themselves: dramatically reduced manual configuration, more efficient API infrastructure, and better business outcomes through high availability and fault tolerance.
Ready to level up your microservices game? Zuplo's code-first approach to API management takes service discovery to the next level with its programmable gateway. By integrating with various discovery systems and adding capabilities like advanced security and sophisticated routing, Zuplo supercharges your API ecosystem. Book a meeting with us today and learn how to dominate the microservices world with service discovery done right.💪