Poor error handling is like giving users a cryptic puzzle with no solution. They get frustrated, abandon your platform, and your security takes a hit when overly detailed messages leak sensitive info. A code-first approach puts the power back where it belongs—in developers' hands—letting your teams evolve their error handling alongside the API itself.
Ready to optimize API error handling and response codes? Let's explore the best practices that will keep your users happy, your system secure, and your support team sane.
- Why Great Error Handling Is Your Secret Weapon
- Server-Side Error Handling That Doesn't Suck
- Keeping Your API Secure While Being Helpful
- Client-Side Strategies That Keep Users Happy
- Creating Error Experiences Users Don't Hate
- Tailoring Your Approach to Different API Architectures
- From Reactive to Predictive: The Future of API Error Handling
- Turn Your API Errors Into Opportunities
Why Great Error Handling Is Your Secret Weapon#
When it comes to APIs, it's not just about how they perform when everything works perfectly. It's about how gracefully they fail when things go wrong. Whether you're dealing with a simple MySQL REST API integration or a complex microservices architecture, great error handling is your secret weapon for building resilient, secure, and developer-friendly APIs.
Expected vs. Unexpected Errors#
When dealing with API errors, you'll typically encounter two main types:
- Expected errors: These are foreseeable issues, like validation failures (e.g., a user submits an invalid email). They’re part of normal operation and should be handled gracefully by providing clear, actionable feedback to clients
- Unexpected errors: These are unplanned disruptions like server crashes, dropped database connections, or weird edge cases (aka the nasty surprises). While harder to predict, great error handling ensures these don’t cascade into larger failures or expose sensitive information.
HTTP Status Codes: Your First Line of Communication#
HTTP status codes are the universal language between APIs and clients, and they instantly tell a story at a glance:
- 1xx codes: Informational ("I got your message, I'm working on it.")
- 2xx codes: Success ("Everything worked perfectly!")
- 3xx codes: Redirection ("Not here, try over there.")
- 4xx codes: Client errors ("It's not me, it's you,” like 404 Not Found or issues requiring HTTP 431 error handling.)
- 5xx codes: Server errors ("We messed up.")
Using precise status codes is critical. Returning a generic 500 for every error confuses clients and makes troubleshooting harder. Always pick the most specific code that fits the situation.
Common HTTP Status Codes You'll Encounter#
These are the codes you'll see most frequently:
- 200 OK: Everything's awesome! Your request succeeded.
- 201 Created: Success! We made something new as requested.
- 400 Bad Request: Your request makes no sense. Fix it and try again.
- 401 Unauthorized: Who are you? Authenticate before proceeding.
- 403 Forbidden: We know who you are, but you can't do that!
- 404 Not Found: That thing you're looking for isn't here.
- 429 Too Many Requests: Slow down! You're hammering our API too hard. (This status indicates that API rate limiting is in effect.)
- 500 Internal Server Error: Something broke on our end. We're sorry.
Impact on Developer Experience#
Good error handling dramatically improves the developer experience. Compare these two responses:
Useless response:
{
"error": "Internal Server Error"
}
Helpful response:
{
"error": {
"code": "DATABASE_CONNECTION_FAILED",
"message": "Unable to connect to the database. Please try again later.",
"timestamp": "2023-05-15T14:30:00Z",
"requestId": "abc123"
}
}
The second example gives developers actual information they can use to troubleshoot and resolve the issue.
Programmable Gateways for Custom Error Handling#
Modern API management platforms let you write code that controls exactly how errors behave, highlighting the benefits of a hosted API gateway:
- Flexibility to tailor error responses exactly to your needs
- Consistency across all your services
- Security by stripping sensitive info before it reaches clients
- Debugging with context that helps solve problems
Server-Side Error Handling That Doesn't Suck#
When it comes to API development, optimizing server-side error handling is crucial for maintaining system reliability and providing a positive developer experience. Whether you're integrating databases with APIs to create a Supabase-like developer experience or building complex services, let's explore how to create APIs that fail gracefully.
Creating Standardized Error Responses#
Use a consistent, machine-readable format (e.g., fields like `code`, `message`, `timestamp`, `requestId`). This enables clients to programmatically handle errors and makes debugging easier. Inconsistent error formats make debugging feel like solving a mystery with different clues in every room. A standardized format should include:
- A unique error code that actually means something
- A human-readable message that doesn't require a PhD to understand
- Detailed information about what went wrong
- Links to documentation where developers can learn more
Adopting the Problem Details in HTTP responses format can further enhance the clarity and consistency of your error responses.
Here's what a proper error response should look like:
{
"error": {
"code": "validation_error",
"message": "The request payload is invalid",
"details": [
{
"field": "email",
"issue": "Invalid email format"
}
],
"documentation_url": "https://api.example.com/docs/errors/validation_error"
}
}
Balancing Security and Helpfulness#
The security tightrope is challenging. Lean too far either way and you're in trouble. Your error messages need to help developers without giving hackers a roadmap to your vulnerabilities. Following API security best practices ensures that your error handling doesn't compromise your system's integrity:
- Never expose system internals—keep stack traces, implementation details, and system specifics hidden in production
- Be vague about authentication failures to prevent attackers from narrowing down their approach
- Log detailed information server-side where only your team can see it
- Provide clear, actionable error messages that help developers resolve issues without leaking sensitive details
- Localize error messages to improve accessibility for non-English-speaking developers
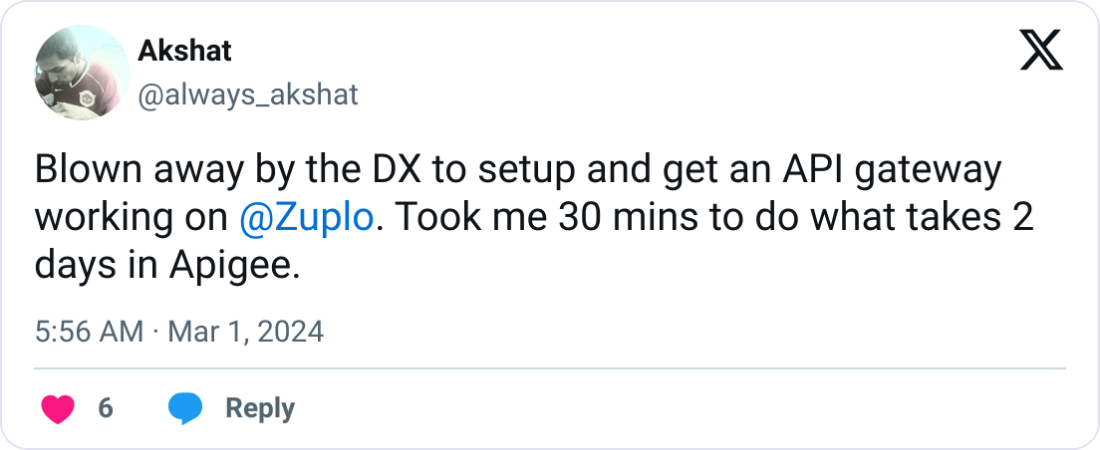
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreLogging and Monitoring for Early Detection#
When it comes to API errors, what you don't know absolutely will hurt you. Proactive monitoring helps catch issues before they impact users and provides data for continuous improvement.
Implement middleware or use API gateways to enforce consistent error handling across all endpoints and services. This ensures uniformity and simplifies maintenance so you can track error rates, types, and patterns.
Utilizing effective API monitoring tools can help detect and resolve issues before they escalate:
- Structured logs that machines can parse and humans can read
- Context preservation that captures the full story, including relevant request details
- Centralized logging that brings everything together in one place
- Real-time alerting for serious issues
Here's what a proper log entry looks like:
{
"timestamp": "2023-04-15T14:23:35.123Z",
"level": "ERROR",
"service": "user-authentication",
"message": "Failed login attempt",
"error_code": "auth_failure",
"request_id": "abc123",
"user_id": "redacted",
"ip_address": "192.168.1.1"
}
Documentation That Actually Helps#
If your API throws errors but doesn't document them, does it make a sound? Yes—the sound of frustrated developers cursing your name. Great API documentation should include:
- A complete catalog of possible error codes with clear explanations
- Real-world example responses showing exactly what errors look like
- Practical guidance on handling or resolving common issues
- Clear advice on when to retry and when to give up
Using interactive design tools can enhance your documentation and help developers and support teams understand error responses better.
Keeping Your API Secure While Being Helpful#
Optimizing API error handling and response codes is a critical balancing act. One wrong move in your error messaging strategy can hand attackers the keys to your kingdom.
Common Pitfalls of API Error Handling#
Here’s what to avoid in your error messages if you want to keep your API robust and your data safe:
Pitfall | Why It’s Dangerous |
---|---|
Exposing stack traces | Reveals internal logic and vulnerabilities |
Returning credentials/tokens | Directly compromises system security |
Overly specific errors/status | Enables resource or user enumeration |
Inconsistent error formats | Causes confusion, accidental data leakage |
Weak input validation | Opens door to injection and other attacks |
Logging sensitive data | Increases risk if logs are accessed by attackers |
No rate limiting | Leaves API open to brute-force and DoS attacks |
Skipping audits/testing | Lets vulnerabilities slip through unnoticed |
Verbose error payloads | Helps with internal debugging; creates public vulnerabilities |
Use HTTP Status Codes Strategically#
HTTP status codes aren't just for show—they're your first line of defense:
- 401 Unauthorized when authentication is missing or invalid
- 403 Forbidden when authorization fails
- 404 Not Found for resources that don't exist (or when you don't want to confirm a protected resource exists)
- 500 Internal Server Error acknowledges something went wrong without specifics
Protect Your System Through Consistency#
Standardization prevents accidental information leakage:
{
"error": {
"code": "AUTH_REQUIRED",
"message": "Authentication is required to access this resource",
"documentation_url": "https://api.example.com/docs/errors/authentication_required"
}
}
This structure gives users what they need without oversharing your system's secrets.
Defensive Measures for API Security#
Beyond error messages, implement these defensive practices:
- Centralize and secure your logs where only authorized personnel can access them
- Implement rate limiting to shut down fishing expeditions
- Validate and sanitize all input before it goes anywhere near your business logic
- Conduct regular security testing to find hidden vulnerabilities in your error handling
Client-Side Strategies That Keep Users Happy#
A seamless user experience means gracefully handling those inevitable moments when things go wrong. In other words, you’ve got to catch problems before they reach the server. You need:
- Syntax validation for required fields, email formats, and numerical values
- Semantic validation for logical relationships between fields
- Real-time feedback that guides users as they complete forms
Craft Error Messages Humans Can Understand#
When errors do happen, the difference between a frustrated user and one who can quickly recover comes down to your error messages:
- Speak human, not machine: Use phrases like, "Please enter a valid email address," instead of "Invalid request parameter."
- Explain what went wrong in terms users understand: Instead of "Error code: 503," say "Our service is temporarily unavailable. We're working to fix it and expect to be back online shortly. Please try again in a few minutes."
- Show exactly how to fix the problem instead of just flagging it: Instead of "Invalid password," say "The password you entered is incorrect. Please double-check your spelling and capitalization, or reset your password if you've forgotten it."
- Maintain your brand voice even when things go wrong: If your brand is playful, instead of "Resource not found," say, "Oops! Looks like that page took a detour. Let's get you back on track."
Plan For the Unexpected#
The internet is unpredictable, and your error handling needs to account for that. It’s a good idea to loop in:
- Network failure detection that tells users when they're offline
- Progress indicators that show something's happening during long operations
- Timeout handling with clear feedback when requests take too long
- Smart retry logic with exponential backoff, random retry timing, and maximum retry limits
Also, being mindful when handling rate limit errors is crucial to ensure your application remains responsive without overloading the API.
Here's a simple implementation:
async function retryRequest(url, maxRetries = 3) {
for (let i = 0; i < maxRetries; i++) {
try {
const response = await fetch(url);
if (response.ok) return response.json();
} catch (error) {
if (i === maxRetries - 1) throw error;
await new Promise((resolve) =>
setTimeout(resolve, Math.pow(2, i) * 1000),
);
}
}
}
Preserve User Work During Failures#
Nothing frustrates users more than losing their work because of an error:
- Preserve form data when submissions fail
- Implement auto-save features for complex data entry
- Use local or session storage to maintain state across page reloads
Creating Error Experiences Users Don't Hate#
Look, API errors happen, it's just a fact of life. But they can really mess with things for users and ultimately affect whether or not they stick around.
The secret to error messages that don't suck? Make them useful by answering:
- What happened?
- Why did it happen?
- What can the user do about it?
Compare these approaches:
❌ "Error 404"
✅ "The employee record you're looking for (ID: 12345) doesn't exist. This could be because the ID is incorrect or the employee has been removed. Try searching by name instead."
How to Fail Gracefully When Systems Crash#
The best apps don't completely break when something goes wrong—they adapt. They do this by containing issues to stop them from spreading, making sure things work even offline, and having backup plans ready.
Let’s look at Stripe's error handling; it’s a masterclass in developer experience.
Detailed Error Objects with Clear, Human-Readable Messages#
Stripe’s error responses are structured and descriptive. Each error object typically includes a clear message, an error code, and additional context:
{
"error": {
"code": "parameter_missing",
"message": "Missing required param: amount.",
"param": "amount",
"type": "invalid_request_error"
}
}
This message tells the developer exactly what went wrong and which parameter needs attention.
Specific Error Types and Codes for Programmatic Handling#
Stripe categorizes errors by both type and code, making it easy for developers to programmatically handle different scenarios:
- Type: Indicates the broad category (e.g., `card_error`, `invalid_request_error`, `authentication_error`, `rate_limit_error`).
- Code: Provides a granular reason (e.g., `card_declined`, `resource_missing`, `parameter_missing`).
Example:
{
"error": {
"code": "card_declined",
"message": "Your card was declined.",
"type": "card_error",
"decline_code": "generic_decline"
}
}
Developers can use the `type` and `code` fields to trigger specific error-handling logic in their applications.
Links to Relevant Documentation for Every Error#
Stripe often includes a `doc_url` field in error responses, linking directly to relevant documentation:
{
"error": {
"code": "resource_missing",
"doc_url": "https://stripe.com/docs/error-codes/resource-missing",
"message": "No such customer: 'cus_xxx'; a similar object exists in live mode, but a test mode key was used to make this request.",
"param": "id",
"type": "invalid_request_error"
}
}
This enables developers to quickly access detailed guidance on resolving the specific error.
Actionable Suggestions for Resolving Issues#
Stripe’s error messages are crafted to be actionable, guiding developers toward resolution. For example, in the case of a missing parameter or an invalid API key, the message is explicit:
- Parameter missing: “Missing required param: amount”
- Authentication error: “No valid API key provided”
Additionally, Stripe’s documentation and error objects often suggest next steps, such as checking parameter names, verifying credentials, or retrying with exponential backoff for rate limit errors
Tailoring Your Approach to Different API Architectures#
Different API architectural styles require specific approaches to optimizing error handling and response codes. Let's see how to handle errors across popular API styles.
REST APIs: Status Code Superheroes#
REST APIs rely heavily on HTTP status codes, but there's more to great REST error handling:
- Use HTTP status codes properly – 4xx for client errors, 5xx for server issues
- Provide detailed error bodies with actionable information
- Include correlation IDs to connect user reports with server logs
- Document your error responses as thoroughly as your successful ones
{
"error": {
"code": "validation_error",
"message": "The request was invalid.",
"details": [
{
"field": "email",
"issue": "Invalid email format"
}
],
"correlation_id": "c7d2a57c-9d36-4a0c-8d7a-28b2e0f3b9ea"
}
}
GraphQL: Partial Success Champions#
GraphQL changes the error handling game with its single endpoint approach:
- Use the standard GraphQL
errors
array structure - Leverage the
extensions
field for custom error codes - Embrace partial success by returning available data alongside errors
- Implement field-level error handling for precision
{
"data": { "user": null },
"errors": [
{
"message": "User not found",
"locations": [{ "line": 2, "column": 3 }],
"path": ["user"],
"extensions": {
"code": "NOT_FOUND",
"classification": "DataFetchingException"
}
}
]
}
gRPC: Status Code Specialists#
gRPC brings its own approach with a dedicated status code system:
- Use gRPC's status codes instead of reinventing the wheel
- Pack detailed error information into messages and metadata
- When exposing gRPC through HTTP gateways, map your status codes carefully
- Utilize the
Status.details
field for contextual information
From Reactive to Predictive: The Future of API Error Handling#
As API ecosystems grow more complex, basic error handling just doesn't cut it anymore. The difference between good and great APIs often comes down to how they leverage advanced techniques to predict, prevent, and rapidly resolve errors. Modern API systems require a proactive approach that predicts and resolves issues before they ever reach production.
Automating Your Way to Better Reliability#
Manual error handling is so 2010. Today's leading APIs implement automation that catches, classifies, and sometimes even resolves errors without human intervention:
- Centralized error logging that correlates errors across your entire ecosystem
- Chaos engineering to find errors before your users do
- Intelligent retry mechanisms with exponential backoff
- Circuit breakers that prevent cascading failures by temporarily disabling problematic dependencies
Implementing federated gateways for productivity can help different teams tailor error-handling practices while maintaining overall consistency.
AI is also revolutionizing how we handle API errors, catching them before they fully manifest. It can spot anomalies and weird patterns that humans would miss. Autonomous agents can classify and sometimes fix issues without human intervention.
Building a Complete Picture of API Health#
Observability goes beyond basic monitoring. It combines distributed tracing, structured logging with correlation IDs, real-time metrics for latency, errors, and resource use, and interactive dashboards for visualizing system health.
When you add machine learning to this rich observability data, you gain the ability to predict failures before they occur:
- Predict traffic spikes and scale resources proactively
- Identify expiring credentials before they cause outages
- Spot unusual patterns that might indicate security issues
- Detect gradual performance degradation before it becomes critical
Turn Your API Errors Into Opportunities#
Throughout this guide, we've seen how thoughtful error handling directly impacts user satisfaction, system reliability, and ultimately your bottom line.
Remember, great error handling isn't a one-and-done project—it's an ongoing practice. Your users might not notice when your error handling is perfect, but that silence speaks volumes about the quality of your API.
Ready to take your API error handling to the next level? Sign up for Zuplo to implement programmable, secure, and user-friendly error handling across all your APIs. With Zuplo's code-first approach, you can customize error responses, implement consistent security practices, and create developer experiences that set your APIs apart.