Redirects to sketchy pages and unexpected pop-ups can often signal a security issue. These disruptions are more than just annoying—they can be serious red flags. If this has happened to you, chances are you've encountered Cross-Site Scripting (XSS) in action. It's one of those sneaky vulnerabilities that lets attackers slip malicious scripts into websites that look perfectly legitimate to you and me.
The scary part? This isn't just a problem for small-time developers working out of their garage. In 2019, a whopping 84% of websites were vulnerable to jQuery-related XSS exploits! That's why every developer needs to know how to fight back against this common threat. In this guide, we'll walk through everything you need to know about XSS and how to keep your applications safe from these insidious attacks.
Understanding Cross-Site Scripting (XSS)#
XSS attacks are like digital sleight of hand. They trick browsers into running malicious code that the user never intended to execute. When successful, these attacks can steal data, hijack user sessions, or even completely deface your website.
So, What Exactly is XSS? At its core, XSS is all about trust. Your users trust your website, and their browsers automatically execute any scripts that appear to come from you. Attackers exploit this trust by injecting their scripts into your web pages. When unsuspecting visitors load those pages, their browsers run the malicious code with all the privileges of your legitimate application. It's kind of like someone sneaking their own ingredient into a chef's recipe—the diners trust the chef, so they eat the food without question, unaware that something dangerous has been added.
The Three Flavors of XSS#
Not all XSS attacks are created equal. They come in three main varieties, each with its own techniques and dangers.
Stored XSS#
Stored XXS is the most persistent and potentially damaging type. The attacker finds a way to permanently store malicious code on your server—perhaps in a database that feeds content to your site. Every visitor who loads the affected page unwittingly executes the hidden script. And this is particularly dangerous on social platforms, forums, or any site where users can post content that others will see later.
Reflected XSS#
Here, the attack comes through the request itself. The malicious script hitches a ride on something like a search query or form submission, and your server unwittingly reflects it back in the response. Users typically fall victim when they click a specially crafted link from an email or another website.
DOM-based XSS#
This sneaky variant executes entirely in the browser through manipulation of the Document Object Model (DOM). The page itself might look safe to server-side scanning tools because the attack happens after the page has loaded, when client-side JavaScript modifies the DOM unsafely.
DOM-based XSS is particularly tricky because it often bypasses server-side protections entirely — like having a security guard at your front door while someone slips in through the back window.
Why XSS Matters to Developers#
The consequences of XSS can be severe for both users and your business. Attackers can steal cookies to hijack user sessions, capture sensitive information from forms, redirect users to phishing sites, or deface your website.
For companies, this means potential data breaches, damaged reputation, and even legal liability if user data is compromised. That's why understanding how to prevent XSS isn't just a technical nice-to-have—it's a business necessity.
Common XSS Prevention Techniques#
Now that we understand the threat, let's talk about how to protect your applications. Fortunately, with the right approaches and adhering to strong security policies, you can dramatically reduce your exposure to XSS vulnerabilities.
Contextual Output Encoding: Your First Line of Defense#
Output encoding is your primary shield against XSS. It transforms potentially dangerous characters into harmless ones before they're rendered in the browser.
When you encode output, you convert characters like <
and >
into their
harmless HTML entity equivalents (<
and >
). This prevents browsers
from interpreting them as HTML tags that could execute scripts.
What makes output encoding tricky is that the encoding needs to match the context where the data appears. There's no one-size-fits-all solution:
HTML Context Encoding#
When outputting data within HTML body content, you need to encode characters
that could form HTML tags or entities. This includes characters like <
, >
,
&
, "
, and '
.
JavaScript Context Encoding#
If you're placing user data within JavaScript code, you need different encoding rules. Characters that could break out of strings or inject code need special handling.
URL Context Encoding#
URLs have their own encoding requirements, especially when building query parameters or fragments from user input.
CSS Context Encoding#
Even CSS can be an attack vector if you're injecting user data into style properties.
Many modern frameworks handle contextual encoding for you, but it's crucial to understand when and how they do this—and when they don't. For instance, React automatically escapes values in JSX, but bypassing this with dangerouslySetInnerHTML requires careful handling.
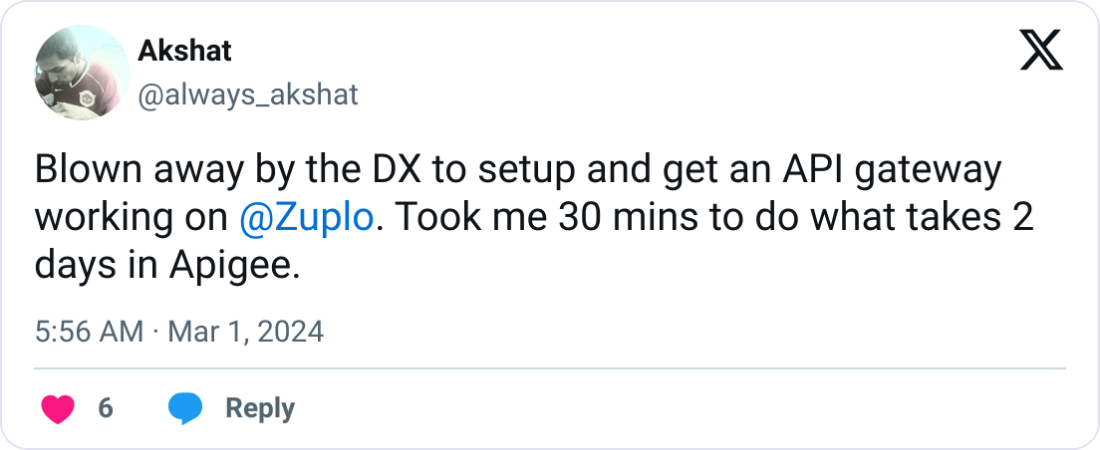
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreHTML Sanitization: Cleaning the Dirty Laundry#
Sometimes you need to allow some HTML—perhaps you're building a rich text editor or a comment system with formatting. This is where HTML sanitization comes in.
Unlike encoding (which makes everything safe but unusable as HTML), sanitization allows some HTML elements and attributes while stripping out dangerous ones.
Libraries like DOMPurify are specifically designed for this task:
The key to effective sanitization is being strict about what you allow, rather than trying to block what you don't want.
Whitelist, Don't Blacklist#
Specify exactly which HTML elements and attributes are permitted, rather than trying to block "bad" ones. New attack vectors emerge constantly, making blacklists perpetually incomplete.
Keep Your Sanitizer Updated#
Sanitization libraries are regularly updated to address new attack vectors. An outdated sanitizer might miss newer exploits.
Test Your Sanitization#
Regularly test your sanitization logic with known XSS payloads to verify it's working as expected.
Input Validation: Checking ID at the Door#
While sanitization and encoding happen on the way out, validation happens on the way in. Input validation, such as using JSON Schema validation, ensures that user-supplied data matches the expected format and constraints before you process it:
Format Constraints#
For fields like email addresses, phone numbers, or dates, validate that they match the expected pattern:
Length Restrictions#
Limiting input length can prevent buffer overflow attacks and reduce the attack surface:
Type Checking#
Ensure numeric fields contain only numbers, etc.:
Remember that client-side validation is easily bypassed, so always validate on the server side as well. Input validation isn't a complete XSS defense on its own, but it's an important part of a layered security approach. For more comprehensive strategies, consider following API security best practices.
Frameworks and Their Security Perspectives#
Modern JavaScript frameworks have built-in protections against XSS, but each has its own approach and potential pitfalls.
React: Automatic Escaping, But Watch Those Props#
React automatically escapes values in JSX, which provides excellent protection against most XSS attacks. However, there are escape hatches that can introduce vulnerabilities if used carelessly.
The dangerouslySetInnerHTML Trap#
This appropriately named prop lets you inject raw HTML, bypassing React's automatic protections:
Always sanitize content before using this prop, preferably with a library like DOMPurify.
URL Attributes Need Attention#
React doesn't validate URLs in attributes like href
, so malicious JavaScript
URLs can slip through:
Always validate URLs before using them in links or other attributes.
Angular: Strong Defaults with Multiple Layers#
Angular treats all values as untrusted by default and automatically sanitizes content based on context. This provides robust protection against most XSS attacks.
The Ahead-of-Time Compiler#
Angular's AOT compiler analyzes templates at build time, detecting many potential security issues before they reach production.
DomSanitizer Service#
For cases where you need to bypass Angular's automatic sanitization, the
DomSanitizer
service provides context-aware methods:
These methods should be used sparingly and only with content you've verified is safe.
Vue.js: Automatic Escaping with Clear Overrides#
Like React and Angular, Vue automatically escapes content in templates. However, it also provides clear mechanisms to override this behavior when needed.
The v-html
Directive#
This directive renders raw HTML, similar to React's dangerouslySetInnerHTML
:
Always sanitize content before using v-html
.
Binding to Dangerous Attributes#
Vue doesn't validate URLs in attributes like href
, so similar precautions are
needed as with React:
Framework Best Practices#
Regardless of which framework you use, follow these best practices:
Stay Updated#
Framework security improvements often come through updates. Keep your dependencies current to benefit from the latest protections.
Understand the Defaults#
Know what your framework protects automatically and where you need to add extra validation or sanitization.
Use TypeScript#
Type checking can catch many potential security issues at build time, before they reach production.
Advanced Concepts in XSS Prevention#
Beyond the basics, there are more sophisticated approaches to XSS prevention that can further strengthen your defenses.
Safe Sinks and Dangerous Contexts#
Not all places where user input ends up (called "sinks" in security parlance) are equally dangerous. Understanding which contexts require special attention can help focus your security efforts.
High-Risk Sinks#
These contexts are particularly dangerous for untrusted input:
- Script tags and event handlers
- Inline CSS with
javascript:
URLs - HTML attributes that can execute code (like
onclick
) - URL protocols that execute code (
javascript:
,data:
)
Even with frameworks that handle sanitization, be especially careful when placing user input in these contexts.
Content Security Policy: Your Safety Net#
A Content Security Policy (CSP) acts as an additional layer of defense by telling browsers which sources of content are legitimate. Even if an XSS vulnerability exists, a properly configured CSP can prevent the malicious script from loading resources or sending data to the attacker.
A basic CSP might look like this:
This policy tells browsers to only load scripts from the same origin or from trusted-cdn.com, blocking inline scripts and scripts from other sources.
CSP Reporting#
CSP can also be configured to report violations, helping you identify potential attacks:
This mode doesn't block anything but sends reports of violations to your specified endpoint.
Methodologies Employed By XSS Attackers#
Understanding how attackers think and operate can help you better defend against them. XSS attacks have evolved over time, becoming increasingly sophisticated.
Classic Attack Vectors#
There are several tried-and-tested techniques that attackers bank on.
- Exploiting Input Fields — Comment forms, search boxes, and profile fields are all potential entry points for XSS attacks. Attackers input malicious scripts that get stored and then executed when other users view the content.
- Crafting Malicious Links — For reflected XSS, attackers create specially crafted URLs that include script code as part of a query parameter or fragment identifier. When users click these links, the script executes in their browsers.
- Targeting Client-Side Templates — Single-page applications that dynamically render templates can be vulnerable to template injection attacks, a variant of XSS where the attacker injects malicious template syntax.
Evasion Techniques#
Modern attackers use various tricks to bypass common protections:
- Encoding Obfuscation — XSS payloads can be encoded in various ways to evade simple filters.
- Event Handler Variety — Rather than using obvious event handlers like
onclick
, attackers might use less common ones likeonmouseover
,onerror
, oronload
. - Script-Less Attacks — Some XSS attacks don't require JavaScript at all, using CSS or HTML to achieve malicious goals.
Implementing Server-Side Security Measures#
While client-side protections are important, server-side security measures form the backbone of your XSS defense strategy.
Content Security Policy Implementation#
We touched on CSP earlier, but its implementation deserves more attention. A robust CSP can be incrementally deployed to minimize disruption:
Start in Report-Only Mode#
Begin with CSP in report-only mode to identify legitimate content that would be blocked:
Refine and Tighten#
Gradually refine your policy based on reports, adding necessary sources and removing inline scripts and styles:
Adopt Nonces for Inline Scripts#
For unavoidable inline scripts, use nonces (random values generated per request) to whitelist specific scripts:
Then include the nonce in your script tags:
Additional Server-Side Controls#
Beyond CSP, several other server-side measures can enhance your XSS protection:
HTTP Security Headers#
Headers like X-XSS-Protection, X-Content-Type-Options, and Referrer-Policy provide additional layers of browser protection:
Cookie Security#
Secure your cookies against theft with appropriate flags:
- HttpOnly prevents JavaScript from accessing the cookie
- Secure ensures the cookie is only sent over HTTPS
- SameSite prevents the cookie from being sent in cross-site requests
CORS Policies#
Implementing custom CORS policies can control cross-origin resource sharing and help protect your application from unauthorized access.
Server-Side Validation and Sanitization#
Even with client-side validation, always validate and sanitize data on the server. Attackers can bypass client-side checks entirely by sending requests directly to your API.
Authentication Controls#
Implementing authentication mechanisms like Basic HTTP Authentication can add an extra layer of security to your applications.
Avoiding XSS Prevention Anti-Patterns#
Some approaches give a false sense of security. Knowing what not to do is just as important as knowing what to do.
Relying Solely on Client-Side Validation#
Client-side validation is easily bypassed. Attackers can modify requests directly or disable JavaScript in their browsers to circumvent these checks.
Using Regular Expressions to Detect XSS#
Simple regex patterns like searching for <script>
are trivially bypassed with
encoding or alternative syntax. Modern XSS payloads can be extremely convoluted
and creative.
Implementing Security by Obscurity#
Renaming parameters or obfuscating your code doesn't provide real security. Assume attackers can and will reverse-engineer your application.
Depending Entirely on Web Application Firewalls#
WAFs can catch known attack patterns but often miss novel or obfuscated attacks. They should be one layer in a comprehensive defense strategy, not your primary protection.
Ensuring Continuous Security Practices#
Security isn't a one-time effort but an ongoing process. Integrating security into your development lifecycle is essential. Here are a few things to keep in mind:
-
Regular Security Training#
Keep your team updated on the latest threats and prevention techniques through regular training sessions and workshops.
-
Security-Focused Code Reviews#
Include security-specific questions in code reviews:
- Is user input properly validated and sanitized?
- Are we using the framework's XSS protections correctly?
- Are we avoiding dangerous patterns like
innerHTML
without sanitization?
-
Automated Security Testing#
Incorporate security testing into your CI/CD pipeline:
- Static Application Security Testing (SAST) to identify code vulnerabilities
- Dynamic Application Security Testing (DAST) to test running applications
- Regular penetration testing to identify vulnerabilities human testers might miss
-
Stay Informed#
Follow security mailing lists, blogs, and CVE announcements to stay aware of new vulnerabilities and attack techniques.
Ace Your XSS Planning#
Cross-Site Scripting remains one of the most prevalent web security threats, but it's preventable with the right approach. Implement contextual output encoding, validate inputs, sanitize HTML, and use your framework's built-in protections—but remember no single technique is enough. Security works best in layers, combining client-side and server-side protections with tools like Content Security Policy to create a comprehensive defense.
Looking for an easier way to implement API security? Zuplo's platform helps you apply XSS protection and other security best practices with minimal effort. From automatic input validation to request filtering and security headers, Zuplo secures your APIs without slowing development. Sign up for free today!