Load balancing isn't just a fancy term – it's the secret sauce that transforms sluggish APIs into speed demons. When implemented right, load balancing spreads incoming requests across multiple servers, preventing any single server from screaming "I give up!" under pressure. And let's be honest, if your API handles significant traffic, you absolutely need this in your tech stack.
Think of load balancing like a restaurant with multiple chefs instead of just one poor soul. Rather than watching orders pile up at a single station, they're evenly distributed, keeping customers happy and food flowing. That's exactly what proper load balancing does for your API.
Let's dive into how load balancing can transform your API performance and keep your users coming back for more.
- What Is Load Balancing in API Development?
- Types of Load Balancing Techniques
- How Load Balancing Improves API Performance
- Implementing Load Balancing in Your API Architecture
- Handling Failover and Redundancy with Load Balancing
- Enhancing API Security with Load Balancing
- Performance Monitoring and Optimization with Load Balancers
- Advanced Load Balancing Strategies
- From Bottlenecks to Breakthroughs: The Load Balancing Advantage
What Is Load Balancing in API Development?#
To fully grasp load balancing in API development, it's important to have a solid understanding of API fundamentals. Load balancing distributes incoming requests across multiple servers, ensuring none of them collapse under pressure. Think of it as your API's traffic cop – standing between users and your backend servers, receiving all incoming requests and intelligently routing them to the most appropriate server in your resource pool.
When you implement load balancing for your API, the system continuously monitors server health and availability. During those inevitable traffic spikes? It efficiently handles increased demand by dynamically adding servers to meet user needs. No drama, no downtime, no problem.
Load balancers come in different flavors – hardware devices with specialized software, purely software-based solutions, or cloud-based services. Each offers different capabilities, but they all serve the same critical purpose: keeping your API running like a well-oiled machine.
Why Load Balancing Is Crucial for API Performance#
Let's be real – without proper load balancing, your API is living on borrowed time. Here's why implementing it is non-negotiable:
- Scale like you mean it: Load balancing lets your API infrastructure grow on demand based on actual traffic needs, not guesswork.
- Reliability that makes DevOps sleep at night: By distributing requests across multiple servers, you create redundancy that minimizes the impact when (not if) a server fails.
- Maintenance without the tears: Need to update a server? Simply remove it from the pool while the load balancer routes traffic elsewhere. No downtime, no angry users.
- Performance that doesn't crack under pressure: During high-traffic periods, load balancing prevents your API from grinding to a halt by efficiently spreading the workload.
- Predict problems before they happen: Advanced load balancers detect potential failures early and manage them proactively. They're basically fortune tellers for your infrastructure.
- Security that actually works: Load balancers add an extra layer of protection to your API, helping defend against those pesky distributed denial-of-service attacks.
Without proper load balancing, your API is basically a ticking time bomb – slow response times, climbing error rates, and complete failures during peak usage are just waiting to happen. And we both know that's not the kind of excitement your business needs.
Types of Load Balancing Techniques#
Not all load balancing is created equal. Let's break down the different approaches and when to use them.
Round Robin Load Balancing#
Round Robin is the straightforward cousin in the load-balancing family – it passes requests to each server in sequence without overthinking it.
What makes it shine? Simplicity and fairness. It has minimal computational overhead and distributes traffic evenly, making it perfect for basic API infrastructures with similar server specs and predictable request patterns.
But here's the catch – Round Robin treats all servers equally, which becomes problematic when your servers have different processing power or when some API requests are more complex than others. It's like assigning the same workload to both a junior and senior developer – someone's going to be either overwhelmed or underutilized.
Least Connections Load Balancing#
While Round Robin obsesses over equality, Least Connections takes a smarter approach by sending new requests to servers with the fewest active connections. It's like picking the checkout line with the fewest customers at the grocery store.
This algorithm absolutely dominates when your API handles connections of varying duration. When one server gets backed up with complex requests, the algorithm automatically directs new traffic elsewhere until that server catches up.
The downside? Increased complexity. You'll need to constantly monitor active connections across all servers, which requires more sophisticated infrastructure.
IP Hash Load Balancing#
IP Hash uses the client's IP address to determine server assignments. The algorithm creates a hash value from the client's IP and uses that value to consistently route that client to the same server.
This "stickiness" is crucial for APIs that maintain session data or user-specific information on particular servers. E-commerce APIs particularly benefit as shopping carts and user preferences stay consistent throughout a browsing session.
The downside? IP Hash doesn't care about server capacity or current load, which can lead to uneven distribution if your user base isn't perfectly balanced geographically.
Weighted Load Balancing#
Weighted approaches give you precise control by assigning different weights to servers based on their actual capacity. It's like recognizing that your senior developer can handle three times the workload of your intern.
This technique shines in heterogeneous environments where servers have different processing capabilities. If server A has twice the capacity of server B, you might assign it a weight of 2, meaning it receives twice as many requests.
The challenge? You'll need to manually determine appropriate weights based on server specifications and regularly adjust them as conditions change. It's more hands-on, but the performance gains are worth it.
Global Load Balancing#
While local load balancing distributes traffic within a single data center, global load balancing routes API requests across multiple geographic regions based on factors like user location, regional server availability, and regional traffic patterns.
This approach dramatically improves performance for international API users by directing them to the closest or most responsive data center. Your users in Tokyo hit your Tokyo servers, while users in London hit your EU servers – everyone wins with faster response times.
This approach is essential when deploying APIs on edge networks to ensure low latency and high availability for users worldwide.
Global load balancing also provides superior disaster recovery. If an entire region experiences issues (natural disasters, power outages, etc.), traffic seamlessly redirects to functioning regions without downtime.
The tradeoff is increased complexity in implementation, monitoring, and maintaining data consistency across distributed environments. But for global APIs, the benefits far outweigh these challenges.
How Load Balancing Improves API Performance#
When properly implemented, load balancing transforms sluggish, unreliable APIs into responsive, resilient systems that users actually want to use.
Performance Improvement Through Load Balancing#
The most immediate benefit is dramatically improved response times. By intelligently spreading requests across multiple servers, load balancers prevent any single server from becoming a bottleneck, which is crucial for optimizing API performance.
The Least Response Time algorithm routes new requests to whichever server can respond fastest, constantly monitoring how quickly each server is processing requests and adjusting routing decisions in real-time. It's like having a personal assistant who always knows which team member can handle your task the quickest.
Beyond just speed, load balancing provides crucial fault tolerance for your API. If one server crashes or needs maintenance, the load balancer immediately redirects traffic to healthy servers—often so seamlessly that users never notice the transition. That's the difference between "our system is down" and "business as usual."
Combined with other strategies such as rate limiting in distributed systems, load balancing ensures your API can handle high traffic efficiently without sacrificing performance.
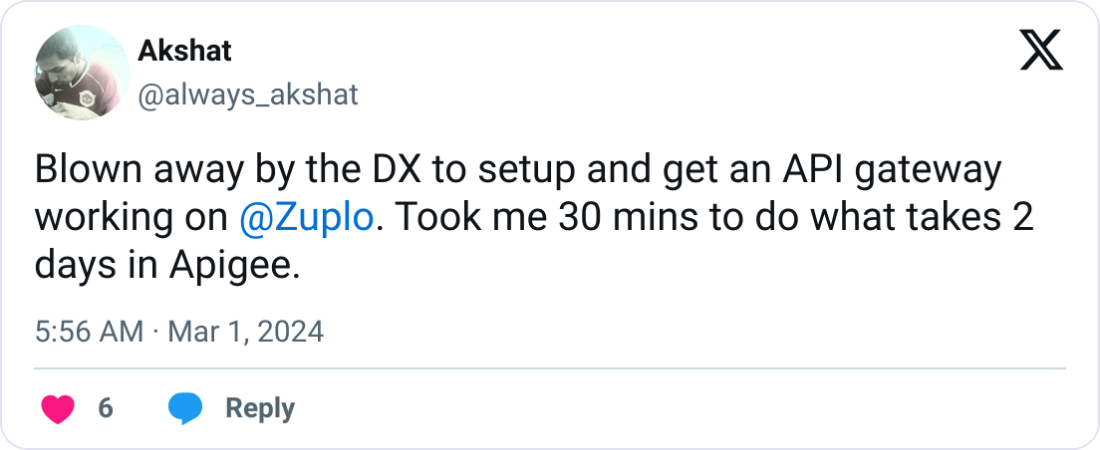
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreScalability and Flexibility#
One of the most powerful aspects of load balancing is how it enables on-demand scalability. When your API traffic suddenly spikes (maybe your product just went viral on Twitter), load balancers distribute that increased load across your server pool, preventing performance degradation.
This scalability becomes particularly valuable for businesses with seasonal or unpredictable traffic patterns. With load balancing, you can seamlessly add or remove servers from your pool without disrupting service. It's like being able to instantly hire extra staff when your store gets busy, then send them home when things slow down.
Tools like Zuplo integrations can enhance scalability by simplifying the addition or removal of services in your API infrastructure.
Load balancing also enhances API security without requiring extensive modifications to your core codebase. Modern load balancers include built-in security features that help defend against distributed denial-of-service (DDoS) attacks.
The ultimate goal of all these improvements is enhancing customer experience by maintaining responsive performance even during traffic surges. Because let's face it – in today's world, users expect instant responses and have zero patience for slow APIs.
Implementing Load Balancing in Your API Architecture#
Ready to transform your API performance? Here's how to integrate load balancing into your existing architecture without losing your mind.
Step-by-Step Guide to Load Balancing Integration#
- Determine your load balancing requirements: Analyze your traffic patterns to guide your strategy. Don't just implement load balancing blindly – understand your specific needs first.
- Choose the right load-balancing algorithm: Different scenarios demand
different algorithms:
- Least Connection: Perfect when your API connections vary in duration.
- Weighted Least Connection: Gives more powerful servers a higher proportion of traffic.
- Resource-based: Dynamically adjusts routing based on CPU, memory, and network utilization.
- Geolocation-based: Routes requests to servers physically closest to the user's location.
- Install redundant load balancers: Don't create a new single point of failure! Deploy multiple load balancers to ensure continuous operation even if one fails.
- Configure SSL termination: Terminate SSL connections at the load balancer to offload the CPU-intensive decryption process from your application servers. This alone can dramatically improve performance.
- Set up health checks: Implement robust health monitoring so your load balancer only routes traffic to healthy servers. Don't send your users to servers that are gasping for air.
- Enable monitoring and logging: Set up comprehensive monitoring to track load balancer performance metrics. You can't improve what you don't measure.
Configuration Examples#
Weighted Round Robin Configuration
This approach distributes varying traffic loads across your network based on server capacity, useful for A/B testing with load balancing new API versions or when gradually introducing new infrastructure.
Layer 7 Application Load Balancer Setup
For APIs that require advanced routing based on request content:
# Sample NGINX configuration for API load balancing
http {
upstream api_servers {
server api1.example.com weight=3;
server api2.example.com weight=2;
server backup.example.com backup;
}
server {
listen 80;
location /api/ {
proxy_pass http://api_servers;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
}
Handling Failover and Redundancy with Load Balancing#
Server crashes happen to the best of us. But with proper load balancing, they'll have minimal impact on your users. That's the power of failover and redundancy – your secret weapon against downtime.
Failover Mechanisms and Importance#
Failover is when load balancers automatically detect a server failure and instantly reroute traffic to healthy alternatives. Modern dynamic load balancers constantly monitor the health and performance of every server in your pool.
These health checks verify server availability by fetching a designated health check endpoint every few seconds. If a server fails to respond correctly multiple times, the load balancer temporarily removes it from the pool. It's like having a bouncer who quickly escorts troublemakers out the door before they cause problems.
Different failover implementations exist:
- DNS Failover: Redirects traffic at the DNS level when your primary server becomes unavailable.
- Round Robin with Failover: Distributes traffic evenly but seamlessly redirects queries if one resource becomes unhealthy.
The beauty of these mechanisms is their transparency to end users. Your customers never need to know that there was a problem – they just experience continuous, uninterrupted service.
Ensuring Redundancy for High Availability#
For proper redundancy implementation, deploy your load balancer instances across multiple availability zones within a region. This provides crucial safeguards in case an entire zone experiences downtime.
When setting up multiple availability zones:
- Select at least two zones from your region.
- Ensure your backend instances are distributed across these zones.
- Implement cross-zone load balancing so requests are distributed based on target health rather than zone.
Regularly test your failover configuration by simulating server failures and monitoring how your system responds. Don't wait for a real disaster to discover your failover doesn't work!
Enhancing API Security with Load Balancing#
Load balancers aren't just performance boosters—they're also powerful security assets that can shield your APIs from various threats. Let's see how they pull double-duty:
Role of Load Balancers in API Security#
Load balancers create an additional protective layer between attackers and your application servers, enhancing security by:
- Eliminating single points of failure that attackers might target.
- Reducing the overall attack surface exposed to potential threats.
- Making it harder for attackers to exhaust your resources.
- Preventing link saturation from malicious traffic.
Modern load balancers support SSL and TLS encryption, ensuring data traveling between clients and your API remains protected, aligning with API security best practices.
Furthermore, integrating API authentication methods with your load-balancing strategy can significantly improve security.
Load balancers also help minimize potential attack vectors by restricting HTTP traffic paths and enforcing connection limits that prevent resource exhaustion. They're like bouncers who not only check IDs but also make sure no single person monopolizes the bar.
Mitigating DDoS Attacks with Load Balancing#
Load balancers provide several powerful defenses against DDoS attacks:
- TCP SYN Flood Protection: Mitigates common exploits that attempt to consume all available connection resources.
- HTTP Request Timeout Implementation: Defends against Slowloris attacks, which work by keeping connections open indefinitely.
- Connection Rate Limiting: Restricts the number of TCP connections per user, reducing the impact of aggressive connection attempts.
- Rerouting During Attacks: Redirects legitimate traffic to unaffected resources during attacks.
When combined with an API gateway, you gain even stronger security capabilities with authentication, authorization, and rate-limiting features alongside traffic distribution. It's like having both a bouncer and a security team working together.
Performance Monitoring and Optimization with Load Balancers#
Understanding how load balancing affects your API performance through monitoring allows you to fine-tune your strategy and squeeze out every last drop of performance.
Key Metrics for Monitoring Load Balancer Performance#
Focus on these essential metrics that actually matter:
- Response times: Track how quickly requests are processed across your infrastructure. This is what your users actually feel.
- Server health: Monitor the health status of your backend servers to ensure traffic is only being directed to fully functional instances.
- Traffic distribution: Keep an eye on how evenly your load is being spread across backend servers. Imbalances can indicate problems.
- Connection counts: The number of active connections handled by each server reveals a lot about your system's behavior.
- TLS handshake times: For secure connections, the time spent on TLS handshakes can significantly impact overall performance.
Utilizing effective API monitoring tools can help you track these metrics and gain insights into your load balancer's performance.
Best Practices for Analyzing Logs and Metrics#
For effective monitoring and analysis:
- Enable access logs: Access logs capture detailed information about every request sent to your load balancer. They're like security cameras for your API traffic.
- Set up comprehensive metrics: Implement monitoring for aggregate statistics like total connections, healthy host count, and TLS handshakes.
- Create custom dashboards: Develop dashboards that display your most important indicators in one place. Don't make yourself hunt for critical data.
- Configure alerts: Set up notifications for when key metrics exceed predefined thresholds. Be proactive, not reactive.
- Regularly review traffic patterns: Analyzing traffic patterns over time helps you optimize your infrastructure for actual usage, not theoretical scenarios.
Advanced Load Balancing Strategies#
When your API traffic grows more complex, it's time to explore advanced techniques that take performance to the next level.
Application-Aware Load Balancing#
Application-aware load balancing operates at Layer 7 of the OSI model, making intelligent routing decisions based on the actual content being processed, analyzing:
- HTTP/S headers
- Session cookies
- Message content
- Application-specific metrics
The Least Pending Requests (LPR) method tracks how many requests each server is actively processing and prioritizes servers with fewer pending requests. It's like knowing which line at the DMV is actually moving faster, not just which one has fewer people.
Resource-Based Adaptive load balancing uses real-time performance data retrieved directly from your backend servers through a custom agent program that provides detailed health information. This approach absolutely dominates in environments where workloads vary significantly in complexity and resource requirements.
Intelligent Load Balancing with Machine Learning#
The cutting edge of load balancing incorporates machine learning and artificial intelligence for truly dynamic traffic distribution. This isn't just smart – it's genius-level traffic management.
SDN (Software Defined Network) adaptive load balancing leverages insights across multiple network layers alongside input from an SDN controller, factoring in:
- Server status and health
- Application performance metrics
- Network infrastructure conditions
- Current congestion levels
ML-powered load balancers make increasingly sophisticated routing decisions that optimize not just for server availability, but for end-to-end performance and user experience.
What makes these systems particularly powerful is their ability to learn and adapt, continuously refining their routing algorithms based on observed performance. They don't just follow rules – they write new ones based on what actually works.
From Bottlenecks to Breakthroughs: The Load Balancing Advantage#
Load balancing isn't just nice to have—it's the backbone of high-performing APIs that users actually want to use. By distributing incoming requests intelligently across multiple servers, you eliminate bottlenecks that cause sluggish performance and send users running to your competitors.
Ready to take your API performance to the next level? Zuplo can help you implement robust, scalable API management with built-in load balancing capabilities that actually work. Book a meeting with Zuplo today to learn how our platform can transform your API infrastructure while keeping implementation simple.