REST or gRPC? A Guide to Efficient API Design
The REST vs gRPC decision shapes how your entire system breathes. REST is like that reliable friend everyone knows—approachable, widely supported, and getting the job done without drama. gRPC, meanwhile, is the speed demon that makes REST look like it's running in slow motion, with performance benefits that can transform your architecture.
This architectural decision ripples through everything—from how quickly your app responds to how easily new developers join your team. What's cool is you don't have to choose just one. Smart teams are using REST for their public APIs while powering their behind-the-scenes communication with gRPC, where every millisecond counts. Let's explore which approach (or combination) makes the most sense for your specific needs.
- Understanding the Core Concepts: REST vs gRPC
- Speed vs Simplicity: The Technical REST Vs gRPC Showdown
- Performance That Speaks Volumes: gRPC Real-World Metrics
- Choosing Your Champion Between REST or gRPC: Use Case Analysis
- Navigating REST and gRPC Implementation Challenges
- Best of Both Worlds with REST and gRPC: A Hybrid Approach
- Future-Proofing Your API Strategy
- Making the Right Choice: A Decision Framework
- The Bottom Line With REST or gRPC: Different Tools for Different Jobs
Understanding the Core Concepts: REST vs gRPC#
REST isn't just another API approach—it's the foundation that's powered web APIs for over two decades. But gRPC is turning heads with performance that makes REST look like it's moving in slow motion.
The Fundamentals of REST#
REST is an architectural style created by Roy Fielding in 2000 that establishes clear principles:
- Statelessness: Every request contains everything servers need to understand it
- Client-Server Architecture: Clear separation between user interfaces and data storage
- Uniform Interface: Resources identified through URIs with standardized HTTP methods
- Resource Orientation: Everything is a resource manipulated through standard HTTP verbs
REST APIs typically use standard HTTP methods for different operations:
- GET: Retrieve a resource
- POST: Create a new resource
- PUT: Update an existing resource
- DELETE: Remove a resource
Data in REST APIs is commonly exchanged using JSON or XML formats, with JSON dominating modern applications due to its lightweight nature and JavaScript compatibility. REST APIs are often documented using API definitions to provide clear specifications for developers.
gRPC and the Power of Protocol Buffers#
gRPC is a high-performance, open-source RPC framework initially developed by Google that makes REST look like it's running on dial-up. Unlike REST's resource-oriented approach, gRPC follows a service-oriented architecture where you define services and methods for remote calls.
At its core, gRPC uses
Protocol Buffers (protobuf)
as its interface definition language. Protocol Buffers let you define data
structures and services in a language-agnostic way in a .proto
file:
syntax = "proto3";
service UserService {
rpc GetUser (UserRequest) returns (User) {}
rpc ListUsers (ListUsersRequest) returns (stream User) {}
rpc CreateUser (User) returns (CreateUserResponse) {}
}
message UserRequest {
string user_id = 1;
}
message User {
string user_id = 1;
string name = 2;
string email = 3;
}
message ListUsersRequest {
int32 page_size = 1;
}
message CreateUserResponse {
bool success = 1;
string user_id = 2;
}
gRPC uses HTTP/2 as its transport protocol, providing benefits like:
- Multiplexing: Multiple requests and responses sent concurrently over a single connection
- Header compression: Reduces overhead
- Bidirectional streaming: Enables real-time communication
One of gRPC's most powerful features is its ability to generate client and server code from the protobuf definition across numerous programming languages, making it ideal for polyglot environments.
Speed vs Simplicity: The Technical REST Vs gRPC Showdown#
When weighing REST against gRPC, you're essentially balancing familiar accessibility against remarkable performance. This tradeoff influences every aspect of your API design.
The Need for Speed: Protocol and Communication#
REST and gRPC operate on completely different planets when it comes to communication models:
REST APIs typically use HTTP/1.1, following a simple request-response pattern with each needing its own TCP connection. Simple? Yes. Efficient at scale? Not even close.
gRPC is built on HTTP/2, which is like strapping a rocket to your API:
- Multiplexing: Multiple requests and responses travel concurrently over a single TCP connection
- Header compression: Slashes bandwidth consumption
- Binary framing: Parses data way more efficiently than HTTP/1.1's text-based approach
- Server push: Servers can proactively send resources to clients
gRPC offers four distinct communication patterns:
- Unary RPC: One request, one response (like REST)
- Server streaming: Client sends one request, server responds with a stream of messages
- Client streaming: Client sends a stream of messages, server responds once
- Bidirectional streaming: Both sides can send message streams independently
The speed difference is dramatic—gRPC connections can be seven times faster than REST for receiving data and roughly ten times faster for sending data in certain scenarios.
Data Diets: Serialization and Efficiency#
REST uses human-readable formats like JSON or XML—great for humans, terrible for efficiency.
gRPC uses Protocol Buffers (Protobuf) as its serialization mechanism with game-changing benefits:
- Tiny payloads: Binary nature makes data packets significantly smaller
- Lightning-fast serialization: Binary formats parse at warp speed compared to text-based ones
- Strong typing: Defines message structures in .proto files, catching errors before they happen
According to benchmarks, gRPC can be 5 times faster for small payloads and 1.5 times faster for larger payloads (1MB)compared to REST—especially valuable for mobile devices or IoT systems where every byte counts.
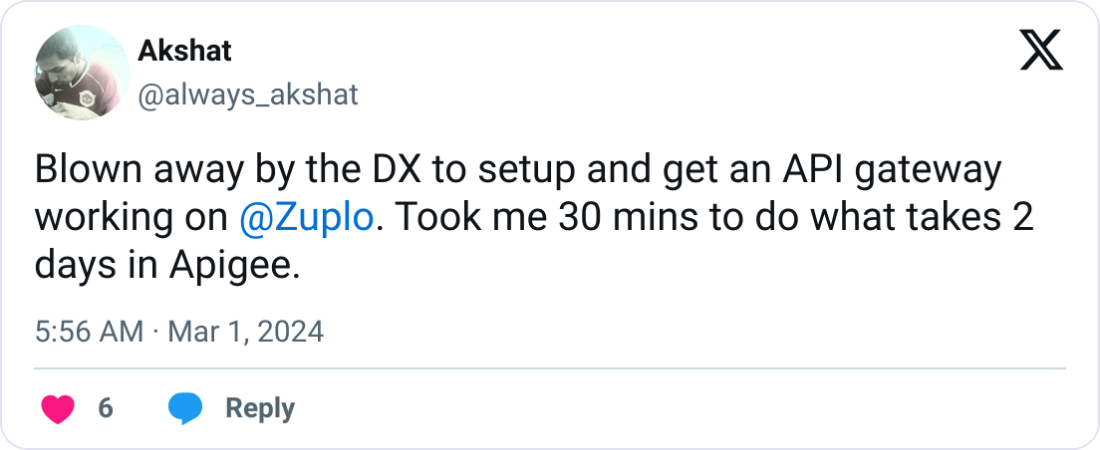
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreContract Evolution: Defining and Growing APIs#
REST APIs typically use the OpenAPI specification (formerly Swagger), which are:
- Descriptive: They document existing API behavior
- Flexible: Easy to change but can lead to inconsistencies
- Human-readable: JSON or YAML formats anyone can understand
gRPC uses Protocol Buffer (Protobuf) definitions, which are:
- Prescriptive: Defines the API contract that implementations must follow
- Strictly typed: Enforces type checking for parameters and return values
- Language-neutral: The same .proto files generate client/server code across multiple languages
For API evolution:
- REST offers flexibility but lacks formal versioning, often relying on URL
paths (e.g.,
/v1/users
). To manage this, understanding API versioning strategies is essential. - gRPC provides built-in backward compatibility through Protobuf's field numbering system, allowing new fields without breaking existing clients
Developer Experience: Tools and Workflow#
REST's main advantage is its ubiquity and simplicity, contributing to a smooth developer experience for APIs:
- Test endpoints directly in a browser or with simple tools like curl
- Use libraries in virtually any programming language
- Debug easily by looking at human-readable request/response bodies
- REST also allows for straightforward authentication mechanisms, such as using API keys for authentication, making initial setup simple for developers.
gRPC requires specialized tools but offers massive productivity advantages:
- Automatic code generation: Eliminates boilerplate code
- Strong typing: Catches errors before they hit production
- Standardized error handling: Consistent error model across languages and platforms
However, debugging gRPC can be trickier with binary Protobuf messages and the need for specialized tools like gRPCurl or gRPC UI for testing.
Many successful systems use both protocols, applying each where its strengths shine brightest—REST for public-facing APIs and browser integration, with gRPC for high-performance internal communication.
Performance That Speaks Volumes: gRPC Real-World Metrics#
gRPC isn't just faster than REST; it's in a completely different performance league with numbers that can transform your entire architecture.
Speed Demons: Latency and Throughput#
As mentioned above, gRPC connections are seven times faster than REST connections for receiving data and roughly ten times faster for sending data for specific payloads. In a microservices architecture where milliseconds matter, this gap is the difference between smooth sailing and sinking under load. If you're looking to increase API performance, adopting gRPC could be a game-changer.
Resource Efficiency: More Bang for Your Buck#
Beyond raw speed, gRPC demonstrates incredible efficiency:
- CPU Usage: Binary serialization requires less processing power than parsing JSON or XML
- Memory Consumption: HTTP/2's header compression means lower memory footprints
- Network Bandwidth: Binary protocols consume significantly less bandwidth
Choosing Your Champion Between REST or gRPC: Use Case Analysis#
Picking between REST and gRPC isn't about choosing the "best" protocol—it's about picking the right tool for your specific job.
When REST Rules the Roost#
REST remains the go-to choice in several scenarios:
- Public-facing APIs: When your API needs to be consumed by the masses, REST's simplicity and ubiquity lower the barrier for third-party developers.
- Simple CRUD operations: For applications primarily doing basic data operations, REST's resource-oriented design maps perfectly to standard HTTP methods.
- Browser-based applications: Since browsers natively support HTTP requests, REST APIs can be directly consumed by frontend JavaScript.
- Maximum developer familiarity: With its longer history and widespread adoption, more developers know REST inside and out.
REST dominates when you need to prioritize broad accessibility over raw performance, such as public weather APIs.
When gRPC Takes the Crown#
gRPC offers massive advantages in specific contexts:
- Microservices communication: For internal service-to-service communication, gRPC's performance advantages are game-changing.
- Low-latency requirements: Applications where milliseconds matter, such as financial trading platforms or real-time bidding systems.
- Real-time streaming needs: gRPC's native support for bidirectional streaming enables efficient real-time applications.
- Complex service interactions: Strong typing provided by Protocol Buffers helps enforce contracts between services.
- Polyglot environments: When your architecture spans multiple programming languages, gRPC ensures consistent API implementations.
gRPC excels in scenarios like IoT platforms or real-time analytics systems processing large volumes of data with minimal latency. Additionally, the business model for APIs can influence your protocol choice.
Navigating REST and gRPC Implementation Challenges#
Implementing either REST or gRPC comes with its own set of headaches, but knowing these challenges upfront will save you countless hours of frustration.
REST Implementation Battlegrounds#
When working with REST APIs, expect these common challenges:
The Complex Query Conundrum
REST APIs often struggle with complex queries, forcing multiple round trips or inefficient data retrieval.
Solution: Implement standardized query parameter patterns, convert SQL to API requests, or consider GraphQL for complex data retrieval scenarios.
Version Control Nightmare
Managing API versions while maintaining backward compatibility creates massive overhead.
Solution: Pick a consistent versioning strategy:
- URI-based versioning (
/api/v1/resources
) - Header-based versioning (
Accept: application/vnd.company.v2+json
) - Query parameter versioning (
/api/resources?version=2
)
Wild West Standardization
REST's flexibility can lead to inconsistent API designs across your system.
Solution: Establish internal API guidelines and use documentation tools like OpenAPI to define and standardize specifications.
gRPC Implementation Hurdles#
gRPC delivers stellar performance, but comes with a few roadblocks that might trip you up without proper planning:
Browser Support Blues
Ever tried running gRPC directly in a browser? Spoiler alert: you can't. Browsers simply don't speak gRPC's native language because of HTTP/2 and Protocol Buffer requirements.
Solution: Implement gRPC-Web as a compatibility layer or create dedicated REST endpoints with JSON transcoding that act as translators for your browser clients.
The Learning Cliff
Developers who've spent years in REST-land often feel overwhelmed when diving into gRPC.
Solution:
- Start with targeted training sessions focused specifically on Protocol Buffer design
- Ease in with hybrid models rather than going all-in overnight
- Build an internal knowledge base with well-documented .proto examples that your team can reference
Debugging in the Dark
When things go wrong, the opaque binary Protocol Buffer format can leave you feeling like you're debugging with a blindfold on.
Solution:
- Equip your team with specialized tools like gRPC CLI and server reflection capabilities
- Develop habits of converting Protocol Buffers to readable JSON during troubleshooting
- Implement detailed logging with correlation IDs that track requests across services
Best of Both Worlds with REST and gRPC: A Hybrid Approach#
Why choose between chocolate and vanilla when you can have both? Many organizations implement hybrid architectures that leverage the strengths of both protocols.
Gateway Pattern: REST Outside, gRPC Inside#
One of the most brilliant hybrid patterns is using REST APIs for external communication while implementing gRPC for internal service-to-service interactions:
- External clients use familiar REST interfaces
- Internal microservices enjoy the mind-blowing performance benefits of gRPC
- Public-facing APIs remain accessible without special client libraries
This pattern typically employs an API gateway as the bridge between protocols, translating between REST and gRPC as needed. Understanding the features of an API Gateway is crucial when implementing this pattern.
Tools That Make Hybrid Architecture Shine#
Several tools make hybrid architectures not just possible but downright simple. These include Envoy, a proxy that supports gRPC-Web, and gRPC-Gateway, which generates RESTful endpoints from gRPC service definitions. A more recent project called gRPC API Gateway shows a lot of promise to be the next go-to solution for exposing and customizing REST endpoints from gRPC. Embracing a hosted API gateway can further simplify deployment and management.
When blending REST and gRPC, build consistency into every layer of your architecture. Start with Protocol Buffers as your single source of truth for data models, then extend this unified approach to authentication mechanisms that work seamlessly across both protocols.
Complete the picture with monitoring tools that track requests from end to end without losing visibility at protocol boundaries, all guided by clear design principles that treat your hybrid system as one cohesive whole rather than two technologies that merely coexist.
Future-Proofing Your API Strategy#
Making the right protocol choice isn't just about today's requirements—it's about building a foundation that can evolve with tomorrow's challenges.
HTTP/3, built on the QUIC protocol, offers major performance improvements over HTTP/2, especially for high-latency networks. This evolution impacts both REST and gRPC, with work underway to support HTTP/3 in gRPC implementations.
Client technologies constantly evolve across web, mobile, and IoT platforms. Your protocol choice should consider:
- Browser compatibility requirements
- Mobile client efficiency needs
- Emerging platforms like wearables and edge computing
As your application scales, performance requirements often evolve dramatically. Implement performance monitoring early to identify bottlenecks and determine if you might need to transition between protocols.
If you need to migrate between protocols, several approaches can minimize disruption:
- Parallel implementations: Maintain both REST and gRPC endpoints during transitions
- API gateway pattern: Abstract the underlying protocol from clients
- Versioning strategy: Implement clear versioning that accommodates protocol transitions
Making the Right Choice: A Decision Framework#
Choosing between REST and gRPC isn't about following trends—it's about finding the perfect fit for your specific project.
Step 1: Map Your Requirements to Protocol Strengths#
Identify your non-negotiable requirements and match them to the core strengths of each protocol:
- For REST: When you need broad client compatibility, simple human-readable formats, or ecosystem maturity
- For gRPC: When performance is critical, you need real-time streaming, or work in a polyglot microservices environment
Step 2: Assess Your Priority Matrix#
Create a priority matrix to evaluate which factors matter most for your project:
Factor | High Priority | Medium Priority | Low Priority |
---|---|---|---|
Performance | gRPC excels | Either works well | REST is sufficient |
Browser Support | REST recommended | Consider REST + gRPC-Web | gRPC with proxy |
Developer Experience | Choose familiar tech | Plan for learning curve | Invest in training |
Contract Enforcement | gRPC offers strong typing | Either with proper tooling | REST with OpenAPI |
Streaming Needs | gRPC's bidirectional | Consider alternatives | REST is adequate |
Step 3: Evaluate Your Team's Capabilities#
Be honest about your team's existing skills and capacity to learn new technologies, and consider your timeline—the learning curve of a new protocol might outweigh its technical benefits in the short term.
Step 4: Consider Your Integration Context#
Evaluate how your choice fits with your broader technology ecosystem:
- Who are your API consumers?
- What existing systems will this need to integrate with?
- Are there security or compliance requirements that favor one approach?
Step 5: Consider a Hybrid Approach#
Many successful organizations don't choose one protocol exclusively, using REST for public-facing APIs and browser interactions while implementing gRPC for internal communication where performance is critical.
The Bottom Line With REST or gRPC: Different Tools for Different Jobs#
REST and gRPC represent two different conversations about API design—one prioritizing accessibility, the other performance. REST offers that familiar, works-everywhere approach developers know well, while gRPC delivers speed that can transform your infrastructure metrics overnight.
Smart teams don't pick sides in this debate. They blend approaches strategically—REST for public endpoints where compatibility matters, gRPC for internal communication where milliseconds count. This gives you both universal accessibility and lightning-fast operations exactly where you need them.
Ready to optimize your APIs? Sign up for a free Zuplo account today and see how our platform supercharges your API management, whichever protocol you choose.