Securing API endpoints is crucial in today's digital landscape, and knowing how to secure API endpoints with TLS and SSL encryption is your best defense against the ever-growing threat landscape. TLS and its predecessor, SSL, create secure channels between API endpoints that protect data integrity, authenticity, and privacy – but there's a world of difference between implementing them correctly and leaving your digital doors unlocked.
Modern APIs need modern protection. While SSL laid the groundwork, TLS has evolved into the gold standard with beefier security features and faster performance. The current versions, TLS 1.2 and 1.3, stand guard against sophisticated cyber threats that older protocols simply can't handle.
Let's take a look at how these protocols work, why they matter, and exactly how to implement them properly across your API infrastructure.
- The Security Magic Behind TLS: How It Actually Works
- Why Your API Security Can't Wait: The Business Impact of Weak Protection
- TLS vs. SSL: Understanding What Really Protects Your APIs
- Implementing Bulletproof API Encryption: A Step-by-Step Guide
- Supercharging Your API Security: Beyond Basic Encryption
- Simplifying Security with API Gateways
- Scaling API Gateway Security for Enterprise Environments
- Mutual TLS: The Ultimate API Security Upgrade
- Real-Time Vigilance: Monitoring and Logging
- Securing Your Digital Future: Building Trust Through Strong API Protection
The Security Magic Behind TLS: How It Actually Works#
When your API communicates with clients, TLS creates a fortress around your data transmission through several brilliant security mechanisms working in harmony:
Handshake Protocol#
When a client connects to your TLS-secured endpoint, they initiate a handshake that establishes trust and security parameters. This includes:
- Negotiating protocol versions
- Selecting the strongest available cryptographic algorithms
- Authenticating the server (and sometimes the client, too)
Key Exchange#
Your client and server establish a shared secret key using asymmetric encryption methods like RSA or ECDHE – mathematically complex operations that ensure only the intended recipients can access the encryption keys.
Encryption#
Once the shared key is established, all further communications are protected through symmetric encryption using algorithms like AES, creating a virtually impenetrable vault around your data.
Data Integrity#
TLS verifies that every byte hasn't been tampered with using message authentication codes (MACs), essentially placing a tamper-evident seal on your data packets.
This security trifecta gives you complete protection: confidentiality through encryption, integrity through tamper detection, and authentication that verifies you're talking to legitimate systems.
Why Your API Security Can't Wait: The Business Impact of Weak Protection#
API security is a business imperative that directly impacts your bottom line and reputation. Here's why securing your endpoints matters more than ever:
The Threats Are Real (And Expensive)#
Your API endpoints face constant threats from sophisticated adversaries:
- Man-in-the-Middle Attacks: Attackers intercepting and potentially altering communications between your API and clients
- Data Breaches: Unauthorized access to sensitive information flowing through unprotected channels
- Unauthorized Access: Malicious actors bypassing authentication to access restricted resources
The consequences hit where it hurts most: data theft, financial losses, and reputation damage that can take years to rebuild—if ever. Implementing strong API authentication techniques is essential to prevent unauthorized access.
Trust Is Your Most Valuable Asset#
Strong API security is a powerful trust signal to users and partners. In a world where data breaches make headlines weekly, demonstrating your commitment to security through properly implemented TLS encryption tells clients their data is safe with you.
Compliance Isn't Optional#
From SOC2 Type 2 to industry-specific regulations, proper encryption is often legally required as part of the fundamentals. Your security approach and adherence to security and compliance policies are your ticket to operating in regulated industries where sensitive data changes hands.
Prevention Beats Recovery Every Time#
The fallout from a single security incident can cascade through your entire business:
- Financial impact from breach costs and operational disruptions
- Brand reputation damage that erodes customer trust
- Legal and regulatory consequences that compound the damage
- Operational standstill while you remediate the breach
TLS vs. SSL: Understanding What Really Protects Your APIs#
While people often use "SSL/TLS" as a catchall term, there's a world of difference between these protocols. Understanding these differences is crucial when learning how to secure API endpoints with TLS and SSL encryption.
The Evolution From SSL to TLS#
SSL (Secure Sockets Layer) was Netscape's original security protocol that gave us versions like SSL 2.0 and 3.0 – which are now about as secure as a paper lock on a bank vault. These outdated versions are vulnerable to attacks like POODLE and have been abandoned by modern applications for good reason.
TLS (Transport Layer Security) emerged as SSL's smarter, stronger successor. Current versions like TLS 1.2 and 1.3 have dramatically improved security architecture and performance optimizations that make SSL look prehistoric by comparison.
Security Showdown: Why TLS Wins Every Time#
When comparing these protocols, the security differences become starkly apparent:
- Encryption Algorithms: SSL relies on outdated algorithms like RC4 and 3DES that modern attackers can compromise. TLS employs robust encryption like AES-GCM and ChaCha20-Poly1305 that would take supercomputers centuries to crack.
- Message Authentication: SSL uses the now-vulnerable MD5 algorithm for integrity checks, while TLS implements robust options like SHA-256 that provide dramatically stronger protection.
- Key Exchange Methods: SSL primarily uses basic RSA and Diffie-Hellman approaches. TLS brings more sophisticated methods like ECDHE and ECC that provide perfect forward secrecy, ensuring today's traffic remains secure even if tomorrow's keys are compromised.
- Vulnerability Profile: SSL has more security holes than Swiss cheese, with multiple critical vulnerabilities that have led to real-world exploits. TLS was specifically engineered to patch these vulnerabilities, particularly in its newer versions.
Misconfigurations in SSL/TLS can also lead to issues such as HTTP 431 errors, affecting API communications.
Every major tech and security organization now recommends TLS over SSL due to its superior security posture and compliance with modern standards. Web servers and browsers have largely abandoned SSL support, making TLS the only credible choice for API security.
Implementing Bulletproof API Encryption: A Step-by-Step Guide#
Let's transform theory into practice with a straightforward approach to secure API endpoints with TLS and SSL encryption. Here's your roadmap to implementing robust protection across common server platforms.
Step 1: Get Your Certificates in Order#
Before touching your server configuration, you need legitimate SSL/TLS certificates from a trusted Certificate Authority (CA):
- Generate a Certificate Signing Request (CSR) using OpenSSL
- Select a reputable CA like DigiCert, GlobalSign, or AWS Certificate Manager for cloud deployments
- Submit your CSR and complete the verification process
- Download your certificate files once verification is complete
Step 2: Configure Your Server#
For Apache Servers:#
<VirtualHost *:443>
ServerName yourdomain.com
DocumentRoot /var/www/html
SSLEngine on
SSLCertificateFile /path/to/your-certificate.crt
SSLCertificateKeyFile /path/to/your-private-key.key
SSLCertificateChainFile /path/to/chain-bundle.crt
# Security configurations
SSLProtocol all -SSLv2 -SSLv3
SSLHonorCipherOrder on
</VirtualHost>
Enable SSL and restart:
sudo a2enmod ssl
sudo systemctl restart apache2
For NGINX Servers:#
server {
listen 443 ssl;
server_name yourdomain.com;
ssl_certificate /etc/nginx/ssl/your-certificate.crt;
ssl_certificate_key /etc/nginx/ssl/your-private-key.key;
ssl_trusted_certificate /etc/nginx/ssl/chain.crt;
# Recommended SSL settings
ssl_protocols TLSv1.2 TLSv1.3;
ssl_prefer_server_ciphers on;
ssl_ciphers ECDHE-RSA-AES256-GCM-SHA512:DHE-RSA-AES256-GCM-SHA512:ECDHE-RSA-AES256-GCM-SHA384:DHE-RSA-AES256-GCM-SHA384;
# HSTS header
add_header Strict-Transport-Security "max-age=63072000; includeSubDomains; preload";
# API endpoint configuration
location /api/ {
proxy_pass http://backend_server;
}
}
# Redirect HTTP to HTTPS
server {
listen 80;
server_name yourdomain.com;
return 301 https://$host$request_uri;
}
Verify and apply:
nginx -t
systemctl reload nginx
For Serverless Deployments:#
If you're running on AWS Lambda with API Gateway:
- Create or import certificates in AWS Certificate Manager
- Create a custom domain in API Gateway and connect it to your ACM certificate
- Set up base path mapping to your API
- Configure DNS to point to the API Gateway endpoint
Alternatively, you can ditch the clunky AWS API gateway and use Zuplo. It includes native support for AWS Lambda so you can deploy a secure REST API over your Lambdas in less than 5 minutes.
Step 3: Follow Security Best Practices#
No matter which platform you're using:
- Use strong certificates (minimum 2048-bit RSA keys or ECC certificates)
- Disable outdated protocols (SSL v2/v3, TLS 1.0/1.1)
- Implement HSTS headers to force secure connections
- Automate certificate renewal to prevent expiration outages
- Regularly test your configuration with tools like SSL Labs
- Force all traffic through HTTPS by redirecting HTTP requests
Supercharging Your API Security: Beyond Basic Encryption#
Basic TLS implementation is just the starting point. To create truly robust API security, you need to implement additional protective measures that build on your encryption foundation.
Enforcing HTTPS With No Exceptions#
HTTP Strict Transport Security (HSTS) transforms "optional" HTTPS into a mandatory requirement. Add this header to all API responses:
Strict-Transport-Security: max-age=31536000; includeSubDomains; preload
This tells browsers to only connect via HTTPS for a full year and extends protection to all subdomains. The 'preload' directive allows submission to browsers' built-in lists for protection even before the first connection.
Selecting Security-First Cipher Suites#
Your encryption is only as strong as your weakest supported cipher. Configure your server to use only the most robust options:
ssl_protocols TLSv1.2 TLSv1.3;
ssl_prefer_server_ciphers on;
ssl_ciphers ECDHE-RSA-AES256-GCM-SHA512:DHE-RSA-AES256-GCM-SHA512:ECDHE-RSA-AES256-GCM-SHA384:DHE-RSA-AES256-GCM-SHA384;
This configuration ensures your API only uses TLS 1.2/1.3 with the strongest available cipher suites.
Implementing Perfect Forward Secrecy#
Perfect Forward Secrecy (PFS) ensures that if your private key is compromised in the future, past communications remain secure. Enable it in Nginx with:
ssl_dhparam /etc/nginx/ssl/dhparam.pem;
ssl_ecdh_curve secp384r1;
Generate a strong Diffie-Hellman group:
openssl dhparam -out /etc/nginx/ssl/dhparam.pem 4096
Deploying Security Headers#
Security headers form your API's immune system against common web vulnerabilities:
X-Frame-Options: DENY
X-Content-Type-Options: nosniff
X-XSS-Protection: 1; mode=block
Content-Security-Policy: default-src 'self'
These headers prevent clickjacking, MIME type sniffing, cross-site scripting attacks, and enforce strict content security policies.
Simplifying Security with API Gateways#
Managing encryption for multiple endpoints can quickly become overwhelming. API gateways provide a centralized control plane that makes security management dramatically simpler and more consistent.
Why API Gateways Transform Security Management#
API gateways (ex. Zuplo) act as security control points, handling certificate management, authentication, and encryption in one place.
The biggest advantages include:
- Centralized certificate management: No more juggling certs across multiple servers
- Private key protection: Keys never leave the secure gateway environment
- Simplified TLS configuration: Point-and-click interface instead of config files
- Automated certificate renewal: Eliminate expiration-related outages
- Consistent security policies: Apply uniform protection across all endpoints
API Gateway Security Best Practices#
To maximize security with API gateways:
- Use only TLSv1.2 and TLSv1.3 with strong cipher suites
- Enable automated certificate lifecycle management
- Keep certificates in the gateway's secure storage
- Configure automatic HTTP-to-HTTPS redirection
- Enable Perfect Forward Secrecy and HSTS at the gateway level
- Regularly test your configuration with tools like SSL Labs
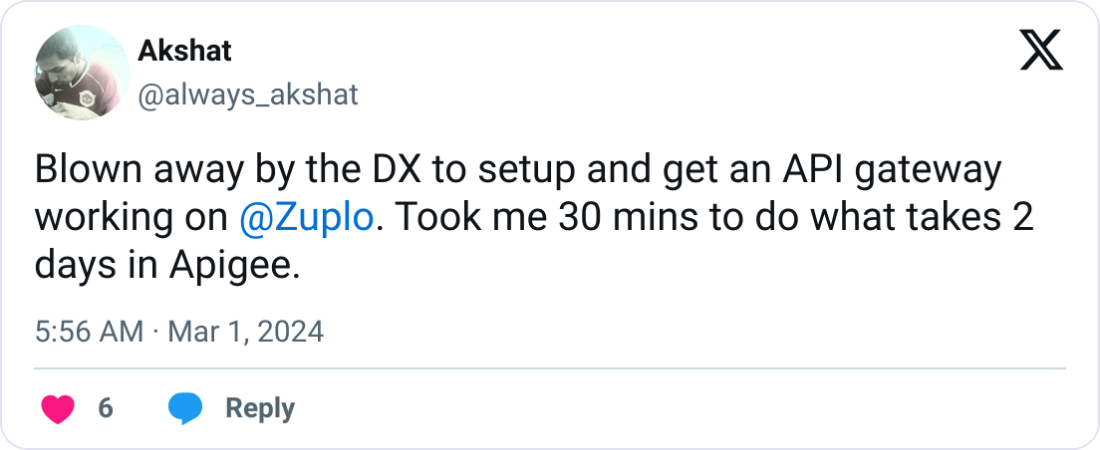
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreScaling API Gateway Security for Enterprise Environments#
Enterprise-scale API ecosystems require additional considerations beyond basic configuration.
Multi-Region Security Deployments#
Global organizations need consistent security across geographical boundaries. Multi-region API gateway deployments require:
- Certificate management across diverse regulatory environments
- Region-specific security policies that maintain baseline standards
- Synchronized configuration changes to prevent security drift
- Global monitoring with localized alerting thresholds
- Disaster recovery planning with security controls preserved
For multinational operations, consider:
- Regional variations in certificate requirements and trustchains
- Maintaining consistent security posture while respecting local regulations
- Cross-region traffic management with appropriate encryption levels
- Geographic DNS routing with security parameter verification
Automating Security at Scale#
Manual security management becomes impractical with dozens or hundreds of APIs. Automation is essential:
- Infrastructure-as-Code (IaC) templates to deploy consistent security configurations
- CI/CD pipelines that include security testing before deployment
- Policy-as-Code frameworks to enforce security standards programmatically
- Automated certificate rotation and renewal processes
- Scheduled security scanning with remediation workflows
- Configuration drift detection and automatic correction
Modern security automation tools allow teams to manage thousands of endpoints with stronger security than manual approaches could achieve with just a handful of APIs.
Mutual TLS: The Ultimate API Security Upgrade#
For APIs handling sensitive data or operating in high-security environments, standard TLS isn't enough. Mutual TLS (mTLS) creates true end-to-end verification where both client and server authenticate each other, making it one of the most secure API authentication methods.
How mTLS Transforms API Security#
In standard TLS, only the server proves its identity. With mTLS, the client must also present a valid certificate, creating a trust relationship that's extraordinarily difficult to compromise. This effectively blocks unauthorized access attempts and man-in-the-middle attacks before they begin.
According to GlobalSign, "Mutual TLS certificates, like Mutual SSL X.509, are the most effective and widely used digital certificates for APIs."
When mTLS Makes Sense#
While mTLS adds complexity, it's invaluable for specific scenarios:
- Microservices architectures: Ensures only authorized services can communicate with each other
- B2B APIs: Adds extra verification when sharing sensitive data with business partners
- IoT deployments: Verifies each device's identity to prevent rogue devices from accessing your APIs
- Financial services: Provides strong authentication for high-value transactions
Implementation Considerations#
Implementing mTLS requires careful planning and robust infrastructure:
Certificate Infrastructure Setup
Create a complete certificate management system:
- Establish your own Certificate Authority (CA) for internal client certificates
- Create separate certificate hierarchies for development, testing, and production
- Implement certificate revocation lists (CRLs) or OCSP responders
- Develop certificate policies defining requirements for issuance and renewal
- Build signing workflows with appropriate approval processes
- Create secure storage for root CA keys with hardware security modules (HSMs)
Server Configuration
Configure your servers to require and validate client certificates:
For Nginx:
server {
listen 443 ssl;
server_name api.example.com;
# Server certificate
ssl_certificate /path/to/server.crt;
ssl_certificate_key /path/to/server.key;
# Client certificate settings
ssl_client_certificate /path/to/ca.crt;
ssl_verify_client on;
ssl_verify_depth 2;
# Only allow TLS 1.2 and 1.3
ssl_protocols TLSv1.2 TLSv1.3;
# Access based on certificate validation
if ($ssl_client_verify != SUCCESS) {
return 403;
}
# Pass certificate information to backend
proxy_set_header X-SSL-Client-DN $ssl_client_s_dn;
proxy_set_header X-SSL-Client-Verify $ssl_client_verify;
}
For Apache:
<VirtualHost *:443>
ServerName yourdomain.com
DocumentRoot /var/www/html
SSLEngine on
SSLCertificateFile /path/to/your-certificate.crt
SSLCertificateKeyFile /path/to/your-private-key.key
SSLCertificateChainFile /path/to/chain-bundle.crt
# Security configurations
SSLProtocol all -SSLv2 -SSLv3
SSLHonorCipherOrder on
</VirtualHost>
For Kubernetes with Istio:
apiVersion: networking.istio.io/v1alpha3
kind: Gateway
metadata:
name: api-gateway
spec:
servers:
- port:
number: 443
name: https
protocol: HTTPS
tls:
mode: MUTUAL
serverCertificate: /etc/certs/server.crt
privateKey: /etc/certs/server.key
caCertificates: /etc/certs/ca.crt
Certificate Lifecycle Management
Create robust processes for the entire certificate lifecycle:
- Automated expiration monitoring with alerting
- Certificate renewal workflows with appropriate approvals
- Emergency revocation procedures for compromised certificates
- Rotation schedules for regular certificate updates
- Audit logging for all certificate operations
Client Integration Support
Help clients successfully implement mTLS:
- Develop client libraries that handle certificate management
- Create detailed documentation for various platforms and languages
- Provide sample code for certificate validation and handling
- Implement staging environments for testing certificate integration
- Establish support processes for certificate-related issues
Monitoring and Troubleshooting
Monitor the health of your mTLS implementation:
- Log all certificate validation failures with detailed error information
- Create dashboards showing certificate usage patterns
- Monitor certificate expiration dates across your ecosystem
- Implement alerting for unusual certificate validation patterns
- Develop runbooks for common certificate issues
Real-Time Vigilance: Monitoring and Logging#
Even the best encryption won't protect you if you can't see attacks in progress. Comprehensive monitoring and logging provide the visibility you need to maintain strong API security.
Essential API Security Logging#
Implement detailed logging that captures:
- Access patterns: Record every API request with client information, timestamps, endpoints accessed, IP addresses, HTTP methods, response codes, and user-agent details
- TLS/SSL activity: Log certificate issuance, renewal, revocation, and handshake failures
- Error events: Capture application errors, TLS handshake issues, and authentication failures
- Administrative changes: Track configuration updates, user/key creation, permission changes, and certificate rotations
Real-Time Security Monitoring#
Don't just collect logs – actively monitor them:
- Set up alerts for suspicious events like multiple failed logins or unusual API usage patterns
- Use machine learning to detect subtle anomalies that human analysis might miss
- Monitor certificate health to prevent expiration-related outages
- Implement rate limiting alerts to detect potential DoS attacks or API abuse, using appropriate API monitoring tools
SIEM Integration#
For enterprise environments, feed your logs into a Security Information and Event Management (SIEM) system to:
- Centralize security visibility across multiple APIs and systems
- Correlate events with threat intelligence feeds
- Perform long-term trend analysis to identify emerging threats
- Generate comprehensive security reports for compliance requirements, including tracking RBAC analytics metrics
Securing Your Digital Future: Building Trust Through Strong API Protection#
The shift from SSL to TLS represents a necessary evolution in our approach to security. Modern TLS versions offer vastly improved protection that makes them the only viable choice for API communications in today's threat landscape. As APIs continue to form the backbone of modern applications, security approaches must evolve alongside them.
Trends like zero-trust security models, mutual TLS, and preparations for post-quantum cryptography show us that staying current with security best practices isn't optional – it's essential for protecting your digital assets.
Are you ready to take your API security to the next level? Zuplo offers enterprise-grade API protection that deploys in seconds while maintaining the performance and developer experience your team expects. Sign up for a free account today and see how Zuplo can strengthen your API security posture while simplifying management across your entire API ecosystem.