SOAP APIs are still widely used in industries like finance and enterprise systems for their reliability and security. Even with the rise of REST APIs, SOAP remains essential for standardized and secure communication. This guide focuses on how to create and manage SOAP APIs using Python libraries like Zeep and Spyne.
Key Takeaways#
- SOAP Basics: SOAP uses XML for messaging with components like Envelope, Header, Body, and Fault.
- WSDL Files: Act as a contract defining operations, endpoints, and data formats.
- Python Tools: Libraries like Zeep simplify consuming SOAP services, while Spyne helps build them.
- Setup: Install Python, Zeep, and optional features like WS-Security and asyncio.
- Deployment: Tools like Zuplo streamline SOAP API management with features like security policies, rate limiting, and real-time monitoring.
SOAP Fundamentals and Setup Requirements#
Here's an overview of the main components and tools you'll need to work with Python SOAP APIs.
SOAP Message Structure#
SOAP messages are XML-based and consist of four main parts that ensure standardized communication:
Component | Purpose | Required |
---|---|---|
Envelope | Wraps the entire message and identifies it as SOAP | Yes |
Header | Holds optional metadata | No |
Body | Contains the main request or response data | Yes |
Fault | Provides error and status details (optional) | No |
Here's an example of a basic SOAP message structure:
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope
xmlns:soap="http://www.w3.org/2001/12/soap-envelope"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<!-- Header (Optional): Contains metadata -->
<soap:Header>
<auth:Credentials xmlns:auth="http://example.org/auth">
<auth:Username>user123</auth:Username>
<auth:Password>pass456</auth:Password>
</auth:Credentials>
<tx:TransactionID xmlns:tx="http://example.org/transaction">
TX-12345
</tx:TransactionID>
</soap:Header>
<!-- Body: Contains the actual request/response data -->
<soap:Body>
<m:GetStockPrice xmlns:m="http://example.org/stock">
<m:StockName>ACME</m:StockName>
</m:GetStockPrice>
</soap:Body>
</soap:Envelope>
WSDL Files and Services#
WSDL, or Web Services Description Language, serves as a contract between the service provider and the consumer. These XML documents define everything you need to interact with the service, including operations, endpoints, and data formats.
A WSDL file is made up of five key elements:
- Types: Specifies the data structures used in messages.
- Message: Defines input and output parameters.
- PortType: Lists the operations available.
- Binding: Links operations to specific protocols.
- Service: Provides endpoint details.
Here's an example of a basic WSDL for a calculator service:
<?xml version="1.0" encoding="UTF-8"?>
<definitions name="CalculatorService"
targetNamespace="http://example.org/calculator"
xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.org/calculator"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<!-- Data types used by the service -->
<types>
<xsd:schema targetNamespace="http://example.org/calculator">
<xsd:element name="AddRequest">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="a" type="xsd:int"/>
<xsd:element name="b" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
<xsd:element name="AddResponse">
<xsd:complexType>
<xsd:sequence>
<xsd:element name="result" type="xsd:int"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
</xsd:schema>
</types>
<!-- Messages for the service operations -->
<message name="AddInput">
<part name="parameters" element="tns:AddRequest"/>
</message>
<message name="AddOutput">
<part name="parameters" element="tns:AddResponse"/>
</message>
<!-- Port Type (Interface) -->
<portType name="CalculatorPortType">
<operation name="Add">
<input message="tns:AddInput"/>
<output message="tns:AddOutput"/>
</operation>
</portType>
<!-- Binding to SOAP protocol -->
<binding name="CalculatorSoapBinding" type="tns:CalculatorPortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="Add">
<soap:operation soapAction="http://example.org/calculator/Add"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<!-- Service definition -->
<service name="CalculatorService">
<port name="CalculatorPort" binding="tns:CalculatorSoapBinding">
<soap:address location="http://example.org/calculator"/>
</port>
</service>
</definitions>
Before diving into development, ensure you have the necessary tools and knowledge to work effectively with SOAP APIs.
Required Tools and Skills#
Software You'll Need:
- Python 3.x (latest stable release)
- The Zeep library (install it with
pip install zeep
) - Tools for processing XML
- A text editor or IDE that supports XML
Skills to Have:
- Basic Python programming
- A solid understanding of XML
- Familiarity with web services
- Knowledge of HTTP protocols
For practice, access sample WSDL files from public SOAP services or create your own. The Zeep library makes it much easier to handle SOAP web services by automatically generating Python code from WSDL files and offering a user-friendly API for calling remote methods.
Python Environment Setup#
Here's how to set up your development environment for working with Python and SOAP APIs:
Python Library Installation#
Start by installing Zeep and its optional features using pip
:
# Basic installation of Zeep
pip install zeep
# Add WS-Security support
pip install zeep[xmlsec]
# Add asyncio support
pip install zeep[async]
If you encounter issues with lxml
, use this specific version:
pip install lxml==4.2.5 zeep
Project Structure Setup#
Organize your project directory like this:
soap_api_project/
├── app.py # Initializes the server
├── controllers.py # Handles CRUD operations
├── models.py # Defines data models
├── views.py # Contains SOAP service methods
└── requirements.txt # Lists project dependencies
Your requirements.txt
file should include these dependencies:
zeep>=4.2.1
lxml>=4.2.5
spyne>=2.14.0
Environment Testing#
Verify your setup with this script:
from zeep import Client
from zeep.transports import Transport
def test_environment():
try:
# Create a test client using a sample WSDL
client = Client('http://www.webservicex.net/ConvertTemperature.asmx?WSDL')
print("✓ Zeep installation successful")
return True
except Exception as e:
print(f"✗ Environment setup issue: {str(e)}")
return False
if __name__ == "__main__":
test_environment()
Zeep simplifies working with SOAP APIs by automatically inspecting WSDL documents to generate the required service and type definitions. With your environment ready, you can now dive into building and interacting with SOAP APIs using Python.
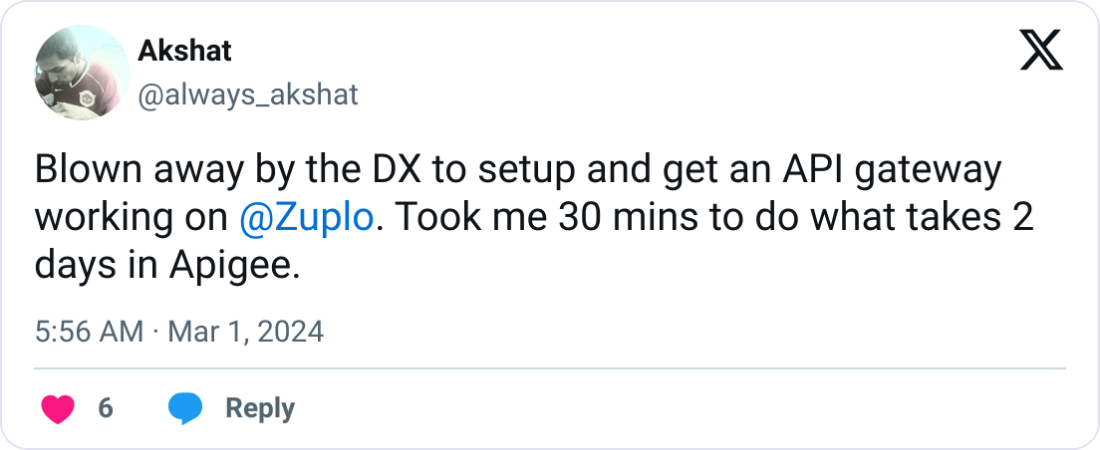
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreCreating and Using SOAP APIs in Python#
Using Zeep and Suds Clients#
Zeep is a Python library that simplifies working with SOAP services. Here's an example of setting up a basic SOAP client:
from zeep import Client
from zeep.transports import Transport
# Create a client instance
client = Client('http://www.soapclient.com/xml/soapresponder.wsdl')
# Make a service call
result = client.service.Method1('Zeep', 'is cool')
print(result)
To boost performance, you can add caching by configuring the transport layer:
from zeep.cache import SqliteCache
from requests import Session
session = Session()
cache = SqliteCache(path='/tmp/zeep-cache.db')
transport = Transport(cache=cache, session=session)
client = Client('http://www.soapclient.com/xml/soapresponder.wsdl', transport=transport)
Manual SOAP Request Creation#
If needed, you can manually construct SOAP requests with Zeep:
from zeep import Client
from zeep.wsdl.utils import etree_to_string
# Create the client
client = Client('http://www.soapclient.com/xml/soapresponder.wsdl')
# Build the request manually
node = client.create_message(
client.service, 'Method1',
message='Hello',
parameters={'param1': 'value1', 'param2': 'value2'}
)
# Convert to string and send
request_body = etree_to_string(node)
response = client.transport.post_xml(
'http://endpoint.url',
request_body,
headers={'Content-Type': 'text/xml'}
)
These methods offer flexibility in handling SOAP API calls while preparing for potential errors.
Building SOAP Services with Spyne#
To create your own SOAP service, the Spyne library is a great option. Here's how to set up a basic service:
from spyne import Application, rpc, ServiceBase, Unicode
from spyne.protocol.soap import Soap11
from spyne.server.wsgi import WsgiApplication
class WeatherService(ServiceBase):
@rpc(Unicode, _returns=Unicode)
def get_weather(ctx, city):
return f"Current weather in {city}: Sunny, 75°F"
# Create and configure the application
application = Application(
[WeatherService],
tns='weather.services',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11()
)
# Wrap the application with WSGI
wsgi_application = WsgiApplication(application)
Error Management and Testing#
Handling errors effectively is crucial in SOAP API interactions. Here’s an example of managing common exceptions:
from zeep import Client
from zeep.exceptions import Fault, TransportError
def safe_soap_call(wsdl_url, method_name, **kwargs):
try:
client = Client(wsdl_url)
method = getattr(client.service, method_name)
return method(**kwargs)
except Fault as soap_error:
print(f"SOAP Fault: {soap_error.message}")
return None
except TransportError as transport_error:
print(f"Transport Error: {str(transport_error)}")
return None
Zeep's popularity among Python developers has grown, with PyPI downloads increasing by 15% between March 2023 and April 2023, jumping from 120,000 to 138,000 monthly downloads [1].
For debugging, detailed logging can be very helpful. Here’s how to enable it:
import logging.config
logging.config.dictConfig({
'version': 1,
'formatters': {
'verbose': {
'format': '%(name)s: %(message)s'
}
},
'handlers': {
'console': {
'class': 'logging.StreamHandler',
'level': 'DEBUG',
'formatter': 'verbose',
},
},
'loggers': {
'zeep.transports': {
'level': 'DEBUG',
'propagate': True,
'handlers': ['console'],
},
}
})
SOAP API Management with Zuplo#
Zuplo API Gateway Features#
Zuplo's API gateway offers a robust solution for managing SOAP APIs. Operating across more than 300 global data centers, it delivers a typical latency of less than 50ms [4]. Zuplo's API gateway is fully programmable, allowing you to write and deploy custom functions to scale controls across your entire SOAP API, for example:
// Example of custom authentication logic in Zuplo
export async function validateSOAPAuth(
request: ZuploRequest,
): Promise<boolean> {
const soapHeader = request.headers.get("SOAPAction");
const apiKey = request.headers.get("X-API-Key");
if (!soapHeader || !apiKey) {
return false;
}
// Validate API key using your authentication service
return await validateApiKey(apiKey);
}
Zuplo seamlessly integrates with tools like DataDog, New Relic, and GCP Cloud Logging, providing real-time monitoring [4]. These integrations make deploying and managing SOAP APIs both fast and secure.
Zuplo Features for SOAP APIs#
Feature Category | Capabilities | Benefits |
---|---|---|
Security | API key auth, OAuth2, mTLS, IP allowlisting | Strong protection for SOAP endpoints |
Performance | Edge deployment, global traffic management | Lower latency and faster response times |
Monitoring | Real-time analytics with integrated tools | Immediate insights into API performance |
Development | TypeScript/JavaScript extensions, custom policies | Flexible and customizable API management |
Zuplo supports backends hosted on AWS, Azure, GCP, or private infrastructure [4].
Conclusion#
Summary of Key Points#
Creating SOAP APIs with Python provides effective solutions for industries like finance and enterprise systems, where secure and standardized communication is crucial. This guide highlighted how Python libraries like Zeep and Spyne simplify both creating and consuming SOAP APIs [3][2].
Here's what we covered:
- Python Tools: Libraries such as Zeep manage XML serialization and schema validation, while Spyne helps develop SOAP-based services [3][2].
- API Deployment: Tools like Zuplo can streamline the deployment and management of your SOAP APIs.
- Development Essentials: WSDL files serve as machine-readable documentation for your services.
These elements provide a solid starting point for implementing SOAP APIs effectively.
FAQs#
What are the main benefits of using SOAP APIs in industries like finance and enterprise systems?#
SOAP APIs offer several key advantages for industries such as finance and enterprise systems, where security, reliability, and transaction integrity are critical. SOAP's WS-Security ensures robust protection for sensitive data, making it ideal for handling financial information or confidential enterprise operations.
Additionally, SOAP provides built-in features like retry logic and error handling, ensuring reliable message delivery even in complex systems. It also supports ACID-compliant transactions, which are essential for maintaining data consistency in enterprise workflows. Thanks to its standardized protocols, SOAP ensures seamless interoperability across various platforms and programming languages, making it a trusted choice for large-scale, mission-critical applications.
How does the Zeep library make it easier to work with SOAP services in Python?#
The Zeep library simplifies working with SOAP services in Python by automatically interpreting the WSDL (Web Service Description Language) document. It generates the necessary code to interact with the SOAP server, making it easy to call services and handle the associated data types.
Built on top of lxml
and requests
, Zeep supports SOAP 1.1, SOAP 1.2,
and HTTP bindings. Its user-friendly interface allows developers to focus on
building their applications without worrying about the complexities of SOAP
communication, making it a powerful tool for both beginners and experienced
developers.
What are the best practices for managing and deploying SOAP APIs with Zuplo's API gateway?#
To effectively manage and deploy SOAP APIs using Zuplo's API gateway, follow these best practices:
- Optimize API Performance: Ensure your SOAP API endpoints are well-structured and optimized for performance. Use Zuplo's caching and rate-limiting features to improve response times and handle traffic spikes efficiently.
- Secure Your APIs: Leverage Zuplo's powerful authentication and authorization tools to protect your SOAP APIs. Implement measures like API keys, OAuth2, or JWT to ensure only authorized users can access your endpoints.
- Monitor and Debug: Use Zuplo's built-in monitoring tools to track API usage and performance metrics. Detailed logging and error tracking can help you quickly identify and resolve issues during development and production.
By incorporating these practices, you can ensure your SOAP APIs are robust, secure, and scalable for real-world applications.