OAuth custom scopes allow you to control API access with precision. They define specific permissions for API actions, enhancing security, guiding developers, and organizing resources effectively. Here's what you need to know:
- What are OAuth Scopes? Permissions that determine what parts of an API an app can access.
- Why Use Custom Scopes? Tailor access, improve security, and align permissions with your API structure.
- Examples:
users.read
: View user profiles.transactions.create
: Initiate financial transfers.articles.publish
: Publish content.
- How to Plan Scopes: Use clear naming conventions (e.g.,
resource.action
), define access levels, and document thoroughly. - Implementation Steps:
- Configure your authorization server with scope details.
- Link scopes to API endpoints (e.g.,
GET
requests requireread
scopes). - Validate scopes during the OAuth flow.
- Key Tips: Test thoroughly, monitor usage, and manage scope updates carefully to maintain security and performance.
Custom scopes improve API security and usability by granting only the necessary permissions for specific operations. Now, let’s dive deeper into how to plan, implement, and manage them.
Planning Custom Scopes#
When planning custom scopes, focus on creating consistent names, defining clear boundaries, and providing thorough documentation. This approach ensures OAuth remains secure and easy to manage.
Scope Naming Rules#
Choose a naming convention that clearly conveys the purpose and access level of each scope. A common and effective method uses dot notation to pair resources with actions:
- Resource.Action Format: Use lowercase letters separated by dots (e.g.,
users.read
,documents.write
). - Versioning: Add version indicators when needed (e.g.,
api.v1.users.manage
). - Action Hierarchy: Arrange actions from least to most privileged (e.g.,
files.list
,files.read
,files.write
,files.delete
).
Setting Scope Boundaries#
Design scope boundaries that strike a balance between security and usability. Each scope should grant only the permissions necessary for its intended function.
Access Level | Example Scope | Usage Purpose |
---|---|---|
Read-only | reports.view | Data retrieval |
Write | reports.create | Creating resources |
Manage | reports.manage | Full CRUD operations |
Admin | reports.admin | Administrative functions |
- Group related actions to align with your API’s structure and minimize complexity.
- Isolate high-risk operations into separate scopes for added security.
Once scope names and boundaries are established, document their purpose and usage in detail.
Scope Documentation#
Clear documentation helps developers use and implement scopes correctly. Include the following in your documentation:
- A detailed explanation of each scope's purpose
- Required permission levels
- Example scenarios and implementation guidance
- Dependencies or relationships between scopes
- Any rate limits or usage restrictions
Using OpenAPI specifications can keep your API documentation aligned with its actual implementation. This minimizes inconsistencies and ensures your API gateway setup matches what developers see in the documentation (you can use tools like Zuplo and Zudoku for this).
Setting Up Custom Scopes#
This section explains how to implement custom scopes within the OAuth flow, building on your scope planning.
Auth Server Setup#
To integrate custom scopes into your authentication flow, configure your authorization server with the following steps:
- Define Scope Details: Specify each scope's resource, permitted actions, permission levels, and usage limits in the server configuration.
- Set Validation Rules: Ensure requested scopes match predefined patterns to block invalid or unauthorized combinations.
- Token Configuration: Include approved scopes in access tokens for downstream verification.
Connecting Scopes to APIs#
Once your authorization server is set up, link the appropriate scopes to secure your API endpoints.
Endpoint Type | Example Scope | Required Configuration |
---|---|---|
Read Operations | users.read | Validate GET requests |
Write Operations | users.write | Verify POST, PUT, or PATCH |
Admin Functions | users.admin | Ensure full CRUD permissions |
System Operations | system.manage | Validate special privileges |
Your API gateway should validate every incoming request against these scopes before granting access to protected endpoints. Check out our full guide on securing your API with OAuth to learn more.
Scope Request Flow#
After defining and connecting scopes, manage them effectively during the OAuth flow:
- The client requests specific scopes during the authorization process.
- The authorization server checks these requests against the allowed scopes.
- The user reviews and consents to the requested permissions.
- Tokens containing the approved scopes are issued.
- The API gateway enforces permission checks for protected endpoints.
This process ensures developers have clear guidance on the required scopes for accessing each endpoint.
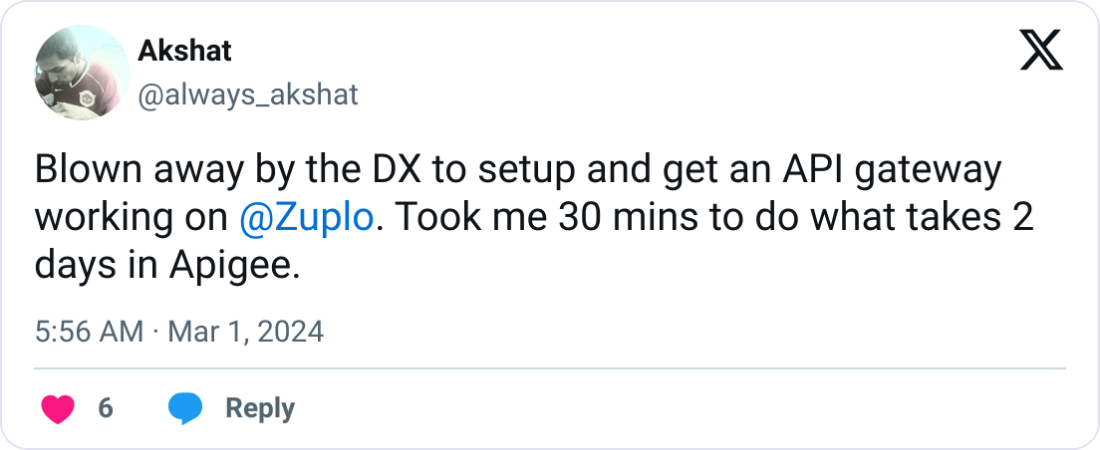
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreScope Management#
Once you've set up custom scopes and integrated them with your API, managing them effectively is key to maintaining security and performance. This involves handling updates, monitoring usage, and managing scope changes to keep your API running smoothly.
Scope Updates and Versions#
When updating scopes, aim to minimize disruptions. For smaller updates, you can simply add new permissions. Larger changes, however, may call for creating a new version of a scope. Maintaining backward compatibility during this transition ensures developers have time to adjust without breaking their integrations. This phased approach aligns with your initial token configuration, keeping security consistent as your API evolves.
Tracking Scope Usage#
Monitoring scope usage helps you understand how your API is accessed and can guide permission settings. Use an API monitoring tool to track metrics like scope request frequency and access patterns. Sharing this data through a developer portal allows your clients to better manage their API keys and improve their integrations.
Scope Changes and Removal#
When retiring or modifying scopes, clear communication is critical. Notify developers well in advance and provide detailed instructions for making updates. During the transition period, support both the old and new scope formats to ensure a seamless migration and avoid disruptions to your API's functionality.
Testing Custom Scopes#
Once your custom scopes are set up and integrated, it's crucial to thoroughly test their performance and reliability. Testing ensures your OAuth scopes work as intended, maintain API security, and help you catch issues before they affect users.
Scope Setup Tests#
Run automated tests to confirm your scope configurations. Focus on:
- Token generation and refresh processes using various scope combinations
- Validating scopes against protected endpoints
- Ensuring proper error handling for invalid or missing scopes
This approach ensures your scope configurations remain consistent and functional through deployments and updates. We have an end-to-end API testing guide that you might find useful for getting set up.
Common Scope Problems#
When working with custom scopes, certain problems tend to crop up. Addressing these early can save time and prevent headaches:
- Scope Bloat: Overly detailed scopes can complicate token management. Keep scope definitions concise and group related permissions where possible.
- Missing Validations: Ensure your authorization server correctly validates all scope combinations. Test edge cases to catch potential conflicts.
- Permission Gaps: Double-check that scope hierarchies don't unintentionally grant access. Carefully document and test scope relationships to avoid oversights.
Once you've tackled these common issues, assess performance to confirm everything runs smoothly.
Speed and Efficiency#
Keep an eye on key performance metrics:
- Time taken to generate tokens with complex scope combinations
- Speed of scope validation
- Authorization response times
- Token refresh efficiency
Your API gateway's monitoring tools can help track these metrics. For instance, Zuplo offers real-time analytics to pinpoint performance bottlenecks in your scope setup.
To boost performance:
- Cache validation results and keep scope strings short
- Use fast, efficient algorithms for parsing scopes
- Optimize token storage and retrieval processes
These steps will help ensure your custom scopes are both secure and efficient.
Summary#
We've covered the planning, setup, and management of custom OAuth scopes. Here's a quick recap with practical tips.
Benefits of Custom Scopes#
Custom OAuth scopes improve API security by offering detailed access control, reducing unnecessary permissions, and ensuring users only access the resources they need.
Key benefits include:
- Improved Security: Restricts access to specific endpoints, lowering the risk of unauthorized actions.
- Streamlined User Experience: Users interact only with what’s necessary, reducing confusion and potential errors.
These benefits lay the groundwork for successful implementation.
Tips for Implementation#
- Designing Scopes
Create a clear hierarchy that matches your API's functionality. Use concise, descriptive, and consistent naming conventions. - Documentation and Tracking
Clearly document each scope's purpose, associated permissions, related API endpoints, and any dependencies. - Managing Scopes
Implement a system to monitor usage, track adoption, identify redundant scopes, and plan updates effectively.
Zuplo's API gateway and real-time analytics can help you manage custom scopes, address bottlenecks, and strengthen security. Try it today for free!