Great APIs respond lightning-fast, handle traffic spikes effortlessly, and use computing resources efficiently. Poor ones? They're sluggish, error-prone, and crumble under pressure. Your API's performance directly impacts everything from user experience to your bottom line – when it runs smoothly, users stay happy and engaged; when it doesn't, you're facing system-wide issues that directly hit your business metrics.
What separates high-performing APIs from the rest isn't just good code – it's a comprehensive approach to design, implementation, and optimization. Let's explore the metrics that matter, common performance pitfalls, and proven strategies for transforming struggling APIs into speed demons that keep your users (and your business) thriving.
Today, we’ll talk about ways to identify what's actually happening with your underperforming API — and how to fix the problem. Let's go!
- Understanding API Health: Warning Signs and What to Watch Out For
- Detective Work: Finding the Real Performance Culprits
- Quick Wins: Immediate Performance Boosts
- Strategic Overhauls for Long-Term Performance Gains
- Cutting-Edge Techniques for Performance Mastery
- Building Your Performance Improvement Roadmap: From Assessment to Implementation
- The Evolving Landscape of API Performance
- The Path Forward: Performance as a Continuous Journey
Understanding API Health: Warning Signs and What to Watch Out For#
Before you can solve performance problems, you need to understand exactly what's happening with your API — which means tracking the right metrics, recognizing warning signs, and appreciating the real business impact of underperforming interfaces.
Critical Metrics That Reveal Your API's True Health#
To understand your API's performance, you need to track these specific metrics:
- Response time (latency): The time between sending a request and receiving a response. This is what users notice most.
- Throughput: The number of requests your API can handle within a given timeframe.
- Error rates: The percentage of requests that result in errors (4xx or 5xx status codes).
- Resource utilization: CPU, memory, and network usage needed to process requests.
Warning Signs Your API Is on Life Support#
Your API is likely struggling if you're experiencing:
- Painfully Slow Response Times – Especially during high-traffic periods when you need performance the most
- Wild Response Time Inconsistency – One request takes 200ms, the next takes 5 seconds, creating unpredictable user experiences
- Error Avalanches During Traffic Spikes – Your API shouldn't collapse just because user traffic increases
- Frequent Timeouts or Connection Failures – These are like check engine lights for your API
- Resource Consumption Gone Wild – When your servers are screaming for mercy
- Post-Deployment Performance Nosedives – New code shouldn't tank your API's performance
These symptoms typically point to problems in your database queries, network configuration, or application code. And those aren’t just coding niggles either. Poor API performance hits your business where it hurts the most:
- Revenue Drain - Those slow API responses? They're literally costing you money with every millisecond. Customers don't wait for laggy checkouts—they just leave.
- Conversion Killer - Watch those conversion rates nosedive when your API starts dragging. People have zero patience for slow experiences, especially on mobile.
- Trust Issues - Performance problems create reputation damage that lingers. Users don't forget the frustration of a system that constantly lets them down.
- Budget Disaster - Throwing more servers at performance problems is like burning cash. Your cloud bill explodes while the real issues remain unfixed.
- Developer Nightmare - Your talented team spends their days troubleshooting instead of building. That's expensive talent wasted on preventable problems.
In other words — the cost of ignoring performance far exceeds what you'd spend to build and maintain high-performing APIs.
Detective Work: Finding the Real Performance Culprits#
Finding the exact reason your API is crawling requires investigating three key areas: backend processing, network infrastructure, and fundamental design flaws.
Backend Processing Issues#
Backend problems are often prime suspects for slow API responses:
- Query Chaos — Your database might be a mess behind the scenes. All those complex joins and missing indexes are actively sabotaging your response times every single day.
- Code Clutter — Sometimes the problem isn't your infrastructure but the code itself. Memory leaks, blocking operations, and inefficient algorithms lurk in seemingly innocent functions, turning simple requests into performance nightmares.
- Resource Hogs — When your CPU utilization spikes and memory consumption goes through the roof for basic operations, something's seriously wrong under the hood. Your servers shouldn't break a sweat handling routine traffic.
- Unoptimized Data Handling — Moving too much data between services? Those bloated responses and unnecessary payload transfers are clogging your API's arteries, which becomes especially noticeable when mobile users with limited bandwidth try to connect.
- Connection Pool Problems — Database connections getting opened and closed constantly? That’s what’s adding unnecessary latency to every request.
Network and Infrastructure Concerns#
Even optimized code performs poorly if your network infrastructure is problematic.
- Latency Testing – Your API might be blazing fast on your development machine, but real users connect from across the globe. Those network delays add up fast, turning your "speedy" API into a frustrating experience for anyone not sitting next to your data center.
- DNS Resolution Issues – When was the last time you thought about your DNS setup? Probably never…until it became the silent bottleneck slowing down every single request before it even reaches your servers.
- Network Congestion — Your beautifully optimized API code means nothing when it's trying to squeeze through network bottlenecks. It's like having a sports car stuck in gridlock traffic—all that power with nowhere to go.
- Proxy Problems — Those extra network hops through load balancers, CDNs, and security layers? Each one adds latency that compounds into noticeable delays for end users.
- SSL Overhead — Security is non-negotiable, but poorly configured SSL can dramatically slow down connection establishment. That handshake process might be happening far more frequently than necessary.
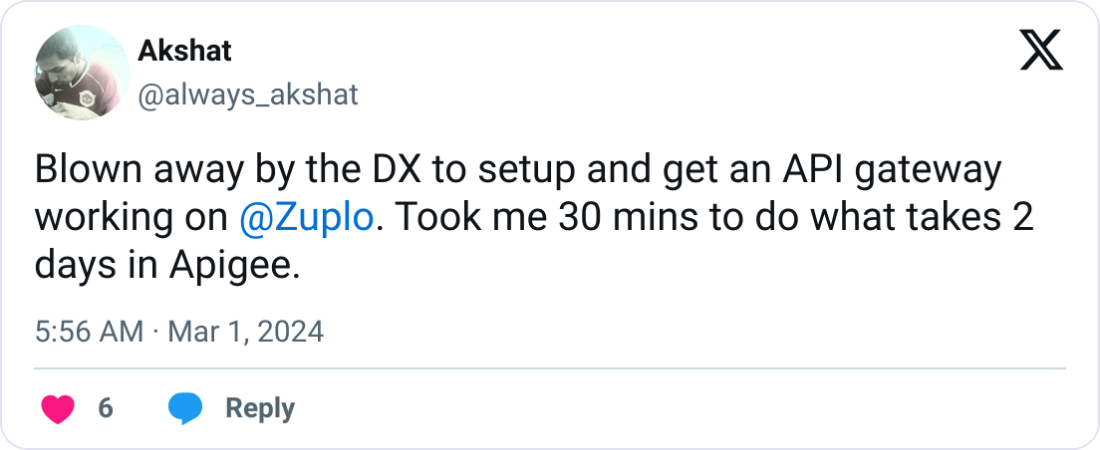
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreDesign and Architecture Flaws#
Sometimes, performance issues stem from fundamental architectural decisions:
- Network Congestion — Your beautifully optimized API code means nothing when it's trying to squeeze through network bottlenecks. It's like having a sports car stuck in gridlock traffic—all that power with nowhere to go.
- Proxy Problems — Those extra network hops through load balancers, CDNs, and security layers? Each one adds latency that compounds into noticeable delays for end users.
- SSL Overhead — Security is non-negotiable, but poorly configured SSL can dramatically slow down connection establishment. That handshake process might be happening far more frequently than necessary.
Quick Wins: Immediate Performance Boosts#
When your API needs immediate relief, these optimizations can deliver significant results without major code changes.
Caching Magic at Multiple Levels#
Caching can be a superpower when it comes to optimizing API performance:
Edge Caching
Caching API responses at
the network edge, closest to users, can significantly improve performance. This
isn't always the easiest thing to implement, so we created
this simple edge caching tutorial.
Hopefully you find it useful! Application-Level Caching
Use Redis or Memcached for data that's expensive to compute but doesn't change often.
Proper caching strategies can cut API response times by 70-90% for cached responses.
Shrinking the Data Pipeline#
While caching reduces server processing, optimizing what you send matters too:
- Compression – Enable GZIP or Brotli compression for 60-80% smaller payloads
- Payload Trimming – Only return data clients actually need
- Sensible Pagination – Break large responses into manageable chunks
- Efficient Formats – Consider Protocol Buffers or MessagePack for performance-critical applications
Traffic Management That Preserves Performance#
Implementing API rate limiting best practices ensures stability while keeping your API responsive:
- Tiered Rate Limiting – Different limits based on user tiers or subscription levels
- Request Prioritization – Keep critical operations running smoothly under load
- Throttling Instead of Blocking – Slow down responses rather than blocking them outright
- Global Rate Limiting – Stop traffic spikes at the edge before they reach your servers
These three strategies can deliver major performance improvements without changing your underlying architecture.
Strategic Overhauls for Long-Term Performance Gains#
When quick fixes aren't enough, these deeper solutions provide massive, lasting improvements.
Architecture Transformations That Deliver#
Revamping your API's architecture can transform performance:
- Microservices Magic – Break monolithic APIs into smaller, independent services that can scale independently, use the right technology for each component, isolate failures, and speed up development.
- Serverless Simplicity – Let cloud providers handle scaling with automatic provisioning, pay-per-use pricing, reduced operational overhead, and global deployment options
- API Gateways Empowerment – Implementing an API gateway can centralize and optimize API traffic, especially significant in modern technology sectors. For example, an API Gateway for AI can streamline requests and improve performance.
Database Transformations That Eliminate Bottlenecks#
Database issues are often silent performance killers. Here’s how to nip them in the bud:
- Structural Optimization – Implement proper indexing, normalize or denormalize based on access patterns, partition large tables, and create materialized views for complex queries.
- Strategic Database Migration – Consider specialized databases for particular needs (NoSQL for flexible schemas, time-series for metrics, etc.). An alternative is to use a serverless PostgreSQL REST API to streamline backend processing and improve scalability.
Asynchronous Patterns That Keep Things Flowing#
For operations that don't need immediate responses, you have two easy options:
- Event-Driven Architecture – Decouple components using message queues or event streams.
- Background Processing – Move resource-intensive tasks to worker processes with priority queues.
Cutting-Edge Techniques for Performance Mastery#
For those seeking the absolute performance frontier, these advanced approaches deliver exceptional results.
AI-Powered Optimization#
AI brings predictive capabilities to performance engineering:
- Smart Traffic Routing – Analyze real-time performance data to route traffic to the fastest endpoints
- Predictive Scaling – ML models predict traffic spikes before they happen
- Anomaly Detection – Spot issues before users notice them
- Automated Query Optimization – Identify and fix inefficient database queries automatically
Edge Computing Supercharges Response Times#
Moving API processing closer to users delivers dramatic performance gains when operating on the worldwide edge:
- Global Edge Distribution – Xata slashed its API latency by 50% using Cloudflare's CDN and edge computing
- Microservices at the Edge – Netflix's deployment of microservices via edge computing boosted API performance by 70%
- Serverless Edge Computing – Cloudflare Workers respond 50% faster than traditional cloud functions
- Edge Caching for Databases – Amazon's DynamoDB accelerator cuts read latencies from milliseconds to microseconds
Advanced Testing and Monitoring#
You can't improve what you don't measure. Here’s what we recommend keeping an eagle eye on:
- Distributed Tracing – Follow requests across your entire microservices ecosystem
- Global Synthetic Monitoring – Test from multiple worldwide locations to ensure consistent performance
- Chaos Engineering – Break things on purpose to find weaknesses before they impact users
- Real User Monitoring – Capture actual user experiences to focus optimization efforts
Building Your Performance Improvement Roadmap: From Assessment to Implementation#
Transforming API performance isn't about random optimizations or following generic best practices—it's about creating a structured, data-driven approach tailored to your specific challenges. Here's how to build an actionable performance improvement roadmap that delivers measurable results:
Phase 1: Assessment and Goal Setting#
-
Start by establishing where you stand and where you need to go:
- Comprehensive Baseline Measurement
- Capture current response times across different endpoints and load conditions
- Document throughput limits before performance degradation occurs
- Track error rates and their correlation with traffic volumes
- Map resource utilization patterns during peak and normal operations
- Analyze cache hit/miss ratios to identify optimization opportunities
- Specific, Measurable Performance Goals
- Replace vague targets like "improve API speed" with quantifiable objectives
- Example targets:
- "Reduce 95th percentile response time from 850ms to 300ms"
- "Support 3x current peak traffic without degradation"
- "Cut error rates during traffic spikes by 80%"
- "Reduce infrastructure costs by 30% while maintaining performance"
- Comprehensive Baseline Measurement
Phase 2: Prioritization and Planning#
With clear goals established, develop a prioritized plan of attack:
-
Impact vs. Effort Analysis
- Plot potential improvements on a matrix based on:
- Business impact (revenue, user experience, operational efficiency)
- Implementation effort (time, resources, complexity, risk)
- Focus first on high-impact, low-effort improvements—these "quick wins" build momentum
- Schedule high-impact, high-effort initiatives for dedicated project cycles
- Deprioritize or eliminate low-impact improvements regardless of effort
- Plot potential improvements on a matrix based on:
-
Phased Implementation Strategy
-
Develop a staged approach that delivers incremental value:
- Immediate (0-30 days): Implement non-invasive optimizations like caching, compression, and connection pooling
- Short-term (1-3 months): Deploy targeted improvements to specific bottlenecks identified in assessment
- Medium-term (3-6 months): Roll out infrastructure upgrades and moderate architectural changes
- Long-term (6+ months): Execute fundamental architectural transformations and advanced optimization strategies
-
Phase 3: Execution and Validation#
Implementation without measurement is just guesswork. Build validation into your execution plan:
- Continuous Monitoring Framework
- Deploy comprehensive observability tools before making changes
- Establish automated performance testing for each deployment
- Create dashboards tracking progress against defined KPIs
- Set up alerting for performance regression
- Iterative Improvement Cycles
- Implement changes in small, measurable increments
- Validate impact against baseline after each change
- Document effective approaches for future reference
- Adjust strategy based on real-world results
Phase 4: Organizational Alignment#
Technical excellence requires organizational support—ensure your performance initiatives have the backing they need:
-
Business Case Development
-
Translate technical metrics into business outcomes:
- Connect response time improvements to conversion rate increases
- Show how reliability enhancements reduce customer churn
- Demonstrate cost savings from more efficient resource utilization
- Calculate developer productivity gains from reduced firefighting
- Explore opportunities for monetizing APIs as a new revenue stream
-
-
Cross-Functional Buy-In
- Engage stakeholders beyond the engineering team:
- Product managers prioritizing feature roadmaps
- Finance teams approving infrastructure investments
- Leadership making strategic technology decisions
- Customer support handling performance-related issues
- Engage stakeholders beyond the engineering team:
Remember that your performance roadmap isn't just a technical exercise—it's a strategic business asset. By approaching optimization methodically and communicating outcomes in business terms, you'll secure the resources and support needed to deliver APIs that delight users and drive competitive advantage.
The Evolving Landscape of API Performance#
The world of API performance optimization continues to transform rapidly, with new technologies and approaches constantly reshaping what's possible for forward-thinking organizations willing to embrace change.
- Open Standards and Interoperability: The rise of standardized API specifications like OpenAPI and GraphQL has led to better tooling, improved documentation, and more consistent performance optimization approaches across the industry.
- Hybrid Deployment Models: Organizations are increasingly adopting flexible deployment strategies that combine the best aspects of on-premises, cloud, edge, and multi-cloud approaches to optimize for both performance and business requirements.
- Zero-Trust Security Without Performance Penalties: Modern API security approaches are integrating authentication and authorization directly into the request path while maintaining exceptional performance through innovations in token validation and distributed identity verification.
- Composable Architecture: The emergence of composable API architectures allows teams to build high-performance systems from specialized, purpose-built components that can be assembled and reassembled to meet changing business needs while maintaining optimal performance characteristics.
The key to maintaining high-performing APIs is continuous optimization—regularly reviewing metrics, embracing new technologies, and refining your approach based on evolving best practices. By implementing the strategies we've discussed, you'll deliver exceptional API performance that creates a competitive advantage through faster response times, lower infrastructure costs, happier customers, and higher conversion rates.
The Path Forward: Performance as a Continuous Journey#
API performance optimization isn't a one-time project—it's an ongoing commitment to excellence that requires consistent attention, measurement, and improvement. The organizations that truly excel understand that performance isn't just about technology; it's about creating exceptional experiences that delight users and drive business success.
Ready to transform your API performance from a liability into a competitive advantage? Zuplo's developer-friendly platform makes it easy to implement many of the optimization techniques we've discussed, from edge caching to traffic management, without complex infrastructure changes. Sign up for a free Zuplo account today and start delivering the lightning-fast API experiences your users deserve.