Security breaches continue to dominate headlines, even after decades of increased awareness. So, why does this keep happening despite best efforts? SQL injection remains one of the most dangerous threats to API security—a vulnerability that's existed for decades yet continues to plague organizations of all sizes. When successful, these attacks expose sensitive data, compromise systems, and cause significant financial and reputational damage.
It's time to protect your APIs from this persistent threat. Let's explore how SQL injection works, why it's particularly dangerous for APIs, and, most importantly, how to implement robust defenses to keep your applications secure.
What Is SQL Injection and Why Should You Care?#
So, first off, what even is SQL injection? SQL injection occurs when attackers insert malicious SQL code into application input fields. When these inputs aren't properly sanitized, attackers can manipulate your database queries to access, modify, or delete data they shouldn't have permission to touch.
This vulnerability typically happens when user-supplied input gets directly incorporated into SQL statements without proper validation. Consider this simple example of vulnerable code:
If an attacker enters ' OR '1'='1 in the username field, the resulting query becomes:
Since 1=1 is always true, this query returns all records from the users table, potentially granting access to administrative accounts. More destructive payloads like '; DROP TABLE users; -- could delete entire database tables if your application has sufficient privileges.
Types of SQL Injection Attacks#
In-band (Classic) SQL Injection#
The attacker sends malicious data through the same channel used for the attack. There are two common approaches:
- Error-based: Triggering database errors that reveal information about the database structure
- Union-based: Using the UNION SQL operator to combine results from the original query with results from an injected query
Blind SQL Injection#
When applications don't display database error messages or query results:
- Boolean-based: Sending true/false questions to the database and inferring answers from the application's response
- Time-based: Inserting commands that cause time delays when conditions are true, allowing data extraction without seeing direct output
Out-of-band SQL Injection#
When attackers can't use the same channel for both injection and retrieval, often involving DNS or HTTP requests from the database server.
The Real Cost of SQL Injection Vulnerabilities#
When SQL injection strikes, the consequences extend far beyond just technical issues. The true impact hits your business where it hurts most—your bottom line, your reputation, and your operational capability.
Data Exposure and Financial Impact#
SQL injection attacks target databases holding sensitive information—personal data, login credentials, financial records, business secrets, and even healthcare information. Once exposed, this data gets sold on dark web marketplaces, used for identity theft, or leveraged for sophisticated fraud schemes.
The financial consequences are severe. Organizations face immediate costs of investigating and remediating the breach, followed by legal fees, regulatory penalties (up to €20 million under GDPR), business downtime, and customer compensation. For smaller organizations, these combined costs can threaten their very survival.
Reputation Damage and System Disruption#
While systems can be patched, rebuilding a damaged reputation requires a much longer healing process. Negative media coverage spreads quickly, diminishing brand value and creating barriers to acquiring new customers. Relationships with partners and vendors become strained as they reassess the risks of association.
Attackers can also delete or corrupt critical database records and create unauthorized backdoor accounts, allowing them to return even after the initial vulnerability is discovered. This leads to operational disruption that affects your entire business—orders can't be processed, customers can't access services, and employees can't perform their jobs.
Essential API Protection Strategies#
Now that we understand the threat, let's tackle the practical defenses every API should have in place. These fundamental protection techniques—including implementing strong API authentication best practices—form the cornerstone of your security strategy and should be implemented before any other measures.
Parameterized Queries: Your First Line of Defense#
The single most effective defense against SQL injection is using parameterized queries. These ensure user inputs remain separate from SQL commands, preventing attackers from injecting malicious code.
Here's how they work in various languages:
Input Validation and Stored Procedures#
Validate all user inputs by implementing strict type checking for numeric fields, limiting input length, and using allowlists for acceptable characters. Apply context-specific validation for formats like email addresses and date ranges to catch malformed inputs before they reach your database. For example, implementing query parameter validation can help ensure inputs are properly sanitized. Implementing policies for secure query parameter handling can further ensure that user inputs are safely managed.
Stored procedures provide an additional security layer by abstracting SQL execution away from your application code. Database permissions can be limited to executing these pre-defined procedures rather than allowing direct table access, and complex SQL logic stays safely on the database side.
ORM Frameworks: Automated Protection#
Object-Relational Mapping frameworks like Hibernate (Java), SQLAlchemy (Python), and Sequelize (Node.js) typically use parameterized queries by default and abstract direct SQL interaction. These frameworks help eliminate common developer errors that might otherwise introduce vulnerabilities.
Advanced Security Measures#
While basic prevention techniques form your foundation, implementing advanced strategies and following API security best practices create multiple defensive layers that significantly strengthen your security posture.
Web Application Firewalls#
A WAF screens incoming HTTP requests for malicious SQL commands, sitting between your server and incoming traffic. For example, you can secure your API Gateway with WAF configurations such as AWS WAF and Shield. Keep your WAF updated as new exploits emerge, adjust settings to match your application's traffic patterns, and integrate WAF logs with your incident response process for quick threat detection. Configuring Cloudflare API security settings properly can further strengthen your defenses.
Least Privilege Principle#
Run databases, servers, and processes with only the privileges they truly require so a breach can't easily spread. Use non-privileged accounts where possible, regularly audit roles, and grant elevated permissions only temporarily when necessary.
API Gateway Security#
A robust API gateway provides multiple security benefits: rate limiting prevents brute force attacks, request validation catches malformed inputs before they reach your application, and traffic monitoring helps identify suspicious patterns. An effective gateway also enforces proper authentication and authorization for every request.
Database Activity Monitoring#
Track unusual query patterns, log all database activities for auditing, and set up alerts for suspicious behaviors like schema modifications or bulk data extraction. For critical systems, implement real-time monitoring to catch attacks as they happen rather than discovering breaches days or weeks later.
For more details on security strategies, refer to this Zuplo doc on security.
Finding and Fixing API Vulnerabilities#
Proactive detection is crucial—identifying weak points before attackers do saves time, money, and reputation. Following API monitoring best practices can help you stay ahead of potential vulnerabilities.
Automated Scanning Tools#
Several tools can help identify potential SQL injection vulnerabilities before attackers discover them:
- SQLMap: An open-source penetration testing tool that automates detection and exploitation
- OWASP ZAP: Comprehensive security scanning for multiple vulnerability types
- Acunetix: Commercial option with advanced SQL injection detection
- Burp Suite: Popular security testing framework with robust SQL injection checks
Manual Code Review and Penetration Testing#
While automated tools are valuable, manual code review identifies vulnerabilities that scanners might miss. Review all database interaction points in your codebase, looking carefully for dynamic SQL construction that could be vulnerable.
Regular penetration testing simulates real-world attacks by putting your defenses to the test. Include both authenticated and unauthenticated testing scenarios, test all API endpoints that interact with databases, and address all findings with priority given to the most serious vulnerabilities.
Database Hardening#
Beyond application-level defenses, proper database configuration forms your foundation of security. Disable unnecessary features and services, implement strong authentication, enable comprehensive logging, and keep database software patched and updated.
Encryption serves as your last line of defense. Encrypt sensitive data at rest using industry-standard algorithms, use TLS/SSL for all database connections, and consider column-level encryption for particularly sensitive information.
Implementing robust API logging practices can aid in tracking and identifying unusual query patterns.
SQL Injection in Modern Contexts#
As technology evolves, so do the potential attack vectors for SQL injection. As organizations move towards API-driven architectures, it's important to carefully convert SQL queries to API requests in a secure manner. Ensuring that security is integrated throughout the process is essential, especially when you generate APIs from databases.
Cloud and Microservices Environments#
In multi-tenant environments, one vulnerable API can potentially expose data belonging to multiple customers. When deploying to the cloud, effectively use cloud provider security features to add valuable additional protections that complement your custom security measures.
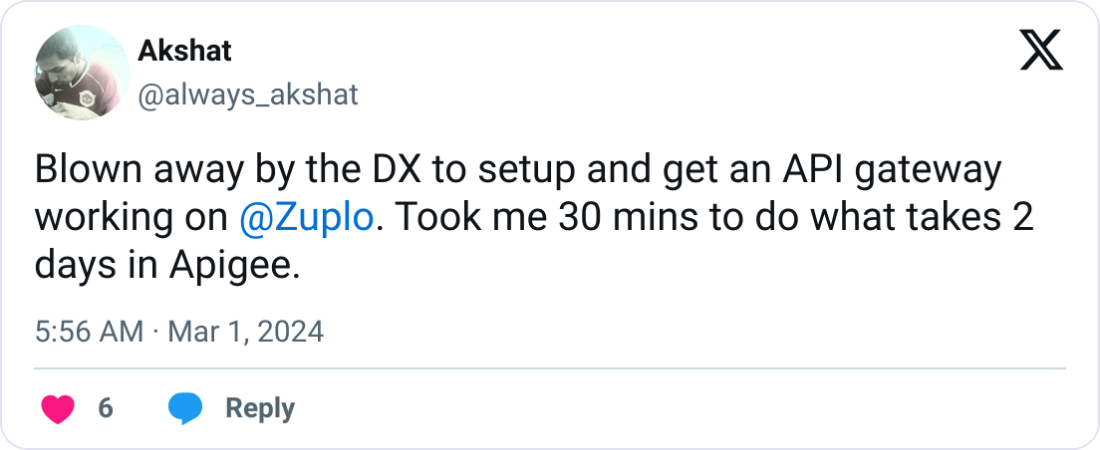
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreNoSQL Injection#
NoSQL databases aren't immune to injection attacks. For example, in MongoDB, vulnerable code might look like:
If the username parameter isn't properly sanitized, an attacker could submit:
This could potentially return all users by creating a query that matches any username greater than an empty string.
Protection requires schema validation to enforce data types, input sanitization to remove potentially malicious elements, and using parameterized queries with MongoDB drivers to separate code and data.
GraphQL API Security#
GraphQL introduces unique security challenges. Implement query depth and complexity limitations to prevent resource exhaustion attacks, ensure proper resolver-level authorization so users can only access permitted data, thoroughly validate all input arguments, and set resource limits to prevent denial of service attacks. Utilizing tools for GraphQL API security can further protect against injection vulnerabilities.
Implementing a Comprehensive Security Response Plan#
Even with solid prevention, incidents happen. A practiced response plan minimizes damage when attacks break through. Let's explore in detail how to build a robust response strategy:
Building Your Incident Response Team#
Before a crisis hits, establish clear roles and responsibilities:
- Incident Commander: The decision-maker who coordinates the overall response
- Technical Lead: Directs technical investigation and remediation efforts
- Communications Manager: Handles all internal and external communications
- Legal/Compliance Officer: Manages regulatory requirements and legal implications
- Documentation Specialist: Records all actions taken throughout the incident
Ensure team members understand their responsibilities through regular tabletop exercises that simulate various attack scenarios. Cross-train personnel so the response isn't crippled if key team members are unavailable.
Detection and Containment#
Effective incident response begins with rapid detection. Implement comprehensive monitoring solutions that can identify signs of SQL injection:
- Deploy database activity monitoring (DAM) systems to detect anomalous query patterns
- Set up alerts for unusual data access volumes or patterns
- Monitor application logs for signs of exploitation attempts
- Utilize network traffic analysis to identify suspicious outbound connections
Once an attack is detected, immediate containment becomes your priority:
- Isolate affected systems by restricting network access
- Disable compromised user accounts and API keys
- Implement temporary "read-only" mode for critical databases if appropriate
- Apply emergency patches or configuration changes to block the specific attack vector
- Preserve forensic evidence by capturing system state and logs before making changes
The goal at this stage isn't complete remediation but preventing further damage while preserving evidence for investigation.
Eradication and Recovery#
After containing the immediate threat, transition to thoroughly removing the vulnerability and restoring normal operations:
Root Cause Analysis:
- Trace the attack path from entry point to impact
- Identify the specific vulnerability that was exploited
- Determine if the same vulnerability exists elsewhere in your systems
Complete Vulnerability Removal:
- Fix the code vulnerability that allowed the injection attack
- Search for and remove any backdoors or malware installed by attackers
- Scan for similar vulnerabilities across your entire codebase
- Revoke and rotate all potentially compromised credentials and secrets
Secure Recovery:
- Restore systems from known clean backups where possible
- Implement additional controls before bringing systems back online
- Perform security validation testing before full restoration
- Gradually restore services with continuous monitoring for signs of persistent threats
Consider implementing a "secure rebuild" rather than simply patching the vulnerability. This approach means rebuilding systems from trusted sources rather than trying to clean compromised ones, significantly reducing the risk of overlooking hidden backdoors.
Communication Protocol#
Communication is often overlooked but crucial during a security incident. Establish protocols for different audiences:
Internal Stakeholders:
- Create communication templates for different incident severity levels
- Establish regular briefing schedules for executives and affected teams
- Use secure communication channels that can't be compromised by the attackers
- Share technical details only with those who need them
Customer Communications:
- Prepare notification templates that comply with regulatory requirements
- Establish timing guidelines—when to notify and with what level of detail
- Train customer service teams to handle inquiries about the incident
- Create a dedicated information page or portal for affected users
Regulatory Bodies:
- Document notification requirements for various jurisdictions where you operate
- Prepare report templates that meet regulatory standards
- Designate team members responsible for regulatory communications
- Establish relationships with regulatory contacts before incidents occur
Media and Public Relations:
- Designate authorized spokespersons with media training
- Prepare holding statements for initial responses
- Develop a strategy for social media monitoring and response
- Create a timeline for updates as the incident progresses
Remember that transparency builds trust, but must be balanced with operational security. Never share information that could help attackers expand their breach or target other organizations.
Documentation and Analysis#
Throughout the incident, maintain detailed records of:
- The attack timeline and all observed indicators of compromise
- Each action taken by the response team with timestamps
- All decisions made and their justifications
- Communications sent to various stakeholders
- Resources allocated and their effectiveness
After resolving the incident, conduct a thorough postmortem:
- Analyze detection time—could you have identified the attack sooner?
- Evaluate containment effectiveness—did you limit the damage?
- Review recovery time and process efficiency
- Assess communication effectiveness with all stakeholders
- Identify security gaps that allowed the attack to succeed
- Develop specific, actionable improvements to prevent similar incidents
Document lessons learned in a format that can be shared (anonymized if necessary) with your security team and incorporated into future training.
Testing and Refining Your Response Plan#
A response plan is only effective if it works in practice:
- Schedule regular tabletop exercises to simulate various SQL injection scenarios
- Conduct surprise drills to test real-world readiness
- Update your plan based on actual incidents and exercise findings
- Train new team members and provide refreshers for existing staff
- Review and update contact information and escalation procedures quarterly
Consider bringing in external security experts annually to evaluate your plan against current best practices and emerging threats.
Staying Ahead: Emerging SQL Injection Threats#
The security landscape is constantly evolving. Here are emerging threats and how to prepare for them:
AI-Powered Injection Attacks#
As attackers leverage machine learning, we're seeing more sophisticated SQL injection attempts. AI systems can now analyze your defenses and generate customized payloads specifically designed to bypass them, while automated bots test thousands of injection variants until finding one that works.
To defend against these threats, implement behavior-based detection systems and advanced WAFs with machine learning capabilities. Consider developing honeypot systems to study attack patterns and regularly update your defenses based on threat intelligence feeds.
Supply Chain SQL Injection#
Modern applications rely on dozens of dependencies, creating new attack vectors that bypass your direct defenses. Attackers target popular packages with backdoored updates, compromise development tools, and exploit vulnerabilities in third-party APIs to reach your data through trusted channels.
Protect your supply chain by implementing strict dependency scanning, using software composition analysis tools, and setting up private package repositories. Thoroughly audit third-party services before integration and deploy API gateways that sanitize inputs from external services.
Integration Testing for SQL Injection Protection#
Beyond unit testing, comprehensive integration tests are vital for SQL injection defense. Create automated test suites that simulate attacks against your entire API surface, not just endpoints you believe are vulnerable.
Test with different database configurations and include edge cases like double-encoded inputs in your scenarios. Integrate security testing into your CI/CD pipeline so every code change is automatically scanned for vulnerabilities before deployment.
Building a Security-First API Culture#
Technical defenses alone aren't enough. Building a culture of security is essential for sustainable protection:
Developer Security Training#
Invest in ongoing education for your development team with specialized training on secure coding practices for APIs. Regular workshops on SQL injection patterns and prevention techniques build practical skills that theoretical knowledge alone cannot provide.
Make security knowledge sharing a regular part of team meetings and conduct code review sessions specifically focused on database interactions. Recognition programs for developers who identify and fix vulnerabilities reinforce that security is everyone's responsibility.
Security Champions Program#
Designate security champions within development teams to serve as local security experts. Select interested developers who show aptitude for security concepts and give them dedicated time to stay current on emerging threats.
These champions bridge the gap between security professionals and developers, speaking both languages and helping translate security requirements into practical coding practices. Include them in architecture discussions and have them review high-risk code changes involving database access.
Metrics and Accountability#
Track security defects with the same importance as functional bugs and set specific team goals for reducing vulnerable code over time. Include explicit security criteria in code review checklists to ensure consistent evaluation.
Make security performance visible to leadership through dashboards and regular reporting. This visibility elevates security to a first-class concern in development processes and encourages teams to prioritize it alongside feature development.
Shift Left: Early Security Integration#
Incorporate security from the beginning of development by including specific security requirements in user stories and performing threat modeling during design phases. Create explicit security acceptance criteria for features that will be verified before completion.
Automate security checks in development environments and provide security-focused code templates that make secure implementation the path of least resistance. This "shift left" approach catches vulnerabilities when they're cheapest to fix—during design and development rather than after deployment.
Don't Let SQL Injection Sink Your APIs#
Let's face it—SQL injection isn't going away anytime soon. Your best defense is a solid offense: parameterized queries, thorough input validation, and layers of protection that catch what your code misses. The good news? These aren't rocket science tactics—they're practical steps any development team can implement starting today.
Want to skip the headache and secure your APIs faster? Zuplo's platform comes with SQL injection protection built right in. Our simple declarative policies handle the security heavy lifting while you focus on building great APIs. Give Zuplo a spin and see how much easier API security can be—your users (and your security team) will thank you.