JSON Web Tokens (JWT) are a secure way to authenticate API requests without relying on server-side sessions. They’re compact, stateless, and designed to ensure data integrity. Here's what you need to know:
- What is a JWT? A JWT is a token with three parts - header, payload, and signature. These components work together to verify the token's validity and prevent tampering.
- Why use JWT? It’s fast, scalable, and eliminates the need for server-side session storage. Plus, it supports cross-domain authentication and includes built-in expiration for added security.
- How does it work? The server generates a JWT after successful login. The client stores the token and includes it in future requests for authentication.
- Key features: Signature verification, expiration controls, and claims validation ensure tokens are secure and trustworthy.
For better security, store tokens securely (e.g., HttpOnly cookies) and avoid common mistakes like skipping validation or using weak keys.
Table of Contents#
- JWT Structure
- JWT Security Mechanisms
- Implementation Guidelines
- Video: What Is JWT and Why Should You Use JWT
- Summary
JWT Structure#
Core JWT Components#
A JSON Web Token (JWT) consists of three parts: the header, payload, and signature. These parts are encoded in base64URL format and separated by dots.
- Header: Contains metadata, including the token type and the signing algorithm.
- Payload: Includes the actual data, known as claims, being transmitted.
- Signature: Ensures the token's authenticity and confirms it hasn't been tampered with.
The header typically includes two fields:
"typ"
: Specifies the token type (always "JWT")."alg"
: Indicates the signing algorithm (e.g., "HS256", "RS256").
The payload contains claims, which are pieces of information about the user and metadata. Common claims include:
"iss"
: Identifies the issuer of the token."sub"
: Refers to the subject of the token."exp"
: Specifies the expiration time after which the token is invalid."iat"
: Indicates when the token was issued."aud"
: Defines the intended audience for the token.
Now, let's break down a JWT example to see how these components work.
JWT Sample Breakdown#
Here’s an example of a JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.
eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.
SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
This token can be broken down as follows:
Header (decoded):
{
"alg": "HS256",
"typ": "JWT"
}
Payload (decoded):
{
"sub": "1234567890",
"name": "John Doe",
"iat": 1516239022
}
The signature is generated by combining the encoded header and payload with a secret key, using the algorithm specified in the header. This process ensures the token’s integrity and confirms that it hasn’t been altered during transmission.
JWTs offer several advantages:
- Quick Validation: Servers can verify tokens without needing to query a database.
- Built-In Integrity: Tampering with the token invalidates the signature.
- Custom Claims: You can include additional claims in the payload for specific needs.
- Compact Design: The base64URL encoding makes tokens URL-safe and easy to handle.
JWT Security Mechanisms#
Authentication Steps#
JWT authentication typically involves these steps:
-
Initial Authentication: The user submits their credentials to the authentication server (ex. Auth0) for verification.
-
Token Generation: If the credentials are valid, the server generates a JWT containing key claims.
-
Request Processing: The client securely stores the JWT and includes it in the Authorization header of future requests. This allows the server to verify the token’s signature, expiration, and claims:
Authorization: Bearer [token]
These steps function alongside critical security measures outlined below.
Security Elements#
JWTs enhance API security through several important mechanisms:
Signature Verification
The signature ensures the token’s integrity using cryptographic algorithms:
Algorithm | Security Level | Common Use |
---|---|---|
HS256 | High | Internal services |
RS256 | Very High | Public APIs |
ES256 | Very High | Mobile apps |
Expiration Controls
JWTs include claims to manage their validity:
exp
(Expiration Time): Specifies the exact time the token expires.iat
(Issued At): Marks when the token was created.nbf
(Not Before): Indicates the earliest time the token is valid.
Claims Validation
Checking claims like iss
(issuer), aud
(audience), sub
(subject), and
jti
(JWT ID) strengthens token security by ensuring they meet expected values.
Payload Protection
While JWTs don’t encrypt payloads by default, sensitive data can be safeguarded
through:
- Including only essential information in claims.
- Using reference tokens for sensitive data.
- Setting short expiration times.
- Adopting secure key management practices.
For advanced security configurations, API developers typically implement JWT authentication within an API gateway (ex. Zuplo) so logic can be applied across an API catalog. Some advanced techniques not covered in this article include JWT scopes - which you can learn to verify in this guide.
Implementation Guidelines#
Signing and Verification#
JWT signing and verification are essential for securing APIs. Ensure private keys are stored securely - use environment variables or key management systems. Avoid exposing keys in your source code or client-side applications.
Select a signing algorithm that aligns with your security requirements:
Algorithm Type | Key Length | Best Use Case |
---|---|---|
HMAC-SHA256 | 256-bit | Internal services or single-server setups |
RSA-SHA256 | 2048-bit+ | Public APIs or distributed systems |
ECDSA-P256 | 256-bit | Mobile apps or low-resource environments |
Proper token management is the next step to safeguard API sessions.
Token Management#
Managing tokens effectively ensures secure API interactions. Here’s how:
Token Storage Recommendations
- Use HttpOnly cookies for web applications or secure keychains for mobile apps.
- Avoid storing tokens in
localStorage
orsessionStorage
to reduce the risk of XSS attacks.
Token Expiration Guidelines
- Set short lifetimes for access tokens (15–30 minutes).
- Use longer lifetimes for refresh tokens (7–14 days).
- Implement automatic token rotation to maintain security.
These methods work alongside JWT's built-in protections, such as payload encryption and signature validation.
Common Mistakes#
Steer clear of these common JWT implementation errors:
- Skipping Validation: Always verify the token's signature and claims to ensure authenticity.
- Using Weak Keys: Generate cryptographically strong keys with adequate length and randomness.
- Lack of Revocation Mechanisms: Set up a token blacklist or other revocation methods for compromised tokens.
- Revealing Error Details: Avoid exposing sensitive information in error messages. For example:
// Correct approach
return {
status: 401,
message: "Authentication failed",
};
// Incorrect approach
return {
status: 401,
message: "Invalid signature algorithm: HS512",
};
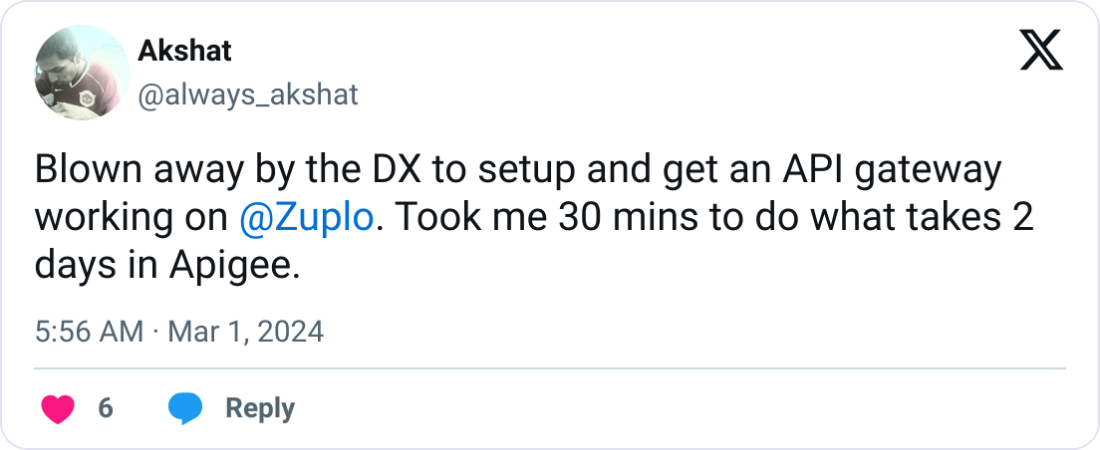
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreVideo: What Is JWT and Why Should You Use JWT#
In case reading isn't your learning style - here's a video summary of what we talked about above.
Summary#
JWT authentication protects APIs by using a standardized token structure that's designed to be tamper-resistant. To implement JWT effectively, focus on these three key areas:
- Token Signing: Select the right algorithm for your needs, such as RS256, HS256, or ES256.
- Validation Process: Always check the token's integrity, expiration, and claims before granting access.
- Lifecycle Management: Use proper expiration times and adopt token rotation strategies to maintain security.
Zuplo's API gateway simplifies adopting JWT authentication across your API by offering built-in integrations with your favorite identity providers including Auth0, Clerk, Cognito, Firebase, Okta, PropelAuth, Supabase.
Additionally, Zuplo is fully-programmable, allowing you to write code at the gateway to do stuff like smart API routing based on JWT contents, enforcing custom access controls, or using jose to validate JWTs for identity providers we don't have built-in support for. Try us out for free today!
Related Resources#
- If you're more familiar with API key authentication, check out our JWT vs API Key Auth guide
- jwt.io is a great playground to get used to working with JWTs