In today's microservices jungle, your API infrastructure needs a reliable traffic cop. Enter Envoy — the high-performance edge and service proxy that's revolutionizing how developers manage API traffic. Unlike legacy gateways that crumble under pressure, Envoy thrives in complex environments, giving you precise control where it matters most.
Whether you're juggling a handful of services or orchestrating hundreds of microservices, this guide will show you how to implement Envoy as your API gateway and transform your traffic management from chaotic to controlled.
- Why Envoy Leaves Other Gateways in the Dust
- Setting Up Your Envoy Gateway in Minutes
- Mastering Traffic Control with Envoy
- See Everything: Envoy's X-Ray Vision for Your APIs
- Fort Knox Your APIs with Envoy Security
- Common Envoy Implementation Pitfalls: Problems and Solutions
- Should You use Envoy as an API Gateway
- Wrapping Up
Why Envoy Leaves Other Gateways in the Dust#
Traditional/Legacy API gateways (ex. WSO2, Axway) were built for simpler times when monolithic applications ruled. Envoy, however, was born in the trenches of microservices complexity at Lyft and designed specifically for modern distributed systems.
What sets Envoy apart is its combination of performance and programmability:
- Dynamic service discovery that automatically adapts to your changing infrastructure
- Advanced load balancing with algorithms that distribute traffic precisely where it needs to go
- Comprehensive observability that shows you exactly what's happening in your system
- Battle-tested security feature, including TLS termination and authentication support
Its code-centric approach perfectly aligns with modern DevOps practices and infrastructure-as-code principles.
Setting Up Your Envoy Gateway in Minutes#
Getting Envoy running doesn't require a PhD in distributed systems. Here's the streamlined approach to get you started quickly:
Prerequisites#
- Kubernetes cluster (local or cloud-based)
- Docker installed on your system
- Basic knowledge of YAML configuration
Quick Installation Steps#
- Start a local Kubernetes cluster:
minikube start --driver=docker --cpus=2 --memory=2g
- Deploy Envoy Gateway:
helm install eg oci://docker.io/envoyproxy/gateway-helm --version v1.0.1 -n envoy-gateway-system --create-namespace
- Apply basic configuration:
kubectl apply -f https://github.com/envoyproxy/gateway/releases/download/v1.0.1/quickstart.yaml -n default
- Expose the service:
export ENVOY_SERVICE=$(kubectl get svc -n envoy-gateway-system --selector=gateway.envoyproxy.io/owning-gateway-namespace=default,gateway.envoyproxy.io/owning-gateway-name=eg -o jsonpath='{.items[0].metadata.name}')
kubectl -n envoy-gateway-system port-forward service/${ENVOY_SERVICE} 8888:80 &
- Test it works:
curl --verbose --header "Host: www.example.com" http://localhost:8888/get
For basic routing configuration, here's a minimal YAML that gets the job done:
route_config:
name: local_route
virtual_hosts:
- name: backend
domains: ["*"]
routes:
- match:
prefix: "/api/users"
route:
cluster: user_service
- match:
prefix: "/api/products"
route:
cluster: product_service
This simple configuration routes /api/users
requests to your user service and
/api/products
to your product service.
Mastering Traffic Control with Envoy#
Implementing sophisticated traffic routing with Envoy feels like having superpowers. Here are the key techniques that will transform your API management:
Path-Based Routing#
One of the foundational uses of an API gateway is to proxy an API, allowing you to route traffic based on URL paths to direct requests to different backend services:
routes:
- match:
prefix: "/api/users"
route:
cluster: user_service
- match:
prefix: "/api/products"
route:
cluster: product_service
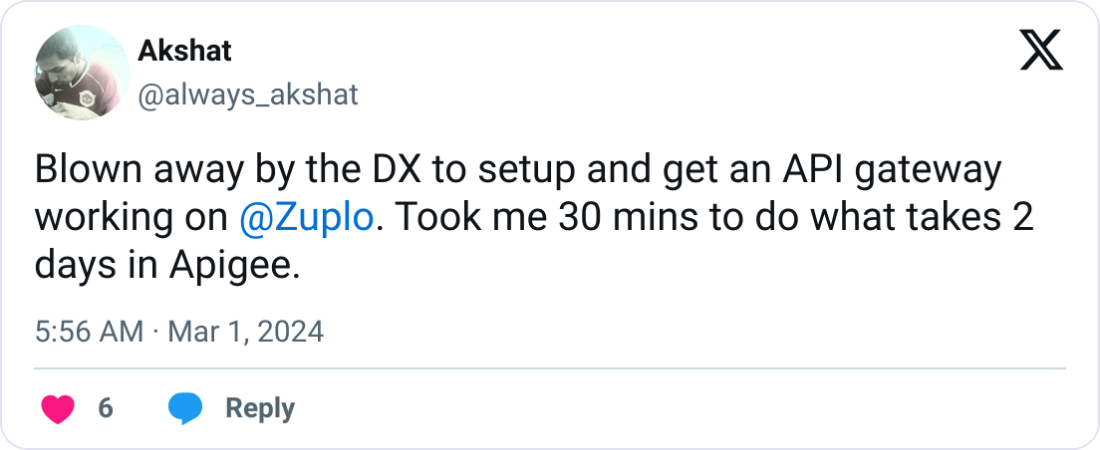
Over 10,000 developers trust Zuplo to secure, document, and monetize their APIs
Learn MoreHeader-Based Routing#
Perfect for API versioning or A/B testing:
routes:
- match:
prefix: "/api"
headers:
- name: "x-api-version"
exact_match: "v2"
route:
cluster: api_v2
- match:
prefix: "/api"
route:
cluster: api_v1
Weighted Routing#
Implement canary releases by gradually rolling out new versions:
routes:
- match:
prefix: "/"
route:
weighted_clusters:
clusters:
- name: new_version
weight: 10
- name: old_version
weight: 90
This configuration sends just 10% of traffic to your new version while keeping 90% on the stable version—perfect for testing changes without risking full-scale problems. Combining these techniques with advanced rate-limiting strategies enhances your control over traffic flows.
See Everything: Envoy's X-Ray Vision for Your APIs#
Flying blind with your APIs is a recipe for 3 AM incidents. Envoy's observability features give you visibility that prevents problems before they impact users:
- Detailed metrics on request rates, latency percentiles, and error counts
- Distributed tracing that follows requests across service boundaries
- Access logging with customizable formats to capture exactly what you need
Setting up basic access logging is straightforward:
access_log:
- name: envoy.access_loggers.file
typed_config:
"@type": type.googleapis.com/envoy.extensions.access_loggers.file.v3.FileAccessLog
path: "/dev/stdout"
For distributed tracing with Zipkin:
tracing:
http:
name: envoy.tracers.zipkin
typed_config:
"@type": type.googleapis.com/envoy.config.trace.v3.ZipkinConfig
collector_cluster: zipkin
collector_endpoint: "/api/v2/spans"
shared_span_context: false
When setting up monitoring dashboards, focus on the metrics that actually matter:
- Request rate to spot traffic spikes
- P50/P90/P99 latency to catch performance issues
- Error rates (4xx/5xx) to identify breaking changes
- Upstream cluster health to monitor backend services
Fort Knox Your APIs with Envoy Security#
In a world where API attacks are skyrocketing, Envoy provides robust security controls that protect your services from threats:
TLS Termination#
Secure all traffic with proper encryption:
filter_chains:
- transport_socket:
name: envoy.transport_sockets.tls
typed_config:
"@type": type.googleapis.com/envoy.extensions.transport_sockets.tls.v3.DownstreamTlsContext
common_tls_context:
tls_certificates:
- certificate_chain:
filename: "/etc/ssl/myserver.crt"
private_key:
filename: "/etc/ssl/myserver.key"
filters:
- name: envoy.filters.network.http_connection_manager
typed_config:
"@type": type.googleapis.com/envoy.extensions.filters.network.http_connection_manager.v3.HttpConnectionManager
stat_prefix: ingress_http
route_config:
name: local_route
virtual_hosts:
- name: local_service
domains: ["*"]
routes:
- match:
prefix: "/"
route:
cluster: example_service
http_filters:
- name: envoy.filters.http.router
Rate Limiting#
Implement API rate limiting to prevent abuse and DoS attacks:
filters:
- name: envoy.filters.http.ratelimit
typed_config:
"@type": type.googleapis.com/envoy.config.filter.http.rate_limit.v2.RateLimit
domain: some_domain
stage: 0
request_type: external
timeout: 0.25s
rate_limit_service:
grpc_service:
envoy_grpc:
cluster_name: rate_limit_cluster
Authentication#
Integrate with external auth services for robust identity verification and manage authentication and authorization. Envoy supports various API authentication methods:
filters:
- name: envoy.filters.http.ext_authz
typed_config:
"@type": type.googleapis.com/envoy.extensions.filters.http.ext_authz.v3.ExtAuthz
service:
server_uri:
uri: "http://localhost:10003/auth"
timeout: 0.1s
To enhance your API's security, integrate with external auth services for robust identity verification and secure API keys.
Common Envoy Implementation Pitfalls: Problems and Solutions#
Even the best tools have pitfalls. Here are the most common implementation challenges with Envoy and how to overcome them:
Configuration Complexity#
Problem: Envoy's YAML configurations can quickly become unwieldy as your routing rules grow.
Solution: Use Envoy Gateway with the Kubernetes Gateway API for more manageable configurations.
“Envoy Gateway extends the Gateway API by introducing enhancements for traffic management, security features, and custom extensions.”
Distributed Debugging Difficulties#
Problem: When requests traverse multiple services, identifying where problems occur can be challenging.
Solution: Implement comprehensive distributed tracing from day one. Set up Jaeger or Zipkin integration before you need it, not after problems arise. This lets you follow requests across service boundaries and pinpoint exactly where issues occur.
Resource Sizing Mistakes#
Problem: Over-provisioning wastes money; under-provisioning causes performance problems.
Solution: Start with conservative resource allocations, then use Envoy's detailed metrics to right-size based on actual usage patterns. Monitor CPU, memory, and connection counts to identify the right scaling parameters for your specific traffic patterns.
Upgrade Anxiety#
Problem: Upgrading Envoy in production can be nerve-wracking without proper testing.
Solution: Use blue-green deployments for Envoy upgrades. Maintain two parallel environments and shift traffic gradually using Envoy's own traffic management capabilities. This gives you immediate rollback ability if issues arise.
Authentication Integration Headaches#
Problem: Integrating with existing auth systems often causes unexpected complications.
Solution: Create a small proof-of-concept that focuses exclusively on auth integration before implementing in production. Test every authentication flow thoroughly, including error cases and token expiration scenarios.
Performance Tuning Complexity#
Problem: Default configurations rarely provide optimal performance for specific workloads, leading to unnecessary latency or resource usage.
Solution: Conduct targeted performance testing with production-like traffic patterns. Focus on optimizing buffer sizes, connection timeouts, and retry policies based on your actual traffic patterns rather than theoretical maximums. Create a performance testing framework that can validate configuration changes before deployment.
Certificate Management Overhead#
Problem: TLS certificate rotation and management become increasingly complex in large Envoy deployments, risking expired certificates and service disruptions.
Solution: Implement automated certificate management using tools like cert-manager for Kubernetes or HashiCorp Vault. Set up proactive monitoring for certificate expiration dates with alerts well before they become critical. Consider using a service mesh like Istio that handles certificate rotation automatically if you're operating at scale.
Should You Use Envoy As An API Gateway?#
Given the various problems mentioned above - you might be wondering if Envoy is actually a good solution for API gateway and API management.
On the API gateway side of things - I think it is a legitimate approach to the problems of load balancing, routing and basic security. On the rate limiting, authorization, and API management side - I'd say that Envoy fails to deliver, here's why...
Rate limiting and authorization are becoming increasingly intertwined with business logic as APIs evolve into products. This includes implementing RBAC in your API or applying dynamic rate limits based on properties like the API subscription plan the caller has. There is no one-size-fits-all solution for expressing the complex relationships between your business logic and API infrastructure - and Envoy's YAML syntax is particularly limiting. Additionally, pushing the logic back into each service defeats the purpose of having a gateway in the first place.
On the API management front - Envoy is quite lacking. Most API management tools (and many gateways these days) have support for OpenAPI and API cataloging to track all of your APIs and how they change. Some even generate a full developer portal with integrated authentication and analytics. This is not possible with Envoy as it sits separately from your services and has no distinct concept of APIs - so you are left in the dark on API behavior beyond basic routing.
Instead of using Envoy as an API gateway - I'd recommend you consider a dedicated solution like Zuplo.
Zuplo vs Envoy#
Below is a side-by-side comparison of Zuplo and Envoy as API gateways, covering routing, security, authentication, rate limiting, customization, and a few additional dimensions you might find useful.
Feature | Zuplo | Envoy |
---|---|---|
Routing | • OpenAPI-powered route builder • Built-in support for path/header routing with granular, code-driven controls | • L7 proxy with advanced routing (header, path, weight-based) |
Security | • Customizable distributed rate limiting • API security linting integration • Integrated with major WAF providers like Cloudflare | • Extensive filter chain (WAF-style via external modules) |
Authentication | • First-class JWT/OIDC flows via visual policy editor • API key management UI • Seamless integration with identity providers (Auth0, Okta, etc.) | • Extensive filter support (JWT, OAuth2 introspection, custom Lua/Wasmtime filters) • No native UI—requires config management or external control plane (e.g. Istio, Gloo) |
Rate Limiting | • Built-in rate-limiting policies with dashboard metrics • Quotas by key, IP, or custom headers | • Local and global rate limits via Ratelimit service (Envoy RLS) • You must deploy/configure an external RLS or use Istio’s adapter |
Customization | • Low-code policy editor for transformation, validation, caching • You can write Typescript anywhere within the request/response flow to integrate business logic into your API gateway | • Custom filters in C++, Lua, WASM (multiple languages) • Very steep learning curve but some flexibility |
Observability | • Dashboard with real-time metrics, logs, traces • Pre-configured Grafana/Prometheus exports | • Native stats (Prometheus), access logs, Tracing (Zipkin, Jaeger) • Requires external tooling assembly and config |
Performance | • Lightweight edge-optimized runtime • Latencies in single-digit ms for simple routes | • High throughput C++ proxy • Battle-tested at massive scale (Lyft, Google) |
Deployment Model | • SaaS, managed dedicated (run in your cloud), or self-hosted via Docker/Kubernetes • Deploy easily via Gitops (ex. Github actions) | • Self-hosted only • Can be standalone or as sidecar in service mesh |
Extensibility | • Plugin marketplace (community + first-party) • API for custom integrations | • Native support for Wasm and Lua • Vast ecosystem but you manage dependencies |
Community & Support | • Growing community focused on API management • Commercial support geared toward enterprise API teams | • Large OSS community, CNCF project • Wide adoption in service mesh and edge use cases |
If you’re looking for a turnkey, user-friendly API gateway with built-in policies and a polished UI, Zuplo is designed to get you up and running quickly. Envoy, on the other hand, offers maximum control and performance at the cost of a very steep learning curve and more configuration overhead. Your choice will hinge on whether you prioritize developer experience, time-to-market, easy tooling integration, API governance, and API productization (Zuplo) or low-level flexibility and scale in a self-managed ecosystem (Envoy).
Some folks even use both - with Envoy being used primarily for load balancing, while Zuplo handles the API management logic.
Ready to Tame Your API Traffic?#
Implementing Envoy as your API gateway transforms how your services communicate. Its performance, flexibility, and robust feature set make it ideal for organizations building modern, resilient API infrastructures.
While Envoy does have a learning curve, the investment pays off with an API gateway that grows with your needs and adapts to whatever challenges come next. Ready for an even simpler approach to API management? Sign up for Zuplo today and get the customizability of Envoy with a developer experience that feels like magic.